Activity 26: CSS FLEX
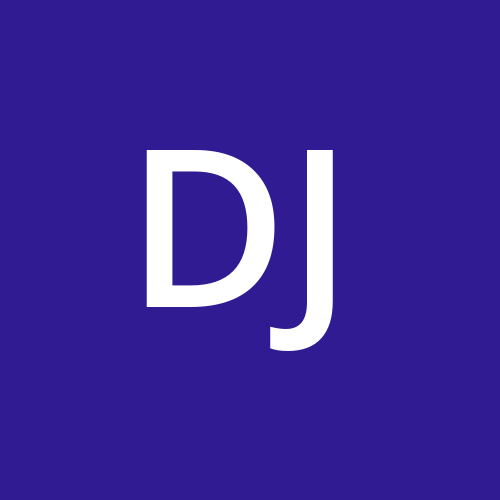

In the world of web development, creating responsive and flexible layouts is key to delivering a great user experience across various screen sizes. CSS Flexbox, short for Flexible Box Layout, is a powerful tool that makes it easier to align, distribute, and manage space among elements in a container. This article provides a hands-on approach to mastering Flexbox by exploring its functions and importance through real-world layouts such as product displays, employee cards, and student profiles.
What is CSS Flexbox?
CSS Flexbox is a layout model designed to allow elements within a container to align and distribute space dynamically, depending on the available screen size or container space. Unlike traditional layouts that rely on floats, CSS Flexbox provides more control over alignment, direction, and space distribution within a flex container. It's perfect for one-dimensional layouts (either a row or a column).
Key Flexbox Properties:
display: flex
: Defines a flex container and enables Flexbox for its child elements.justify-content
: Aligns items horizontally in a flex container (e.g., start, center, space-between).align-items
: Aligns items vertically in a flex container.flex-wrap
: Determines whether flex items should wrap onto multiple lines when necessary.flex-direction
: Sets the direction of the flex items (row, column, row-reverse, column-reverse).
Practical Use Cases:
Example 1: product.html
The product layout page demonstrates how we can use Flexbox to align product cards in a responsive grid. We set the container’s display
property to flex
and use justify-content: space-around
to distribute the product cards evenly.
Flexbox properties used:
display: flex
: Defines the container as a Flexbox.justify-content: space-around
: Distributes space between and around the product items.flex-wrap: wrap
: Ensures that the products wrap into multiple rows when the screen size is reduced.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Product Layout</title>
</head>
<body style="font-family: Arial, sans-serif;">
<h1>Our Products</h1>
<div style="display: flex; justify-content: space-around; flex-wrap: wrap;">
<div style="border: 1px solid #ddd; padding: 10px; width: 30%; margin-bottom: 20px;">
<img src="https://i.pinimg.com/236x/d4/13/f3/d413f351550c68c4297b3e39032eac83.jpg" alt="Product 1" style="width: 100%;">
<h3>Wireless Headphones</h3>
<p>Price: Php500</p>
</div>
<div style="border: 1px solid #ddd; padding: 10px; width: 30%; margin-bottom: 20px;">
<img src="https://i.pinimg.com/236x/d5/1b/0d/d51b0d8826063f245dc38e9ff6c5c263.jpg" alt="Product 2" style="width: 100%;">
<h3>Smartphone</h3>
<p>Price: Php6000</p>
</div>
<div style="border: 1px solid #ddd; padding: 10px; width: 30%; margin-bottom: 20px;">
<img src="https://i.pinimg.com/control/236x/78/bf/a8/78bfa893270a0b531705b1c56f25674d.jpg" alt="Product 3" style="width: 100%;">
<h3>Laptop</h3>
<p>Price: Php23000</p>
</div>
</div>
</body>
OUTPUT:
Example 2: employee.html
In the employee cards page, we use Flexbox to center the cards and ensure equal spacing between them. Flexbox simplifies aligning and distributing the cards in a neat row. This is especially useful when displaying team member profiles or similar content.
