How to Set Up Your Development Environment for Open Source Contributions

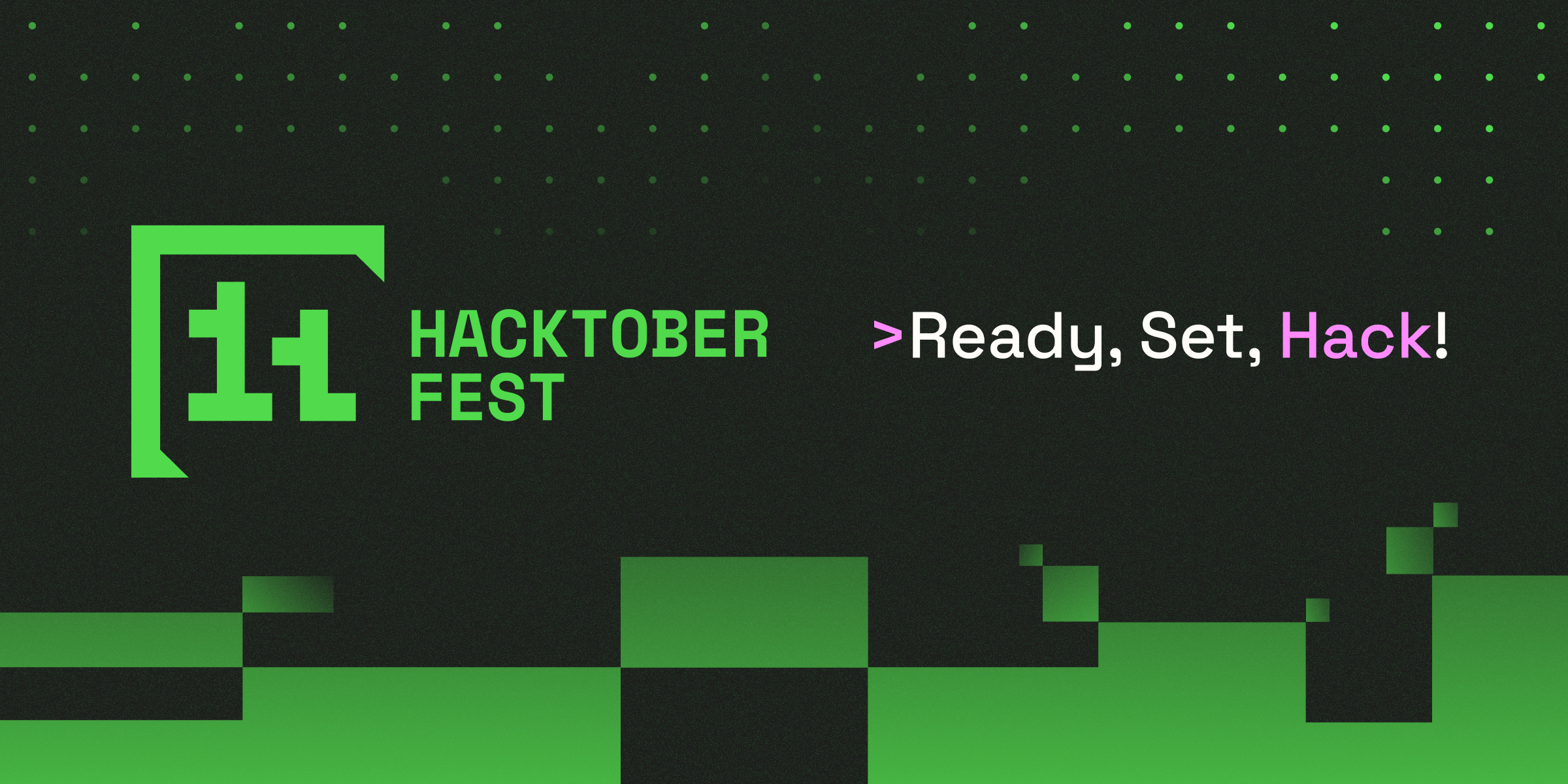
Contributing to open source can feel overwhelming, especially when it comes to setting up your development environment correctly. A good setup can significantly enhance your productivity and make the process of contributing less daunting. In this blog, we'll guide you through the basics, including setting up Git and GitHub, choosing an IDE, using Docker, and configuring debugging and testing tools.
1. Git and GitHub Setup
Git and GitHub are the lifeblood of open source contributions. They allow you to clone repositories, make changes, and submit pull requests for review. Here’s how to get started:
Installing Git
First, you need to install Git. If you’re on a Linux system, use:
sudo apt-get update
sudo apt-get install git
For macOS, you can install it using Homebrew:
brew install git
On Windows, download it from Git's official site.
Configuring Git
After installing Git, it’s essential to configure your username and email address to ensure that your contributions are properly attributed.
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
These commands set up your identity for Git commits. The --global
flag applies these settings across all repositories, which is typically what you want.
