React Native Architecture: A Deep Dive

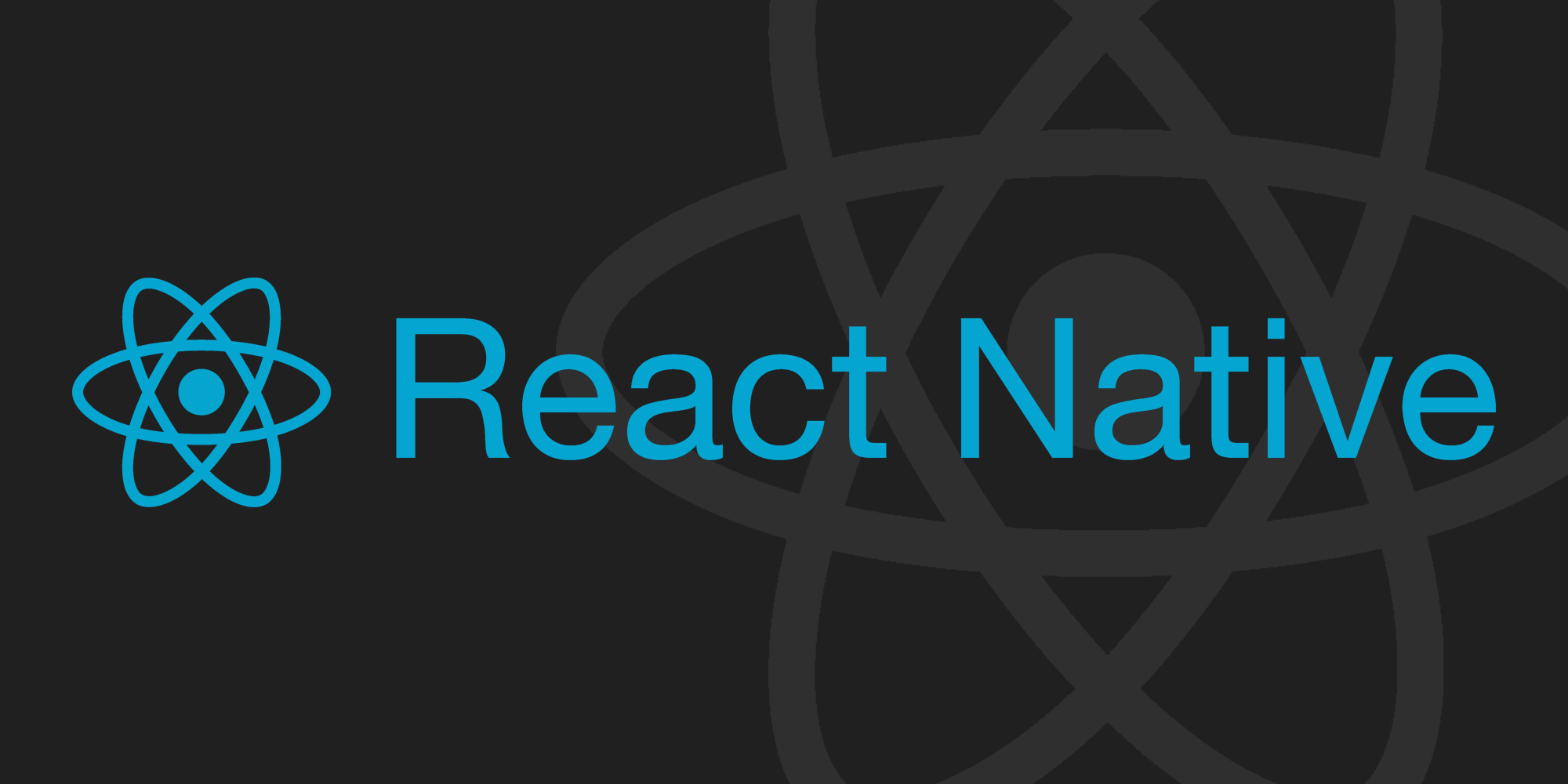
I. Introduction
Brief Overview of React Native
React Native, first released by Facebook in 2015, has revolutionized mobile app development by allowing developers to build native mobile applications using JavaScript and React. This open-source framework enables the creation of apps for multiple platforms (iOS, Android, and more) with a single codebase, significantly reducing development time and costs.
Key features of React Native include:
Cross-platform development: Write once, run on multiple platforms.
Native components: Utilizes the same building blocks as regular iOS and Android apps.
Hot Reloading: Allows developers to see changes in real-time without rebuilding the entire app.
Large community and ecosystem: Extensive libraries and third-party plugins available.
Performance: Near-native performance due to optimized native components.
React Native has been adopted by many major companies, including Facebook, Instagram, Walmart, and Airbnb, demonstrating its capability to produce high-quality, performant mobile applications at scale.
