How does Math.random() work in JS?
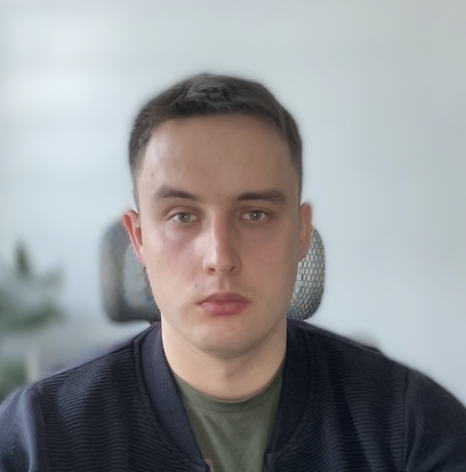
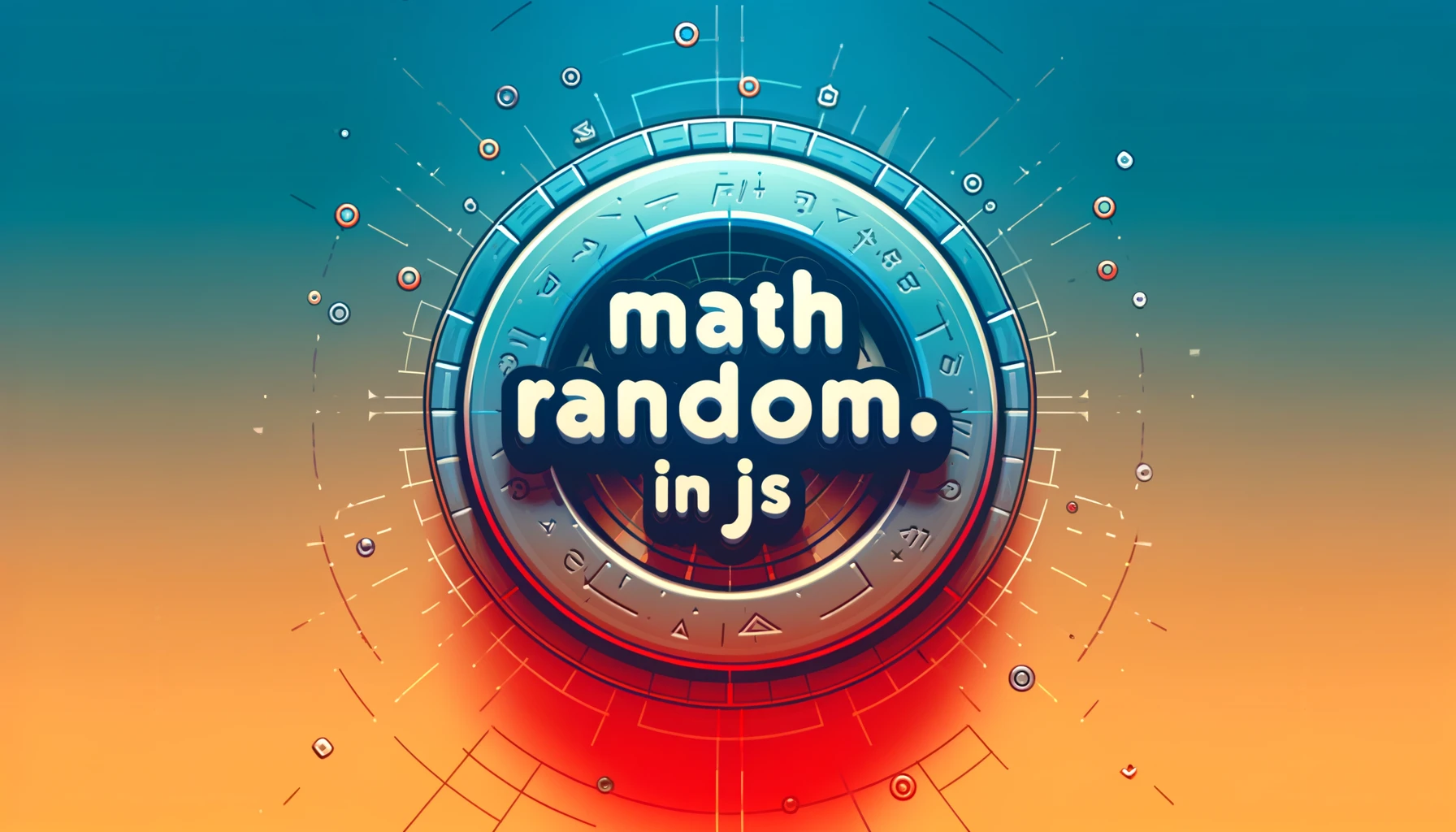
While researching random number generation using quantum programming, I wondered how Math.random() works in JS.
Here are a few points to highlight:
Math.random() is part of the browser API, not the language itself.
In all browsers after 2015, Math.random() uses the pseudorandom number generation algorithm xorshift128+.
xorshift128+ uses a seed phrase to set the initial values of state0 and state1. You can see the algorithm code below:
uint32_t state0 = 1;
uint32_t state1 = 2;
uint32_t mwc1616() {
state0 = 18030 * (state0 & 0xFFFF) + (state0 >> 16);
state1 = 30903 * (state1 & 0xFFFF) + (state1 >> 16);
return state0 << 16 + (state1 & 0xFFFF);
}
- For Node.js, you can specify the seed phrase at startup using the parameter —random_seed. If you start Node with this parameter and generate a number twice, the result will be the same. The code for the calls is below:
$ node --random_seed=42
Welcome to Node.js v16.0.0-pre.
Type ".help" for more information.
> Math.random()
0.7939112874678715
> for (let i = 0; i < 1000; i++) Math.random();
0.6681221903420669
> Math.random()
0.009229884165582902
>
$ node --random_seed=42
Welcome to Node.js v16.0.0-pre.
Type ".help" for more information.
> Math.random()
0.7939112874678715
> for (let i = 0; i < 1000; i++) Math.random();
0.6681221903420669
> Math.random()
0.009229884165582902
Some links:
Article: https://apechkurov.medium.com/v8-deep-dives-random-thoughts-on-math-random-fb155075e9e5 V8 blog post: https://v8.dev/blog/math-random
If you're interested in reading about random number generation through quantum programming, please let me know in the comments.
Subscribe to my newsletter
Read articles from Dmytro Sapozhnyk directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
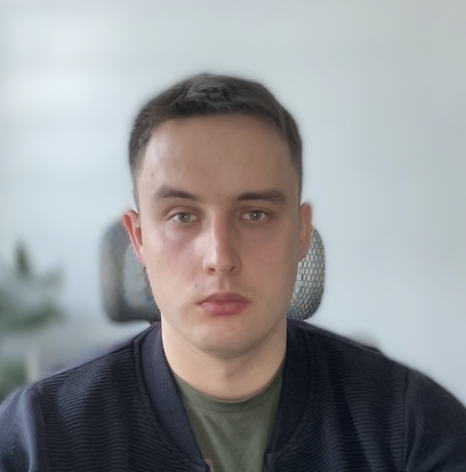
Dmytro Sapozhnyk
Dmytro Sapozhnyk
I have known in different languages (Python, C/C++, basic knows in Java, Solidity), but work with JavaScript. My stack is React.js, Node.js etc...