Deep dive Throttling in React Native (JavaScript)

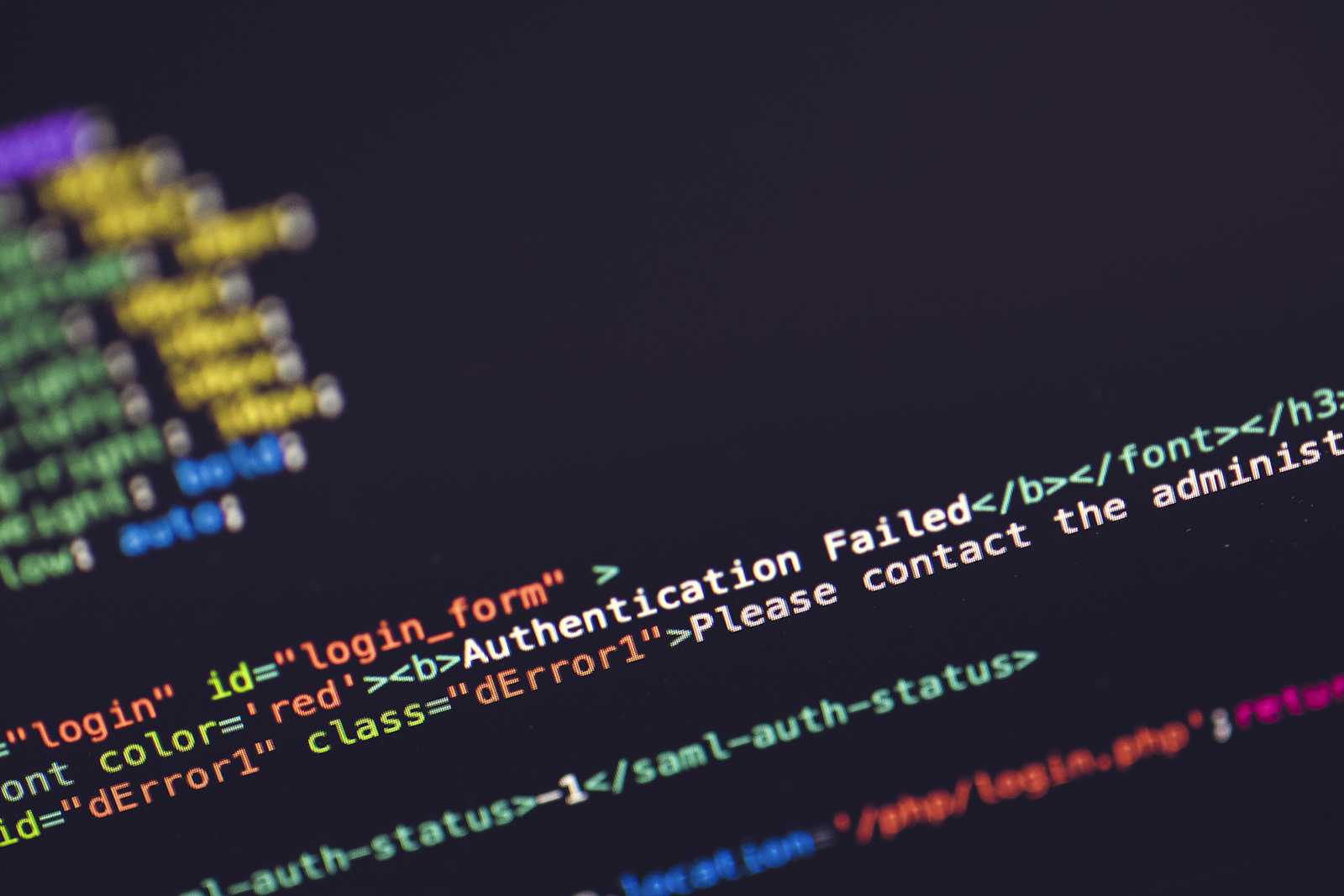
Throttling is a technique used to limit the number of times a function can be called over a certain period of time. Unlike debouncing, which delays function execution until after a quiet period, throttling guarantees that a function is called at a regular interval, regardless of how many times it's invoked.