Setting Up GitHub SSH Key
An SSH key allows you to securely connect with GitHub without entering your credentials every time. To create one, use:
ssh-keygen -t ed25519 -C "your.email@example.com"
After generating the SSH key, add it to the ssh-agent:
eval "$(ssh-agent -s)"
ssh-add ~/.ssh/id_ed25519
Finally, copy the key to GitHub by pasting it into the "SSH keys" section of your GitHub account settings:
cat ~/.ssh/id_ed25519.pub
This command displays your public key, which you can then copy to GitHub.
Forking and Cloning a Repository
Once you have your Git and GitHub set up, the next step is to fork and clone a repository you want to contribute to.
Forking: Go to the repository you want to contribute to on GitHub and click the "Fork" button. This creates a copy of the repository under your GitHub account.
Cloning: To work on the repository locally, you need to clone it. Use the following command:
git clone git@github.com:arya2004/xyfin.git
This command creates a local copy of the repository on your computer.
Creating Branches
When contributing to an open source project, it's a good practice to create a new branch for each feature or bug fix. This way, you keep your changes organized and avoid conflicts.
git checkout -b feature-branch-name
This command creates and switches to a new branch named feature-branch-name
.
Making Commits and Pushing Changes
After making changes to the code, you need to commit and push them to your GitHub repository.
Staging Changes: Use
git add
to stage the files you’ve modified:git add .
This command stages all changed files. You can also specify a particular file to stage, e.g.,
git add filename
.Committing Changes: To commit your changes, use:
git commit -m "Add a descriptive commit message"
A good commit message briefly describes what you’ve done, making it easier for others to understand your changes.
Pushing Changes: Push your branch to GitHub with:
git push origin feature-branch-name
Now, your changes are available in your GitHub fork, and you can open a pull request (PR) to the original repository.
Opening a Pull Request
To contribute your changes back to the original repository, navigate to your GitHub repository, and you’ll see a prompt to open a pull request. Click on "Compare & pull request," add a descriptive title and summary, and submit the pull request for review.
2. Setting Up Your IDE
Your choice of IDE (Integrated Development Environment) is crucial. It can greatly streamline your workflow when contributing to open source projects.
Visual Studio Code (VSCode)
VSCode is one of the most popular IDEs for open source contributors, mainly due to its flexibility and wide range of extensions. Here’s how to make the most out of VSCode for open source:
Extensions to Consider:
GitLens: Provides a powerful visualization of Git history, annotations, and file changes.
Prettier: Helps maintain consistent code formatting.
ESLint: Catches JavaScript/TypeScript errors as you write code.
Live Share: Enables real-time collaboration with other developers, which can be very useful for mentoring or pair programming sessions.
These extensions help maintain code quality and make it easier to collaborate on projects with multiple contributors.
Integrated Terminal: VSCode has an integrated terminal, making it easy to run Git commands, tests, or other tools without leaving the editor. You can open it by pressing
Ctrl +
` or from the menu.Settings Sync: VSCode also offers a Settings Sync feature that allows you to save your settings, extensions, and configurations to the cloud. This way, if you switch devices, you can restore your environment effortlessly.
IntelliJ IDEA
For Java or Kotlin projects, IntelliJ IDEA is a great option. It has a powerful built-in toolset, including:
Version Control Integration: IntelliJ has Git integration that allows you to manage branches, view diffs, and handle merge conflicts directly in the IDE.
Code Analysis Tools: IntelliJ automatically detects many code issues, helping you maintain clean, functional code.
Maven and Gradle Integration: IntelliJ seamlessly integrates with build tools like Maven and Gradle, which are commonly used in Java projects. This is essential for building and managing project dependencies.
IDE Plugins
Docker Plugin: If you’re using Docker (more on this soon), IDE plugins for Docker, such as the Docker extension for VSCode or IntelliJ, allow you to manage and visualize your containers directly from the IDE.
Debugger Tools: Most IDEs come with built-in debugging tools for various languages. Setting up breakpoints and stepping through code can help diagnose issues quickly.
Code Quality Plugins: Plugins like CheckStyle or SonarLint can help maintain code quality by enforcing style guidelines and detecting code smells or potential bugs.
3. Using Docker for Consistent Environments
Docker is an invaluable tool for creating consistent development environments, which is particularly helpful when working on multiple projects or collaborating with contributors across different setups.
Why Docker?
Imagine contributing to a Python project that requires Python 3.7, while your system has Python 3.9 installed. This mismatch can cause compatibility issues. Docker helps solve this by packaging all dependencies and environments into an isolated container.
Docker creates an abstraction layer between your system and the code you are working on, meaning that your code runs in the same way regardless of what operating system or software versions you have installed.
Installing Docker
Windows and macOS: Docker Desktop is the simplest way to install Docker. It provides an easy-to-use interface for managing containers.
Linux: Use the following commands:
sudo apt-get update
sudo apt-get install docker-ce docker-ce-cli containerd.io
After installation, make sure Docker is running properly by using:
docker --version
Setting Up a Docker Container
Let’s say you’re contributing to a Node.js project. You can create a Dockerfile that specifies the Node.js version and dependencies:
# Dockerfile
FROM node:14
WORKDIR /app
COPY package.json ./
RUN npm install
COPY . .
