Introduction to Trees in Data Structures | Day #11
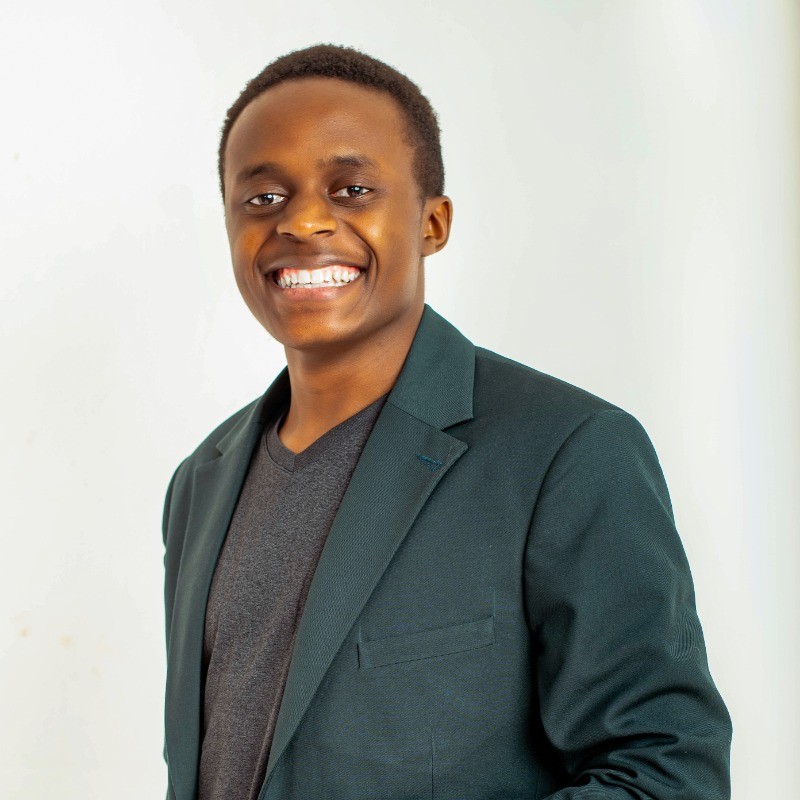
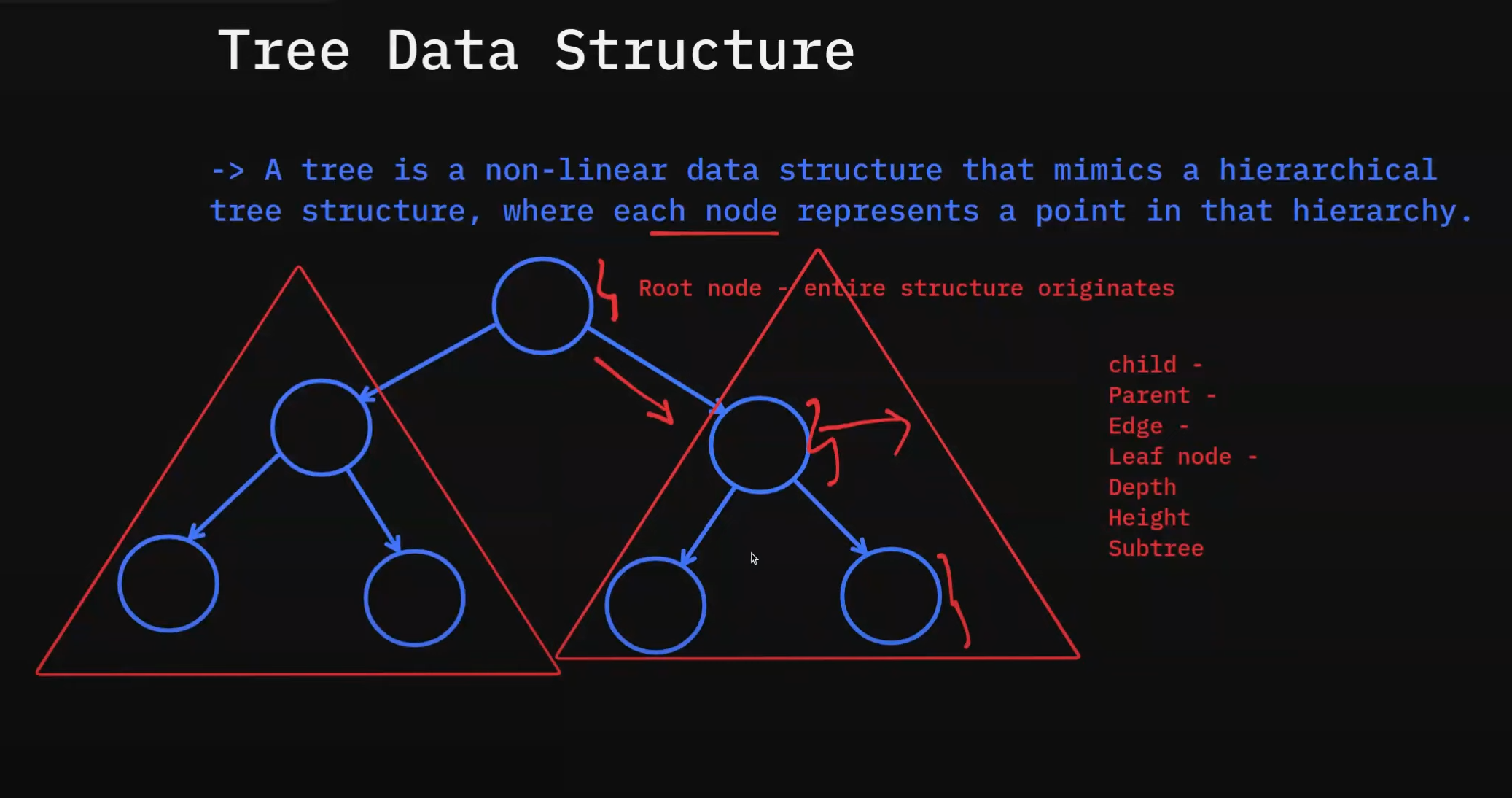
Trees are fundamental data structures used in computer science to represent hierarchical relationships. They are essential for organizing data in a way that allows efficient access and manipulation. In this article, we’ll explore tree data structures, their types, traversal techniques, implementations in Python and JavaScript, and their real-world applications.
What is a Tree?
A tree data structure consists of nodes connected by edges. It has a root node from which all other nodes descend. Each node can have zero or more child nodes, forming a parent-child relationship. This structure makes trees particularly useful for representing hierarchical data, such as organizational charts or file systems.
Significance of Trees
Trees are crucial in various algorithms and applications due to their efficiency in data organization and retrieval. They allow for quicker search, insert, and delete operations compared to linear data structures like arrays and linked lists.
Types of Trees
1. Binary Trees
A binary tree is a tree structure where each node has at most two children, referred to as the left and right child.
2. Binary Search Trees (BST)
A binary search tree is a special type of binary tree where the left child's value is less than its parent's value, and the right child's value is greater. This property makes searching efficient.
3. AVL Trees
AVL trees are self-balancing binary search trees. They maintain a balance factor (the difference in height between the left and right subtrees) to ensure that the tree remains balanced, resulting in improved search times.
4. N-ary Trees
An N-ary tree is a generalization of a binary tree where each node can have up to N children. This is useful for representing more complex hierarchical structures.
For a more detailed look at binary search trees, check out our article on Binary Search Trees Explained.
Tree Traversal Techniques
Tree traversal refers to the process of visiting all nodes in a tree. The three primary traversal techniques are:
Inorder Traversal: Visits the left subtree, the root, and then the right subtree.
Preorder Traversal: Visits the root, the left subtree, and then the right subtree.
Postorder Traversal: Visits the left subtree, the right subtree, and then the root.
