Understanding ERC20 Tokens: A Deep Dive into the Standard Interface.

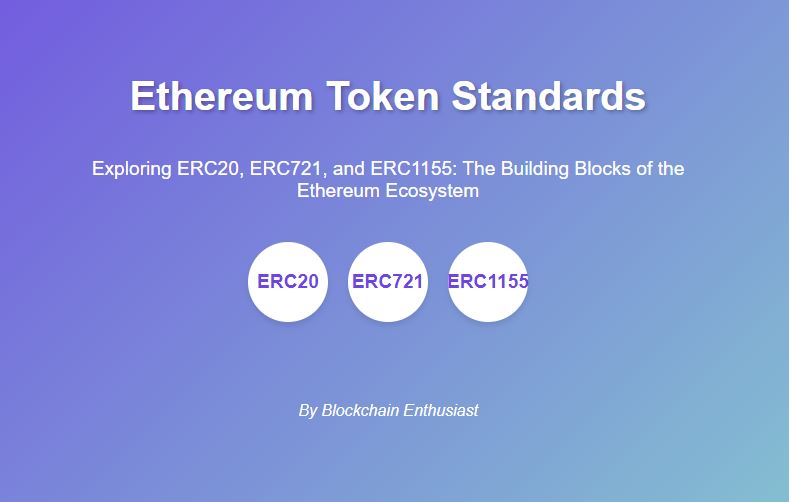
Introduction
ERC20 tokens are a fundamental part of the Ethereum ecosystem. They represent a standard for fungible tokens on the Ethereum blockchain, allowing for easy interoperability between different applications and exchanges. In this article, we'll explore the ERC20 standard in depth, focusing on the functions that make up the ERC20 token interface.
What is ERC20?
ERC20 (Ethereum Request for Comment 20) is a technical standard for implementing tokens on the Ethereum blockchain. It defines a common list of rules that an Ethereum token contract must implement, allowing developers to program how new tokens will function within the Ethereum ecosystem.
The ERC20 Interface
The ERC20 standard defines several functions that a token contract must implement. Let's break down each of these functions:
1. totalSupply()
This function returns the total number of tokens in existence.
function totalSupply() public view returns (uint256);
2. balanceOf(address account)
This function returns the account balance of the specified address.
function balanceOf(address account) public view returns (uint256);
3. transfer(address recipient, uint256 amount)
This function transfers a specified amount of tokens from the function caller's address to the recipient's address.
function transfer(address recipient, uint256 amount) public returns (bool);
4. allowance(address owner, address spender)
This function returns the remaining number of tokens that the spender is allowed to spend on behalf of the owner.
function allowance(address owner, address spender) public view returns (uint256);
5. approve(address spender, uint256 amount)
This function sets the amount of allowance the spender is allowed to transfer from the function caller's address.
function approve(address spender, uint256 amount) public returns (bool);
6. transferFrom(address sender, address recipient, uint256 amount)
This function allows a contract to transfer tokens on your behalf. It's used for delegated token transfers.
function transferFrom(address sender, address recipient, uint256 amount) public returns (bool);
Events
The ERC20 standard also defines two events that must be emitted when certain actions occur:
1. Transfer Event
This event should be emitted when tokens are transferred, including zero value transfers.
event Transfer(address indexed from, address indexed to, uint256 value);
2. Approval Event
This event should be emitted when the approve function is called successfully.
event Approval(address indexed owner, address indexed spender, uint256 value);
Optional Functions
While not required by the ERC20 standard, many token implementations include these additional functions:
1. name()
Returns the name of the token.
function name() public view returns (string);
2. symbol()
Returns the symbol of the token.
function symbol() public view returns (string);
3. decimals()
Returns the number of decimals the token uses.
function decimals() public view returns (uint8);
Putting It All Together
Here's a basic implementation of an ERC20 token contract that includes all the required functions and events:
pragma solidity ^0.8.0;
contract ERC20Token {
string public name;
string public symbol;
uint8 public decimals;
uint256 private _totalSupply;
mapping(address => uint256) private _balances;
mapping(address => mapping(address => uint256)) private _allowances;
event Transfer(address indexed from, address indexed to, uint256 value);
event Approval(address indexed owner, address indexed spender, uint256 value);
constructor(string memory _name, string memory _symbol, uint8 _decimals, uint256 initialSupply) {
name = _name;
symbol = _symbol;
decimals = _decimals;
_totalSupply = initialSupply;
_balances[msg.sender] = initialSupply;
}
function totalSupply() public view returns (uint256) {
return _totalSupply;
}
function balanceOf(address account) public view returns (uint256) {
return _balances[account];
}
function transfer(address recipient, uint256 amount) public returns (bool) {
require(_balances[msg.sender] >= amount, "Insufficient balance");
_balances[msg.sender] -= amount;
_balances[recipient] += amount;
emit Transfer(msg.sender, recipient, amount);
return true;
}
function allowance(address owner, address spender) public view returns (uint256) {
return _allowances[owner][spender];
}
function approve(address spender, uint256 amount) public returns (bool) {
_allowances[msg.sender][spender] = amount;
emit Approval(msg.sender, spender, amount);
return true;
}
function transferFrom(address sender, address recipient, uint256 amount) public returns (bool) {
require(_balances[sender] >= amount, "Insufficient balance");
require(_allowances[sender][msg.sender] >= amount, "Insufficient allowance");
_balances[sender] -= amount;
_balances[recipient] += amount;
_allowances[sender][msg.sender] -= amount;
emit Transfer(sender, recipient, amount);
return true;
}
}
Conclusion
Understanding the ERC20 standard is crucial for anyone working with Ethereum tokens. By implementing these functions and events, developers ensure that their tokens can interact seamlessly with other contracts and applications in the Ethereum ecosystem. Whether you're creating your own token or integrating existing tokens into your dApp, a solid grasp of ERC20 is essential.
Remember, while ERC20 is the most widely used token standard, there are other standards like ERC721 for non-fungible tokens (NFTs) and ERC1155 for multi-token contracts. Each serves different purposes in the ever-evolving world of blockchain technology.
Subscribe to my newsletter
Read articles from lilmisssomeone directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
