Document you NodeJS APIs the best way

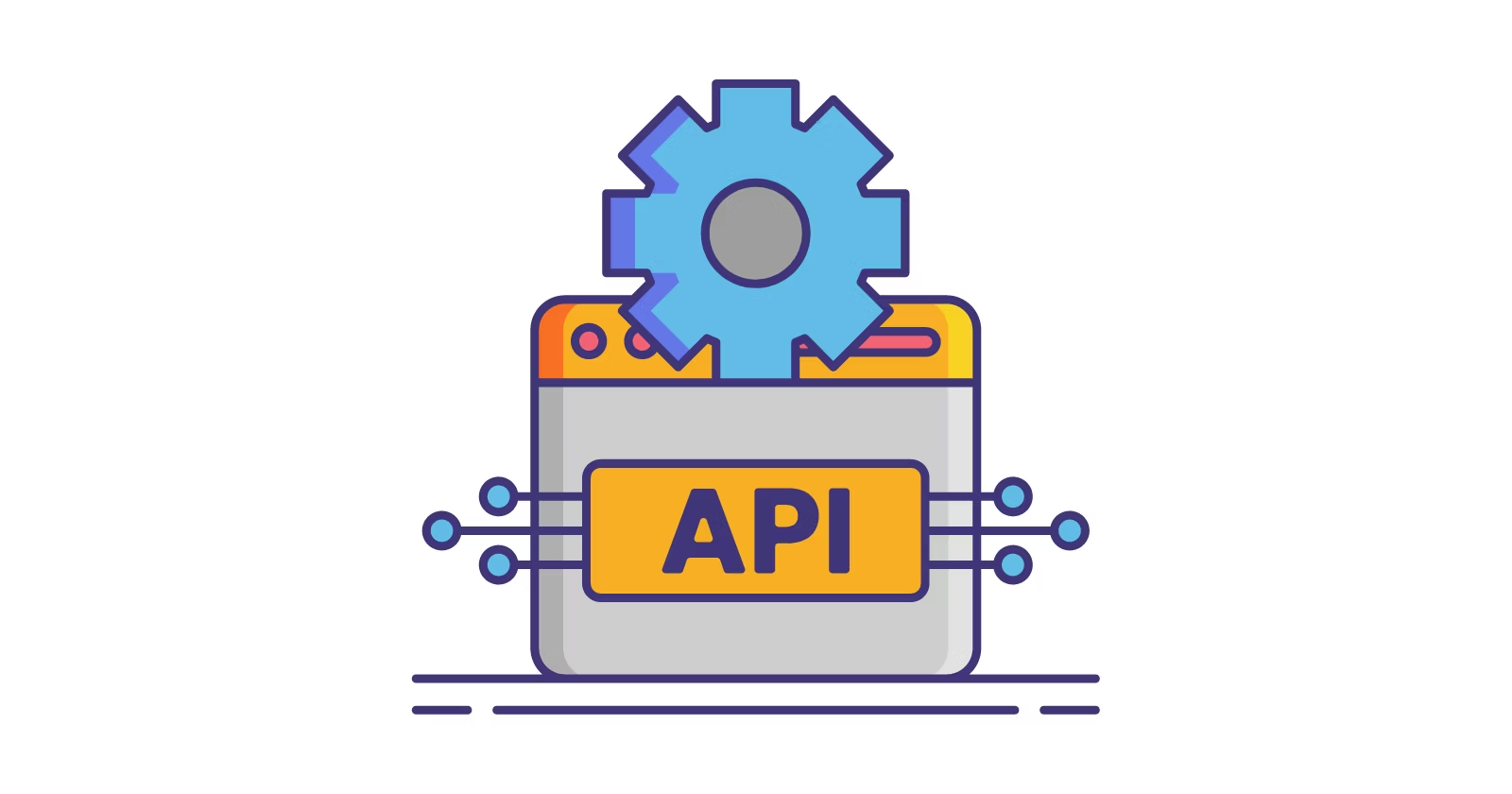
Introduction
As a Node.js developer, I’ve faced the same issue countless times: you build an awesome API with Express.js, it works great, and then… documentation. The excitement fizzles out. Let’s be real, no one loves documenting APIs, but we all appreciate good documentation when we’re on the user side.
But what if I told you that documenting your API doesn’t have to be a tedious process? In fact, with the right tools and strategies, you can create documentation that’s not only helpful but also enjoyable to write. So, let’s dive into the best ways to document APIs built with Node.js and Express.js, without sacrificing our sanity or humor (because we need both).
Why Document Your API? (Besides Guilt-Tripping Future You)
Let’s start with the basics: why should we bother documenting our API?
Onboarding – It’s much easier to bring new developers onto your project when they don’t have to reverse-engineer every API call.
Maintenance – Future-you will thank present-you when six months have passed and you’ve forgotten everything.
User Adoption – If your API is meant for public consumption, documentation is essential for getting others to use it.
Bug Prevention – Clear documentation can prevent misuse, which can reduce bugs. It’s a win-win!
Now that we’re guilt-tripped into writing documentation, let’s move on to the how.
Step 1: Use Swagger (OpenAPI)
What Is Swagger ?