Flexbox properties used:
justify-content: space-evenly
: Distributes equal space between the employee cards.align-items: center
: Vertically centers the cards within the container.flex-direction
: Ensures the layout is horizontal.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Employee Cards</title>
</head>
<body style="font-family: Arial, sans-serif;">
<h1>Meet Our Team</h1>
<div style="display: flex; justify-content: space-evenly; flex-wrap: wrap;">
<div style="border: 1px solid #ddd; padding: 20px; width: 25%; margin-bottom: 20px; text-align: center;">
<img src="https://i.pinimg.com/control/236x/0d/29/9d/0d299d73242a80d8943f2dc81d21ea3c.jpg" alt="Employee 1" style="width: 100%; border-radius: 50%;">
<h3>Alice Johnson</h3>
<p>Position: Project Manager</p>
</div>
<div style="border: 1px solid #ddd; padding: 20px; width: 25%; margin-bottom: 20px; text-align: center;">
<img src="https://i.pinimg.com/236x/fd/07/b2/fd07b262040c7521fdd4184dcce83806.jpg" alt="Employee 2" style="width: 100%; border-radius: 50%;">
<h3>Bob Smith</h3>
<p>Position: Lead Developer</p>
</div>
<div style="border: 1px solid #ddd; padding: 20px; width: 25%; margin-bottom: 20px; text-align: center;">
<img src="https://i.pinimg.com/236x/c0/28/d8/c028d83e001aaf922a211b5804b0cdac.jpg" alt="Employee 3" style="width: 100%; border-radius: 50%;">
<h3>Claire Brown</h3>
<p>Position: UI/UX Designer</p>
</div>
</div>
</body>
</html>
Output:
3.student.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Student Profiles</title>
</head>
<body style="font-family: Arial, sans-serif;">
<h1>Student Profiles</h1>
<div style="display: flex; flex-direction: column; align-items: center;">
<div style="display: flex; width: 50%; justify-content: space-between; margin-bottom: 20px;">
<div>
<img src="https://i.pinimg.com/236x/99/5f/81/995f8106f7812e5e2ce5419ea006c82b.jpg" alt="Student 1" style="width: 100px; height: 100px; border-radius: 50%;">
</div>
<div style="flex-grow: 1; margin-left: 20px;">
<h3>John Doe</h3>
<p>Major: Computer Science</p>
<p>Year: Sophomore</p>
</div>
</div>
<div style="display: flex; width: 50%; justify-content: space-between; margin-bottom: 20px;">
<div>
<img src="https://i.pinimg.com/236x/93/79/d2/9379d261dfd39d582b0dc96a09f8a324.jpg" alt="Student 2" style="width: 100px; height: 100px; border-radius: 50%;">
</div>
<div style="flex-grow: 1; margin-left: 20px;">
<h3>Jane Smith</h3>
<p>Major: Mechanical Engineering</p>
<p>Year: Senior</p>
</div>
</div>
</div>
</body>
</html>
OUTPUT:
4. project.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Project Display</title>
</head>
<body style="font-family: Arial, sans-serif;">
<h1>Current Projects</h1>
<div style="display: flex; justify-content: space-evenly; flex-wrap: wrap;">
<div style="border: 1px solid #ddd; padding: 20px; width: 45%; margin-bottom: 20px;">
<h3>AI Research Initiative</h3>
<p>We are exploring new ways to integrate AI into daily workflows.</p>
</div>
<div style="border: 1px solid #ddd; padding: 20px; width: 45%; margin-bottom: 20px;">
<h3>Sustainable Energy Project</h3>
<p>This project focuses on developing sustainable energy solutions.</p>
</div>
</div>
</body>
</html>
OUTPUT:
5. blog.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Blog Articles</title>
</head>
<body style="font-family: Arial, sans-serif;">
<h1>Recent Blog Posts</h1>
<div style="display: flex; flex-direction: column; align-items: center;">
<div style="display: flex; width: 60%; justify-content: space-between; margin-bottom: 20px;">
<h2>How to Learn Flexbox</h2>
<p>Published on Oct 10, 2024</p>
</div>
<div style="display: flex; width: 60%; justify-content: space-between; margin-bottom: 20px;">
<h2>The Future of Web Development</h2>
<p>Published on Oct 12, 2024</p>
</div>
<div style="display: flex; width: 60%; justify-content: space-between; margin-bottom: 20px;">
<h2>Understanding CSS Grid</h2>
<p>Published on Oct 14, 2024</p>
</div>
</div>
</body>
</html>
OUTPUT:
Why is Flexbox important?
Flexbox plays a critical role in modern web development, especially for responsive design. Here’s why:
Responsive by Default: Flexbox helps create layouts that adapt to different screen sizes without additional CSS media queries.
Better Alignment Control: It simplifies the complex alignment of items (both vertically and horizontally) that previously required hacks like floats or negative margins.
Dynamic Layouts: With Flexbox, you can easily create layouts that change based on the size of the container, ideal for building adaptable UIs.
Flexbox Best Practices
Use Flexbox for one-dimensional layouts (either row or column). For two-dimensional layouts (grid-like), CSS Grid may be a better choice.
Always define a fallback for older browsers that do not support Flexbox fully (e.g., IE).
Combine Flexbox with media queries to further refine responsiveness.
In conclusion, CSS Flexbox is an essential tool for creating responsive, flexible layouts with ease. By applying it to real-world scenarios, such as product grids and profile cards, you’ll gain a better understanding of how Flexbox simplifies web design. Now, it's time to try Flexbox in your projects and see how it transforms your layouts!
Github Repository Link: CSS_FLEX
Subscribe to my newsletter
Read articles from Danilo Buenafe Jr directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
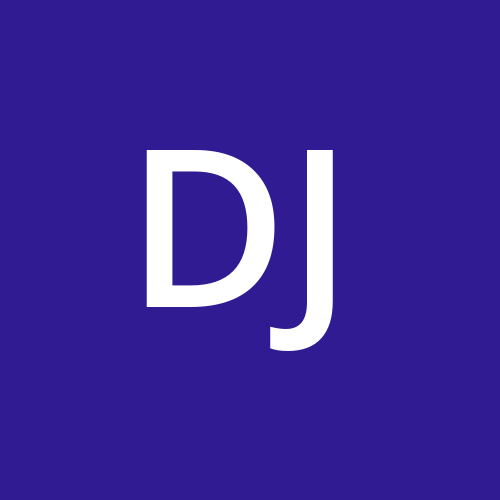