Key aspects of throttling:
Regular Execution: A throttled function will execute at a steady rate, even if it's called more frequently.
First Call Execution: Often, throttled functions execute on the first call, then wait for the specified time before allowing another execution.
Consistent Timing: The time between function executions is consistent, determined by the throttle delay.
Useful for Continuous Events: Throttling is particularly useful for events that fire rapidly and continuously, like scrolling or resizing.
Here's a basic implementation of a throttle function:
function throttle(func, limit) {
let inThrottle;
return function(...args) {
const context = this;
if (!inThrottle) {
func.apply(context, args);
inThrottle = true;
setTimeout(() => inThrottle = false, limit);
}
}
}
// Usage
const throttledScroll = throttle(() => {
console.log('Scroll event');
}, 1000);
// Attach to scroll event
window.addEventListener('scroll', throttledScroll);
In this example, no matter how many times the scroll event fires, the throttledScroll
function will only execute once every 1000 milliseconds (1 second).
Differences between Throttling and Debouncing:
Timing of Execution:
Throttling: Executes the function at a regular interval.
Debouncing: Delays execution until after a quiet period.
Frequency of Execution:
Throttling: Guarantees function execution at a steady rate.
Debouncing: May prevent function execution entirely if events keep firing.
Use Cases:
Throttling: Best for regular updates (e.g., game loop, scroll handlers).
Debouncing: Best for final state updates (e.g., search input, resize end).
First Call Behavior:
Throttling: Often executes on the first call.
Debouncing: Typically delays even the first call.
In React Native, you might use throttling for scenarios like:
Handling rapid button presses to prevent double submissions.
Limiting the rate of updates during gesture responses (e.g., in a custom slider component).
Controlling the frequency of API calls in a real-time data fetching scenario.
Here's a simple example of using throttling in a React Native component:
import React from 'react';
import { Button } from 'react-native';
import _ from 'lodash'; // Lodash provides a throttle function
const ThrottledButton = () => {
const handlePress = _.throttle(() => {
console.log('Button pressed');
// Perform action here
}, 1000);
return (
<Button
title="Press Me"
onPress={handlePress}
/>
);
};
In this example, no matter how rapidly the user presses the button, the action will only be performed once per second.
Both throttling and debouncing are valuable techniques for optimizing performance in React Native apps, especially when dealing with events that can fire rapidly or continuously. The choice between them depends on the specific requirements of your use case.
Q: What is the main difference between throttling and debouncing in the context of React Native performance optimization?
The main difference is in how they control the frequency of function execution:
Throttling limits the rate at which a function is called, ensuring it's not executed more than once in a specified time interval. It's useful for scenarios where you want regular updates at a controlled rate.
Debouncing delays the execution of a function until after a period of inactivity. It's useful when you want to wait for a pause in activity before executing a function.
Q: Write a basic throttle function in JavaScript that can be used in a React Native component.
function throttle(func, limit) {
let lastFunc;
let lastRan;
return function(...args) {
const context = this;
if (!lastRan) {
func.apply(context, args);
lastRan = Date.now();
} else {
clearTimeout(lastFunc);
lastFunc = setTimeout(function() {
if ((Date.now() - lastRan) >= limit) {
func.apply(context, args);
lastRan = Date.now();
}
}, limit - (Date.now() - lastRan));
}
}
}
This JavaScript code defines a throttle function. Throttling ensures that a function (func) is executed at most once every limit milliseconds, even if it is triggered multiple times. It prevents a function from being called too frequently, which can improve performance, especially in scenarios like window resizing, scrolling, or user input events.
Initial Execution Check:
const context = this;
if (!lastRan) {
func.apply(context, args);
lastRan = Date.now();
}
• context = this: Ensures that the original context (object calling the function) is preserved when func is called.
• If lastRan is not set (meaning the function hasn’t run yet), it immediately runs func using func.apply(context, args) and sets lastRan to the current time (Date.now()).
