JAVA: Conditional Statements

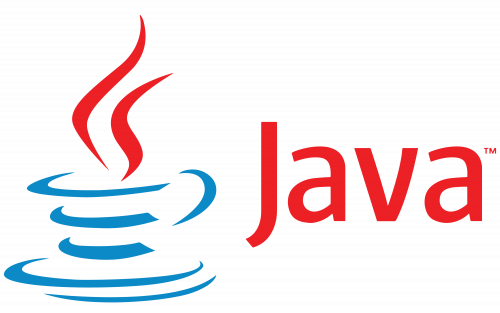
Prologue
Have you ever made a decision? What thought process did you use to make it? You probably considered the conditions you had and then made your decision based on that. Voilà, you now understand conditional statements.
What are conditional statements?
Conditional statements, also known as decision-making statements, are programming tools that let a program perform specific actions or run blocks of code depending on whether a given condition is true or false. These statements control the flow of a program by checking conditions and directing the execution path accordingly.
Conditional statements are crucial for making decisions and managing program behavior based on changing inputs or situations.
Types Of Conditional Statements
There are different types of conditional statements and each have different keywords that perform distinct actions and break up the flow of execution, it helps in determining which code block to execute, thereby altering the natural flow of executions based on those conditions.
if statements
It is the most basic decision control statement of all. It tells your program to execute a certain section of code only if a particular test evaluates to true
, if it evaluates to false, the code inside the block is skipped.
// Syntax
if (condition)
{
// code to execute if the coditon is true
}
FLOW CHART:
if-else statements
This statement provides an alternative execution path when the condition of an if
clause evaluates to false. Unlike the if
statement, it includes the else
keyword, which executes its code only when the condition is false.
// Syntax
if (condition){
// Code to execute if the condition is true
}else{
// Code to execute if the condition is false
}
FLOW CHART:
else - if ladder
The else-if ladder is a programming construct that lets you evaluate multiple conditions one after another. It extends the basic if-else
statement by allowing you to check more conditions if the previous ones are false. This structure is especially useful when you need to handle several distinct cases.
// Syntax
if (condition1) {
// Code block for condition1
} else if (condition2) {
// Code block for condition2
} else if (condition3) {
// Code block for condition3
} else {
// Code block if none of the above conditions are true
}
FLOW CHART:
switch statements
This statement is used to choose one of many code blocks to run based on the value of a variable or expression.
The switch
statement checks an expression and compares its result with the values in each case
. When a match is found, the matching block of code runs. If no match is found, the default
block (if present) is executed.
// Syntax
switch (expression) {
case value1:
// Code to be executed if expression matches value1
break; // Exit the switch block
case value2:
// Code to be executed if expression matches value2
break;
// Add more cases as needed
default:
// Code to be executed if no matching case is found
break;
}
FLOW CHART:
Program
Program (if, if-else, else-if ladder)
import java.util.*;
class Condition {
public static void main(String[] args) {
// Asking user to input the marks by creating object of Scanner
Scanner s = new Scanner(System.in);
System.out.print("Enter Your Marks: ");
int marks = s.nextInt();
// If statement
if (marks >= 80) {
System.out.println("Passed with distinction.");
}
// If-else statement for printing the status
if (marks >= 40 ) {
System.out.println("Passed ");
} else {
System.out.println("Fail");
}
// Else-if ladder for printing the grade
if (marks >= 90) {
System.out.println("Grade: A+");
} else if (marks >= 80) {
System.out.println("Grade: A");
} else if (marks >= 70) {
System.out.println("Grade: B+");
} else if (marks >= 60) {
System.out.println("Grade: B");
} else if (marks >= 50) {
System.out.println("Grade: C");
} else if (marks >= 40) {
System.out.println("Grade: D");
} else {
System.out.println("Grade: F");
}
s.close();
}
}
OUTPUT:
Program (switch case)
import java.util.*;
class SwitchDemo {
public static void main(String[] args) {
// Asking the user to enter the day number
Scanner s = new Scanner(System.in);
System.out.print("Enter a number (1-7) to get the corresponding day of the week: ");
int day = s.nextInt();
String dayName; // Storing the day name corresponding to the day number
// switch case
switch (day) {
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
case 4:
dayName = "Thursday";
break;
case 5:
dayName = "Friday";
break;
case 6:
dayName = "Saturday";
break;
case 7:
dayName = "Sunday";
break;
default:
dayName = "Invalid day! Please enter a number between 1 and 7.";
break;
}
System.out.println(dayName); // Printing the day name corresponding to the day number user entered
s.close();
}
}
OUTPUT:
What’s next?
So, today we learned about Conditional Statements, and there is much more to come. In the next part, we’ll learn about Constructors, how they work, and what their importance is.
So, Stay tuned!!
PS: I am also learning Java while teaching it. I believe in continuous growth and am committed to learning as much as I am teaching. If you have any questions, suggestions, or just want to chat about Java, please leave a comment! I'm also new to blogging, so any feedback is appreciated as I strive to improve. Thank you for joining me on this journey! Let's learn and grow together.
Subscribe to my newsletter
Read articles from Sarthak Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sarthak Sharma
Sarthak Sharma
Hi, I’m Sarthak, a passionate tech enthusiast, a student and blogger. I’m on a mission to share the things that i learn or find interesting.