Rust Programming Language: An Overview
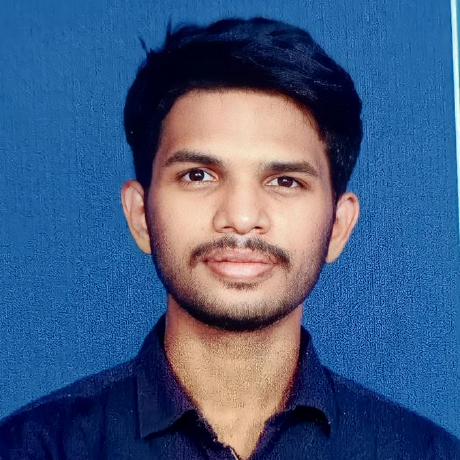
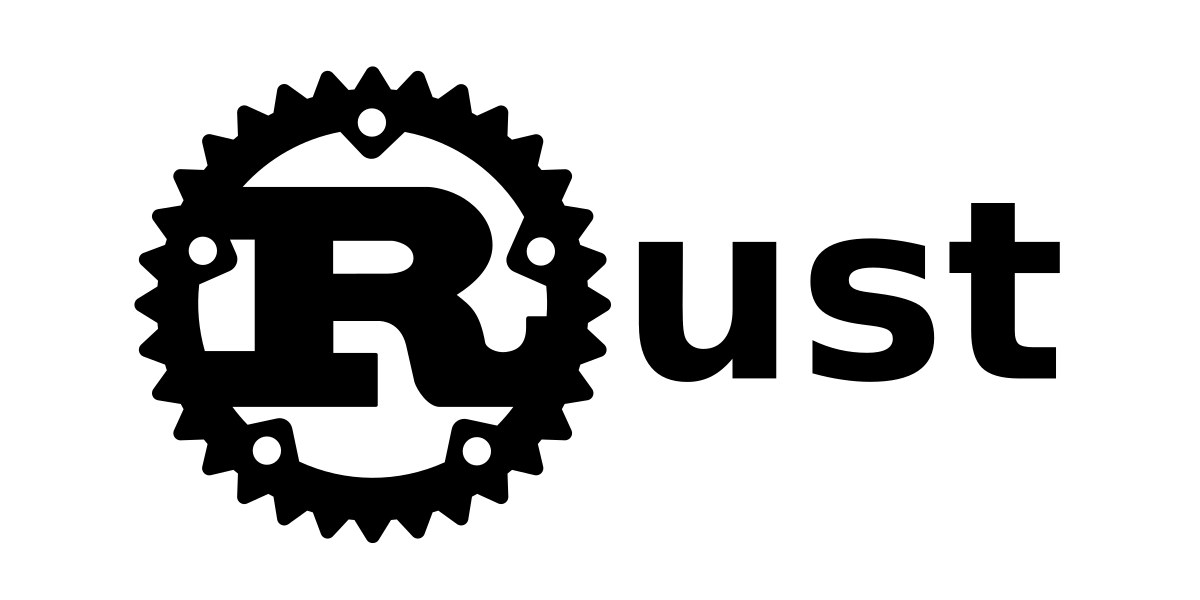
Rust is a high-level programming language with powerful capabilities for low-level memory management. Unlike languages like Java and JavaScript, Rust does not have a garbage collector to automatically clear out unused data. In Rust, it’s not possible to assign one variable’s data to another without explicit permission, which is managed through concepts like ownership and borrowing that we'll explore further.
Data Types in Rust
Rust’s data types are divided into those stored in the stack and those stored in the heap. Primitive data types such as integers, floating points, and booleans are stored on the stack. For example, integers can be defined with types like i8
, i16
, or i32
, where the suffix indicates the memory size allocated for the variable. Booleans don’t require suffix specifications.
Strings, on the other hand, are stored in the heap because their size can vary based on their length and mutability. However, the stack still plays a role in handling strings by storing the memory address of the first character, along with other essential data.
This gives a high-level view of data types in Rust.
Memory Management in Rust
Mutability in Rust means the ability to change the value of an already assigned variable. Unlike Java, which has classes like StringBuilder
and StringBuffer
for mutable strings, Rust does not allow mutability by default. This design choice is advantageous in large-scale projects where controlled memory usage is crucial. To make a variable mutable in Rust, you must use the mut
keyword.
Here's a simple example demonstrating data types and mutability in Rust:
fn main() {
let x: i16 = 1200;
println!("x: {}", x);
let mut name = String::from("Gowtham");
println!("Name: {}", name);
}
Ownership and Borrowing in Rust
Ownership is a core feature in Rust that enables memory efficiency. When a variable is assigned a value, it becomes the owner of that data. If you try to assign the same data to another variable, ownership is transferred, and the original owner can no longer access it. Rust doesn’t allow multiple owners for the same data, which helps prevent memory-related errors.
Borrowing is another critical concept in Rust. Since only one owner is allowed for each value, borrowing lets you reference data without taking ownership of it. This is done using the &
operator, similar to pointers in C.
Here's an example of ownership and borrowing in Rust:
fn main() {
// Ownership
let s1 = String::from("Hello Gowtham");
let s2 = s1;
println!("{}", s1); // Error: s1 is no longer the owner
// Borrowing
let mut str = String::from("Hello");
update_string(&mut str);
println!("{}", str);
}
fn update_string(str: &mut String) {
str.push_str(" World");
println!("{}", str);
}
Pattern Matching in Rust
Pattern matching in Rust is a way to match the structure of a value and bind variables to its parts. It might sound complex, but it's a powerful feature for handling different scenarios in your code.
fn main() {
let name = String::from("Gowtham");
println!("Name: {}", name);
let char1 = name.chars().nth(13);
match char1 {
Some(c) => println!("{}", c),
None => println!("No character found at the specified index!"),
}
}
Error Handling in Rust
Unlike other programming languages like C++, Java, or JavaScript, Rust does not use a try-catch block for error handling. Instead, Rust uses an enum called Result<T,E>
, which has two variants: Ok(T)
for successful operations and Err(E)
for errors. This approach makes error handling straightforward and predictable.
Here's a code snippet demonstrating error handling in Rust:
use std::fs;
//This is just crate(package) in rust feel free to ignore this
fn main() {
let res = fs::read_to_string("example.txt");
//Ok is the condition where if the file exists and Err is the case if the file doesn't exist
match res {
Ok(content) => {
println!("File content: {}", content);
}
Err(err) => {
println!("Error: {}", err);
}
}
}
Conclusion
This blog provides a high-level overview of Rust's memory management, data types, ownership, borrowing, pattern matching, and error handling. Rust's features make it a powerful tool for building safe and efficient systems.
Special thanks to Harkirat Singh for explaining these concepts so clearly!
Subscribe to my newsletter
Read articles from VVSS Gowtham Korupolu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
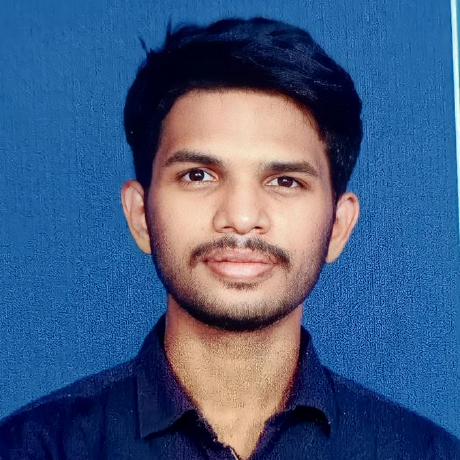