Google’s Firebase with React.js: A Comprehensive Guide
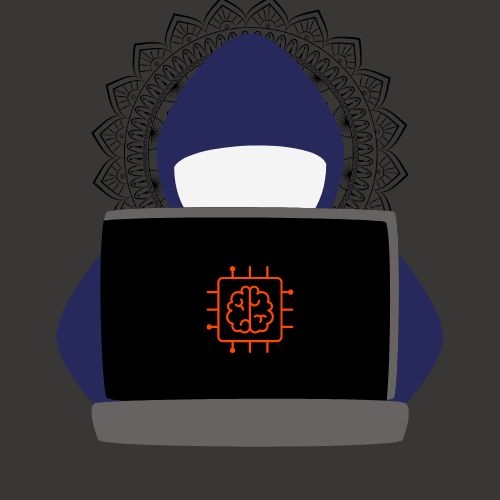
In the ever-evolving landscape of web development, the integration of powerful tools and frameworks can significantly enhance the efficiency and effectiveness of your projects. One such powerful duo is Google’s Firebase and React.js. In this blog, we’ll explore the uses, benefits, and potential drawbacks of using Firebase with React, as well as provide some insights on how to get started.
What is Firebase?
Firebase is a platform developed by Google that offers a suite of tools to help developers build and manage web and mobile applications. It provides a robust backend service that simplifies the development process, allowing developers to focus on creating engaging user experiences.
What is React.js?
React.js is a popular JavaScript library for building user interfaces, particularly single-page applications where a dynamic user experience is crucial. Developed by Facebook, React allows developers to create reusable UI components, making it easier to manage and scale applications.
Uses of Firebase with React.js
Integrating Firebase with React can unlock a range of possibilities for developers. Here are some common use cases:
Real-time Database: Firebase’s Firestore or Realtime Database allows for real-time data synchronization. This is particularly useful for applications like chat apps, collaborative tools, or any app requiring real-time data updates.
User Authentication: Firebase Authentication provides an easy way to authenticate users using various methods, such as email/password, Google, Facebook, and more. This simplifies the process of implementing secure authentication in React applications.
Cloud Functions: Firebase Cloud Functions allow developers to run backend code in response to events triggered by Firebase features. This can be beneficial for handling tasks like sending notifications or processing data in the background.
Hosting: Firebase offers seamless hosting for static and dynamic web applications, making it easier to deploy your React app without worrying about the underlying infrastructure.
Analytics: Firebase Analytics provides insights into user behavior, helping developers understand how users interact with their applications.
Benefits of Using Firebase with React.js
Ease of Use: Firebase offers a straightforward setup process and a user-friendly interface. Integrating Firebase into a React app can often be done with just a few lines of code.
Scalability: Firebase is built on Google’s infrastructure, providing a scalable solution that can grow with your application. Whether you’re a small startup or a large enterprise, Firebase can handle increased traffic without a hitch.
Cross-Platform Support: With Firebase, you can develop applications for both web and mobile platforms using the same backend, saving time and effort in development.
Community and Support: Firebase has a large community and extensive documentation, making it easy to find help and resources as you develop your application.
Integrated Services: The wide range of services offered by Firebase, such as cloud storage, hosting, and messaging, means you can build and manage your entire application from a single platform.
Demerits of Using Firebase with React.js
Vendor Lock-In: Relying heavily on Firebase can lead to vendor lock-in. Migrating to another backend solution can be challenging due to the proprietary nature of Firebase services.
Pricing: While Firebase offers a free tier, costs can escalate quickly as your app scales and you begin to use additional features. It’s essential to monitor usage to avoid unexpected charges.
Limited Querying Capabilities: Firebase’s NoSQL structure can be limiting for complex querying. Developers may find it challenging to perform certain types of queries compared to traditional SQL databases.
Performance Issues: In some cases, developers have reported performance issues with Firebase’s Realtime Database, particularly with large datasets. Careful planning and implementation are required to optimize performance.
Getting Started
To get started with Firebase and React, follow these simple steps:
Create a Firebase Project: Go to the Firebase Console and create a new project.
Install Firebase: In your React project, install Firebase via npm:
npm install firebase
Initialize Firebase: Add your Firebase configuration to your React app:
import firebase from "firebase/app"; import "firebase/auth"; // For authentication import "firebase/firestore"; // For Firestore // Add other Firebase services as needed const firebaseConfig = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_PROJECT_ID.firebaseapp.com", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_PROJECT_ID.appspot.com", messagingSenderId: "YOUR_MESSAGING_SENDER_ID", appId: "YOUR_APP_ID", }; firebase.initializeApp(firebaseConfig);
Implement Features: Start building your application by implementing Firebase features, such as authentication, database access, and cloud functions.
Conclusion
Combining Firebase with React.js provides a powerful toolkit for developers looking to create dynamic, real-time applications. While there are some potential drawbacks, the benefits often outweigh them, especially for smaller projects or those that require rapid development. With a robust set of tools at your disposal, you can focus on building engaging user experiences without getting bogged down by backend complexities.
Whether you’re a seasoned developer or just starting, leveraging Firebase with React can significantly enhance your development process. So why not give it a try in your next project?
Subscribe to my newsletter
Read articles from Aradhya Srivastava directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
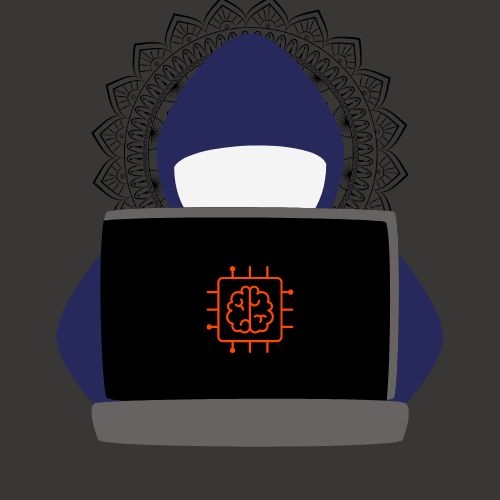