Subsequent Executions:
If lastRan is already set (i.e., the function has run before), the function execution is delayed if it is triggered too soon:
clearTimeout(lastFunc);
lastFunc = setTimeout(function() {
if ((Date.now() - lastRan) >= limit) {
func.apply(context, args);
lastRan = Date.now();
}
}, limit - (Date.now() - lastRan));
• clearTimeout(lastFunc): If there’s a pending function execution (from a previous throttle call), it clears the timeout to prevent multiple executions stacking up.
• setTimeout: A timeout is set to schedule the execution of func if the necessary time (limit) has passed since the last run.
• The timeout waits for limit - (Date.now() - lastRan) milliseconds, ensuring that the function runs exactly after the throttled interval.
How it works:
• Initial call: When the throttled function is first called, it executes the func immediately and stores the current time.
• Subsequent calls within the limit: If the function is triggered again before the limit time has passed, it won’t run immediately but will set a timeout to execute it after the remaining time.
• Execution after timeout: Once the remaining time has passed, func runs again, and the timer resets.
Imagine you want to attach this throttled function to a scroll event to avoid executing a heavy task too frequently when a user scrolls:
window.addEventListener('scroll', throttle(function() {
console.log('Scroll event triggered!');
}, 200)); // Limit function execution to once every 200 milliseconds.
This would ensure that the scroll event handler only runs at most once every 200 milliseconds, no matter how frequently the user scrolls.
Q: How would you implement throttling on a button press to prevent rapid, unintended multiple submissions in a React Native form?
import React from 'react';
import { Button } from 'react-native';
const ThrottledSubmitButton = () => {
const throttledSubmit = React.useCallback(
throttle(() => {
// Form submission logic here
console.log('Form submitted');
}, 1000),
[]
);
return <Button title="Submit" onPress={throttledSubmit} />;
};
Q. In a React Native app with a scrollable list, how can throttling improve the performance of scroll event handlers?
Throttling can improve scroll performance by limiting the frequency of scroll event handler executions. This is particularly useful for expensive operations like layout calculations or API calls. Here's an example:
import React from 'react';
import { FlatList } from 'react-native';
const ThrottledScrollList = () => {
const handleScroll = React.useCallback(
throttle((event) => {
// Perform expensive operation here
console.log('Scroll position:', event.nativeEvent.contentOffset.y);
}, 200),
[]
);
return (
<FlatList
data={someData}
renderItem={renderItem}
onScroll={handleScroll}
scrollEventThrottle={16} // Native driver throttle, different from JS throttle
/>
);
};
Q: Create a custom hook called useThrottle
that can be used to throttle any function in a functional component.
import { useCallback, useRef } from 'react';
function useThrottle(callback, limit) {
const lastRan = useRef(Date.now());
const lastFunc = useRef(null);
return useCallback((...args) => {
if (Date.now() - lastRan.current >= limit) {
callback(...args);
lastRan.current = Date.now();
} else {
clearTimeout(lastFunc.current);
lastFunc.current = setTimeout(() => {
callback(...args);
lastRan.current = Date.now();
}, limit - (Date.now() - lastRan.current));
}
}, [callback, limit]);
}
// Usage
const MyComponent = () => {
const throttledHandler = useThrottle(() => {
console.log('Throttled function called');
}, 1000);
return <Button onPress={throttledHandler} title="Throttled Action" />;
};
Q: You're building a React Native app with a real-time chat feature. Implement a throttled function that limits the rate of message sending to once every 500ms.
import React, { useState } from 'react';
import { TextInput, Button, View } from 'react-native';
const ChatInput = () => {
const [message, setMessage] = useState('');
const sendMessage = useThrottle((text) => {
// API call to send message
console.log('Sending message:', text);
setMessage(''); // Clear input after sending
}, 500);
return (
<View>
<TextInput
value={message}
onChangeText={setMessage}
placeholder="Type a message"
/>
<Button title="Send" onPress={() => sendMessage(message)} />
</View>
);
};
Q: How would you modify a throttle function to handle and propagate errors that might occur in the throttled function?
