Stack, Java

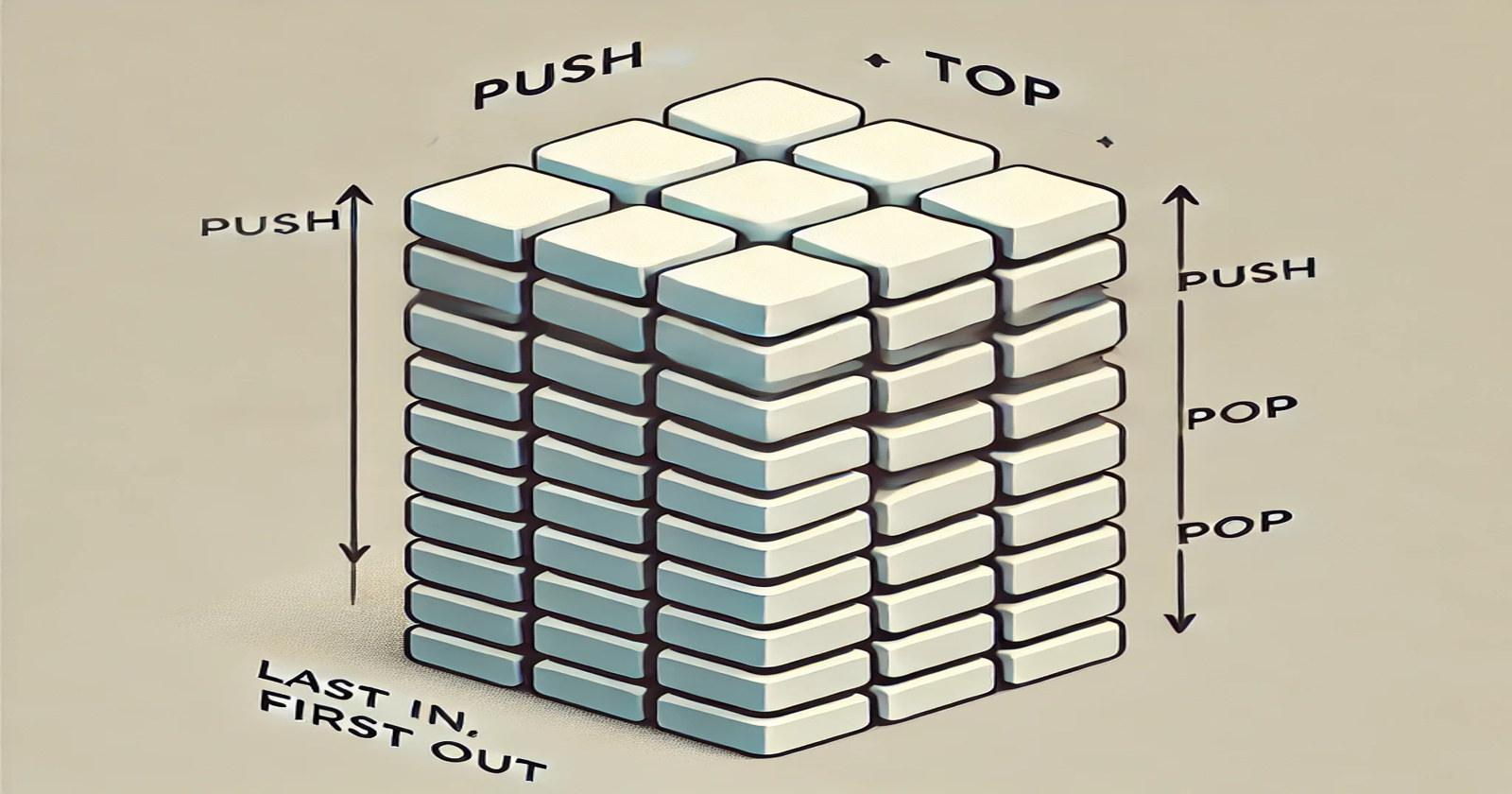
Overview
The stack is a fundamental data structure that operates on the principle of Last In, First Out (LIFO), like Queue but just reverse. This means that the last element added to the stack will be the first one to be removed. Stacks are widely used in various applications, including expression evaluation, backtracking algorithms, and memory management. It is a linear data structure; means we can travel all the elements of it in using single loop.
Characteristics of Stack
LIFO Principle: The last element added is the first to be removed.
Single End Access: Elements can only be added or removed from one end known as the "top" of the stack.
Dynamic Size: Depending on the implementation, stacks can grow or shrink dynamically.
Operations: The primary operations are
push
(to add an element),pop
(to remove an element), andpeek
(to view the top element without removing it).
Usage of Stack in Java
In Java, stacks can be implemented using the built-in Stack
class from the java.util
package, or they can be manually implemented using arrays or linked lists. Some popular usages of stack are - Parsing expressions (infix to postfix conversion), Here’s how to use the built-in stack:
Importing Stack Class
import java.util.Stack;
Creating a Stack
Stack<Integer> myStack = new Stack<>();
Basic Operations
Push Operation: Adds an element to the top of the stack.
myStack.push(5); myStack.push(10);
Pop Operation: Removes and returns the top element of the stack.
int topElement = myStack.pop(); // returns 10
Peek Operation: Returns the top element without removing it.
int peekElement = myStack.peek(); // returns 5
Check if Empty: Determines if the stack is empty.
boolean isEmpty = myStack.isEmpty(); // returns false
Size of Stack: Returns the number of elements in the stack.
int size = myStack.size(); // returns 1
Advantages of Using Stack
Memory Efficiency: Stacks use less memory compared to other data structures like queues when implemented with arrays.
Easy Implementation: The stack operations are straightforward and easy to implement.
Reversibility: Stacks can easily reverse data, making them useful for tasks like string reversal.
Disadvantages of Using Stack
Limited Access: Only allows access to elements at one end (the top), which can lead to inefficiencies if random access is needed.
Fixed Size (in Array Implementation): If implemented with a fixed-size array, it can lead to overflow if not managed properly.
No Built-in Capacity Management: Unlike some other data structures, stacks do not automatically resize unless explicitly coded.
Code Example
Here’s a complete example demonstrating stack operations in Java:
import java.util.Stack;
public class StackExample {
public static void main(String[] args) {
Stack<Integer> stack = new Stack<>();
// Push elements onto the stack
stack.push(1);
stack.push(2);
stack.push(3);
// Displaying current top element
System.out.println("Top element is: " + stack.peek());
// Pop an element from the stack
System.out.println("Popped element: " + stack.pop());
// Check size of stack
System.out.println("Current size of stack: " + stack.size());
// Check if stack is empty
System.out.println("Is stack empty? " + stack.isEmpty());
}
}
Conclusion
The stack data structure is essential for various computational tasks due to its LIFO nature. Whether using Java's built-in Stack
class or implementing your own, understanding its operations and characteristics can significantly enhance your programming skills. Stacks are particularly useful in scenarios requiring backtracking or managing function calls, making them a critical tool in a developer's toolkit.
Subscribe to my newsletter
Read articles from Arpit Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Arpit Singh
Arpit Singh
AI engineer at Proplens AI, a final year student pursuing bachelor's in computer science and engineering.