Understanding WSGI, ASGI, HTTP and Web Servers: Clarifying the Confusion

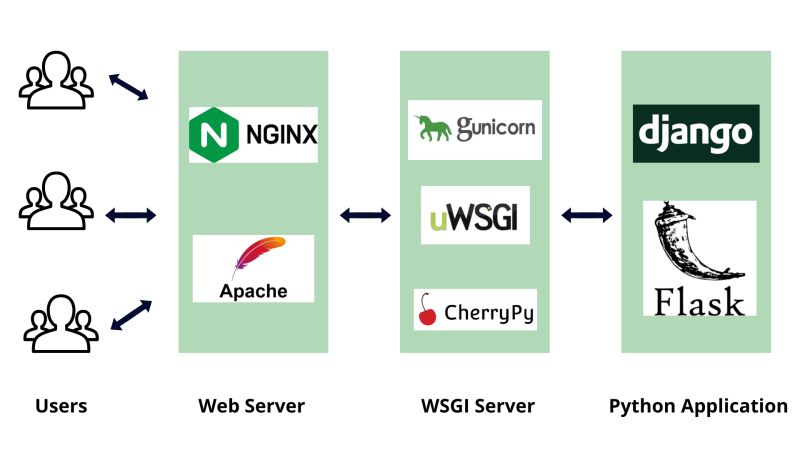
In the world of web development, especially when working with Python, developers often encounter various concepts like WSGI (Web Server Gateway Interface), ASGI (Asynchronous Server Gateway Interface), and web servers like Nginx. This article aims to clarify these concepts, their roles, and how they interact with each other and the operating system (OS).
1. The Role of Programming Languages in Web Development
Why Python Doesn't Have a Built-in Server
Python is a versatile programming language that allows for various applications, including web development. However, it does not come with a built-in web server module capable of handling production-level web traffic robustly. Instead, Python provides several lower-level modules in its standard library for networking and HTTP handling.
While these modules enable developers to create simple servers for testing or learning purposes, they lack the performance, scalability, and robustness required for real-world applications. Hence, Python developers often rely on external servers and frameworks.
Comparison with Node.js
Unlike Python, Node.js is built on the V8 JavaScript engine and comes with a powerful http
module that allows developers to create HTTP servers easily. This design choice reflects Node.js's focus on asynchronous I/O and performance for web applications.
2. How Servers are Created in Programming Languages
Building a Server
example of some standard server running on specfic port : -
In programming languages like Python and Node.js, developers create servers using specific modules that abstract the underlying network functionalities. These modules interact with the OS to manage network communications.
Node.js Example: In Node.js, the
http
module allows developers to create an HTTP server with minimal code. When a server is instantiated, the module communicates with the OS to open a specified port (e.g., port 80 for HTTP). Here’s a simple example of creating an HTTP server in Node.js:const http = require('http'); const server = http.createServer((req, res) => { res.statusCode = 200; // HTTP status code res.setHeader('Content-Type', 'text/plain'); res.end('Hello, World!\n'); // Response body }); server.listen(80, () => { console.log('Server is running on port 80'); });
Python Example: In Python, while there is no built-in robust HTTP server, you can create a simple server using the
http.server
module for testing purposes. It also communicates with the OS to open a specified port:from http.server import BaseHTTPRequestHandler, HTTPServer class SimpleHTTPRequestHandler(BaseHTTPRequestHandler): def do_GET(self): self.send_response(200) # HTTP status code self.send_header('Content-Type', 'text/plain') self.end_headers() self.wfile.write(b'Hello, World!\n') # Response body server_address = ('', 8000) # Open port 8000 httpd = HTTPServer(server_address, SimpleHTTPRequestHandler) print('Server running on port 8000...') httpd.serve_forever()
Interaction with the Operating System
Opening Ports: When a server is created using these modules, the code instructs the OS to open the specified port. The OS allocates the port and prepares to listen for incoming requests.
Receiving Requests: When a request comes in, the OS forwards it to the server program listening on the specified port. This is achieved through system calls and socket programming, where the OS handles the low-level details of network communication.
Handling Requests: The server processes the request according to the logic defined in the application code (in either Node.js or Python). It may involve routing the request to different functions or methods based on the request path, handling data, querying databases, and preparing a response.
Sending Responses: After processing the request, the server sends the appropriate response back to the OS, which then communicates it to the client that made the initial request.
3. What is WSGI?
The WSGI Specification
WSGI is a specification that defines a standard interface between web servers and Python web applications or frameworks. It allows Python applications to communicate with web servers in a consistent manner.
When a WSGI application is created, it typically consists of a callable (a function or class) that takes two arguments:
environ
: A dictionary containing all the request data.start_response
: A function to initiate the HTTP response.
