🚀 Day 8: Shell Scripting Challenge – Mastering the Basics of Bash!

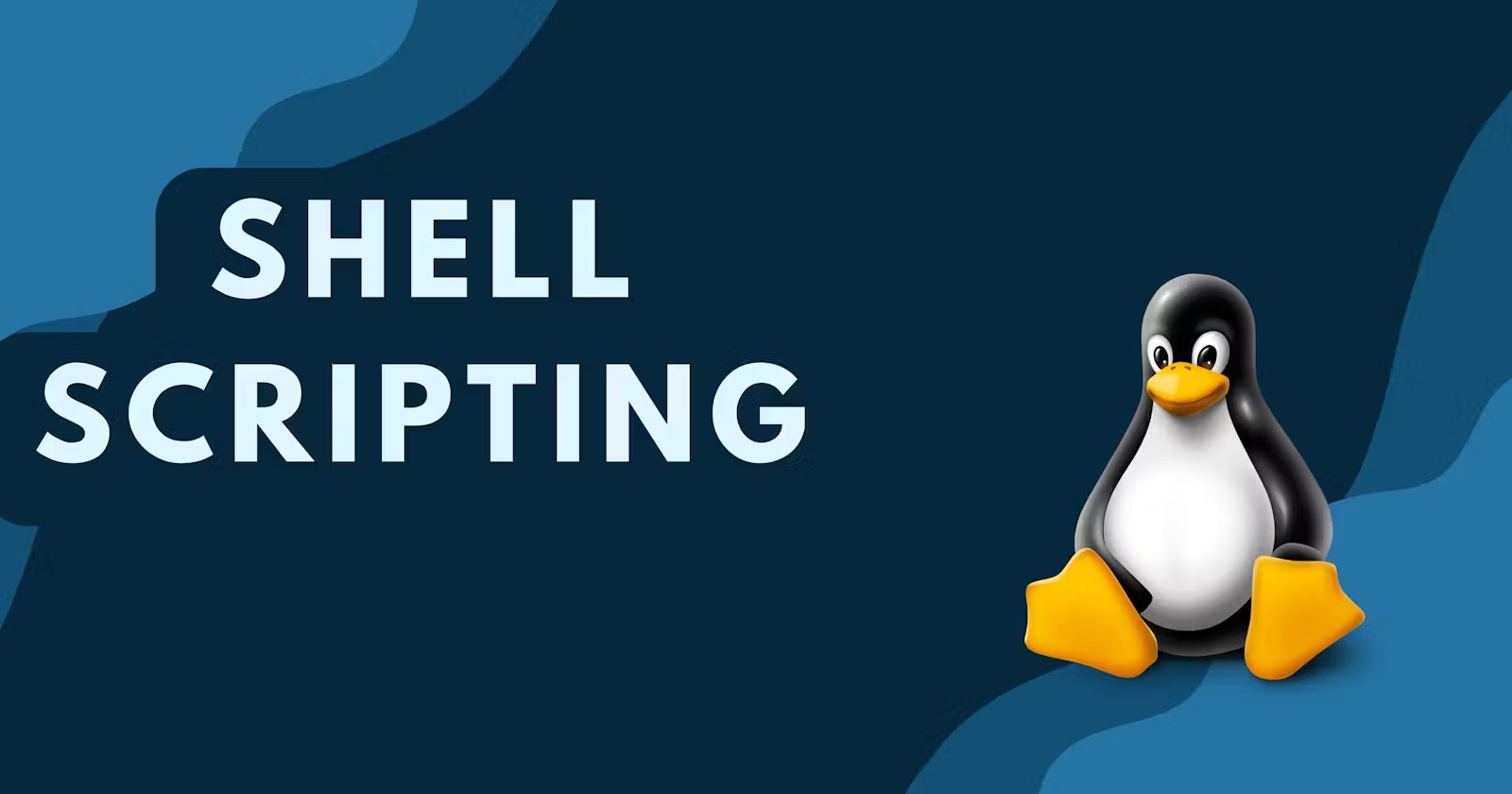
Hey Techies! 👋
Today, we dive deep into the world of Bash scripting! If you’ve been following along with the #90DaysOfDevOps journey, you know how critical shell scripting is in automating tasks and making your system administration life easier. 💻
🧠 What’s the Task?