Swagger (now known as OpenAPI) is the industry standard for API documentation. It allows you to describe your API in a standardized format that can be easily read by both humans and machines. Swagger also generates interactive documentation where users can try out API endpoints in real time.
How to Implement ?
The best way to integrate Swagger into your Express.js app is by using the swagger-jsdoc
and swagger-ui-express
packages.
Install the required packages:
npm install swagger-jsdoc swagger-ui-express
Set up Swagger in your app: Add this snippet to your
app.js
orindex.js
file:const swaggerUi = require('swagger-ui-express'); const swaggerJSDoc = require('swagger-jsdoc'); const swaggerOptions = { swaggerDefinition: { openapi: '3.0.0', info: { title: 'My Express API', version: '1.0.0', description: 'API documentation for my awesome Express app', }, }, apis: ['./routes/*.js'], // Adjust to match your project structure }; const swaggerDocs = swaggerJSDoc(swaggerOptions); app.use('/api-docs', swaggerUi.serve, swaggerUi.setup(swaggerDocs));
This exposes the Swagger UI at
http://localhost:3000/api-docs
(or your chosen port).Document your routes: Add comments above your API routes in the format Swagger expects. For example:
/** * @swagger * /users: * get: * summary: Retrieve a list of users * responses: * 200: * description: A list of users */ app.get('/users', (req, res) => { res.send([/* some users */]); });
Pros of Swagger:
Interactive: Users can test API endpoints directly from the documentation.
Standardized: Swagger is widely recognized, so anyone familiar with APIs will be comfortable with it.
Automation: A lot of the documentation is auto-generated, reducing manual effort.
Cons of Swagger:
Verbose: Writing the Swagger annotations can get a bit repetitive.
Setup Time: It requires some initial setup, though it pays off quickly.
Step 2: JSDoc for Inline Documentation
While Swagger is great for documenting the "what" and "how" of your API, you might still want to document the code itself. That’s where JSDoc comes into play.
What Is JSDoc?
JSDoc is a tool for adding inline comments to your code. It allows you to describe the purpose of functions, parameters, and return values, and it integrates nicely with IDEs to provide autocompletion and documentation hints.
How to Implement JSDoc:
Start by adding comments above your functions and routes, like so:
/**
* Get a list of all users.
* @param {Object} req - Express request object
* @param {Object} res - Express response object
* @returns {void}
*/
app.get('/users', (req, res) => {
res.send([/* some users */]);
});
Pros of JSDoc:
Simple: It’s just comments in your code—no need to install extra packages.
IDE Integration: IDEs like VSCode can automatically recognize and display JSDoc comments.
Inline: It’s part of the code itself, so it’s easy to keep up to date.
Cons of JSDoc:
Not User-Facing: JSDoc is great for developers working on the code, but it’s not a substitute for a public API doc like Swagger.
Limited: It won’t generate beautiful, interactive docs on its own.
Step 3: Postman Collections for Sharing and Testing
After implementing Swagger, if you want an easy way to share your API with your team or other developers, Postman collections are a lifesaver. Postman allows you to organize your API requests and share them as collections with others.
How to Implement:
Download Postman if you don’t have it already.
Manually add your API endpoints, or better yet, export them from your Swagger UI (Postman lets you import OpenAPI specs).
Group endpoints logically (e.g., User, Product, Auth).
Add descriptions, example responses, and pre-request scripts if necessary.
Once you’ve got your collection ready, you can export it and share it with others, or host it on Postman’s platform for easy access.
Pros of Postman:
Collaboration: Easily share collections with your team or external developers.
Testing: Postman is great for testing your API during development.
Automated Docs: If you combine it with Swagger, you can automatically generate collections based on your Swagger spec.
Cons of Postman:
Manual Work: You might have to manually sync changes between your API and Postman collections if you don’t automate it.
Another Tool: It’s one more tool to manage, but the benefits are huge.
Step 4: Keep a README
Finally, while Swagger and JSDoc are excellent for technical details, don’t forget your README file. This is often the first piece of documentation someone will see, and it should provide a high-level overview of your API.
What to Include in Your README:
Installation Instructions: How to set up and run the API.
Authentication Details: If your API requires authentication, explain how it works.
Endpoints Overview: Link to your Swagger docs or Postman collection for the full API reference.
Example Requests: Provide a few common requests and responses.
The README should be a user-friendly introduction, not an exhaustive technical doc. Think of it as your API’s elevator pitch.
Conclusion: The Perfect Documentation Stack
In an ideal world, here’s how you should document your Node.js and Express API:
Swagger for your interactive, user-facing API documentation.
JSDoc for internal documentation that helps developers understand your code.
Postman Collections for easy sharing and testing of your API.
A well-maintained README for a friendly overview and quick start guide.
With these tools, you can have documentation that’s easy to maintain, pleasant to use, and — dare I say — fun to write. So the next time you build an API, don’t put off the documentation. Embrace it, automate it, and make future-you proud.
Subscribe to my newsletter
Read articles from Utkarsh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Utkarsh
Utkarsh
I'm a MERN Stack developer and technical writer that loves to share his thoughts in words on latest trends and technologies. For queries and opportunities, I'm available at r.utkarsh.0010@gmail.com