function throttleWithErrorHandling(func, limit) {
let lastRan = 0;
let lastError = null;
return function(...args) {
const context = this;
return new Promise((resolve, reject) => {
const now = Date.now();
if (now - lastRan >= limit) {
lastRan = now;
try {
const result = func.apply(context, args);
resolve(result);
} catch (error) {
lastError = error;
reject(error);
}
} else if (lastError) {
reject(lastError);
} else {
resolve(null);
}
});
};
}
Q: Implement a throttle function that includes a method to cancel the throttled operation if needed.
function throttleWithCancel(func, limit) {
let lastRan, lastFunc;
function throttled(...args) {
const context = this;
if (!lastRan) {
func.apply(context, args);
lastRan = Date.now();
} else {
clearTimeout(lastFunc);
lastFunc = setTimeout(function() {
if ((Date.now() - lastRan) >= limit) {
func.apply(context, args);
lastRan = Date.now();
}
}, limit - (Date.now() - lastRan));
}
}
throttled.cancel = function() {
clearTimeout(lastFunc);
lastRan = 0;
};
return throttled;
}
// Usage
const throttledFunc = throttleWithCancel(someFunction, 1000);
// ... later ...
throttledFunc.cancel(); // Cancels any pending execution
Q: In a React Native app using the PanResponder for a custom slider component, how would you use throttling to optimize the update frequency during a gesture?
import React, { useRef } from 'react';
import { View, PanResponder } from 'react-native';
const CustomSlider = () => {
const panResponder = useRef(
PanResponder.create({
onPanResponderMove: throttle((_, gestureState) => {
// Update slider value based on gestureState.dx
console.log('Slider value:', gestureState.dx);
}, 16) // Throttle to ~60fps
})
).current;
return <View {...panResponder.panHandlers} style={styles.slider} />;
};
Q: Explain how you would optimize a throttle function for memory usage in a long-running React Native application, especially when used with rapidly firing events like scroll or gesture updates.
To optimize a throttle function for memory usage in a long-running React Native app:
Use a shared throttle utility: Create a single throttle function that can be reused across components.
Clear timeouts and references: Ensure all timeouts are cleared and references are removed when components unmount.
Use WeakMap for memoization: If memoizing throttled functions, use WeakMap to allow garbage collection.
Avoid closures where possible: Minimize the use of closures that can lead to memory leaks.
Use React's useCallback and useMemo: These hooks can help prevent unnecessary re-creation of throttled functions.
Example of an optimized throttle utility:
const throttleMap = new WeakMap();
export function optimizedThrottle(func, limit, key = func) {
if (!throttleMap.has(key)) {
let lastRan = 0;
const throttled = function(...args) {
const context = this;
const now = Date.now();
if (now - lastRan >= limit) {
lastRan = now;
func.apply(context, args);
}
};
throttleMap.set(key, throttled);
}
return throttleMap.get(key);
}
// Usage in a component
const MyComponent = () => {
const throttledScroll = React.useMemo(() =>
optimizedThrottle(handleScroll, 200, 'scrollHandler'),
[]
);
React.useEffect(() => {
return () => {
throttleMap.delete('scrollHandler');
};
}, []);
return <ScrollView onScroll={throttledScroll} />;
};
These optimizations help manage memory usage by reducing the number of created functions and ensuring proper cleanup, which is crucial for long-running React Native applications dealing with frequent events.
We'll create a search component that throttles API calls as the user types, which is a common use case for throttling in real-world applications.
