Batch Normalization is like A Human Moderator
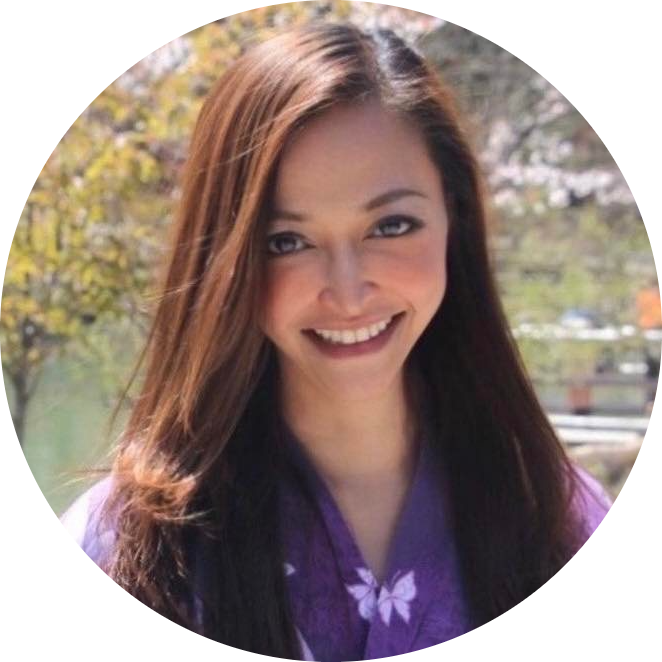
Imagine you're in a group project with 10 people, and each person has to contribute an idea (data) to make the final presentation (prediction). But there's a problem:
Some people talk too much, giving too many ideas.
Some people are too quiet, barely contributing anything.
Others are somewhere in between.
This creates imbalance. The group struggles to work together smoothly because some people's input is overwhelming, while others are not contributing enough.
Why is this a problem?
In neural networks, each layer takes input data from the previous layer. If the values coming in from different neurons (features) have very different scales (some are very large, some are very small), it causes:
Learning to slow down: The network has to adjust more to deal with large variations in input.
Difficulty in updating weights: During backpropagation (when the network learns from its mistakes), it can be harder to find the right adjustments for the weights if the input values are all over the place.
Vanishing/exploding gradients: If the input values are too small, the network might not learn anything. If they're too big, the network might "explode," leading to unstable learning.
How Normalization fixes this:
Normalization is like a moderator in the group project. The moderator ensures that:
Everyone talks the same amount: No one is too loud (large values) or too quiet (small values). Instead, everyone's contribution (input data) is adjusted to have a similar scale.
This makes it easier for everyone (neurons) to work together smoothly and learn faster.
How does it work in neural networks?
In the network, batch normalization standardizes the input values coming into a layer. This means it adjusts the data so that:
The mean (average) is zero: It centers the data around 0.
The variance (spread of the data) is one: It ensures that the data doesn't have too large or too small a range.
import torch # Batch Normalization Function def batchnorm(X, gamma, beta, eps=1e-5): # Calculate the mean and variance across the batch for each feature mean = X.mean(dim=0, keepdim=True) variance = X.var(dim=0, keepdim=True) # Normalize the input: (X - mean) / sqrt(variance + epsilon) X_normalized = (X - mean) / torch.sqrt(variance + eps) # Scale and shift the normalized input using gamma (scale) and beta (shift) out = gamma * X_normalized + beta return out # Example Usage # Suppose we have a batch of 3 data points with 4 features each X = torch.tensor([[1.0, 2.0, 3.0, 4.0], [5.0, 6.0, 7.0, 8.0], [9.0, 10.0, 11.0, 12.0]]) # Gamma and Beta: scaling and shifting parameters gamma = torch.ones(X.shape[1]) # Set gamma to ones for simplicity beta = torch.zeros(X.shape[1]) # Set beta to zeros for simplicity # Apply batch normalization output = batchnorm(X, gamma, beta) print("Input:\n", X) print("Normalized Output:\n", output)
Explanation of Code:
Mean and Variance Calculation:
We first calculate the mean and variance for each feature (along the batch dimension). This ensures we can standardize each feature (each column of the data).
mean = X.mean(dim=0, keepdim=True)
computes the mean for each feature across the batch (i.e., for each column in X).variance = X.var(dim=0, keepdim=True)
computes the variance for each feature across the batch.
Normalization:
We normalize the data using the formula:
Here,
eps
(epsilon) is a small value added to avoid division by zero.
Scaling and Shifting:
After normalization, we scale and shift the data using the learnable parameters
gamma
andbeta
. These parameters allow the model to adjust how much of the normalized data is used.If
gamma=1
andbeta=0
, the normalized data remains unchanged.`out = gamma * X_normalized + beta
What gamma and beta do?
1. The Mean (μ): Getting the Baseline of the Conversation
The mean (μ) gives the moderator a sense of the overall balance of the conversation. For example, if everyone speaks for 5 minutes on average, the mean is 5 minutes.
2. The Standard Deviation (σ): Measuring Imbalance
Now, the moderator measures how far each person’s contribution (talk time) is from that average.
If someone talks for 8 minutes, that’s 3 minutes above the average.
