A Beginner's Guide to Redux: What and Why You Need It

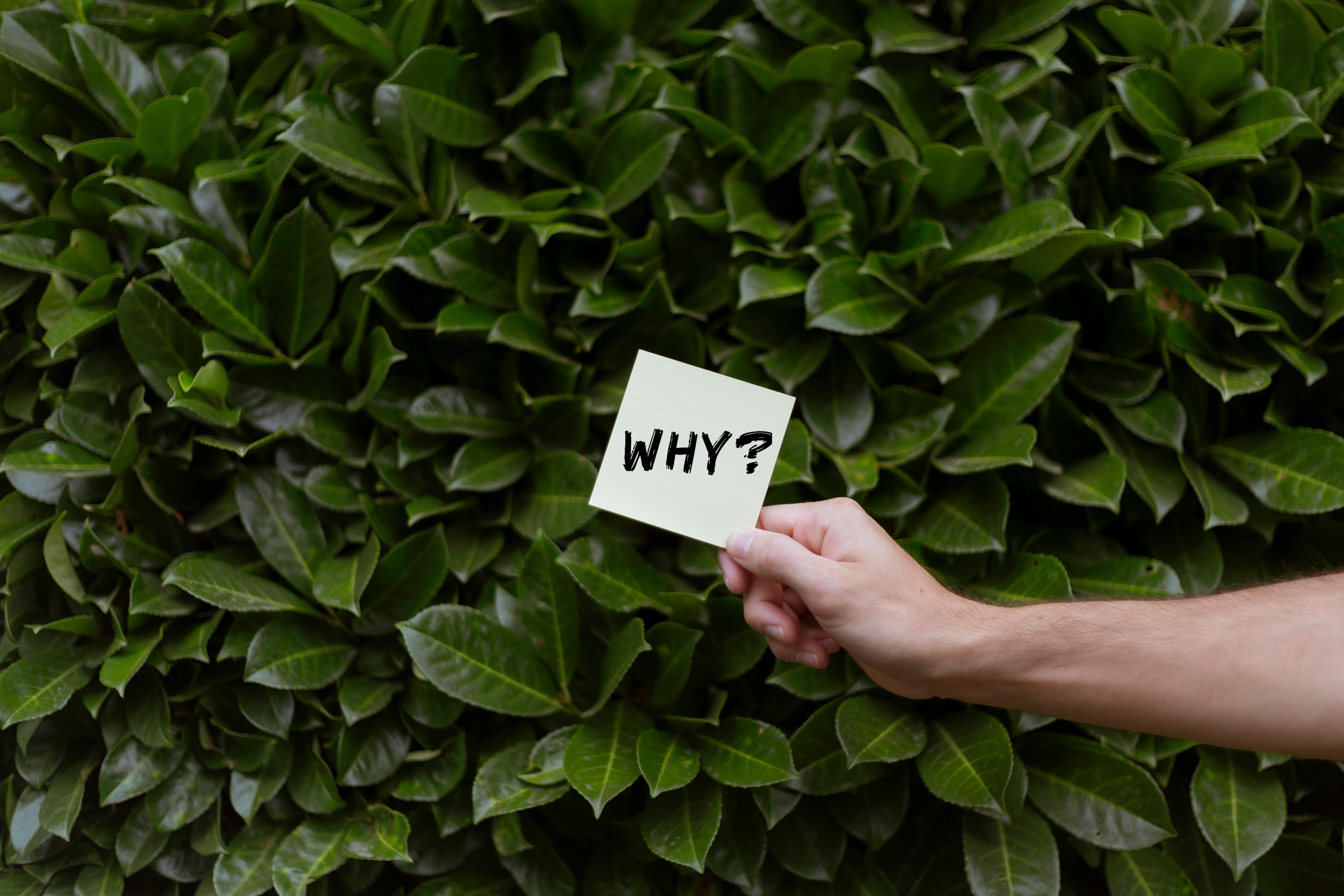
Redux is a tool in JavaScript that helps you manage all the information (or State) in your application in one central place. Its like a large shelf where all the important data your app uses are neatly arranged
Why Redux
Now , lets visualise something. Think of a kitchen representing you app. Each ingredient (flour, sugar, rice etc) represent pieces of information or state in your app.
