A Comprehensive Guide to NextAuth.js: Secure Authentication for Next.js Applications
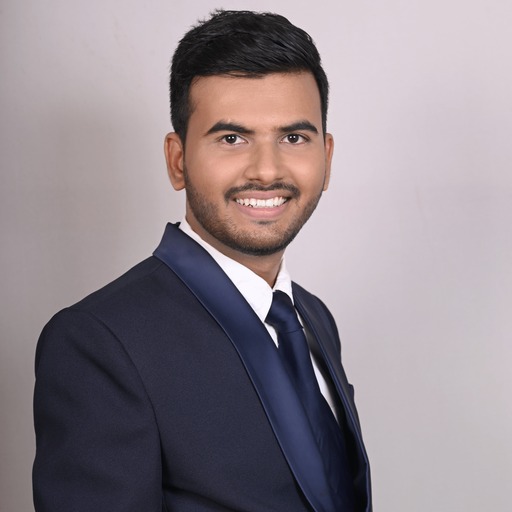
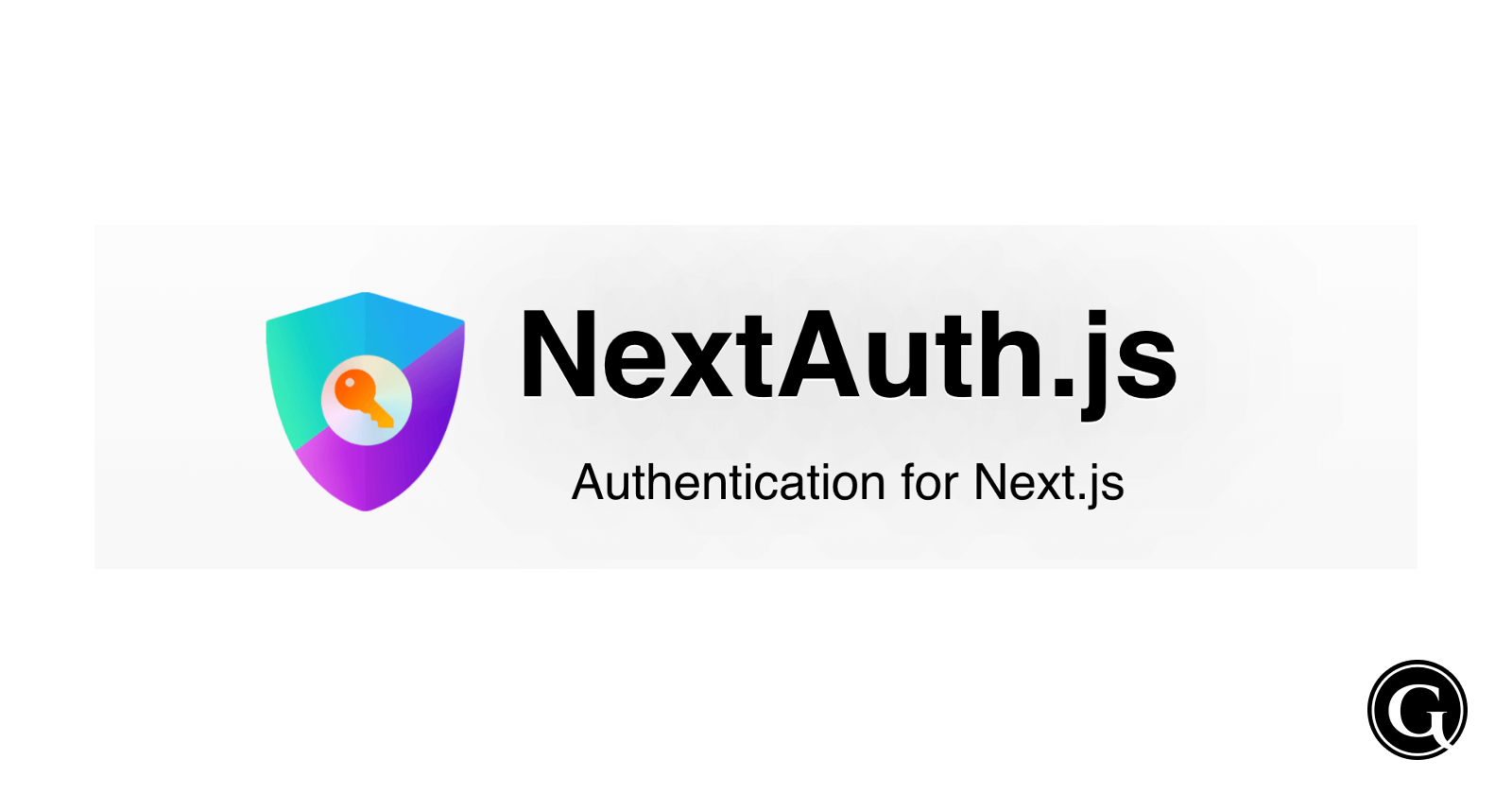
In modern web applications, authentication plays a crucial role in providing users with a personalized experience while ensuring their data's security. If you're using Next.js, one of the most powerful tools for handling authentication is NextAuth.js. In this guide, we'll explore what NextAuth.js is, its key features, and how to integrate it into your Next.js application for seamless authentication.