If someone talks for 2 minutes, that’s 3 minutes below the average.
The standard deviation (σ) captures this spread. A large standard deviation means the contributions vary a lot (some people talk much more than others), while a small deviation means everyone is speaking for about the same amount of time.
3. Normalization: Balancing Contributions
The moderator now normalizes the conversation. This means adjusting everyone’s contribution so that no one talks too much or too little. After normalization:
Each person’s contribution is adjusted to be closer to the average.
Those who were talking too much now talk less, and those who weren’t speaking enough now contribute more.
Mathematically, the moderator subtracts the mean (μ) from each person’s talk time and divides it by the standard deviation (σ), bringing everyone in line with the group average.
4. Gamma (γ): Adjusting the Emphasis
Once the conversation is normalized, the boss steps in. The boss looks at the normalized contributions and decides:
"The marketing team should have more weight in this meeting." So, the boss increases their contribution using gamma (γ).
Meanwhile, the HR team's input might be less relevant, so the boss scales down their contributions.
Gamma (γ) allows the network to emphasize or downplay certain features (or participants in the meeting) after normalization. Even though everyone is balanced, some voices may still carry more weight based on the context.
5. Beta (β): Shifting the Conversation
Now, the CEO enters the room and says, “Let’s shift our focus.” Even though everyone is contributing equally (thanks to the moderator and the boss), the CEO shifts the entire conversation to a new baseline (using beta (β)).
- If the CEO says, “Let’s focus more on sales,” then the whole conversation shifts, making sales the primary topic, even though everyone is still contributing equally.
This shift allows the conversation to be focused on different aspects of the business, even though it’s still balanced.
What code output should look like?
import torch
# Batch Normalization Function
def batchnorm(X, gamma, beta, eps=1e-5):
# Calculate the mean and variance across the batch for each feature
mean = X.mean(dim=0, keepdim=True)
variance = X.var(dim=0, keepdim=True)
# Normalize the input: (X - mean) / sqrt(variance + epsilon)
X_normalized = (X - mean) / torch.sqrt(variance + eps)
# Scale and shift the normalized input using gamma (scale) and beta (shift)
out = gamma * X_normalized + beta
return out
# Example Usage
# Suppose we have a batch of 3 data points with 4 features each
X = torch.tensor([[1.0, 2.0, 3.0, 4.0],
[5.0, 6.0, 7.0, 8.0],
[9.0, 10.0, 11.0, 12.0]])
# Gamma and Beta: scaling and shifting parameters
gamma = torch.ones(X.shape[1]) # Set gamma to ones for simplicity
beta = torch.zeros(X.shape[1]) # Set beta to zeros for simplicity
# Apply batch normalization
output = batchnorm(X, gamma, beta)
X = torch.tensor([[1.0, 2.0, 3.0, 4.0], [5.0, 6.0, 7.0, 8.0], [9.0, 10.0, 11.0, 12.0]])
The
batchnorm
function calculates the mean and variance of the features (columns) across the batch (rows). Then, it normalizes each feature using the formula:Mean of each column:
[5.0, 6.0, 7.0, 8.0]
Variance of each column:
[10.6667, 10.6667, 10.6667, 10.6667]
The output is the normalized version of the input, scaled by gamma
(which is [1, 1, 1, 1]
in this case) and shifted by beta
(which is [0, 0, 0, 0]
in this case).
For example:
For the first element in the first column:
This will be repeated for all the elements in the tensor, giving us a normalized output tensor. Let me now try to show a typical output manually.
Output (approximate values):
Normalized Output:
[[-1.2247, -1.2247, -1.2247, -1.2247],
[ 0.0000, 0.0000, 0.0000, 0.0000],
[ 1.2247, 1.2247, 1.2247, 1.2247]]
Subscribe to my newsletter
Read articles from Anix Lynch directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
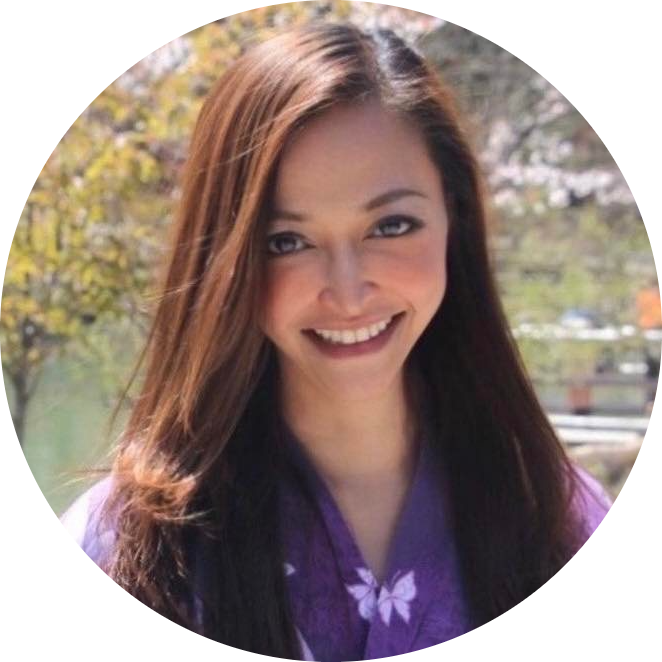