Understanding the Difference Between pluck() and select() in Laravel 11

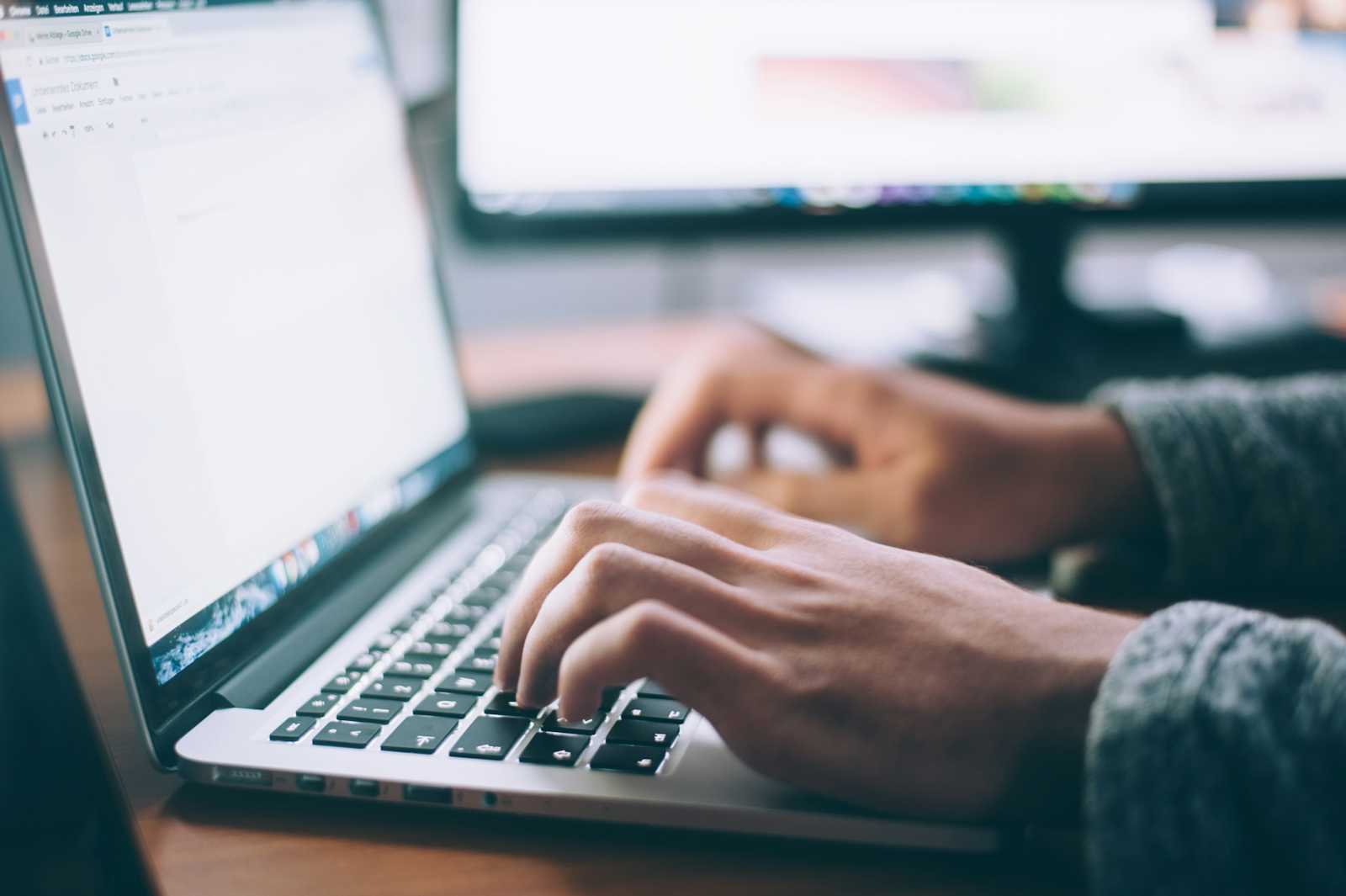
Laravel, one of the most popular PHP frameworks, offers a wide array of methods to simplify data manipulation. Among these methods, pluck()
and select()
are frequently used to extract data from collections. While they may seem similar at first glance, they serve different purposes. In this article, we’ll explore the differences between pluck()
and select()
, when to use each one, and provide examples for better clarity.
What is pluck()
?
