Web Development with Go and Gin

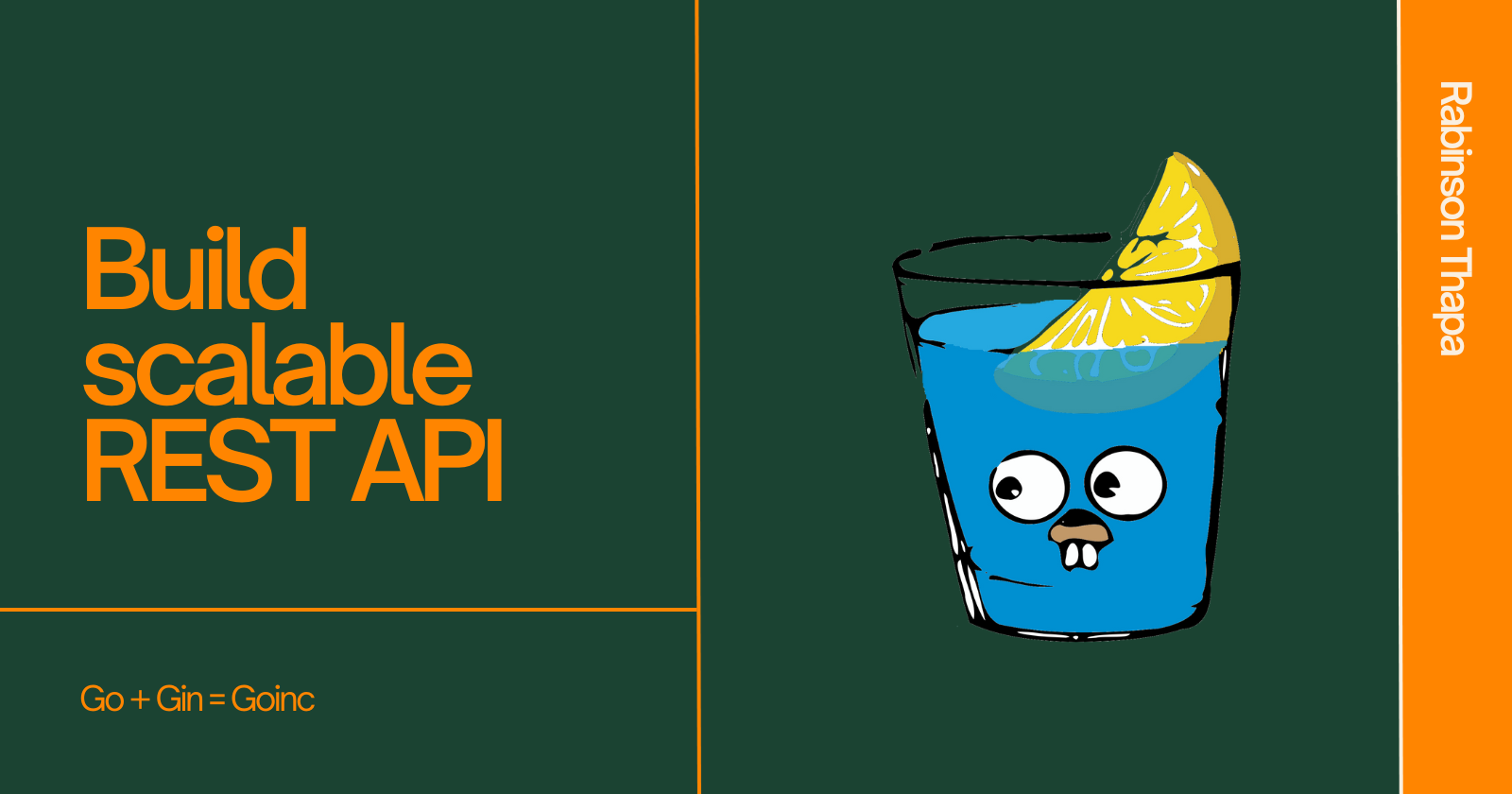
Introduction
In the rapidly evolving world of web development, the choice of programming language and framework can significantly influence the success of your project. Among the many options available, Go (or Golang) is renowned for its efficiency, simplicity, and performance.
One of the standout frameworks within the Go ecosystem is Gin, a lightweight web framework designed to streamline the process of building robust web applications. Combining speed with an easy-to-use interface, Gin has become a preferred choice for developers creating high-performance APIs and microservices.
In this blog post, we'll explore the benefits of using Go for web development and delve into the Gin framework, examining its features, advantages, and practical applications. This guide will equip you with the knowledge needed to leverage Go and Gin effectively.
Introduction to Gin: A Lightweight Web Framework
What is Gin?
