Customize your PowerShell prompt
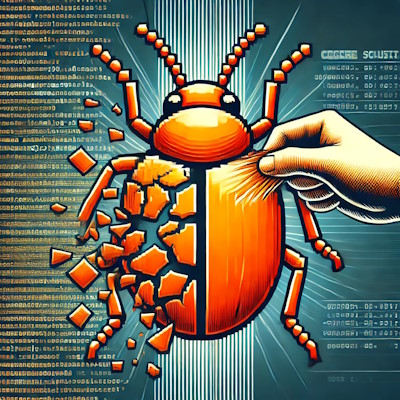
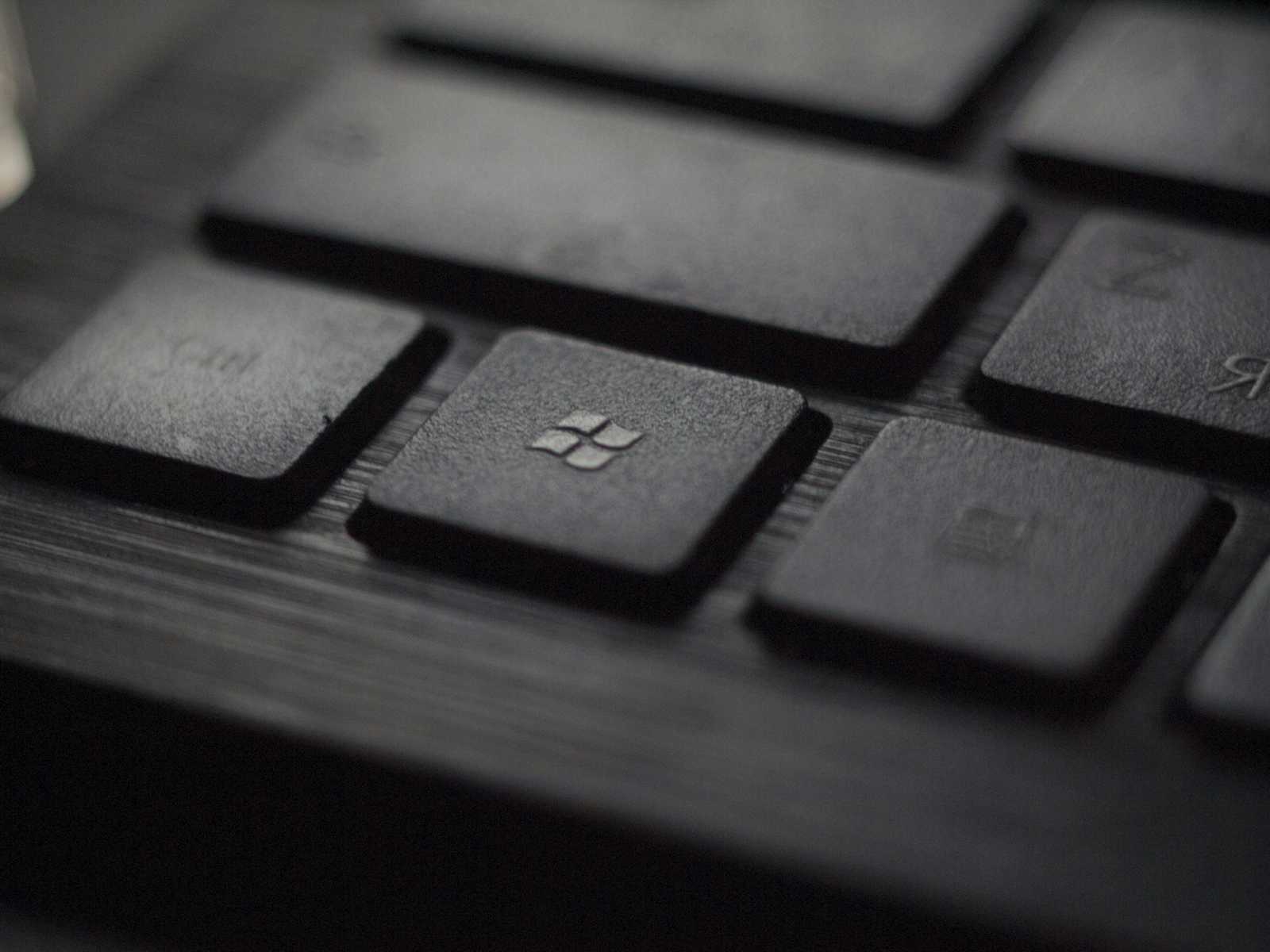
The default PowerShell prompt is pretty basic and not very informative. It only shows “PS”, followed by a “>” sign. Luckily, it's possible to customize it by editing the PowerShell profile file.
Here’s what it looks like before and after:
PowerShell profile
I'm assuming you're using a recent version of PowerShell, and not the old “Windows PowerShell”. If that's not the case, download it here. This guide has been tested on PowerShell 7.4.5. I also recommend using the Windows Terminal as your PowerShell console.