CMD ["npm", "start"]
With this Dockerfile, you can create a containerized environment:
docker build -t my-node-app .
docker run -p 3000:3000 my-node-app
Using Docker ensures that your environment is identical to that of the project's maintainers and other contributors.
Docker Compose for Multi-Container Projects
Some projects involve multiple services (e.g., a backend server, a database, a message broker). Docker Compose is a tool for defining and running multi-container Docker applications.
Here’s an example docker-compose.yml
file for a project with a Node.js backend and a MongoDB database:
version: '3.8'
services:
web:
build: .
ports:
- "3000:3000"
depends_on:
- db
db:
image: mongo
ports:
- "27017:27017"
Run the following command to start all services:
docker-compose up
This approach makes it much easier to manage projects with several dependencies and ensures that everything runs seamlessly.
4. Debugging and Testing Tools
Debugging and testing are essential parts of any development process, especially in open source projects where code quality is crucial.
Debugging Tools
VSCode Debugger: VSCode has an excellent built-in debugger for languages like JavaScript, Python, and more. You can set breakpoints, watch variables, and step through code to identify issues. To start debugging, use the
Run and Debug
view or pressF5
.IntelliJ Debugger: For Java or Kotlin projects, IntelliJ offers a robust debugger. You can evaluate expressions, set conditional breakpoints, and examine threads. This makes diagnosing problems in complex applications significantly easier.
Browser Developer Tools: For front-end development, tools like Chrome Developer Tools are invaluable. They allow you to inspect elements, view console logs, and debug JavaScript directly in the browser.
Testing Tools
Testing ensures that your code works as intended and that any changes you make don't break existing functionality.
Pytest (Python): Pytest is a powerful testing framework for Python projects. It allows you to write simple unit tests and run them with detailed output:
pip install pytest pytest tests/
Pytest also supports fixtures, which help in setting up resources required for testing, such as database connections.
Mocha (JavaScript): Mocha is a popular JavaScript test framework for Node.js applications. To use it:
npm install --save-dev mocha
Then, add a simple test file,
test.js
:const assert = require('assert'); describe('Array', function() { describe('#indexOf()', function() { it('should return -1 when the value is not present', function() { assert.strictEqual([1, 2, 3].indexOf(4), -1); }); }); });
Run it with:
npx mocha
Jest (JavaScript): Jest is another popular testing framework for JavaScript, especially for React projects. Jest includes features like test coverage, mocking, and snapshot testing, making it a comprehensive testing solution.
JUnit (Java): For Java projects, JUnit is the most widely used testing framework. It integrates seamlessly with IntelliJ IDEA and other IDEs and allows developers to write unit tests for their Java code.
CI/CD Integration: Integrating your tests with Continuous Integration (CI) tools like GitHub Actions, Travis CI, or Jenkins ensures that tests are run automatically whenever changes are made. This practice helps catch issues early in the development cycle.
Best Practices for Development Setup
Keep Your Environment Clean: Use virtual environments for Python (
venv
orvirtualenv
) ornvm
(Node Version Manager) for Node.js to avoid dependency issues across projects.Documentation: Always document your setup and the dependencies you need. Creating a
README.md
or aSETUP.md
helps other contributors understand how to set up the environment quickly.Use
.gitignore
: Ensure that unnecessary files like logs, build artifacts, and environment configurations are not included in version control. Use.gitignore
files to specify which files to ignore.Consistent Formatting: Use tools like Prettier or Black (for Python) to ensure consistent code formatting. Consistent formatting reduces friction during code reviews and makes it easier for others to read your code.
Linting: Use linting tools to catch syntax errors and enforce style guides. ESLint for JavaScript and Pylint for Python are common choices.
Communicate with Maintainers: Before setting up your environment, always read the project’s contribution guide (often found in
CONTRIBUTING.md
). This document typically contains valuable information on setting up the environment and coding standards.
Final Thoughts
Setting up a development environment that works well for open source contributions takes a bit of effort, but it pays off in the long run. By correctly configuring Git, using a capable IDE, leveraging Docker for consistent environments, and incorporating debugging and testing tools, you'll have a solid foundation for making meaningful contributions to open source projects.
As you prepare for Hacktoberfest or your next open source venture, remember: the more time you invest in your setup, the more seamless your coding experience will be. Don't hesitate to experiment with different tools until you find what works best for you.
Last Year, even I wasn’t able to get a single PR merged, however this year I completed the challenge within 12 Days!
Open source is all about collaboration, growth, and learning. Your contributions, no matter how small, can have a significant impact. Happy coding, and welcome to the open source community!
This comprehensive guide was written by Arya M. Pathak. Follow his account to read such informative blogs !
Subscribe to my newsletter
Read articles from MicrosoftLearnStudentClub directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