Implementing Trees in Python and JavaScript
Python Code Example
class Node:
def __init__(self, key):
self.left = None
self.right = None
self.value = key
class BinaryTree:
def __init__(self):
self.root = None
def insert(self, key):
if self.root is None:
self.root = Node(key)
else:
self._insert_rec(self.root, key)
def _insert_rec(self, node, key):
if key < node.value:
if node.left is None:
node.left = Node(key)
else:
self._insert_rec(node.left, key)
else:
if node.right is None:
node.right = Node(key)
else:
self._insert_rec(node.right, key)
def inorder(self, node):
if node:
self.inorder(node.left)
print(node.value, end=' ')
self.inorder(node.right)
# Usage
tree = BinaryTree()
tree.insert(10)
tree.insert(5)
tree.insert(15)
print("Inorder Traversal:")
tree.inorder(tree.root)
JavaScript Code Example
class Node {
constructor(key) {
this.left = null;
this.right = null;
this.value = key;
}
}
class BinaryTree {
constructor() {
this.root = null;
}
insert(key) {
if (this.root === null) {
this.root = new Node(key);
} else {
this._insertRec(this.root, key);
}
}
_insertRec(node, key) {
if (key < node.value) {
if (node.left === null) {
node.left = new Node(key);
} else {
this._insertRec(node.left, key);
}
} else {
if (node.right === null) {
node.right = new Node(key);
} else {
this._insertRec(node.right, key);
}
}
}
inorder(node) {
if (node) {
this.inorder(node.left);
console.log(node.value);
this.inorder(node.right);
}
}
}
// Usage
const tree = new BinaryTree();
tree.insert(10);
tree.insert(5);
tree.insert(15);
console.log("Inorder Traversal:");
tree.inorder(tree.root);
Time Complexity Analysis
Insertion: O(log n) for balanced trees (like AVL) and O(n) for unbalanced trees.
Deletion: O(log n) for balanced trees and O(n) for unbalanced trees.
Search: O(log n) for balanced trees and O(n) for unbalanced trees.
Real-World Applications of Trees
Trees are widely used in various applications:
File Systems: Organizing files and directories.
Databases: Efficient data retrieval and storage.
Routing Algorithms: Used in networking to determine the best path for data transmission.
Expression Parsing: In compilers and interpreters, trees help parse expressions and represent syntactic structure.
FAQs
What are trees used for in programming?
Trees are used for efficient data storage, searching, and manipulation. They help represent hierarchical data, optimize search operations, and manage datasets in databases and file systems.
What is the difference between a binary tree and a binary search tree?
A binary tree allows each node to have up to two children without any specific order, while a binary search tree enforces a strict order where the left child is smaller than the parent, and the right child is larger.
How do AVL trees maintain balance?
AVL trees maintain balance by ensuring the height difference between the left and right subtrees of any node is at most one. This is achieved through rotations during insertion and deletion operations.
For more insights, check out our articles on Comparing Trees and Graphs and Introduction to Binary Search Trees.
Subscribe to my newsletter
Read articles from Bonaventure Ogeto directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
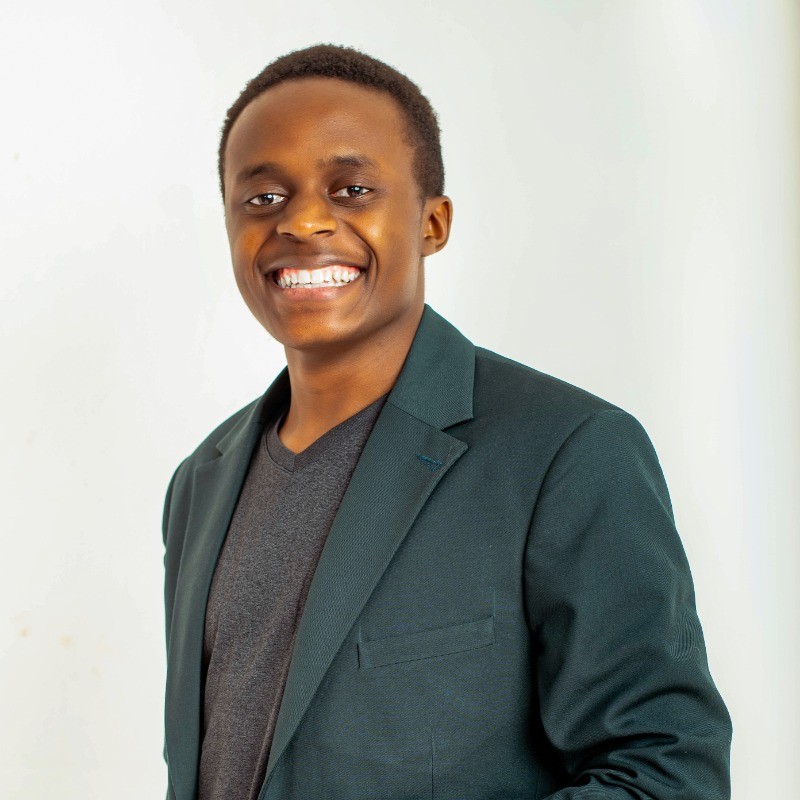
Bonaventure Ogeto
Bonaventure Ogeto
Software Engineer & Technical Writer