Enhancing iOS App Security with SSL Pinning Through URLSession

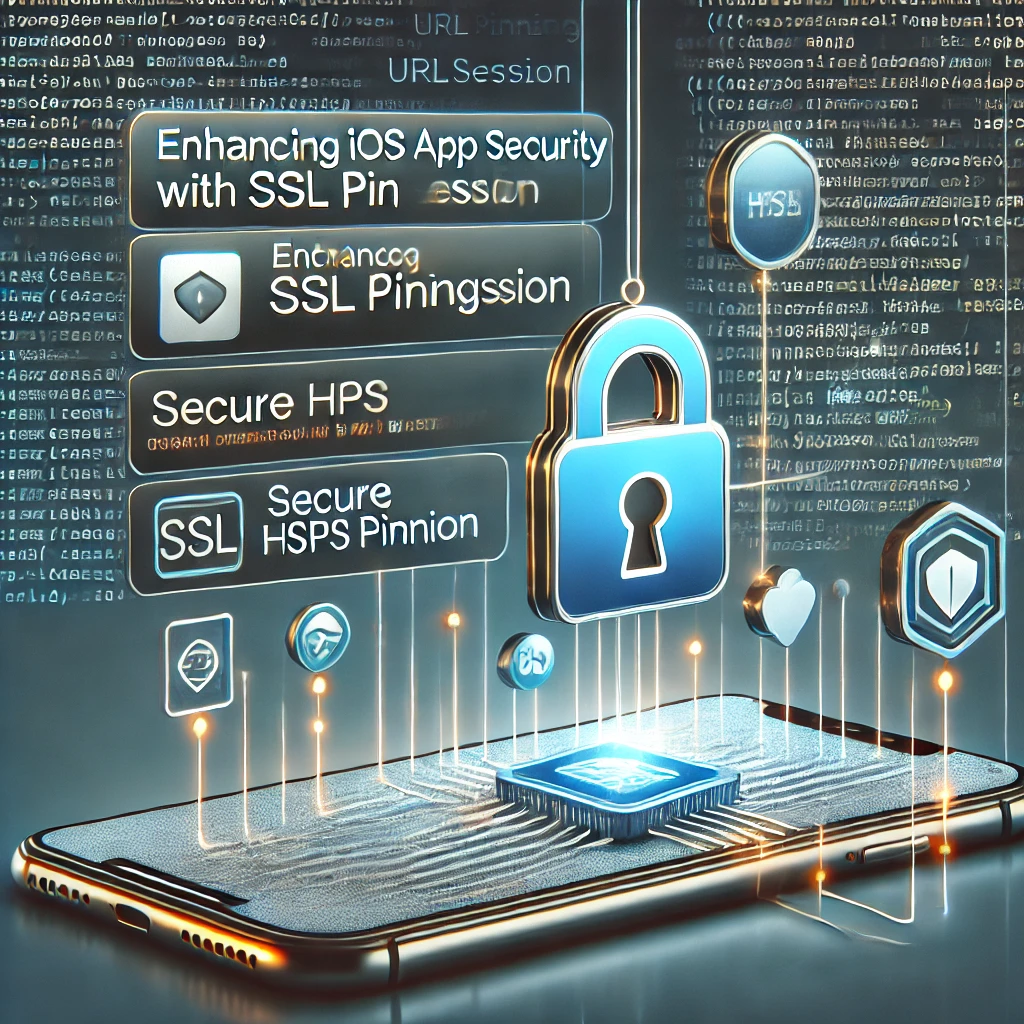
Introduction
In today's digital landscape, securing user data is more crucial than ever. One effective way to protect your iOS applications from man-in-the-middle (MITM) attacks is by implementing SSL pinning. This technique ensures that your app only trusts specific SSL certificates, providing an additional layer of security when communicating with your server.
What is SSL Pinning?
SSL pinning is a security measure that allows your app to validate the server's SSL certificate against a known good certificate. If the certificates do not match, the app will reject the connection, preventing potential data breaches.
Implementing SSL Pinning in iOS
To implement SSL pinning in your iOS app, you can use the URLSession
API. Here’s a step-by-step guide:
Handle Server Trust Challenges: Implement the
URLSession(_:didReceive:completionHandler:)
method in your session delegate. This method is triggered when a server trust challenge occurs.Evaluate the Server's Certificate: Retrieve the server's SSL certificate from the
challenge.protectionSpace.serverTrust
and evaluate it against your local certificate. If the server's certificate matches the local one, you can proceed with the connection.Code Snippet: Here’s a sample implementation in Swift:
func urlSession(_ session: URLSession, didReceive challenge: URLAuthenticationChallenge, completionHandler: @escaping (URLSession.AuthChallengeDisposition, URLCredential?) -> Void) { let serverTrust = challenge.protectionSpace.serverTrust let certificate = SecTrustGetCertificateAtIndex(serverTrust!, 0) // Set SSL policies for domain name check let policies = NSMutableArray() policies.add(SecPolicyCreateSSL(true, challenge.protectionSpace.host)) SecTrustSetPolicies(serverTrust!, policies) // Evaluate server certificate var result: SecTrustResultType = .invalid SecTrustEvaluate(serverTrust!, &result) let isServerTrusted = (result == .unspecified || result == .proceed) // Compare certificates let remoteCertificateData = SecCertificateCopyData(certificate!) let localCertificateData = NSData(contentsOfFile: Bundle.main.path(forResource: "localCert", ofType: "cer")!)! if isServerTrusted && remoteCertificateData.isEqual(to: localCertificateData) { let credential = URLCredential(trust: serverTrust!) completionHandler(.useCredential, credential) } else { completionHandler(.cancelAuthenticationChallenge, nil) } }
Benefits of SSL Pinning
Implementing SSL pinning significantly enhances your app's security by:
Reducing Risk of MITM Attacks: By ensuring the server’s identity, SSL pinning makes it much harder for attackers to intercept communications.
Building User Trust: Users are more likely to trust applications that prioritize their security.
Best Practices
While SSL pinning is effective, it’s important to follow best practices:
Keep Certificates Updated: Regularly update your pinned certificates to accommodate changes in your server's SSL configuration.
Consider Public Key Pinning: Instead of pinning entire certificates, you can pin the public keys, which allows for greater flexibility in certificate management.
Subscribe to my newsletter
Read articles from thevenkat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

thevenkat
thevenkat
I am a Senior iOS Developer, crafting Native iOS applications using Swift. My passion lies in creating innovative solutions that enhance user experience and drive customer satisfaction. I excel in problem-solving, code optimization, and staying updated with the latest trends and technologies in the iOS ecosystem. I thrive in collaborative environments and am always eager to share knowledge and learn from others.