The Starfish’s Search
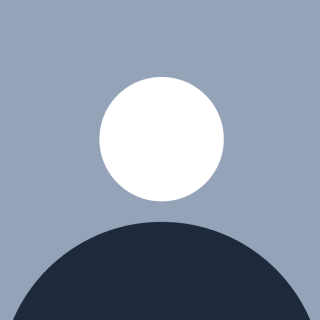
Table of contents

Imagine a beach where a starfish is carefully selecting shells, one at a time, from a pile. The starfish picks up each shell, compares it with the others, and places it in the correct spot before moving on to the next shell. This method is known as Selection Sort, and it works by repeatedly finding the smallest (or largest) element from the unsorted portion and swapping it with the first unsorted element.
In this article, we’ll explore how Selection Sort works, optimize it, and tackle some interesting challenges. By the end, you’ll have a deep understanding of how this methodical process sorts elements and where it’s best applied.
Simple Selection Sort – The Starfish’s Method
Selection Sort 101 – Finding the Smallest Element
Selection Sort works by selecting the smallest element from the unsorted portion of the list and swapping it with the first unsorted element. It’s a slow but steady process, much like a starfish methodically sorting shells on the beach.
Step-by-Step Example with Code
Let’s take a list of shell sizes: [7, 3, 9, 1, 5]
.
First Pass:
The starfish starts by finding the smallest shell. It compares each shell in the list and finds that1
is the smallest. The starfish swaps1
with7
, the first shell in the list.
List becomes:[1, 3, 9, 7, 5]
Second Pass:
The starfish now looks at the remaining unsorted portion[3, 9, 7, 5]
. The smallest shell is3
, which is already in the correct spot, so no swap is needed.
List remains:[1, 3, 9, 7, 5]
Third Pass:
The starfish moves on to the next unsorted portion[9, 7, 5]
. It finds that5
is the smallest, so it swaps5
with9
.
List becomes:[1, 3, 5, 7, 9]
Fourth Pass:
Finally, the starfish checks the remaining unsorted portion[7, 9]
. The smallest element is7
, so no swap is needed.
List remains:[1, 3, 5, 7, 9]
After four passes, the starfish has successfully sorted all the shells!
Code Snippets for Simple Selection Sort
def selection_sort(arr):
n = len(arr)
for i in range(n):
min_idx = i
for j in range(i+1, n):
if arr[j] < arr[min_idx]:
min_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
return arr
# Example usage
shell_sizes = [7, 3, 9, 1, 5]
print(selection_sort(shell_sizes))
function selectionSort(arr) {
let n = arr.length;
for (let i = 0; i < n; i++) {
let minIndex = i;
for (let j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
[arr[i], arr[minIndex]] = [arr[minIndex], arr[i]];
}
return arr;
}
// Example usage
let shellSizes = [7, 3, 9, 1, 5];
console.log(selectionSort(shellSizes));
#include <iostream>
using namespace std;
void selectionSort(int arr[], int n) {
for (int i = 0; i < n-1; i++) {
int min_idx = i;
for (int j = i+1; j < n; j++) {
if (arr[j] < arr[min_idx]) {
min_idx = j;
}
}
swap(arr[min_idx], arr[i]);
}
}
int main() {
int shellSizes[] = {7, 3, 9, 1, 5};
int n = sizeof(shellSizes) / sizeof(shellSizes[0]);
selectionSort(shellSizes, n);
for (int i = 0; i < n; i++)
cout << shellSizes[i] << " ";
return 0;
}
Optimizing Selection Sort – Can We Speed Up the Starfish?
Is There a Better Way to Select?
Selection Sort is inherently simple but not very efficient for large datasets. Unfortunately, there are no major optimizations that drastically improve its time complexity. However, we can look at two-way selection sort to reduce the number of swaps.
In two-way selection sort, we select both the smallest and the largest elements in each pass, placing them in their correct positions simultaneously. This can reduce the number of iterations.
Step-by-Step Example of Two-Way Selection Sort
Let’s use the same list [7, 3, 9, 1, 5]
:
First Pass:
The starfish selects both the smallest shell (1
) and the largest shell (9
). It swaps1
with7
and9
with5
.
List becomes:[1, 3, 5, 7, 9]
Second Pass:
The remaining list[3, 5, 7]
is already sorted, so no further swaps are needed. The sorting is complete in just two passes!
Python Code for Two-Way Selection Sort:
def two_way_selection_sort(arr):
n = len(arr)
for i in range(n//2):
min_idx = i
max_idx = i
for j in range(i, n-i):
if arr[j] < arr[min_idx]:
min_idx = j
if arr[j] > arr[max_idx]:
max_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
arr[n-i-1], arr[max_idx] = arr[max_idx], arr[n-i-1]
return arr
# Example usage
shell_sizes = [7, 3, 9, 1, 5]
print(two_way_selection_sort(shell_sizes))
Time and Space Complexity – Understanding Selection Sort’s Efficiency
Time Complexity:
Worst Case (O(n²)): Selection Sort always has to scan through the entire list to find the smallest element, resulting in
n × n
comparisons, even if the list is already sorted.Best Case (O(n²)): Unlike some other algorithms, Selection Sort doesn’t become faster on sorted lists. It still has to perform the same number of comparisons.
Space Complexity:
- Space Complexity (O(1)): Selection Sort only uses a small amount of extra space for swapping elements, making it memory-efficient.
Drawbacks and Real-World Applications
Drawbacks of Selection Sort
Inefficiency for Large Datasets: Selection Sort performs O(n²) comparisons even in the best-case scenario, making it inefficient for large datasets.
More Comparisons Than Necessary: Unlike other algorithms, Selection Sort doesn’t take advantage of already sorted portions of the list.
Real-World Applications of Selection Sort
Small or Nearly Sorted Data: Selection Sort works well on small datasets where its inefficiencies aren’t a major concern.
Choosing from a Collection: If you need to select a specific number of the smallest (or largest) elements from a list, Selection Sort can be useful.
Challenge Time – Test Your Skills!
Challenge: Sorting Coral
You’re sorting a collection of coral by size. Use Selection Sort to arrange them:[9, 4, 7, 2, 8]
.Challenge: Choosing the Best Seashells
You’ve collected seashells and want to choose the top three largest ones. Can you use Selection Sort to find the top three largest shells from the list:[5, 2, 9, 1, 7, 6]
?
Think you’ve mastered Selection Sort? Try the challenges and share your solutions in the comments!
Conclusion: The Starfish’s Slow but Steady Method
The starfish may be slow, but with patience and a methodical approach, it manages to sort all the shells. Selection Sort is reliable for smaller datasets and situations where simplicity is key. While not the fastest, it’s a valuable sorting method to know. Staytuned as we continue our sorting journey.
Ready for the next step? Subscribe to stay updated on the next algorithm: Merge Sort!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by