The Need for Architectural Evolution
While React Native has been successful, its original architecture, designed in 2015, began to show limitations as mobile devices became more powerful and user expectations for app performance increased. Several factors drove the need for architectural evolution:
Performance Bottlenecks: The original architecture relied heavily on the bridge for communication between JavaScript and native code. This asynchronous, serialization-based communication became a performance bottleneck, especially for apps with complex UI or heavy data processing.
Initialization Time: As apps grew larger and more complex, the time taken to initialize all native modules at startup increased, leading to slower app launch times.
Asynchronous Layout: The asynchronous nature of layout calculations sometimes led to UI inconsistencies and flickering.
Limited Access to Native Features: The bridge-based architecture made it challenging to work with certain native APIs, especially those requiring synchronous access.
Scaling Challenges: As apps scaled in complexity, the original architecture made it difficult to optimize performance without significant workarounds.
Evolving Mobile Landscape: The rapid evolution of mobile hardware and software necessitated an architecture that could better leverage new capabilities.
Developer Experience: There was a need to improve debugging, testing, and the overall developer experience, especially for larger teams and more complex projects.
To address these challenges and set React Native up for long-term success, the React Native team at Facebook began work on a new architecture. This architectural overhaul, known as the "New Architecture," aims to resolve these limitations while maintaining the core principles that made React Native popular.
In the following sections, we'll explore both the old and new architectures in detail, with a particular focus on the transformative changes introduced in the new architecture and their implications for React Native developers.
II. Old Architecture (Pre-2022)
A. Overview
The old React Native architecture, which was the foundation of the framework from its inception in 2015 until the rollout of the new architecture in 2022, was based on a three-thread model. This design was crucial in enabling React Native to bridge the gap between JavaScript and native platforms while maintaining good performance. The three main threads in this architecture were:
JavaScript (JS) Thread: Responsible for executing JavaScript code, including the React application logic.
Native Thread: Handles rendering of native components and executes native module operations.
Shadow Thread: Performs layout calculations using the Yoga layout engine.
This multi-threaded approach allowed React Native to perform complex JavaScript operations without blocking the UI, while still providing a native look and feel to the applications.
B. Key Components
1. JavaScript Core
At the heart of React Native's old architecture was JavaScriptCore, the JavaScript engine that powers the framework.
Key aspects of JavaScriptCore in React Native:
Execution of JS Code: JavaScriptCore runs all the JavaScript code of the React Native application. This includes the React component logic, state management, and any other JavaScript-based business logic.
React Reconciliation: The process of determining what changes need to be made to the UI based on the current and previous states of the application. This reconciliation process, a core part of React, runs entirely in the JavaScript thread.
Virtual DOM: React Native uses a virtual DOM (Document Object Model) in JavaScript to represent the structure of the UI. The reconciliation process compares the previous virtual DOM with the new one to determine necessary updates.
2. Bridge
The Bridge was a crucial component in the old architecture, serving as the communication layer between the JavaScript realm and the Native realm.
Key features of the Bridge:
Serialization and Deserialization: All data passing through the Bridge had to be serialized on the sending side and deserialized on the receiving side. This process converted complex JavaScript objects into a format that could be understood by native code, and vice versa.
Asynchronous Communication: The Bridge operated asynchronously, meaning that calls from JavaScript to Native (and back) didn't block the execution of either side. This ensured smooth performance but also introduced complexity in handling time-sensitive operations.
Batched Updates: To optimize performance, the Bridge batched multiple messages together before sending them across the thread boundary. This reduced the overhead of frequent small communications but could sometimes lead to delays in updates.
3. Native Modules
Native Modules provided a way to write platform-specific code and expose it to JavaScript.
Characteristics of Native Modules:
Platform-Specific Code: Native Modules were written in the native language of the platform - Objective-C or Swift for iOS, and Java or Kotlin for Android.
Bridged API: These modules exposed an API that could be called from JavaScript. The Bridge handled the communication between JavaScript calls and the native implementation.
Access to Platform Features: Native Modules allowed React Native applications to access platform-specific features that weren't available in JavaScript, such as specific hardware functionalities or third-party SDKs.
4. Shadow Thread
The Shadow Thread was responsible for handling layout calculations in React Native applications.
Key aspects of the Shadow Thread:
Yoga Layout Engine: The Shadow Thread used Yoga, a cross-platform layout engine developed by Facebook. Yoga implements a subset of Flexbox, allowing for consistent layouts across different platforms.
Asynchronous Layout Calculation: Layout calculations were performed asynchronously to the main thread, which helped in maintaining UI responsiveness, especially for complex layouts.
Translation to Native Layouts: Once the layout was calculated, the results were sent to the Native Thread to be applied to the actual native views.
This architecture allowed React Native to achieve its goal of enabling cross-platform development with native performance. However, as applications grew more complex and performance demands increased, limitations of this architecture, particularly around the Bridge, began to surface, leading to the development of the new architecture.
C. Data Flow
The data flow in the old React Native architecture was primarily centered around the Bridge, which facilitated communication between the JavaScript realm and the Native realm. Understanding this flow is crucial to appreciating both the capabilities and limitations of the old architecture.
