How to Design Scalable APIs: A Real-World Case Study
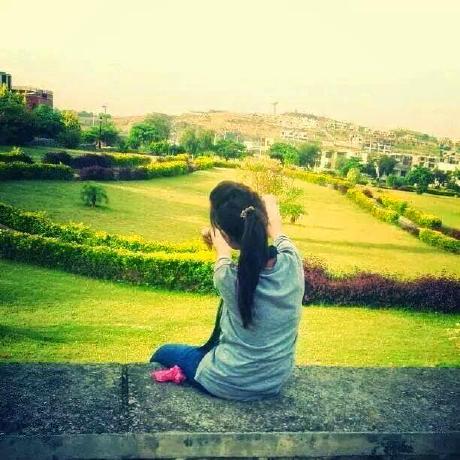
Let’s imagine you’re building a food delivery app like UberEats or Zomato. The system has to handle multiple actions such as browsing restaurants, placing orders, checking order status, and tracking delivery. At any given moment, thousands of users could be interacting with the system, placing simultaneous requests.
Designing an API that scales under such demand requires thoughtful architecture and techniques. Let's break down the key principles and strategies to design a scalable API for this use case, while keeping things easy to understand.
1. RESTful Principles for API Design
REST (Representational State Transfer) is a widely used architecture for building APIs, based on a few guiding principles. Here’s how you can apply them in your food delivery app:
Resources and Endpoints: Each API endpoint should represent a resource—something that your system manages, like restaurants, orders, or customers. RESTful APIs use HTTP methods like
GET
,POST
,PUT
, andDELETE
to operate on these resources.For example, you can define endpoints like:
GET /restaurants
– Get a list of restaurantsPOST /orders
– Place an orderGET /orders/{orderId}
– Get order statusPUT /orders/{orderId}/cancel
– Cancel an order
Statelessness: Each API call should be stateless, meaning the server doesn't store any information about the user's previous requests. All the information the server needs to fulfill the request should be included in the API call itself.
Example: Each API request must include authentication details (like a token) and necessary data (like user ID or order ID), so the server doesn't rely on session data from previous requests.
Uniform Interface: The API responses should follow a consistent structure. For instance, every successful response should return HTTP status
200 OK
with a standard JSON response body, like:{ "status": "success", "data": { "orderId": "12345", "status": "In Progress" } }
2. Rate Limiting
In a food delivery app, during peak hours (lunch/dinner times), there could be thousands of users trying to place orders simultaneously. If the API isn’t controlled properly, the surge in requests might overwhelm the system, leading to crashes or slow performance.
Rate limiting is a technique used to restrict the number of requests a user can make in a given time period. This helps prevent a single user or a bot from spamming the system and degrading the performance for others.
How it works: You could limit the API so that any user can only make 100 requests per minute. If a user tries to exceed that, the server will respond with an HTTP
429 Too Many Requests
status, like:{ "status": "error", "message": "Rate limit exceeded. Try again in 30 seconds." }
- Token Bucket Algorithm: A common rate-limiting technique is the token bucket algorithm. Each user is given a certain number of tokens (representing requests) per time window. Each time a request is made, one token is used up. Once tokens are depleted, the user must wait for the bucket to refill at a fixed rate. This helps to balance traffic during peak usage times.
Why is it important? Rate limiting ensures fair usage by all users and protects the API from malicious users or bots trying to abuse the service.
3. Versioning the API
As your app evolves, you might add new features or change how certain endpoints work. This could potentially break older versions of the app that users are still using. To prevent this, you need to version your API.
API versioning allows you to make changes to your API without affecting existing clients who might still be using older versions.
How to implement it: You can include the version number in the URL:
GET /v1/restaurants
– Version 1 of the endpointGET /v2/restaurants
– Version 2 of the endpoint with improved features (e.g., now supports location-based filtering)
Backward compatibility: When you release new features or change how a particular resource is handled, ensure that older API versions continue to work, allowing users to slowly migrate to the latest version. This ensures a seamless user experience without forcing app updates.
4. Scalability Techniques
To handle thousands of requests simultaneously without crashing or slowing down, here are some key techniques:
Load Balancing: Spread incoming API traffic across multiple servers using a load balancer. If one server becomes too busy, the load balancer can route requests to another, ensuring that no single server is overwhelmed.
Example: During a flash sale in your app, thousands of users may attempt to place orders. A load balancer can distribute these requests to multiple servers, ensuring everyone’s requests are processed efficiently.
Caching: Not all data changes frequently. For example, restaurant menus may not change every second, so you can cache these responses to reduce load on the database.
How it works: When a user requests the list of restaurants, instead of querying the database every time, the API can serve a cached response, which speeds up the process and reduces database load.
Use HTTP headers like
Cache-Control
to specify caching rules:Cache-Control: max-age=3600 // Cache response for 1 hour
This ensures that repeated requests for the same data don't unnecessarily tax the server.
Database Optimization: When dealing with thousands of users, poorly optimized database queries can become a bottleneck.
Use techniques like database indexing to speed up common queries (e.g., finding restaurants by location).
Implement pagination for large datasets. For example, when showing a list of 1,000 restaurants, don’t return them all at once. Instead, return 20 at a time using pagination parameters like:
GET /restaurants?page=1&limit=20
5. Error Handling
A well-designed API should gracefully handle errors and provide meaningful messages to clients. Error handling is critical when scaling because different types of errors (e.g., rate limit exceeded, invalid input) need to be addressed clearly.
For instance, if a user tries to place an order at a restaurant that’s no longer available, the API should return an appropriate error:
{ "status": "error", "message": "Restaurant not found", "code": 404 }
For validation errors, like missing fields in the order request, return a 400 Bad Request with details about what went wrong:
{ "status": "error", "message": "Invalid request: Missing 'address' field", "code": 400 }
Clear and consistent error responses are especially important when dealing with high volumes of traffic because they allow clients to handle errors and retry appropriately.
6. Security Considerations
When dealing with user data, especially financial and personal information (like addresses, payment details), ensuring your API is secure is critical.
Authentication: Use OAuth2.0 or JWT tokens to authenticate API requests. This ensures that only valid users with the correct tokens can access the API.
Example: Each request to
POST /orders
must include a validAuthorization
token in the header:Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR...
HTTPS: Always use HTTPS to encrypt data being transmitted between the client and the server. This protects sensitive information (like credit card numbers) from being intercepted by attackers.
Summary
To design a scalable API for a food delivery app, consider:
Applying REST principles to structure resources and interactions.
Implementing rate limiting to protect the system from overload.
Using API versioning to manage changes without disrupting users.
Scaling the system with load balancing, caching, and database optimization.
Providing meaningful error handling and ensuring robust security practices.
By considering these elements, your API will be able to handle high traffic volumes, adapt to changes, and provide a seamless user experience.
Subscribe to my newsletter
Read articles from Sriza Sen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
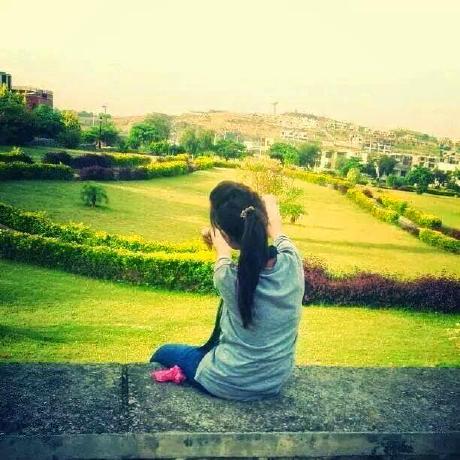