Day 11: Diving Deeper into CSS—A Key Piece of My React Journey
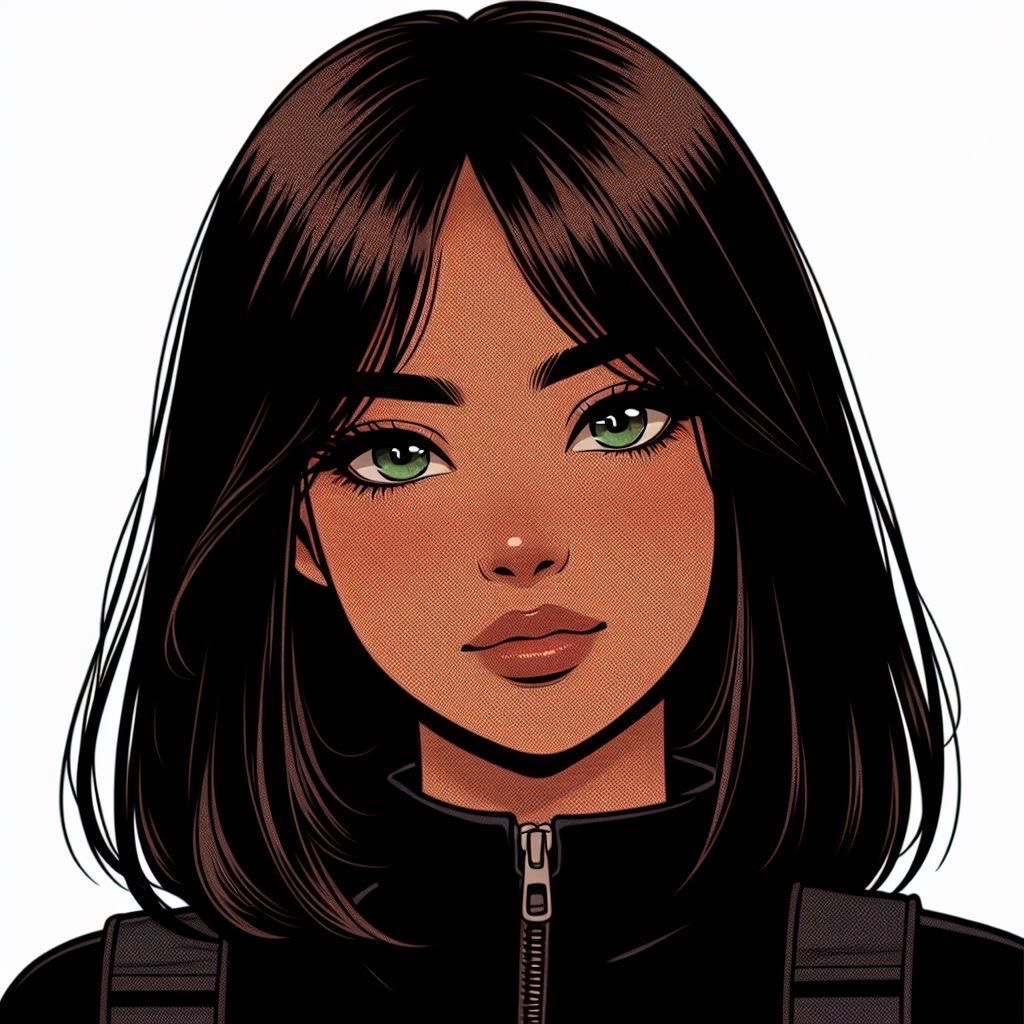
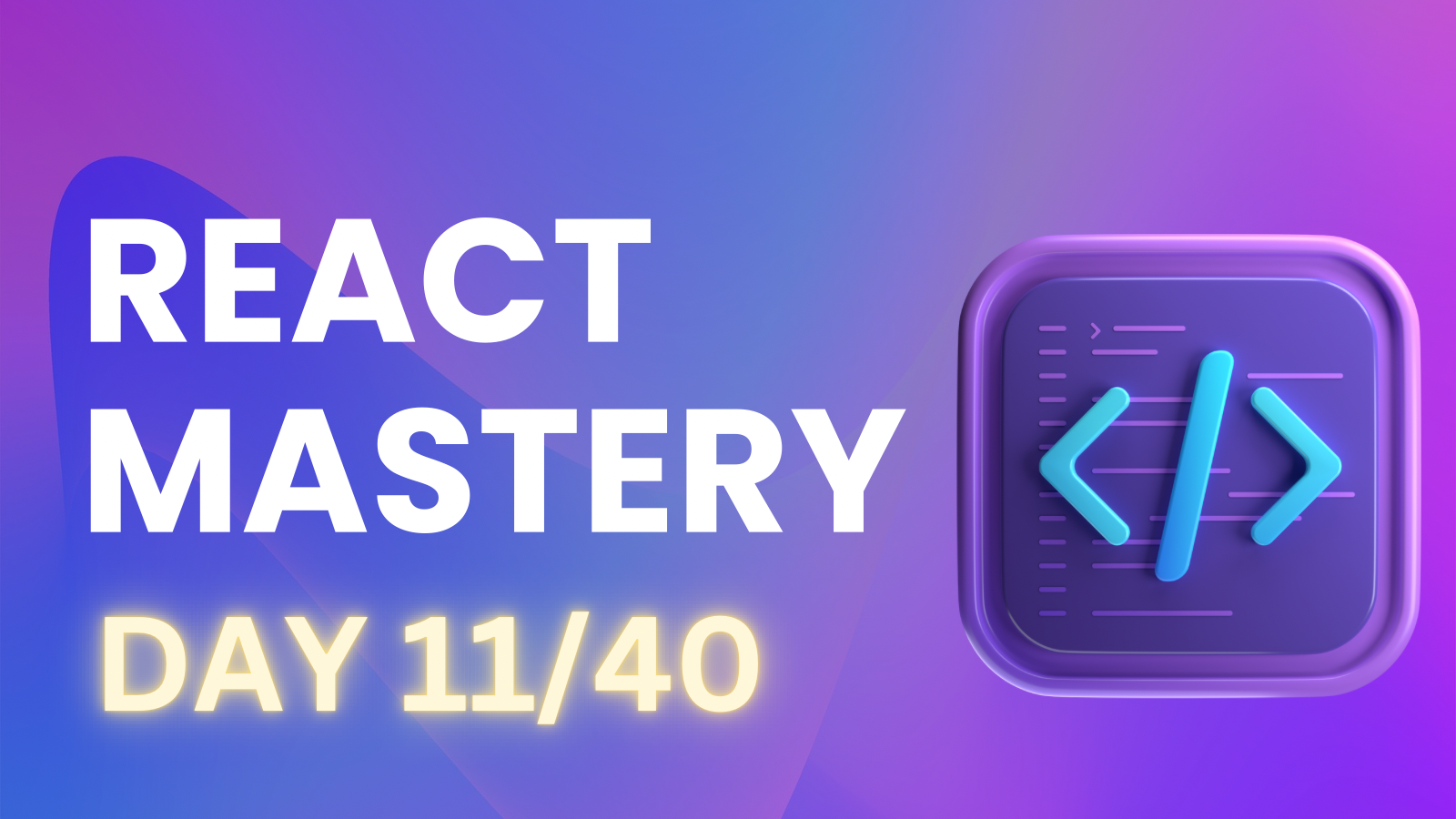
As I continue my 40-day React journey, day 11 has been slightly different. While building my project, I realized that I’ve been neglecting an important aspect of front-end development: CSS.
While I did add some styling I wasn’t doing them on my own, I was copying the styling part from other sources and focusing on the React functionality only. The result? I felt like I was moving further by missing some pieces of the puzzle. And this was beginning to hold me back, as I realized that good design and clean styling play just as critical a role as the functionality of an app.
Revisiting CSS Fundamentals
So, today, I decided to go back to the drawing board and really revisit CSS. I followed a few tutorials to refresh my memory on how to set up and structure a website using Tailwind. For me, this wasn’t just about learning how to style things, but more about understanding how to organize my stylesheets and structure the layout of my application in a way that makes sense.
Here are a few things that stood out to me during this process:
File Management: I learned how to organize my CSS into different files for easier management. Instead of dumping everything into one giant stylesheet, it made sense to separate styles based on the components or sections of the page. This practice will make it easier to scale my projects later on.