1. JS to Native
When an action in the JavaScript code needed to interact with native components or APIs, the following process occurred:
JavaScript Execution: The action (e.g., a state update triggering a UI change) is executed in the JavaScript thread.
Serialization: The JavaScript object or message is serialized into a format that can be sent across the Bridge.
Queueing: The serialized message is queued to be sent over the Bridge.
Bridge Crossing: At the next opportunity (often batched with other messages for efficiency), the message is sent across the Bridge to the Native side.
Deserialization: On the Native side, the message is deserialized back into a format the Native code can understand.
Native Execution: The corresponding Native code is executed, such as updating UI components or calling platform APIs.
2. Native to JS
Communication from Native to JavaScript followed a similar but reverse process:
Native Event: A native event occurs (e.g., a touch event or a callback from a Native Module).
Serialization: The native data is serialized for transmission across the Bridge.
Bridge Crossing: The serialized data is sent across the Bridge to the JavaScript side.
Deserialization: On the JavaScript side, the data is deserialized into JavaScript objects.
JavaScript Execution: The relevant JavaScript code (e.g., event handlers) is executed in response to the native event.
3. Layout Process
The layout process in React Native's old architecture involved both the JavaScript and Native realms, with the Shadow Thread playing a crucial role:
React Rendering: The React component tree is rendered in the JavaScript thread, producing a description of the desired layout.
Shadow Tree Creation: This layout description is sent to the Shadow Thread, where a shadow tree of native components is created or updated.
Yoga Calculation: The Yoga engine, running on the Shadow Thread, calculates the precise layout based on the shadow tree and flexbox rules.
Layout Application: The calculated layout is sent to the Native Thread, where it's applied to the actual native views.
Rendering: The native views are rendered on screen according to the calculated layout.
D. Limitations
While the old architecture of React Native was groundbreaking and enabled the development of many successful apps, it had several limitations that became more pronounced as apps grew in complexity and user expectations increased.
Performance Bottlenecks
Bridge Overhead: Every communication between JavaScript and Native incurred the overhead of serialization, deserialization, and message passing across the Bridge. For apps with frequent JS-to-Native communication, this could lead to performance issues.
Batching Delays: While batching messages helped reduce Bridge crosses, it could also introduce delays in updates, leading to perceived lag in UI responses.
Single JavaScript Thread: All JavaScript operations ran on a single thread, which could lead to bottlenecks in CPU-intensive applications.
Asynchronous Communication Issues
Callback Hell: The asynchronous nature of Bridge communication often led to complex chains of callbacks, making code harder to write and maintain.
Race Conditions: The asynchronous and batched nature of updates could sometimes lead to race conditions, where the order of operations became unpredictable.
Difficulty with Synchronous APIs: Some native APIs require synchronous responses, which were challenging to implement given the asynchronous nature of the Bridge.
Initialization Time
Upfront Module Loading: All native modules had to be initialized at app startup, even if they weren't immediately used. This could lead to longer app launch times, especially for larger apps with many modules.
JavaScript Bundle Load Time: The initial loading and parsing of the JavaScript bundle could cause noticeable delays in app startup, particularly on lower-end devices.
Bridge Initialization: The time taken to set up the Bridge and establish the communication channel between JavaScript and Native added to the initialization overhead.
These limitations, while not insurmountable, began to pose significant challenges as React Native applications grew in complexity and scale. They were key drivers in the decision to develop a new architecture that could address these issues while maintaining the core benefits of React Native.
Real-World Examples of React Native Old Architecture Limitations
- Facebook Ads Manager
Facebook's Ads Manager app was one of the early adopters of React Native. While it generally performed well, the team encountered issues with the Bridge becoming a bottleneck when handling large datasets.
Problem: When users interacted with campaigns containing thousands of ads, the app would struggle to maintain smooth performance due to the high volume of data passing through the Bridge.
Impact: Users experienced lag when scrolling through large lists or applying filters to massive datasets.
Workaround: The team had to implement complex data virtualization and lazy loading techniques to minimize the amount of data crossing the Bridge at any given time.
- Airbnb's React Native Experience
Airbnb, which was one of the most prominent adopters of React Native, ultimately decided to move away from it in 2018. Their experience highlighted several limitations of the old architecture.
Problem: Initialization times for their app became problematic as the codebase grew.
Impact: Users experienced longer app startup times, especially on lower-end Android devices.
Issue: The need to initialize all native modules at startup, regardless of whether they were immediately needed, contributed to this problem.
- Discord's Android App
Discord's experience with React Native on Android showcased the challenges with the asynchronous nature of the old architecture.
Problem: Certain UI interactions, particularly those involving complex animations or rapid user input, suffered from noticeable lag due to the round-trip communication through the Bridge.
Impact: Users reported a less fluid experience compared to the iOS version or native Android apps.