Here's a simple example of a WSGI application:
def application(environ, start_response):
response_body = b'Hello, World!' # Sample response body
status = '200 OK' # HTTP status code
headers = [('Content-Type', 'text/plain')]
start_response(status, headers) # Initiate response
return [response_body] # Return the response body
4. The Role of ASGI
Asynchronous Support
ASGI, or Asynchronous Server Gateway Interface, is an evolution of WSGI that supports asynchronous programming. While WSGI is synchronous, meaning it can only handle one request at a time, ASGI allows for handling multiple requests simultaneously, making it more suitable for applications that require real-time features like WebSockets or long-polling.
ASGI as an Interface
Like WSGI, ASGI serves as an interface between your Python applications and web servers. It also can listen on a specified port (like port 8000) to receive requests from servers like Nginx. By doing so, ASGI enables asynchronous handling of requests, allowing developers to write more scalable applications that can handle numerous connections concurrently.
5. How Requests Flow Through the System
Web Server (Nginx)
example of some popular webserver : -
Listening on Port 80: Nginx, a popular web server, listens for incoming HTTP requests on port 80, which is the default port for HTTP.
Receiving Requests: When a request comes in, Nginx processes it based on its configuration.
Forwarding to WSGI/ASGI Server: Nginx forwards the request to a WSGI or ASGI server running on a different port, often port 8000. This is achieved through OS-level networking.
WSGI/ASGI Server
One key point to understand is that both WSGI and ASGI servers are built similarly to traditional HTTP servers. In every programming language, there is typically a module that allows the creation of a server. This module doesn’t do much on its own—it simply communicates with the operating system, instructing it to open a specific port. The module then tells the OS, "Any request that comes through this port, hand it off to our HTTP server."
We have over 65,000 ports available on a machine, with some reserved for standard services. For example, HTTP operates on port 80 for unsecured traffic and 443 for HTTPS (secured). The HTTP module’s job is to communicate with the OS, asking it to open port 80 (or 443 for HTTPS) for incoming HTTP requests.
With WSGI and ASGI servers, the process is similar. However, we aren't creating a full web server ourselves. Instead, we’re building an interface server where an existing web server (like NGINX or Apache) forwards the requests to our Python application.
For example:
- A WSGI server, such as Gunicorn or uWSGI, typically runs on a port like 8000 or 8080. It receives HTTP requests from the web server (like NGINX) and passes them to the Python application.
Listening on Port 8000: The WSGI or ASGI server listens for requests on port 8000.
Handling Requests: Upon receiving a request from Nginx, the WSGI or ASGI server invokes the corresponding application, passing along the
environ
andstart_response
parameters (for WSGI) or handling asynchronous calls (for ASGI).Processing the Request: The WSGI or ASGI application processes the request, performs any necessary logic (e.g., querying a database), and prepares a response.
Sending Response Back: The response generated by the WSGI or ASGI application is sent back to the WSGI/ASGI server, which then forwards it to Nginx.
Final Response to Client
Nginx sends the response back to the original client (like a web browser or another application).
6. The Role of the Operating System
OS Networking Capabilities
The operating system plays a crucial role in managing network communications. It handles:
Opening Ports: When a server starts, the OS opens the specified ports for communication.
Managing Socket Connections: The OS manages the network sockets that handle incoming and outgoing requests.
Routing Requests: It ensures that incoming requests to a specific port (like 8000 for the WSGI or ASGI server) are delivered to the appropriate application.
7. Data Parsing and Format Handling
String Data Transfer
When data flows over the network, it is typically in the form of strings (e.g., HTTP requests). This data can be interpreted by any programming language that understands the relevant protocols. Common formats for data exchange include:
JSON: A lightweight data interchange format that is easy to read and write for humans and machines.
XML: A markup language that defines rules for encoding documents in a format that is both human-readable and machine-readable.
How Different Languages Communicate
Data Serialization: Different programming languages (e.g., Java, Python) can communicate effectively by serializing data into universally accepted formats like JSON or XML.
Flow of Data: For instance, if a Java application needs to send data to a Python WSGI application:
Java Builds JSON: The Java application constructs a JSON object containing the necessary data.
Sending Data: The JSON data is sent to the OS, which routes it to the appropriate server (e.g., Nginx).
Nginx to WSGI: Nginx forwards the request to the WSGI server.
WSGI Application: The WSGI application receives the request and can parse the JSON data into a Python dictionary for processing.
Environment Variables (environ)
The environ
dictionary passed to the WSGI application contains information about the request, including:
Request method (GET, POST, etc.)
Request path
Query parameters
HTTP headers
Any other relevant request data
Conclusion
In conclusion, understanding the roles of WSGI, ASGI, and web servers like Nginx is crucial for Python web development. These components work together to handle web requests efficiently, with WSGI providing a synchronous interface and ASGI offering asynchronous capabilities for modern applications. By leveraging these technologies, developers can build scalable and robust web applications. Additionally, the operating system plays a vital role in managing network communications, ensuring that requests are routed correctly and efficiently. As web development continues to evolve, mastering these concepts will empower developers to create more dynamic and responsive applications.
Subscribe to my newsletter
Read articles from ritiksharmaaa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ritiksharmaaa
ritiksharmaaa
Hy this is me Ritik sharma . i am software developer