What is NextAuth.js?
NextAuth.js is an open-source authentication library specifically designed for Next.js applications. It simplifies the process of implementing authentication using various providers, including email/password, OAuth providers like Google, GitHub, Twitter, and even custom credentials. With NextAuth.js, you can easily manage user sessions, handle OAuth flows, and maintain secure login mechanisms.
Key Features of NextAuth.js
Provider Flexibility: NextAuth.js supports a wide range of providers like Google, Facebook, GitHub, and more. You can also implement custom credentials for scenarios where OAuth is not needed.
Session Management: It manages user sessions seamlessly with features like JWT (JSON Web Token) and session cookies.
Built-In Security: NextAuth.js is designed with security best practices, including support for CSRF (Cross-Site Request Forgery) protection.
API Route Integration: NextAuth.js integrates directly with Next.js API routes, making it easy to set up secure authentication flows.
Ease of Use: Configuration is straightforward, making it beginner-friendly while still being powerful enough for advanced scenarios.
Prerequisites
Before diving into the implementation, ensure you have the following:
A Next.js application (version 10+ recommended)
Basic knowledge of JavaScript and React
An understanding of how API routes work in Next.js
Getting Started with NextAuth.js
Let’s set up NextAuth.js in a Next.js project from scratch.
Step 1: Install NextAuth.js
Begin by installing the NextAuth.js library:
npm install next-auth
Step 2: Set Up Authentication Configuration
NextAuth.js requires an API route to handle authentication. Create a file named [...nextauth].js
in the pages/api/auth
directory:
// pages/api/auth/[...nextauth].js
import NextAuth from 'next-auth';
import GoogleProvider from 'next-auth/providers/google';
export default NextAuth({
providers: [
GoogleProvider({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
],
secret: process.env.NEXTAUTH_SECRET,
});
GoogleProvider: This allows users to sign in using their Google account. Replace
GOOGLE_CLIENT_ID
andGOOGLE_CLIENT_SECRET
with your actual Google API credentials.Environment Variables: Store sensitive data like API keys in environment variables for security. Add these to your
.env.local
file:GOOGLE_CLIENT_ID=your-google-client-id GOOGLE_CLIENT_SECRET=your-google-client-secret NEXTAUTH_SECRET=your-nextauth-secret
Step 3: Create a Sign-In Page
Create a page for users to sign in using the Google provider:
// pages/auth/signin.js
import { signIn, signOut, useSession } from 'next-auth/react';
export default function SignIn() {
const { data: session } = useSession();
if (session) {
return (
<div>
<p>Welcome, {session.user.name}!</p>
<button onClick={() => signOut()}>Sign Out</button>
</div>
);
}
return (
<div className="flex items-center justify-center min-h-screen bg-gray-100">
<div className="bg-white p-6 rounded shadow-md text-center">
<h1 className="text-2xl font-bold mb-4">Sign In</h1>
<button
onClick={() => signIn('google')}
className="bg-blue-500 hover:bg-blue-600 text-white px-4 py-2 rounded"
>
Sign in with Google
</button>
</div>
</div>
);
}
useSession: This hook from
next-auth/react
gives you access to the user's session, allowing you to render different content based on whether the user is signed in.signIn and signOut: These functions handle user login and logout actions, respectively.