Workaround: The team had to carefully optimize their JavaScript code and, in some cases, reimplement critical sections in native code to bypass the Bridge.
- Bloomberg's Consumer Mobile App
Bloomberg's app demonstrated how the single JavaScript thread could become a bottleneck in data-intensive applications.
Problem: When processing large amounts of financial data and updating multiple UI components simultaneously, the app would sometimes become unresponsive.
Impact: Users experienced freezes or significant lag when rapidly switching between different financial instruments or loading data-heavy screens.
Workaround: The team had to implement careful JavaScript optimizations and move some data processing to native modules to alleviate pressure on the JS thread.
- Instagram's Performance Challenges
Even though Instagram (owned by Facebook) had the advantage of direct access to React Native's core developers, they still faced challenges with the old architecture.
Problem: As the app grew in complexity, the team found it increasingly difficult to maintain smooth performance, especially during transitions between screens or when loading media-rich content.
Impact: Users on older devices or with slower internet connections experienced jittery animations and delayed content loading.
Solution: This was one of the driving forces behind Facebook's push to develop the new React Native architecture, aiming to address these performance bottlenecks at a fundamental level.
These real-world examples illustrate how the limitations of the old React Native architecture manifested in actual, widely-used applications. They highlight the practical challenges developers faced and the types of workarounds they had to implement. More importantly, they underscore why the development of a new architecture was necessary to address these fundamental limitations and improve the overall performance and developer experience of React Native applications.
III. New Architecture (2022 onwards)
A. Overview
React Native's new architecture, introduced in 2022, represents a significant leap forward in addressing the limitations of the old architecture while enhancing performance and developer experience. This architectural overhaul aims to bring React Native closer to native performance and capabilities, making it an even more compelling choice for cross-platform mobile development.
Improved Performance and Synchronous Communication
The new architecture significantly improves performance through several key enhancements:
Synchronous Communication: Unlike the old architecture's asynchronous bridge, the new architecture allows for direct, synchronous communication between JavaScript and native code.
Reduced Overhead: By eliminating the need for serialization and deserialization of data between JS and native, the new architecture reduces communication overhead.
Improved Threading Model: The new architecture makes better use of multiple threads, allowing for more efficient execution of tasks.
Faster Initialization: With lazy loading of native modules (TurboModules), app startup times are significantly reduced.
Key Pillars of the New Architecture
The new React Native architecture is built on four main pillars:
JavaScript Interface (JSI): A low-level interface that allows JavaScript to hold and manipulate native C++ objects.
Fabric: A new rendering system that improves UI performance and enables better integration with native UI components.
TurboModules: A system for more efficient native module loading and execution.
CodeGen: An automatic code generation tool that creates type-safe interfaces between JavaScript and native code.
These pillars work together to create a more performant, flexible, and developer-friendly framework.
B. JavaScript Interface (JSI)
The JavaScript Interface (JSI) is a crucial component of the new architecture, fundamentally changing how React Native bridges the gap between JavaScript and native code.
1. Direct Communication between JS and Native
JSI enables direct communication between JavaScript and native code (C++), eliminating the need for the bridge used in the old architecture.
Key aspects of this direct communication:
No Message Passing: Instead of passing messages through a bridge, JSI allows JavaScript to directly call C++ functions and vice versa.
Shared Memory Space: JavaScript and C++ can now share memory, allowing for more efficient data handling.
Lower Latency: Direct function calls significantly reduce the latency of JS-to-native operations.
2. Elimination of Serialization/Deserialization
One of the most significant performance improvements in the new architecture comes from eliminating the need to serialize and deserialize data when communicating between JavaScript and native code.
Benefits of this elimination:
Reduced Overhead: No need to convert complex objects into JSON and back, significantly reducing processing time and memory usage.
Handling of Complex Data Structures: Ability to pass more complex data structures between JS and native without the limitations imposed by JSON serialization.
Improved Performance for Data-Intensive Operations: Operations that require frequent JS-native communication (e.g., animations, gesture handling) become much more efficient.
3. Host Objects and Functions
JSI introduces the concept of "host objects" and "host functions," which are C++ objects and functions that can be directly accessed and called from JavaScript.
Key features of host objects and functions:
Direct Manipulation: JavaScript can directly manipulate C++ objects, allowing for more complex and efficient interactions with native components.
Type Safety: Host objects can be strongly typed, reducing runtime errors and improving developer experience.
Performance Optimization: Frequently used native functionalities can be exposed as host objects, allowing for highly optimized performance-critical operations.
Extended JavaScript Capabilities: JSI allows JavaScript to perform operations that were previously impossible or inefficient, such as direct memory manipulation or use of native APIs.
Example of using a host object in JavaScript:
javascriptCopy// Hypothetical example
const nativeModule = global.nativeModules.getModule('ExampleModule');
const result = nativeModule.performComplexOperation(param1, param2);
In this example, nativeModule
is a host object that JavaScript can interact with directly, without going through a bridge or requiring serialization.
