Control Structures in Shell Scripting

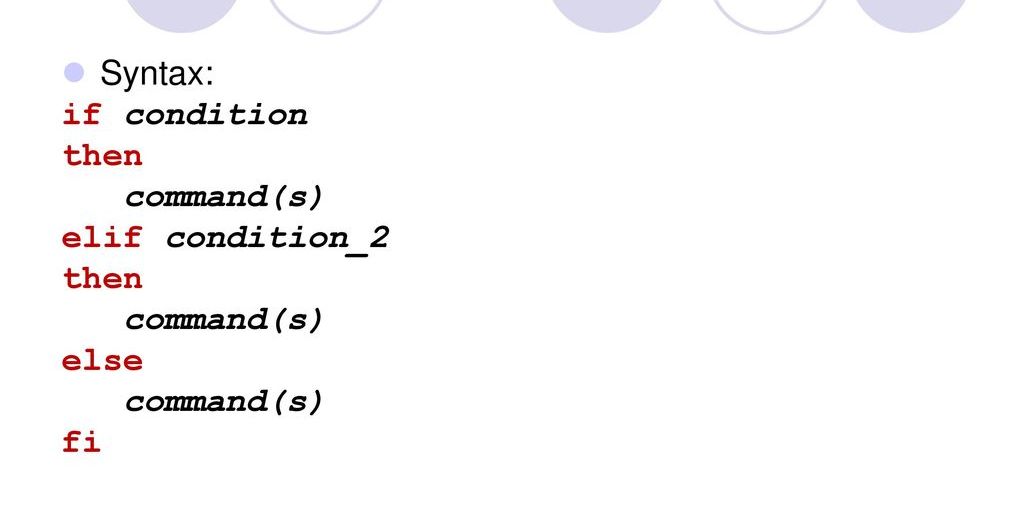
Control structures are essential components of shell scripting that allow you to control the flow of execution based on certain conditions or to repeat tasks. This blog post will explore conditional statements, loops, and case statements, providing you with the foundational knowledge needed to create more dynamic and powerful scripts.
1. Conditional Statements
Conditional statements enable your scripts to make decisions based on specified conditions. The primary conditional statements in shell scripting are if
, else
, and elif
.
Basic if
Statement
The simplest form of an if
statement checks if a condition is true.
if [ condition ]; then
# Commands to execute if condition is true
fi
Example:
number=5
if [ $number -gt 0 ]; then
echo "$number is positive."
fi
Output:
if-else
Statement
The else
clause allows you to define an alternative action if the condition is false.
if [ condition ]; then
# Commands if true
else
# Commands if false
fi
Example:
number=-3
if [ $number -gt 0 ]; then
echo "$number is positive."
else
echo "$number is not positive."
fi
Output:
elif
Statement
The elif
(else if) statement lets you check multiple conditions sequentially.
if [ condition1 ]; then
# Commands if condition1 is true
elif [ condition2 ]; then
# Commands if condition2 is true
else
# Commands if all conditions are false
fi
Example:
number=0
if [ $number -gt 0 ]; then
echo "$number is positive."
elif [ $number -lt 0 ]; then
echo "$number is negative."
else
echo "$number is zero."
fi
Output:
Condition Operators
Common condition operators include:
-eq
: Equal to-ne
: Not equal to-lt
: Less than-gt
: Greater than-le
: Less than or equal to-ge
: Greater than or equal to
2. Case Statements
The case
statement provides a way to execute different commands based on the value of a variable, allowing for multi-conditional branching.
Syntax:
case variable in
pattern1)
# Commands for pattern1
;;
pattern2)
# Commands for pattern2
;;
*)
# Default commands if no patterns match
;;
esac
Example:
fruit="apple"
case $fruit in
apple)
echo "It's an apple."
;;
banana)
echo "It's a banana."
;;
*)
echo "Unknown fruit."
;;
esac
Output:
3. Loops
Loops allow you to execute a block of code multiple times, making it easier to handle repetitive tasks. The most common types of loops in shell scripting are for, while, and until.
for
Loop
The for
loop is used to iterate over a list of items. It is particularly useful when you know the number of iterations beforehand.
Syntax:
for variable in list; do
# Commands to execute for each item
done
Example:
for i in {1..5}; do
echo "Number: $i"
done
In this example, the loop iterates over the sequence {1..5}
, and on each iteration, it prints the current value of i
.
Output:
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
while
Loop
The while
loop continues executing as long as a specified condition is true. It’s ideal when the number of iterations is not predetermined, and you want to repeat a task until a certain condition changes.
Syntax:
while [ condition ]; do
# Commands to execute while condition is true
done
Example:
count=1
while [ $count -le 5 ]; do
echo "Count: $count"
count=$((count + 1)) # Increment the count
done
In this example, the loop prints the value of count
and increments it by 1 on each iteration. The loop stops when count
becomes greater than 5.
Output:
Count: 1
Count: 2
Count: 3
Count: 4
Count: 5
until
Loop
The until
loop is similar to the while
loop, but it continues executing until a specified condition becomes true. This means that it keeps looping while the condition is false and stops when the condition is true.
Syntax:
until [ condition ]; do
# Commands to execute until condition is true
done
Example:
count=1
until [ $count -gt 5 ]; do
echo "Count: $count"
count=$((count + 1)) # Increment the count
done
In this example, the loop prints the value of count
and increments it by 1 on each iteration. The loop stops when count
becomes greater than 5.
Output:
Count: 1
Count: 2
Count: 3
Count: 4
Count: 5
Conclusion
Understanding control structures is vital for effective shell scripting. By using conditional statements, loops, and case statements, you can create scripts that are dynamic and adaptable to various scenarios.
Practice implementing these control structures in your scripts to enhance your scripting skills and automate tasks efficiently!
Subscribe to my newsletter
Read articles from Dinesh Kumar K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Dinesh Kumar K
Dinesh Kumar K
Hi there! I'm Dinesh, a passionate Cloud and DevOps enthusiast. I love to dive into the latest new technologies and sharing my journey through blog.