Learn EJS: A Beginner's Guide to Embedded JavaScript Templates
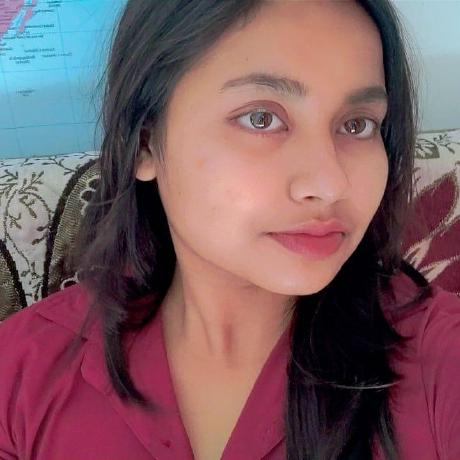
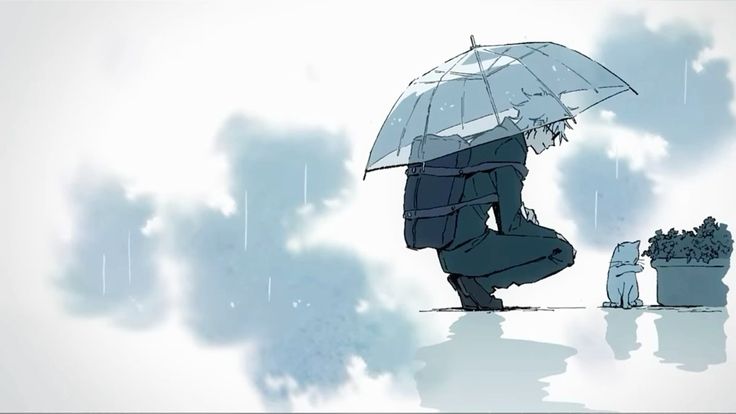
First Download the ejs, run this following command in console
npm i ejs
Let’s Start
1st start a Node js application
you should have express install in that folder
write the basic express code in the index.js file
import express from "express";
const app = express();
app.listen(5000, () => {
console.log("server is on");
})
make a ‘views’ folder and make a ‘index.ejs’ file in ‘views’ folder
The ‘index.ejs’ file is a basic HTML file
You can copy the ‘index.ejs’ file if you want (not necessary to be the same)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>EJS</title>
</head>
<body>
<h2>Embedded JavaScript Templates</h2>
</body>
</html>
Setting up a view engine
Add this to index.js file
app.set("view engine", "ejs");
app.get("/", (req, res) => {
res.render("index");
})
Just for checking, Your Package.json kind of be like this
{
"name": "ejs",
"version": "1.0.0",
"description": "",
"main": "index.js",
"type": "module",
"scripts": {
"start": "node index.js",
"dev": "nodemon index.js"
},
"author": "",
"license": "ISC",
"dependencies": {
"ejs": "^3.1.10",
"express": "^4.21.1",
"nodemon": "^3.1.7"
}
}
Now run this project, you can run this command
npm run dev
or
npm start
by using .render you can send a value through JavaScript
in index.js
app.get("/", (req, res) => {
res.render("index", {name: "Suto"}); //Here you can pass the value of a variable, you can change the name Here
})
Now to access the name data
in index.ejs
<h2>Embedded JavaScript Templates</h2>
<h2>Hello I am <%=locals.name%></h2>
Now for making a Static file, so that we can call the file in ‘inde.js’, we 1st create a folder named ‘public’ and the we add the files which we want to use in index.js,
for example, create a css file in ‘public folder’
Now if we connect the css file with ‘index.ejs’ file
<link rel="stylesheet" href="style.css">
Then although the root folder is different, still it will work.
ame you can do with any other file, like ‘script.js’ file
(Everything you put in ‘public’ folder is publically available.