The introduction of JSI in the new React Native architecture represents a paradigm shift in how hybrid applications can be built. By allowing JavaScript to directly interact with native code, JSI opens up new possibilities for performance optimization and feature implementation, bridging the gap between the flexibility of JavaScript and the power of native platform capabilities.
React Native New Architecture: A Comprehensive Overview
Comparison Table: Old vs New Architecture
Code Snippets: Interacting with JSI
Old Architecture (Using Bridge):
javascriptCopy// Calling a native module
import { NativeModules } from 'react-native';
const result = await NativeModules.MyModule.performOperation(data);
console.log(result);
New Architecture (Using JSI):
javascriptCopy// Direct interaction with a native module via JSI
const MyModule = global.nativeModules.getModule('MyModule');
const result = MyModule.performOperation(data);
console.log(result);
// Using a host object
const nativeObject = MyModule.getNativeObject();
nativeObject.updateValue(10); // Direct manipulation of native object
Migration Implications and Preparation
Gradual Adoption: React Native team is providing a way for gradual adoption. Developers can start using parts of the new architecture while keeping others on the old system.
Audit Native Modules: Review all native modules in your application. Custom modules will need to be converted to the new TurboModule system.
Update Dependencies: Ensure all third-party libraries are compatible with the new architecture. Many popular libraries are already working on compatibility.
Fabric Preparation: For custom UI components, prepare to migrate to the new Fabric rendering system. This may involve rewriting some native code.
Use CodeGen: Start incorporating CodeGen into your development process. This tool will help in creating type-safe interfaces between JS and native code.
Performance Profiling: Conduct thorough performance profiling of your app to identify areas that will benefit most from the new architecture.
Training and Education: Invest in training your team on the new concepts, especially JSI and the new threading model.
Challenges and Potential Drawbacks
Learning Curve: The new architecture introduces complex concepts like JSI, which may require significant learning for teams.
Migration Effort: For large, existing applications, migration to the new architecture can be a substantial undertaking.
Potential for Memory Leaks: With direct memory access in JSI, there's an increased risk of memory leaks if not managed properly.
Debugging Complexity: While offering more power, the new architecture can make debugging more complex, especially for JSI interactions.
Increased Native Knowledge Required: Developers may need a deeper understanding of native iOS and Android concepts to fully leverage the new architecture.
Potential Performance Overhead: In some cases, the overhead of JSI could potentially be higher than the bridge for very simple operations.
Community Support: Initially, there may be less community support and fewer resources available compared to the well-established old architecture.
New Tools and Debugging Techniques
React Native Debugger Updates: The popular React Native Debugger is being updated to support the new architecture, including JSI interactions.
Flipper Plugin for New Architecture: Facebook's Flipper debugging platform is getting plugins specifically designed for debugging Fabric and TurboModules.
Native Debugging Tools: With more native interactions, tools like Xcode Instruments for iOS and Android Profiler become more relevant.
JSI Console: A new JSI-based console is being developed, offering more powerful logging capabilities.
Fabric Inspector: A new tool for inspecting the Fabric rendering tree and performance.
TurboModules Inspector: Allows developers to inspect and debug TurboModules at runtime.
CodeGen CLI: A command-line tool for generating type-safe interfaces between JS and native code.
By understanding these aspects of the new React Native architecture, developers can better prepare for the transition and take full advantage of the improvements it offers. While there are challenges, the benefits in terms of performance and capabilities make the new architecture a significant step forward for React Native development.
C. Fabric (New Rendering System)
Fabric is React Native's new rendering system, designed to improve UI performance and enable better integration with native components.
1. C++ Core for Cross-Platform Consistency
Fabric's core is written in C++, providing a unified codebase for both iOS and Android.
This approach ensures consistent behavior across platforms and simplifies maintenance.
The C++ core allows for better performance optimizations and more efficient memory management.
2. Three Phases: Render, Commit, Mount
Fabric's rendering process is divided into three distinct phases:
a) Render Phase:
React components are rendered to produce a tree of React elements.
This phase is similar to the render phase in the old architecture.
b) Commit Phase:
The React element tree is translated into a C++ shadow tree.
This phase is where Fabric differs significantly from the old architecture.
The shadow tree contains all the information needed to create or update native views.
c) Mount Phase:
The shadow tree is used to create or update native views.
This phase is optimized for performance, with minimal work done on the main thread.
3. Shadow Tree in C++
The shadow tree is a lightweight representation of the UI in C++.
It allows for efficient updates and calculations without involving the more expensive native view operations.
The shadow tree can be manipulated and measured without affecting the actual UI, enabling more efficient layout calculations.
4. Improved Layout Performance with Yoga
Fabric continues to use Yoga for layout calculations, but with improved integration.
Layout calculations can now be performed more efficiently in C++, reducing the need for context switching.
The tighter integration with Yoga allows for more optimized layout updates and animations.