Flexbox & Grid Layouts: While I had a basic understanding of these concepts, today I really drilled into them. I realized how powerful CSS Grid and Flexbox can be when building complex layouts. They’re intuitive once you get the hang of them, and using them will save me hours of frustration with layout issues.
Responsiveness: Making sure my designs were responsive on different screen sizes became a priority. It was something I had known about but hadn’t fully implemented in my projects before. Understanding media queries and how to adjust styles based on device width helped me see the importance of building mobile-friendly websites from the start.
What I Learned: The Misconception Between Inline CSS and Tailwind
Today, I came across a common misconception that many developers seem to have, especially beginners. I realized that there’s often confusion between inline CSS and utility-first CSS frameworks like Tailwind CSS—and it’s something I hadn’t fully considered before.
With inline CSS, styles are applied directly within the style
attribute of an element. This means you’re writing custom styles for each individual element right there in the markup, which can lead to code duplication and difficulties with maintainability as projects grow.
Tailwind, on the other hand, uses utility classes, which are predefined styles. You apply these classes to your elements by referencing simple class names like bg-blue-500
for background color or text-xl
for text size. Instead of writing custom styles every time, Tailwind lets you quickly apply reusable styles that are defined in a single, centralized CSS file. The styles are already written and maintained for you, so all you need to do is use the class names, making your code cleaner and much more maintainable.
Understanding Tailwind CSS: How It Works and What Class Names Actually Are
The key takeaway for me was understanding that these class names in Tailwind are not custom styles written for individual elements; rather, they are pre-written, reusable styles. So, when I use a class like bg-blue-500
, Tailwind is simply pulling the appropriate style from its massive, precompiled CSS file and applying it to the element. It’s not about writing styles from scratch every time; it’s about leveraging the power of predefined styles.
What’s powerful about Tailwind is how it separates logic from style. Instead of manually writing CSS for each element or component, I can simply use Tailwind’s utility classes to quickly and consistently style elements across my project. This approach makes the development process faster and more efficient, without the need to worry about style conflicts or redundant code.
In short, class names in Tailwind are really just references to pre-existing styles in the CSS file, allowing us to apply styling in a more modular, scalable way I now see that Tailwind is not about reinventing the wheel with inline styles, but rather about efficiency and scalability—where we apply utility classes to elements, and the framework takes care of the underlying CSS.
Customizing Tailwind CSS for Your Own Needs
Another valuable insight I gained today was how customizable Tailwind CSS really is. While Tailwind comes with a set of predefined utility classes for things like colors, spacing, and text sizes, it’s not limited to just what it offers. I learned that you can easily extend or customize Tailwind to fit your own project needs, allowing you to create custom class names, colors, sizes, and more.
Customizing Class Names and Values
One of the coolest features of Tailwind is its configuration file, usually named tailwind.config.js
, where you can define your own custom utility classes. This file acts as the central hub for customizing the default settings Tailwind provides. For example, if the default color palette doesn't quite match your design system, you can add your own custom colors to the theme
object. Here's how you can do it:
module.exports = {
theme: {
extend: {
colors: {
'custom-blue': '#1E40AF',
},
},
},
}
With this setup, custom-blue
is now a valid class that you can use just like any other Tailwind class (e.g., bg-custom-blue
for background color). This makes it really easy to integrate Tailwind with your existing design system, without having to manually write custom CSS.
Square Bracket Notation for Custom Values
Tailwind also allows you to create custom sizes, which is super helpful when the default sizes don’t quite match your project. You can define custom values like font sizes, margins, padding, or widths. If you want to set a custom pixel size (like 27px
), you can use the square bracket notation:
<div class="text-[27px]">
Custom text size of 27px
</div>
Using @apply
to Apply Custom CSS
This is a Tailwind feature that lets you apply utility classes within your own custom CSS. This means you can create reusable custom styles that still take advantage of Tailwind’s utility-first approach.
For example, if you want to create a custom button with a specific set of styles, you can use @apply
to apply multiple utility classes within a custom CSS class:
cssCopy code/* In your CSS file */
.btn-custom {
@apply bg-blue-500 text-white py-2 px-4 rounded-lg;
}
Now, instead of repeating the utility classes every time, you can just use the .btn-custom
class in your HTML or React component:
htmlCopy code<button class="btn-custom">
Custom Button
</button>
This is really powerful because it allows you to still leverage Tailwind’s utility system, but now you can organize and reuse those styles more easily in your components without cluttering your markup with too many class names.