Day 8 is all about mastering the basic yet essential elements of bash scripting. From comments to wildcards, each concept makes your scripts more powerful and efficient. So, let’s walk through this one task at a time and see some code examples.
Task 1: 📝 Comments in Bash
Just like in any programming language, comments are used in Bash scripts to explain what certain parts of your script are doing. This makes your code more readable and maintainable.
Here’s an example:
#!/bin/bash
# This script demonstrates the use of comments in bash
# The line below prints a message to the terminal
echo "Hello, World! Welcome to the Bash Scripting Challenge!"
The #
symbol is used to add comments in bash. Make sure to add comments that explain each important part of your script. It'll help anyone (including future-you) understand what the script is doing.
Task 2: 🗣️ Echo – Displaying Messages
The echo
command is your go-to for printing messages to the terminal. It's perfect for displaying outputs or debugging information.
Here’s a simple example:
#!/bin/bash
# Display a message to the user
echo "Today's challenge is all about mastering bash scripting!"
This script will display:Today's challenge is all about mastering bash scripting!
You can add this to your scripts to give feedback or explain results to users.
Task 3: 📦 Variables in Bash
Variables in Bash allow you to store data like text or numbers that you can reuse throughout your script. You can declare a variable using simple syntax: variable_name=value
.
Here's an example:
#!/bin/bash
# Declare variables
greeting="Hello"
name="Chintamani"
# Use the variables
echo "$greeting, $name! Let's get started with bash scripting."
In this example, greeting
stores the text "Hello" and name
stores "Chintamani". The echo command then uses these variables to display a personalized message.
Task 4: 🧮 Using Variables for Math
Now let’s do something a little more useful! How about performing arithmetic using variables?
#!/bin/bash
# Declare two numbers
num1=15
num2=35
# Calculate their sum
sum=$((num1 + num2))
# Display the result
echo "The sum of $num1 and $num2 is: $sum"
In this script, we take two numbers, calculate their sum, and print it. The output will be:The sum of 15 and 35 is: 50
Now, imagine doing complex calculations in your scripts without having to do the math manually. 😉
Task 5: 🛠️ Using Built-in Variables
Bash has some amazing built-in variables that give you information about the system, the script itself, and the user running it.
Here’s how you can use them:
#!/bin/bash
# Display information using built-in variables
echo "The script name is: $0"
echo "Current user: $USER"
echo "Home directory: $HOME"
$0
shows the name of the script.$USER
gives the current logged-in user.$HOME
shows the path to the user’s home directory.
Output:
The script name is: ./task.sh
Current user: chintamani
Home directory: /home/chintamani
Task 6: 🔍 Wildcards for File Matching
Wildcards are special characters that allow you to match files based on patterns, saving you a lot of time.
Let’s use wildcards to list all .sh
files in the current directory:
#!/bin/bash
# Use wildcards to list all .sh files
echo "Listing all shell script files (*.sh) in the current directory:"
ls *.sh
This script will find and display all files ending with .sh
. You don’t have to list files manually—wildcards handle it for you. ✨
👨💻 My Day 8 Task Scripts in Action!
Here’s a sneak peek at my Bash scripts in action. Check out the results for each task and how I used comments, echo commands, variables, built-in variables, and wildcards. Take a look at the screenshots for a real-world view!
🎯 Wrapping Up
Day 8 is complete! 🏅 Each task you worked through gives you a solid foundation for writing Bash scripts. You’ve learned how to:
Use comments to document your code
Display messages with echo
Declare and use variables
Perform arithmetic operations
Utilize built-in variables for handy system info
Leverage wildcards to match files
Thanks for reading, and happy scripting! 😄🚀
Subscribe to my newsletter
Read articles from Chintamani Tare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Chintamani Tare
Chintamani Tare
👨💻 Chintamani Tare | DevOps Enthusiast & Linux Advocate 🌐 I'm a passionate DevOps engineer with a solid foundation in Linux system administration. With a deep interest in automation, cloud technologies, and CI/CD pipelines, I love simplifying complex tasks and building scalable infrastructure. Whether it's scripting in Bash, managing servers with Ansible, or deploying applications with Docker and Kubernetes, I'm always eager to explore the latest tools and practices in the DevOps space.