The PowerShell profile is a PowerShell script that is automatically run when a new PowerShell session is started. It's located in the ~\Documents\PowerShell\Microsoft.PowerShell_profile.ps1
file. This file path is also stored in the $PROFILE
environment variable, so if you're in doubt, just type $PROFILE
in a PowerShell session. This file probably doesn't exist if you've never tinkered with your PowerShell configuration. If that is the case, you should create it.
Prompt function
In this file, there's a function named prompt
that returns the prompt string. As a test, let's write this:
function prompt {
return "test"
}
Open a new terminal tab or window, and you should see the “test” string in the prompt. In the screenshot above, we can see that the new prompt is composed of four parts:
“PS” in blue, because I want to remember that I'm using PowerShell and not another shell
The current directory, in cyan
The current Git branch, in gold, but only if the current directory is inside a Git repository
“>”, which is colored in green because I don't have admin privileges. It will be a red “#” if I do have admin privileges.
Current directory
We'll start by getting the current directory path, and then extract the last part to a variable. It will contain the current folder name.
$currentFolder = Split-Path -Leaf -Path (Get-Location)
Current Git branch
For the next part, you'll need to have Git installed. The following command gets the name of the current branch.
$gitBranch = git rev-parse --abbrev-ref HEAD 2>$null
rev-parse
is a Git command to retrieve specific information about Git references (like branches). abbrev-ref
tells rev-parse
to print the shortest possible reference name, which is the branch name. HEAD
is the reference to the current branch. The 2>$null
part is used to suppress any error message in case we're not in a Git repository. 2>
redirects the standard error output (stream 2) to another location. And we direct it to $null
, which means that any error message will be suppressed.
Administrator status
To know if the current user has administrator privileges, we can use the [Security.Principal.WindowsPrincipal]
class, which represents a Windows user or group. We then check if the current user has the “Administrator” role.
$isAdmin = ([Security.Principal.WindowsPrincipal] [Security.Principal.WindowsIdentity]::GetCurrent()).
IsInRole([Security.Principal.WindowsBuiltInRole] "Administrator")
We can then choose which prompt character to use based on the $isAdmin
variable.
$promptChar = if ($isAdmin) { "#" } else { ">" }
Piecing it together
Let's define a color for each part of the prompt.
$psColor = [System.ConsoleColor]::Blue
$folderColor = [System.ConsoleColor]::Cyan
$gitColor = [System.ConsoleColor]::DarkYellow
$promptCharColor = if ($isAdmin) { [System.ConsoleColor]::Red } else { [System.ConsoleColor]::Green }
It's now time to write the prompt to the standard output, with the appropriate colors and spacing.
# Write each part of the prompt with appropriate colors
Write-Host "PS " -NoNewline -ForegroundColor $psColor
Write-Host $currentFolder -NoNewline -ForegroundColor $folderColor
if ($gitBranch) {
Write-Host " $gitBranch" -NoNewline -ForegroundColor $gitColor
}
Write-Host " $promptChar" -NoNewline -ForegroundColor $promptCharColor
# Return a space to separate the prompt from user input
return " "
Here's the complete script.
function prompt {
# Get the name of the current folder
$currentFolder = Split-Path -Leaf -Path (Get-Location)
# Get the current Git branch name, if in a Git repository
$gitBranch = git rev-parse --abbrev-ref HEAD 2>$null
# Check if the current user has administrator privileges
$isAdmin = ([Security.Principal.WindowsPrincipal][Security.Principal.WindowsIdentity]::GetCurrent()).
IsInRole([Security.Principal.WindowsBuiltInRole] "Administrator")
# Set the prompt character based on admin status
$promptChar = if ($isAdmin) { "#" } else { ">" }
# Define colors for different parts of the prompt
$psColor = [System.ConsoleColor]::Blue
$folderColor = [System.ConsoleColor]::Cyan
$gitColor = [System.ConsoleColor]::DarkYellow
$promptCharColor = if ($isAdmin) { [System.ConsoleColor]::Red } else { [System.ConsoleColor]::Green }
# Write each part of the prompt with appropriate colors
Write-Host "PS " -NoNewline -ForegroundColor $psColor
Write-Host $currentFolder -NoNewline -ForegroundColor $folderColor
if ($gitBranch) {
Write-Host " $gitBranch" -NoNewline -ForegroundColor $gitColor
}
Write-Host " $promptChar" -NoNewline -ForegroundColor $promptCharColor
# Return a space to separate the prompt from user input
return " "
}
Conclusion
You can go crazy with this, adding background colors and more. I wanted to keep it clean and simple, and you can use this as a starting point to create your own. Do you have any cool functionality in your PowerShell profile? Please let me know!
Subscribe to my newsletter
Read articles from Bug Buster Bruno directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
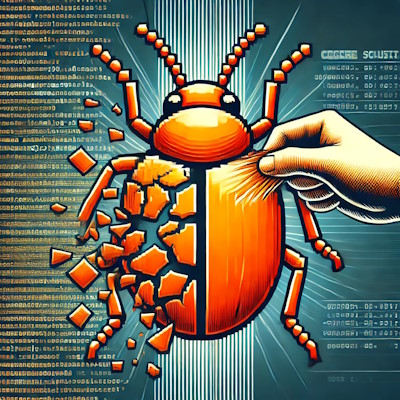