Version Control 101: How Git and GitHub Power Your Development Workflow

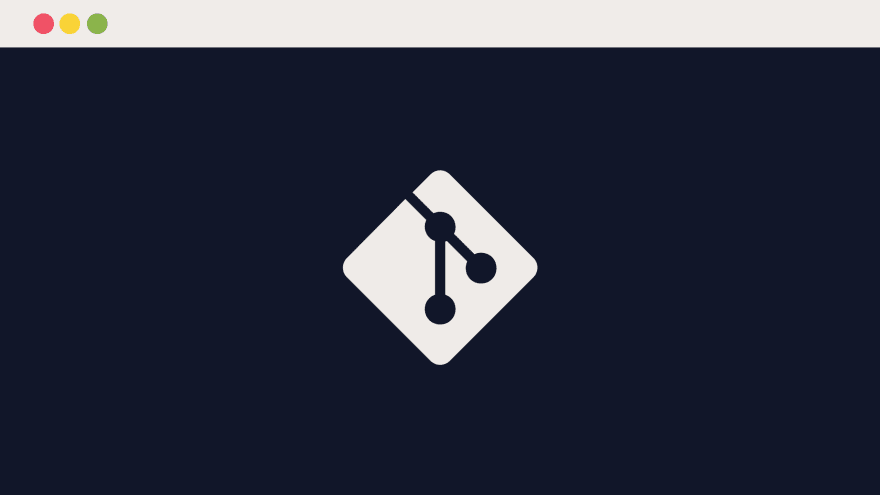
What is Git?
Git is an open-source, distributed version control system.
It’s lightweight and works on almost all operating systems.
Tracks and manages changes in code across collaborative projects.
History of Git
BitKeeper was a commercial distributed version control system (DVCS) developed in the late 1990s by Larry McVoy. It allowed Linux kernel developers to use it for free.
Due to a conflict over reverse-engineering, Linus Torvalds (creator of Linux) developed Git in 2005 as a free, open-source alternative to BitKeeper.
Git vs GitHub
Git: A version control system to track changes in code. It’s software used locally for managing repositories.
GitHub: A platform that hosts Git repositories online, offering collaboration tools like pull requests, issue tracking, and more.
GitLab: Similar to GitHub but includes built-in CI/CD tools for automation.
Bitbucket: A Git repository hosting platform, often integrated with Atlassian tools like Jira.
Why Git in DevOps?
CI/CD Pipelines: Git is crucial for creating pipelines, tagging releases, and version control in DevOps.
Infrastructure as Code (IaC): Tools like Terraform use Git to manage infrastructure configurations.
GitOps: Git serves as the source of truth for infrastructure, automating deployments based on repository changes.
Git Basics
Starting a Git Project
Initialize Git in a project:
git init
- This command sets up a new Git repository in your project.
Add files to staging area:
git add <file-path>
- Adds files to the staging area, preparing them for a commit.
Commit changes:
git commit -m "Commit message"
- Saves changes from the staging area into the repository with a description.
View commit history:
git log git log --oneline
Check file differences:
git diff
Track file history by line:
git blame <file-path>
Check the repository status:
git status
- Shows the status of files in your working directory and staging area.
Staging Area and Commits
Files go through these stages:Local Workspace → Staging Area → Committed to Repository
Only staged files are committed.
Proper commit history helps in reverting to any commit point.
Reverting to Previous Commits
Reset to a commit:
git reset --hard <commit_id>
- Moves the head to a specific commit, removing later changes.
Revert a commit (safer):
git revert <commit_id>
- Creates a new commit that reverses changes from a previous commit without deleting history.
Collaboration in Git
Remote Repositories
GitHub, GitLab, Bitbucket are popular hosting services for Git repositories.
To connect your local repository to a remote server:
git remote add origin <remote-url>
- Origin is the name of the remote server (commonly used name).
Syncing Changes
Push local commits to a remote repository:
git push
Authentication Methods: HTTPS vs SSH
HTTPS:
Uses Personal Access Tokens (PAT) for authentication.
Simple setup but requires entering credentials unless cached.
SSH:
More secure, using public and private keys.
Once set up, it doesn’t require credentials for every operation.
Check remote URL type:
git remote -v
HTTPS looks like
https://github.com/user/repo.git
SSH looks like
git@github.com
:user/repo.git
Branching in Git
Why Branching?
Branches allow multiple developers to work in parallel without affecting the main codebase.
Each branch represents an independent line of development.
Common Branching Commands
Create a new branch:
git branch <branch-name>
Switch to a branch:
git checkout <branch-name>
Push a branch to the remote repository:
git push --set-upstream origin <branch-name>
Merging Branches
Merge a remote branch:
git merge origin/<branch-name>
- Merges changes from the remote branch into your current branch.
Merge locally and push:
git checkout master git merge <branch-name> git push origin master
Remote Merge and Pull Workflow
Merge remotely using a Pull Request:
- Merge the branch on the remote platform (e.g., GitHub) using a Pull Request.
Pull changes locally:
git pull origin master
- Updates your local master with the latest changes from the remote repository.
Best Practices in Git
Make frequent, meaningful commits: A separate commit for each functionality keeps the history clean.
Use branches for features: Develop each feature on a separate branch to keep the main branch stable.
Write descriptive commit messages: This makes the history easy to follow.
Avoid large commits: Commit smaller, focused changes for better tracking and rollback options.
Subscribe to my newsletter
Read articles from Harsh Butani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