D. TurboModules
TurboModules represent a significant improvement in how React Native handles native modules.
1. Lazy Loading of Native Modules
Unlike the old architecture where all native modules were loaded at startup, TurboModules are loaded on-demand.
This lazy loading approach significantly reduces app startup time, especially for larger apps with many modules.
Modules are instantiated only when they're first accessed from JavaScript.
2. Type-Safe Interface Between JS and Native
TurboModules use CodeGen to create type-safe interfaces between JavaScript and native code.
This type safety helps catch errors at compile-time rather than runtime, improving app stability.
The interfaces are generated based on JavaScript specifications, ensuring consistency between JS and native sides.
3. Reduced Startup Time
By lazy-loading modules and eliminating the need for a separate native module registry, TurboModules significantly reduce app startup time.
The reduction in startup time is especially noticeable in larger apps with many native modules.
E. CodeGen
CodeGen is an automatic code generation tool that bridges the gap between JavaScript, C++, and native code.
1. Automated Code Generation for Type Definitions
CodeGen creates type definitions based on JavaScript specifications.
These type definitions are used in both JavaScript (for TypeScript support) and native code.
This automation ensures consistency between JS and native interfaces.
2. Bridging JS, C++, and Native Code
CodeGen generates the necessary C++ and native code (Objective-C for iOS, Java for Android) to bridge JavaScript and native functionality.
This generated code handles the conversion between JS and native types, method calls, and error handling.
3. Reduced Manual Coding and Error Potential
By automating the creation of bridging code, CodeGen significantly reduces the amount of boilerplate code developers need to write.
This automation also reduces the potential for errors in interface definitions and type conversions.
F. Hermes JavaScript Engine
Hermes is a JavaScript engine optimized for React Native, offering significant performance improvements.
1. Optimized for React Native
Hermes is designed specifically for the needs of React Native applications.
It focuses on improving the performance of React Native-specific patterns and operations.
2. Improved Start-up Time and Memory Usage
Hermes uses ahead-of-time (AOT) compilation to precompile JavaScript into efficient bytecode.
This precompilation results in faster app startup times and reduced memory usage.
Hermes also implements various optimizations like string interning and lazy parsing to further improve performance.
3. Integration with New Architecture Components
Hermes is designed to work seamlessly with JSI, Fabric, and TurboModules.
Its integration with these components allows for even greater performance optimizations in the new architecture.
G. Data Flow in New Architecture
The new architecture introduces a more efficient and flexible data flow model.
1. JS to C++ to Native (and vice versa)
In the new architecture, data flows from JavaScript to C++ (via JSI) and then to native code.
This flow is bidirectional, allowing for efficient communication in both directions.
The C++ layer acts as an intermediary, providing type safety and performance optimizations.
2. Synchronous Communication Pathways
Unlike the old architecture's asynchronous bridge, the new architecture allows for synchronous communication between JavaScript and native code.
This synchronous communication is made possible by JSI and the C++ core of Fabric and TurboModules.
Synchronous communication reduces latency and simplifies certain types of operations, especially those related to UI updates.
3. Performance Optimizations
The new data flow allows for various performance optimizations:
Reduced overhead by eliminating serialization/deserialization.
More efficient memory management through shared memory between JS and native.
Ability to batch certain operations for improved efficiency.
Lazy evaluation of certain operations, reducing unnecessary work.
This new architecture, with its improved rendering system, efficient module loading, automated code generation, optimized JavaScript engine, and streamlined data flow, represents a significant evolution in React Native's capabilities. It addresses many of the performance and flexibility limitations of the old architecture while providing developers with powerful new tools and abstractions.
React Native New Architecture: Enhanced Explanation of Key Components
Fabric (New Rendering System)
Comparison with Old Architecture
In the old architecture, the rendering process was managed by the JavaScript thread, with layout calculations happening on a separate shadow thread. This approach often led to performance issues, especially for complex UIs or when frequent updates were required.
Old Architecture:
CopyJavaScript Thread -> Bridge -> Shadow Thread -> Main Thread
New Architecture with Fabric:
CopyJavaScript -> JSI -> C++ Fabric Core -> Main Thread
Real-World Example
Consider an app with a complex, constantly updating UI, like a real-time collaborative whiteboard. In the old architecture, each update would require multiple thread jumps and potentially cause jank in the UI.
With Fabric, the direct communication between JavaScript and C++ allows for more efficient updates. The C++ shadow tree can quickly process changes and apply them to the native UI, resulting in smoother real-time collaboration experiences.
Quote from React Native Team
"Fabric is not just an optimization; it's a reimagining of how React Native can interact with host platforms. It opens up possibilities for deeper integration and higher performance that were simply not possible before."
-- Sophie Alpert, former React Core team member
Step-by-Step Explanation of Fabric's Three Phases
Imagine an animation with three main sections: JS Realm, C++ Realm, and Native Realm. The animation would proceed as follows:
Render Phase
Show React components in the JS Realm transforming into a tree of React elements.