The Bottom Line
In summary, I realized that Tailwind is not just a set of static utility classes—it’s highly customizable. You can define your own colors, sizes, and other styles, and even use square bracket notation for quick adjustments without having to modify the configuration file every time. This level of flexibility makes Tailwind an incredibly versatile tool for front-end development, and it can be tailored perfectly to meet the specific needs of any project.
That’s it for Day 11! Thanks for reading and following along on this journey! I’m excited to keep building on this foundation and see where day 12 takes me.
Subscribe to my newsletter
Read articles from Aaks directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
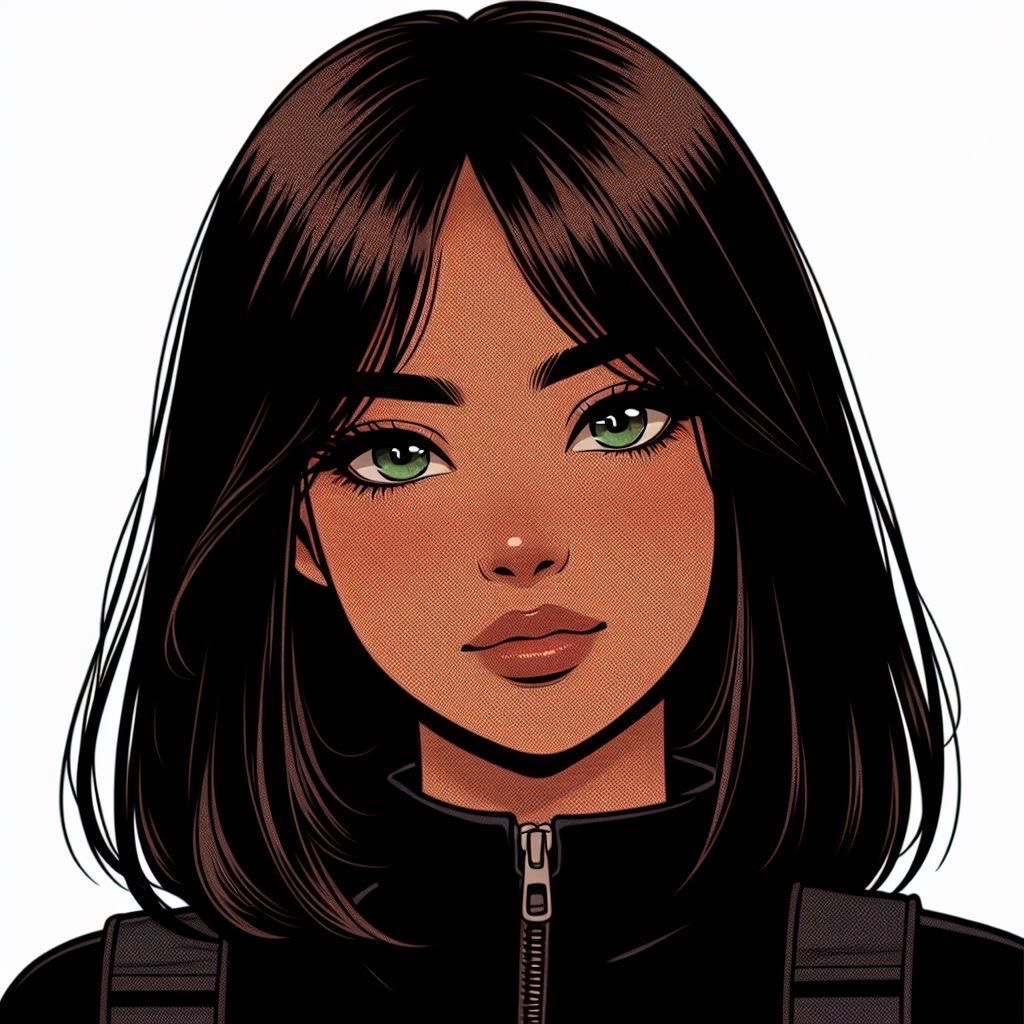