The pluck()
method is used to retrieve all values for a specific key from a collection. It’s a simple and efficient way to extract a single attribute from a list of objects.
Example of pluck()
Consider a collection of products with their IDs and names:
$collection = collect([
['product_id' => 'prod-100', 'name' => 'Desk'],
['product_id' => 'prod-200', 'name' => 'Chair'],
]);
// Pluck only the names of the products
$plucked = $collection->pluck('name');
$plucked->all();
// Output: ['Desk', 'Chair']
You can also specify how to key the resulting collection. For instance, if you want to key by product_id
:
$plucked = $collection->pluck('name', 'product_id');
$plucked->all();
// Output: ['prod-100' => 'Desk', 'prod-200' => 'Chair']
Nested Values with pluck()
The pluck()
method supports retrieving nested values using dot notation. For instance:
$collection = collect([
[
'name' => 'Laracon',
'speakers' => [
'first_day' => ['Rosa', 'Judith'],
],
],
[
'name' => 'VueConf',
'speakers' => [
'first_day' => ['Abigail', 'Joey'],
],
],
]);
$plucked = $collection->pluck('speakers.first_day');
$plucked->all();
// Output: [['Rosa', 'Judith'], ['Abigail', 'Joey']]
Handling Duplicates in pluck()
If there are duplicate keys, the last matching element will be used in the resulting collection:
$collection = collect([
['brand' => 'Tesla', 'color' => 'red'],
['brand' => 'Pagani', 'color' => 'white'],
['brand' => 'Tesla', 'color' => 'black'],
['brand' => 'Pagani', 'color' => 'orange'],
]);
$plucked = $collection->pluck('color', 'brand');
$plucked->all();
// Output: ['Tesla' => 'black', 'Pagani' => 'orange']
What is select()
?
The select()
method in Laravel is similar to the SQL SELECT
statement. It allows you to retrieve specified columns from a collection, returning a new collection containing only the selected keys.
Example of select()
Consider a collection of users with various attributes:
$users = collect([
['name' => 'Taylor Otwell', 'role' => 'Developer', 'status' => 'active'],
['name' => 'Victoria Faith', 'role' => 'Researcher', 'status' => 'active'],
]);
$selectedUsers = $users->select(['name', 'role']);
$selectedUsers->all();
// Output: [
// ['name' => 'Taylor Otwell', 'role' => 'Developer'],
// ['name' => 'Victoria Faith', 'role' => 'Researcher'],
// ]
With select()
, you can specify multiple attributes to be returned for each item in the collection, creating a new structure with only the selected keys.
Key Differences Between pluck()
and select()
Purpose:
pluck()
is designed to retrieve a single attribute from a collection.select()
allows you to retrieve multiple attributes and returns a collection with only the specified keys.
Return Structure:
pluck()
provides a flat collection of values or a key-value pair collection if a key is specified.select()
returns an array of arrays, with each array containing the selected keys.
Usage:
Use
pluck()
when you need values from a single key in the collection.Use
select()
when you need to extract multiple attributes from each item.
When to Use pluck()
vs. select()
Use
pluck()
when:You need a list of values for a single attribute from a collection.
You want to retrieve nested values from complex objects.
Use
select()
when:You need to extract multiple keys or fields from a collection.
You want to return a new collection that includes only the selected attributes.
Conclusion
Both pluck()
and select()
are incredibly useful methods in Laravel’s collection toolkit. Understanding the difference between them allows you to choose the right method based on your needs. Use pluck()
for quick extractions of a single attribute and select()
when you need to return multiple attributes. Mastering these methods will make your data manipulation more efficient and keep your Laravel code clean and concise.
By leveraging these powerful methods, you can enhance the performance and readability of your Laravel applications. Happy coding!
Subscribe to my newsletter
Read articles from Asfia Aiman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Asfia Aiman
Asfia Aiman
Hey Hashnode community! I'm Asfia Aiman, a seasoned web developer with three years of experience. My expertise includes HTML, CSS, JavaScript, jQuery, AJAX for front-end, PHP, Bootstrap, Laravel for back-end, and MySQL for databases. I prioritize client satisfaction, delivering tailor-made solutions with a focus on quality. Currently expanding my skills with Vue.js. Let's connect and explore how I can bring my passion and experience to your projects! Reach out to discuss collaborations or learn more about my skills. Excited to build something amazing together! If you like my blogs, buy me a coffee here https://www.buymeacoffee.com/asfiaaiman