Highlight that this process is similar to the old architecture.
Commit Phase
Animate the React element tree moving from the JS Realm to the C++ Realm.
Show the transformation of React elements into C++ objects forming the shadow tree.
Highlight that this shadow tree contains all layout information.
Mount Phase
Animate the C++ shadow tree reaching into the Native Realm.
Show native UI components popping up or updating based on the shadow tree.
Highlight the efficiency of this process, with minimal main thread work.
The animation could use color coding (e.g., blue for JS, green for C++, red for native) to make the flow clear. It could also show a performance graph at the bottom, illustrating how this process reduces main thread load compared to the old architecture.
TurboModules
Comparison with Old Architecture
In the old architecture, all native modules were initialized at app startup, regardless of whether they were immediately needed. This led to longer startup times, especially for apps with many modules.
Old Architecture:
CopyApp Start -> Initialize All Native Modules -> App Ready
New Architecture with TurboModules:
CopyApp Start -> Initialize JS -> Load Modules as Needed -> Improved App Ready Time
Real-World Example
Consider a React Native app that includes functionality for in-app purchases, push notifications, and AR features. In the old architecture, all these modules would be initialized at startup, even if the user only needed the basic features initially.
With TurboModules, the app can load only the essential modules at startup, dramatically reducing initial load time. The AR features, for instance, would only be loaded when the user navigates to that part of the app.
Quote from React Native Team
"TurboModules represent a fundamental shift in how we think about native modules in React Native. They're not just faster; they're smarter, loading exactly what you need when you need it."
-- Héctor Ramos, React Native Core team member
Practical Implementation Example
Here's a simplified example of how a TurboModule might be defined and used:
javascriptCopy// TurboModule specification (in JavaScript)
export interface Spec extends TurboModule {
multiply(a: number, b: number): Promise<number>;
}
// Using the TurboModule
import { TurboModuleRegistry } from 'react-native';
const mathModule = TurboModuleRegistry.get<Spec>('MathModule');
const result = await mathModule.multiply(5, 3);
console.log(result); // Outputs: 15
In this example, the MathModule
is only loaded when it's first accessed, not at app startup. The type-safe interface ensures that the correct types are used when calling the native method.
These enhancements to Fabric and TurboModules explanations provide a clearer picture of how the new architecture improves upon the old one, with real-world examples and insights from the React Native team. The step-by-step explanation (or animation) of Fabric's phases helps visualize the complex process of rendering in the new architecture.
React Native: Comparative Analysis, Migration, and Future Prospects
IV. Comparative Analysis: Old vs New Architecture
A. Performance
Start-up time:
Old: All native modules loaded at launch, increasing initial load time.
New: TurboModules load on-demand, significantly reducing startup time.
Runtime performance:
Old: Bridge serialization/deserialization caused latency in UI updates.
New: Direct communication via JSI allows for near-native performance in UI operations.
Memory usage:
Old: Separate memory spaces for JS and Native, with redundant data representations.
New: Shared memory through JSI reduces overall memory footprint.
B. Developer Experience
Debugging capabilities:
Old: Limited visibility into native side from JS debuggers.
New: Enhanced debugging with tools like Flipper, providing better insights into C++ and native code.
Testing improvements:
Old: Testing often required separate setups for JS and native components.
New: Improved integration testing capabilities with Fabric's C++ core.
Code reusability:
Old: Limited reusability between platforms due to platform-specific native modules.
New: Increased code sharing with C++ core in Fabric and TurboModules.
C. Cross-Platform Consistency
Shared C++ core benefits:
Fabric's C++ core ensures more consistent behavior across iOS and Android.
Reduced platform-specific bugs and inconsistencies.
Platform-specific optimizations:
While maintaining a shared core, the new architecture allows for platform-specific optimizations where needed.
Better utilization of platform-specific features without compromising cross-platform consistency.
V. Migration Considerations
Gradual Adoption Strategy
Opt-in Approach: The React Native team has designed the new architecture to allow gradual adoption.
Compatibility Mode: Existing components can run alongside new architecture components.
Incremental Migration: Developers can migrate feature by feature, starting with the most performance-critical ones.
Compatibility Layers
Bridge Compatibility: A compatibility layer allows old native modules to work with the new JSI.
Fabric Interop: Allows mixing of old and new renderer components during migration.
Community Support and Resources
Official Documentation: Comprehensive migration guides and best practices from the React Native team.
Community Plugins: Growing ecosystem of plugins and tools supporting the new architecture.
Case Studies: Emerging case studies from early adopters sharing migration experiences and best practices.
VI. Future Prospects
Ongoing Developments
Continued Performance Optimizations: Further improvements to Fabric and JSI for even better performance.
Enhanced Developer Tools: Ongoing development of debugging and profiling tools specific to the new architecture.
Expanded TurboModule Ecosystem: Growing number of core and third-party modules adopting the TurboModule system.
