Understanding the Core Concepts of Object-Oriented Programming in Java

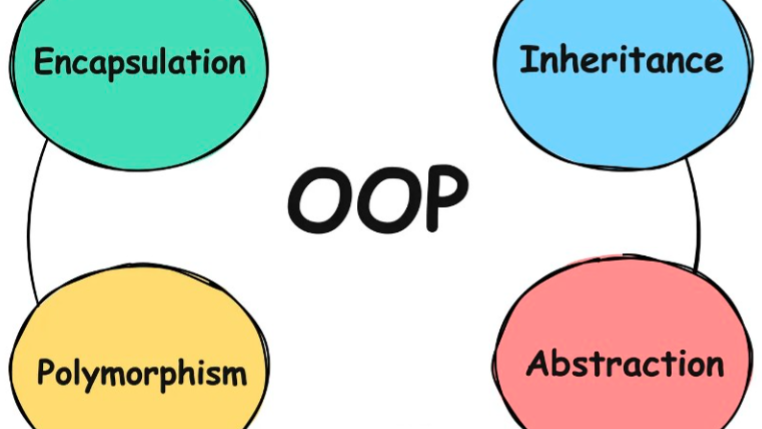
Object-Oriented Programming (OOP) is a fundamental concept in modern software development. OOP allows you to create programs that are more modular, reusable, and scalable. In this article, we will explore the four main pillars of OOP: Encapsulation, Inheritance, Polymorphism, and Abstraction, using simple Java code examples to illustrate these concepts.
1. Encapsulation: Protecting Data
Encapsulation refers to bundling data (variables) and methods (functions) into a single unit, typically a class, and restricting direct access to some of an object's components. This helps ensure that data is not accidentally changed from outside the class, maintaining its integrity.
In Java, encapsulation is achieved using private fields and public getter and setter methods. Here's an example:
public class Person {
private int age;
public int getAge() {
return age;
}
public void setAge(int age) {
if (age > 0) {
this.age = age;
} else {
System.out.println("Age must be a positive number.");
}
}
}
In this example, the age
field is private and cannot be accessed directly. Instead, we use the getAge
and setAge
methods to interact with it. This helps protect the data and ensures that only valid values are set for the age
.
2. Inheritance: Reusing Code
Inheritance allows one class to inherit properties and methods from another class, promoting code reuse and establishing a hierarchical relationship between classes. The class that inherits is called the "subclass" or "child class," and the class being inherited from is called the "superclass" or "parent class."
Let's see an example:
public class Animal {
public void eat() {
System.out.println("This animal eats food.");
}
}
public class Dog extends Animal {
public void bark() {
System.out.println("The dog barks.");
}
}
In this example, the Dog
class inherits the eat()
method from the Animal
class. This allows Dog
objects to reuse the functionality of the Animal
class without duplicating the code.
3. Polymorphism: One Interface, Many Forms
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It also allows methods to perform different actions based on the object they are called on. In Java, polymorphism is achieved through method overriding and method overloading.
Here's an example:
public class Animal {
public void speak() {
System.out.println("The animal makes a sound.");
}
}
public class Dog extends Animal {
@Override
public void speak() {
System.out.println("The dog barks.");
}
}
public class Cat extends Animal {
@Override
public void speak() {
System.out.println("The cat meows.");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.speak();
Animal myCat = new Cat();
myCat.speak();
}
}
In this example, even though both myDog
and myCat
are of type Animal
, the appropriate speak()
method is called based on the actual object type (Dog
or Cat
). This is polymorphism in action!
4. Abstraction: Hiding Complexity
Abstraction allows you to hide the complex implementation details of a class and expose only the essential features. It lets you focus on what an object does, rather than how it does it. In Java, abstraction is achieved using abstract classes or interfaces.
Here's an example of an abstract class:
public abstract class Shape {
public abstract double calculateArea();
}
public class Rectangle extends Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
public double calculateArea() {
return width * height;
}
}
public class Main {
public static void main(String[] args) {
Shape rectangle = new Rectangle(5, 10);
System.out.println("Area of the rectangle: " + rectangle.calculateArea()); // Output: Area of the rectangle: 50
}
}
In this example, the Shape
class defines an abstract method calculateArea()
that must be implemented by any subclass. The Rectangle
class provides the actual implementation of how the area is calculated, hiding the complexity from the user.
Conclusion
Object-Oriented Programming (OOP) in Java helps developers create more organized, maintainable, and reusable code. The four key principles—Encapsulation, Inheritance, Polymorphism, and Abstraction—are essential for building robust Java applications. By using these concepts, you can structure your code in a way that is clean, efficient, and easy to understand.
Whether you’re building a small application or a large enterprise system, mastering OOP concepts is a crucial step in your journey to becoming a proficient Java developer.
#Java #Object-Oriented #Programming #Inheritance #Polymorphism #Abstraction #Encapsulation #OOP #JavaProgramming #Coding #Tech #SoftwareDevelopment #LearnJava
Subscribe to my newsletter
Read articles from Shubham Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shubham Khan
Shubham Khan
Full-Stack Software Engineer with over 3 years of experience in building scalable web applications using React, Spring Boot, and RDBMS. Proven ability to enhance system performance, increase efficiency, and build secure authentication systems. Skilled in developing and deploying applications with cloud services like AWS and Docker, while driving team collaboration through Agile methodologies.