Let's build a search component that queries an API as the user types, but limits the rate of API calls using throttling. We'll use the following:
A custom throttle function
React Native's
TextInput
for user inputReact hooks for state management
A mock API call function
Here's the detailed implementation:
import React, { useState, useCallback, useRef } from 'react';
import { View, TextInput, Text, FlatList, StyleSheet } from 'react-native';
// Custom throttle function
const throttle = (func, limit) => {
let lastFunc;
let lastRan;
return function(...args) {
const context = this;
if (!lastRan) {
func.apply(context, args);
lastRan = Date.now();
} else {
clearTimeout(lastFunc);
lastFunc = setTimeout(function() {
if ((Date.now() - lastRan) >= limit) {
func.apply(context, args);
lastRan = Date.now();
}
}, limit - (Date.now() - lastRan));
}
}
};
// Mock API call
const searchAPI = async (query) => {
// Simulate API delay
await new Promise(resolve => setTimeout(resolve, 300));
// Mock results
return [`Result 1 for ${query}`, `Result 2 for ${query}`, `Result 3 for ${query}`];
};
const SearchComponent = () => {
const [query, setQuery] = useState('');
const [results, setResults] = useState([]);
const [isLoading, setIsLoading] = useState(false);
// Throttled search function
const throttledSearch = useRef(
throttle(async (text) => {
setIsLoading(true);
try {
const searchResults = await searchAPI(text);
setResults(searchResults);
} catch (error) {
console.error('Search error:', error);
setResults([]);
} finally {
setIsLoading(false);
}
}, 300) // Throttle to once every 300ms
).current;
// Handle text input changes
const handleSearchInput = useCallback((text) => {
setQuery(text);
if (text.length > 2) { // Only search if query is more than 2 characters
throttledSearch(text);
} else {
setResults([]);
}
}, []);
// Render each result item
const renderItem = ({ item }) => (
<Text style={styles.resultItem}>{item}</Text>
);
return (
<View style={styles.container}>
<TextInput
style={styles.input}
value={query}
onChangeText={handleSearchInput}
placeholder="Search..."
/>
{isLoading && <Text style={styles.loadingText}>Searching...</Text>}
<FlatList
data={results}
renderItem={renderItem}
keyExtractor={(item, index) => index.toString()}
style={styles.resultList}
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
padding: 20,
backgroundColor: '#f5f5f5',
},
input: {
height: 40,
borderColor: 'gray',
borderWidth: 1,
paddingHorizontal: 10,
marginBottom: 20,
borderRadius: 5,
},
loadingText: {
marginBottom: 10,
fontStyle: 'italic',
},
resultList: {
flex: 1,
},
resultItem: {
padding: 10,
borderBottomWidth: 1,
borderBottomColor: '#ddd',
},
});
export default SearchComponent;
Let's break down the key aspects of this implementation:
Custom Throttle Function:
We define a
throttle
function that limits how often the wrapped function can be called.It ensures the function is not called more than once in the specified time limit (300ms in this case).
Mock API:
searchAPI
simulates an asynchronous API call with a delay.In a real application, this would be replaced with actual API calls.
Component State:
query
: Stores the current search text.results
: Stores the search results.isLoading
: Indicates whether a search is in progress.
Throttled Search Function:
We create a throttled version of the search function using
useRef
to ensure it's not recreated on every render.This function is called at most once every 300ms, preventing rapid, successive API calls as the user types quickly.
Input Handling:
handleSearchInput
updates the query state and triggers the throttled search.We only search if the query is more than 2 characters long to avoid unnecessary API calls.
UI Rendering:
We use a
TextInput
for user input and aFlatList
to display results.A loading indicator is shown while the search is in progress.
Benefits of Throttling in this Example:
Performance Optimization: By limiting API calls to once every 300ms, we reduce the load on both the client and server, especially important for mobile devices with limited resources.
Better User Experience: The UI remains responsive as we're not blocking the main thread with excessive API calls.
Resource Conservation: Reduces unnecessary API calls, which is particularly important for mobile apps where data usage and battery life are concerns.
Cost Efficiency: For apps that pay per API call, throttling can significantly reduce costs by limiting the number of calls made.
To use this component in your React Native app, you would simply import and render it:
import React from 'react';
import { SafeAreaView } from 'react-native';
import SearchComponent from './SearchComponent';
const App = () => (
<SafeAreaView style={{ flex: 1 }}>
<SearchComponent />
</SafeAreaView>
);
export default App;
This example demonstrates a practical use of throttling in React Native, showing how it can be applied to real-world scenarios like search functionality to optimize performance and user experience.
Subscribe to my newsletter
Read articles from Saif directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