Community Feedback and Iterations
Open Development Process: React Native team actively seeking and incorporating community feedback.
Regular RFC Process: Continued use of Request for Comments (RFC) process for major feature additions and changes.
Collaborative Problem Solving: Community-driven solutions to challenges encountered in the new architecture.
Potential Impact on Mobile App Development Landscape
Narrowing the Gap: The new architecture narrows the performance gap between React Native and fully native development.
Attracting More Developers: Improved performance and developer experience may attract more developers to React Native.
Influencing Other Frameworks: The innovations in React Native's new architecture may influence other cross-platform frameworks.
VII. Conclusion
Recap of Key Differences
Transition from bridge-based to direct, synchronous communication (JSI).
Shift from JavaScript-centric to a more balanced JS-C++-Native architecture.
Move towards lazy loading and on-demand initialization of native modules.
Enhanced type safety and automated code generation for better reliability.
The Transformative Potential of the New Architecture
The new React Native architecture represents a significant leap forward in cross-platform mobile development. It addresses longstanding performance issues, improves developer experience, and provides a more robust foundation for building complex, high-performance mobile applications. This transformation positions React Native as an even more compelling option for businesses and developers looking to efficiently create native mobile experiences.
Encouragement for Developers to Explore and Adopt
As the new architecture matures, developers are encouraged to:
Start exploring the new concepts, particularly JSI, Fabric, and TurboModules.
Begin planning for migration, identifying which parts of their apps would benefit most from the new architecture.
Participate in the React Native community, sharing experiences and contributing to the ecosystem.
Consider the new architecture for upcoming projects to leverage its full potential from the start.
The journey to the new React Native architecture is not just an upgrade—it's a reimagining of what's possible in cross-platform mobile development. By embracing these changes, developers can create faster, more efficient, and more powerful mobile applications, pushing the boundaries of what's achievable with React Native.
React Native New Architecture: Myth vs. Reality
Myth 1: The new architecture is a complete rewrite of React Native.
Reality: While the new architecture introduces significant changes, it's not a complete rewrite. It's an evolution of React Native that maintains backwards compatibility while introducing new components like JSI, Fabric, and TurboModules. Many core concepts and much of the existing React Native codebase remain intact.
Myth 2: Adopting the new architecture requires rewriting your entire app.
Reality: The React Native team has designed the new architecture for gradual adoption. You can migrate your app piece by piece, starting with the most performance-critical parts. The new architecture includes compatibility layers that allow old and new components to work together during the transition.
Myth 3: The new architecture only benefits large, complex apps.
Reality: While complex apps may see the most dramatic improvements, the new architecture benefits apps of all sizes. Even simple apps can see improvements in startup time, runtime performance, and memory usage. The new architecture also provides a more solid foundation for future development, benefiting apps as they grow.
Myth 4: The new architecture eliminates the need for native developers.
Reality: While the new architecture does increase JavaScript developers' capabilities, it doesn't eliminate the need for native expertise. In fact, it opens up new possibilities for deeper native integration. Native knowledge is still valuable for optimizing performance, handling platform-specific features, and troubleshooting complex issues.
Myth 5: Debugging is more difficult with the new architecture.
Reality: While debugging processes have changed, new tools are being developed to make debugging more powerful and intuitive. Tools like Flipper provide enhanced debugging capabilities, including better insights into the C++ layer and native code. In many ways, debugging can be more straightforward due to the more direct communication between JavaScript and native code.
Myth 6: The new architecture is not stable enough for production use.
Reality: As of 2024, many companies have successfully adopted the new architecture in production apps. While it's true that the ecosystem is still evolving, the core components of the new architecture are stable and well-tested. The React Native team and community are actively working to ensure stability and provide support for production use.
Myth 7: TurboModules make all existing native modules obsolete.
Reality: While TurboModules offer significant advantages, they don't immediately obsolete existing native modules. Many popular native modules are being updated to support the new architecture, and there's a compatibility layer that allows existing modules to work alongside TurboModules during the transition period.
Myth 8: The new architecture solves all performance issues in React Native.
Reality: The new architecture provides substantial performance improvements, but it's not a magic solution for all performance issues. Good coding practices, efficient state management, and thoughtful app design are still crucial. The new architecture provides better tools and a more efficient foundation, but it's still up to developers to build performant apps.
Myth 9: Fabric makes platform-specific UI customizations impossible.
Reality: Fabric actually provides more opportunities for platform-specific optimizations, not fewer. Its C++ core ensures consistency where needed, but it also allows for platform-specific implementations when necessary. Developers can still create and use platform-specific UI components when required.
Myth 10: Learning the new architecture requires starting from scratch with React Native.
Reality: While the new architecture introduces new concepts, much of your existing React Native knowledge remains relevant. The core principles of React and the basic structure of React Native apps remain the same. Developers familiar with React Native will find that learning the new architecture is more about understanding new capabilities rather than relearning everything.
Subscribe to my newsletter
Read articles from Saif directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