Step 4: Protecting Pages with NextAuth.js
To restrict access to certain pages based on user authentication, you can use the useSession
hook and conditionally render content:
// pages/protected.js
import { useSession, signIn } from 'next-auth/react';
import { useEffect } from 'react';
export default function ProtectedPage() {
const { data: session, status } = useSession();
useEffect(() => {
if (status === 'unauthenticated') {
signIn(); // Redirects to sign-in page if not authenticated
}
}, [status]);
if (status === 'loading') {
return <p>Loading...</p>;
}
return (
<div>
<h1>Protected Page</h1>
<p>Welcome, {session.user.email}!</p>
</div>
);
}
In this example, users are automatically redirected to the sign-in page if they try to access a protected page without being authenticated.
Step 5: Managing Sessions with NextAuth.js
To manage user sessions globally, you can use NextAuth.js's built-in session management. Update _app.js
to include the session provider:
// pages/_app.js
import { SessionProvider } from 'next-auth/react';
import '../styles/globals.css';
function MyApp({ Component, pageProps: { session, ...pageProps } }) {
return (
<SessionProvider session={session}>
<Component {...pageProps} />
</SessionProvider>
);
}
export default MyApp;
This wraps the entire application with SessionProvider
, making session data available throughout the app.
Advanced Features
Custom Authentication: NextAuth.js supports custom credential providers, allowing you to define how users authenticate using your own logic. This is ideal for situations where you have a custom API.
JWT and Session Management: Customize session handling by configuring JWT expiration, encryption, and storage mechanisms.
Secure APIs: Use
getSession
orgetServerSession
on API routes to secure backend logic based on the user's session.
Best Practices
Use Environment Variables: Store secrets and keys in environment variables and avoid exposing them in your codebase.
HTTPS for Production: Ensure your Next.js app is deployed with HTTPS, as OAuth and sensitive user data should always be transmitted securely.
Regular Updates: Keep NextAuth.js updated to leverage new features and security patches.
Conclusion
NextAuth.js provides a robust and flexible way to implement authentication in Next.js applications. Whether you're working with popular OAuth providers like Google or implementing custom authentication logic, NextAuth.js makes the process seamless and secure. By following the steps outlined in this guide, you can have a fully functioning authentication flow in no time, enhancing your app's user experience and security.
With its simplicity and power, NextAuth.js is a go-to solution for Next.js developers looking to integrate authentication without the hassle of building it from scratch. Happy coding, and secure your Next.js apps with ease!
Sources
NextAuth.js Official Documentation: This is the primary resource for understanding the setup, providers, and configuration of NextAuth.js.
Next.js Documentation: Helpful for understanding how to create API routes, environment variables, and overall Next.js features like routing.
NextAuth.js GitHub Repository: Contains information about the latest updates, issues, and community discussions.
Environment Variables in Next.js: Useful when setting up credentials and secrets for OAuth providers.
OAuth 2.0 Overview: Provides a deeper understanding of how OAuth 2.0 works, which is beneficial when using providers like Google, GitHub, etc.
Community Articles and Tutorials:
Subscribe to my newsletter
Read articles from Gaurav Vishwakarma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
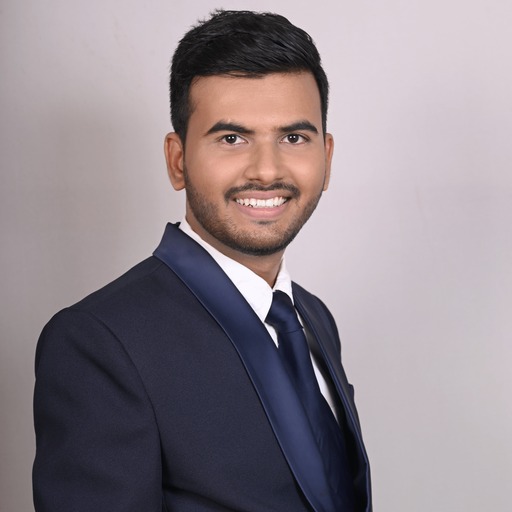