Gin is a web framework written in Go that is designed for speed and simplicity. With a focus on performance, Gin offers a small footprint and rapid routing capabilities, making it an excellent choice for developers looking to build scalable web applications and APIs quickly. Its minimalistic design does not compromise functionality, ensuring developers have access to the tools they need to succeed.
Key Features of Gin
Routing: Gin’s router is designed for simplicity and speed. It supports dynamic routing, allowing developers to create clean and maintainable routes with ease. The intuitive syntax makes it straightforward to define routes and their corresponding handlers.
Middleware: Gin supports built-in and custom middleware, enabling developers to easily add functionality such as logging, authentication, and error handling to their applications. This modular approach enhances code reusability and keeps applications organized.
JSON Handling: Gin simplifies the handling of JSON requests and responses with built-in support for JSON parsing and validation. This feature is precious for API development, allowing developers to work efficiently with structured data.
Error Handling: Gin streamlines error handling with built-in mechanisms for logging and managing errors. This simplification lets developers focus on building features rather than worrying about complex error management.
Setting Up Go and Gin & Building a Basic Web Application
Setting Up Go and Gin
Prerequisites: Before you begin, ensure you have Go installed on your machine. You can download it from the official Go website.
Installing Gin: Open your terminal and run the following command to install Gin:
go get -u github.com/gin-gonic/gin
Creating a Simple Gin Project:
Create a project directory for your application:
mkdir my-gin-app cd my-gin-app
Initialize the project with Go modules:
go mod init my-gin-app
Set up the folder structure. You can create a simple structure like this:
my-gin-app/ ├── main.go └── go.mod
Building a Basic Web Application
Now that your environment is set up, let’s create a basic web server using Gin.
Creating a Basic Web Server: Open the main.go
file and add the following code:
package main
import (
"net/http"
"github.com/gin-gonic/gin"
)
func main() {
router := gin.Default()
// creating a sample get request
router.GET("/api/hello", func(c *gin.Context) {
c.JSON(http.StatusOK, gin.H{"message": "Hello, World!"})
})
// creating a sample post request
router.POST("/api/data", func(c *gin.Context) {
var json map[string]interface{}
if err := c.ShouldBindJSON(&json); err == nil {
c.JSON(http.StatusOK, gin.H{"status": "Data received!", "data": json})
} else {
c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()})
}
})
// creating a sample put request
router.PUT("/api/data/:id", func(c *gin.Context) {
id := c.Param("id")
c.JSON(http.StatusOK, gin.H{"status": "Data updated!", "id": id})
})
// creating a sample delete request
router.DELETE("/api/data/:id", func(c *gin.Context) {
id := c.Param("id")
c.JSON(http.StatusOK, gin.H{"status": "Data deleted!", "id": id})
})
// starting the server on port 8080
router.Run(":8080")
}
In this example, we define simple routes that respond with a JSON message when accessed, and each route processes data in a way that mimics typical CRUD operations. To run your application, execute the following command in your terminal:
go run main.go
You can test your server in your web browser or using a tool like Postman.
Middleware in Action
Gin allows you to utilize middleware to extend your application’s functionality. You can apply middleware globally to all routes, or specifically to certain routes. Let’s add an authentication middleware to our backend.
func AuthMiddleware() gin.HandlerFunc {
return func(c *gin.Context) {
// only check for the token on POST, PUT, and DELETE requests
if c.Request.Method == http.MethodPost || c.Request.Method == http.MethodPut || c.Request.Method == http.MethodDelete {
// getting the token from the Authorization header
token := c.GetHeader("Authorization")
// getting the expected token from the environment variable
expectedToken := os.Getenv("AUTH_TOKEN")
// checking if the provided token matches the expected token
if token != expectedToken {
c.JSON(http.StatusUnauthorized, gin.H{"error": "Unauthorized"})
c.Abort() // aborting the request
return
}
}
c.Next() // proceeding to the next handler
}
}
func main (){
... // pervious code
router := gin.Default()
router.Use(AuthMiddleware())
... // pervious code
}
This middleware checks the Authorization
header for POST, PUT, and DELETE requests, ensuring only authorized users can modify data. Securing APIs is crucial, especially when they expose sensitive data or perform data manipulation. You can also extend this to use JWT-based authentication for enhanced security and flexibility.
Conclusion
By now, you’ve set up a Go development environment, built a basic web application with Gin, and implemented custom middleware to enhance your API’s security. The combination of Go's simplicity and Gin's performance makes them a powerful duo for building fast, scalable web applications. Stay tuned for more!
Subscribe to my newsletter
Read articles from Rabinson Thapa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
