Rendering Lists in React: Using for Loop vs map

Table of contents
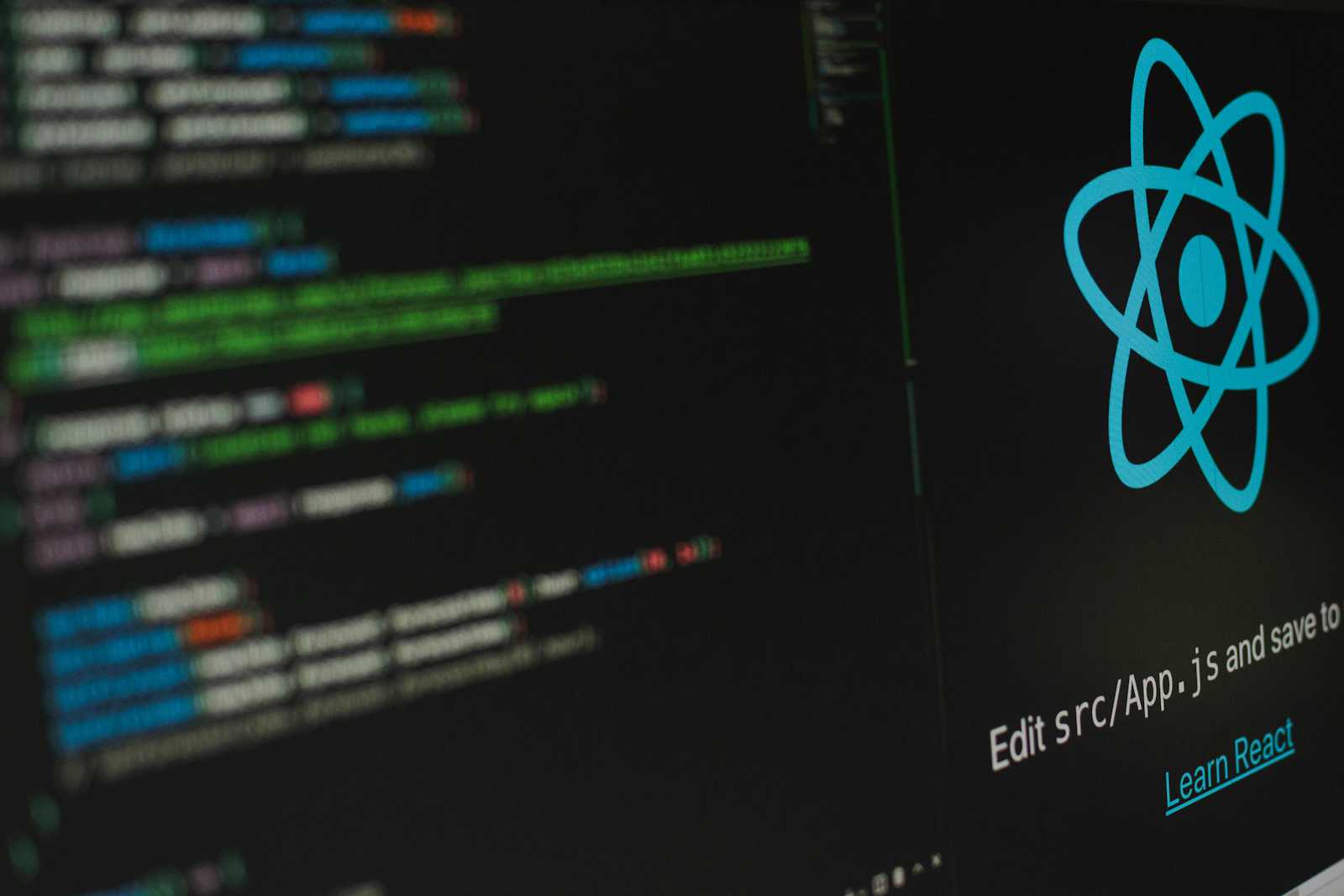
When working with arrays in React, you often need to render a list of components. There are two common ways to achieve this: using a for
loop or the map
method. In this blog post, we'll explore both methods with simple examples.
Using map
The map
method is a built-in JavaScript function that creates a new array populated with the results of calling a provided function on every element in the calling array. It's concise and idiomatic in React.
Example
Let's say we have an array of user objects, and we want to render a list of UserComponent
components.
import React from 'react';
const users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' }
];
const UserComponent = ({ name }) => <div>{name}</div>;
const App = () => {
return (
<div>
{users.map(user => (
<UserComponent key={user.id} name={user.name} />
))}
</div>
);
};
export default App;
In this example:
We use the map method to iterate over the
users
array.For each user, we return a UserComponent with a unique
key
prop and the user's name.
Using for
Loop
While map
is more concise, you can also use a for
loop to achieve the same result. This approach involves creating an array of components and then rendering that array.
Example
Here's how you can use a for
loop to render the same list of UserComponent
components:
import React from 'react';
const users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' }
];
const UserComponent = ({ name }) => <div>{name}</div>;
const App = () => {
const userComponents = [];
for (let i = 0; i < users.length; i++) {
userComponents.push(
<UserComponent key={users[i].id} name={users[i].name} />
);
}
return (
<div>
{userComponents}
</div>
);
};
export default App;
In this example:
We create an empty array
userComponents
.We use a
for
loop to iterate over theusers
array.For each user, we push a
UserComponent
into theuserComponents
array.Finally, we render the
userComponents
array inside thereturn
statement.
Important Note
When using a for
loop, you need to prepare an array of components before returning it. You cannot use a for
loop directly inside the return
statement.
Subscribe to my newsletter
Read articles from Nitesh Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