The whole frontend Code will be in this ‘public’ folder)
Making a contact form using Nodejs & ejs
In this project based learning, we create a contact form and when the user click on the submit button, the data will com in console(later we learn how to store the data in databse)
First create a basic html form,
index.ejs
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>EJS</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="contact-form">
<h2>Contact Us</h2>
<form>
<div class="form-control">
<label for="name">Name</label>
<input type="text" id="name" name="name" required>
</div>
<div class="form-control">
<label for="email">Email</label>
<input type="email" id="email" name="email" required>
</div>
<div class="form-control">
<button type="submit">Submit</button>
</div>
</form>
</div>
</body>
<script src="script.js"></script>
</html>
style.css
body {
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
background-color: #9cbff3;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.contact-form {
background: #ffffff;
padding: 30px;
border-radius: 15px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
max-width: 350px;
width: 100%;
text-align: center;
}
.contact-form h2 {
margin-bottom: 25px;
color: #333;
font-size: 1.8rem;
}
.form-control {
margin-bottom: 20px;
text-align: left;
}
.form-control label {
display: block;
font-size: 0.9rem;
color: #555;
margin-bottom: 5px;
}
.form-control input {
width: 93%;
padding: 12px;
border: 1px solid #ccc;
border-radius: 10px;
font-size: 1rem;
transition: border-color 0.3s ease;
}
.form-control input:focus {
border-color: #0066cc;
outline: none;
}
.form-control button {
width: 100%;
padding: 12px;
background-color: #0066cc;
color: white;
border: none;
border-radius: 10px;
font-size: 1rem;
cursor: pointer;
transition: background-color 0.3s ease;
}
.form-control button:hover {
background-color: #005bb5;
}
Now, by default <form>’s method is get, change it into ‘post method and add a ‘action’, (action means, after submitting the form, where the user will be redirected, or in which end-point it will hit)
<form method="post" action="/">
Now we have to make an API for the post-method
in index.js
//using middlewares
app.use(express.urlencoded({extended: true}));
app.post("/", (req, res) => {
console.log(req.body);
})
Now we have the form data (email and name) in the console
Now we also can make a empty array and store the data from the form, given by the user, in that array
in index.js
const user = [];
app.post("/", (req, res) => {
console.log(req.body);
user.push({userName: req.body.name, email: req.body.email})
})
app.get("/users", (req, res) => {
res.json({
users,
})
})
Now in ‘/users’, we can see the inputs submitted by the users
Now rendering another file named ‘ano_file’
make a file in ‘views’ folder named ‘ano_file.ejs’ & write a basic html code
add this in app.post in index.js
res.render("ano_file");
Also we can redirect to that file by
res.redirect("ano_file");
Project
creating a addmission form and get data from users and store it in an array & access it by a api
index.js
import express from "express";
const app = express();
const users = [];
// Middleware to serve static files (like CSS)
app.use(express.static("public"));
// Middleware to parse form data
app.use(express.urlencoded({ extended: true }));
app.get("/", (req, res) => {
res.render("index.ejs");
});
app.post("/", (req, res) => {
users.push({
full_name: req.body.name,
email: req.body.email,
contact_number: req.body.contact,
date_of_birth: req.body.dob,
gender: req.body.gender,
address: req.body.address,
course: req.body.course,
});
res.send("Application submitted successfully!");
});
app.get("/students", (req, res)=>{
res.json({
users,
});
});
app.listen(3000, () => {
console.log("server is running");
});
views/index.ejs
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Addmission form</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="form-container">
<h2>Admission Form</h2>
<form method="POST">
<label for="name">Full Name</label>
<input type="text" id="name" name="name" required>
<label for="email">Email</label>
<input type="email" id="email" name="email" required>
<label for="contact">Contact Number</label>
<input type="tel" id="contact" name="contact" required>
<label for="dob">Date of Birth</label>
<input type="date" id="dob" name="dob" required>
<label for="gender">Gender</label>
<select id="gender" name="gender" required>
<option value="" disabled selected>Select your gender</option>
<option value="male">Male</option>
<option value="female">Female</option>
<option value="other">Other</option>
</select>
<label for="address">Address</label>
<textarea id="address" name="address" rows="4" required></textarea>
<label for="course">Select Course</label>
<select id="course" name="course" required>
<option value="" disabled selected>Select a course</option>
<option value="btech">B.Tech</option>
<option value="bsc">B.Sc</option>
<option value="ba">B.A</option>
<option value="bcom">B.Com</option>
</select>
<button type="submit">Submit Application</button>
</form>
</div>
</body>
</html>
public/style.css
body {
font-family: Arial, sans-serif;
background-color: #f2f2f2;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.form-container {
background-color: white;
padding: 20px;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
width: 400px;
}
.form-container h2 {
text-align: center;
margin-bottom: 20px;
}
.form-container label {
display: block;
margin-bottom: 8px;
font-weight: bold;
}
.form-container input,
.form-container select,
.form-container textarea {
width: 100%;
padding: 10px;
margin-bottom: 15px;
border-radius: 4px;
border: 1px solid #ccc;
}
.form-container button {
width: 100%;
padding: 10px;
background-color: #28a745;
color: white;
border: none;
border-radius: 4px;
font-size: 16px;
cursor: pointer;
}
.form-container button:hover {
background-color: #218838;
}
package.json
{
"name": "practice",
"version": "1.0.0",
"description": "",
"main": "index.js",
"type": "module",
"scripts": {
"start": "node index.js",
"dev": "nodemon index.js"
},
"author": "",
"license": "ISC",
"dependencies": {
"ejs": "^3.1.10",
"express": "^4.21.1",
"nodemon": "^3.1.7"
}
}
Subscribe to my newsletter
Read articles from Sutapa Biswas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
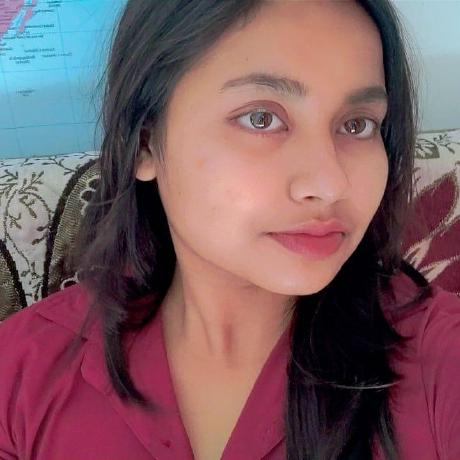