Without Redux: A Messy Kitchen
In a kitchen without Redux, every cook (different parts of your app) keeps their own ingredients in random cupboards, drawers, or even on different counters. So when one cook needs sugar, they have no idea where it is—maybe it’s in a cupboard on the other side of the kitchen, or maybe someone used it and left it in the fridge by mistake. Trust me, It becomes pure chaos because:
Cooks waste time running around the kitchen to find ingredients.
One cook might accidentally take too much sugar, leaving none for the others.
Some cooks might even mess up the recipes because they don’t have access to the right ingredients.
This is how messed up React was before Redux. Developers had to rely on React’s inbuilt state and props to manage and pass data between components. However, as the applications became more complex, several challenges arose, especially props drilling and state synchronization across multiple components. Thanks to Dan Abramov and Andrew Clark, who created redux in 2015, we can now manage our data better. Lets take a look at our Kitchen with Redux
With Redux: An Organized Kitchen
Now that we introduce Redux into our kitchen, here is how things change
The Store is now the Pantry: Redux now gives us one central pantry(store), where all ingredients(or information or states) are stored. Instead of looking all over the place for our ingredients, every cook now knows they can go to the pantry to get what they need. The pantry is always organized and has everything in one place
Actions are Recipe Requests: When a cook needs to add something new to the pantry (like more flour) or change something (swap sugar for honey), they don’t just grab things randomly. Instead, they write a request (action) on a note: "We need more flour!" or "Change sugar to honey!"
Reducers are the Head Chefs: The pantry doesn’t update itself. Head chefs (reducers) get the note (action) and decide how to update the pantry. If the request is reasonable, they update the ingredient in the pantry, making sure everything stays organized.
Selectors are Measuring Cup: When a cook needs an ingredient, they use a measuring cup (selector) to take the right amount. Instead of taking too much or too little, they get exactly what they need from the pantry.
You can see how much more organized our kitchen is now with redux. Its a kitchen every chef wants to work in.Now, let's explore it with a bit more technical language.
Organised Memory mangement with Redux
As we mentioned in our kitchen example, there are four basic building blocks of Redux: Store, Actions, Reducers, and Selectors. Let's look at their definitions while building a small counter application.
Note: To use it in your local environment, you need to have Redux as a dependency. You can run npm install redux
or yarn add redux
, depending on your package manager. Now, let's dig in.
Store: The Store is the central place where all your app states are kept.Its like a database for your app where the global state is stored. You create the store by combining reducers that tell Redux how to change the state based on actions.
The “createStore” function takes a reducer and creates the Redux store.
The “store” will now hold the current counter value in its state.
Actions: Actions are plain JavaScript objects that describe what needs to change in the state. They are instructions that tell Redux what needs to happen. An action is an object with a type property that describes what kind of action it is, and optionally a payload with some data.
For the counter app, we need two actions: one to increment the counter and one to decrement it.
Here, the “increment” and “decrement” actions tell the reducer what should be done: increase or decrease the counter.
Reducers: Reducers are functions that listen for actions and decide how the state should change.Reducers take the current state and an action, and then return a new state based on that action. In our counter example, the reducer will decide what happens when the “Increment” or “Decrement” action is dispatched
The reducer listens for “Increment” or “Decrement” actions and updates the counter accordingly.
Selectors: Selectors are helper functions to extract specific pieces of state from the store.They are functions that help retrieve specific pieces of data from the store. In this case, we will create a selector to get the current value of the counter from the store.
- “getCounterValue” is a simple selector that returns the entire state because our state is just a single number (the counter). In more complex apps, the state might have more structure, like
{ counter: 0, user: {}, ... }
.
Using React with our Redux, you’ll connect Redux to components using “useDispatch” and “useSelector” from the "react-redux"
package.
useSelector
is used to read the current state (counter value) from the Redux store.useDispatch
is used to send actions (increment or decrement) to the Redux store.
Summary:
Store holds the global state (in this case, the counter).
Actions are objects that describe what should happen (like increment or decrement).
Reducers are functions that listen to actions and update the state accordingly.
React components use
useSelector
to get the state anduseDispatch
to dispatch actions.
Subscribe to my newsletter
Read articles from David Owolabi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
