10 Famous Node.js Packages with Examples

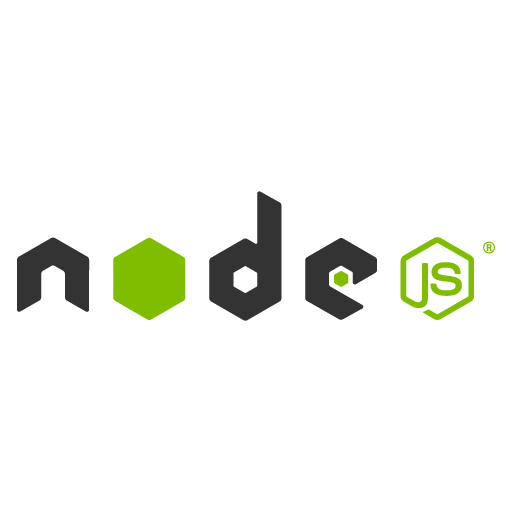
what is Node.js?
Node.js is a JavaScript runtime built on Chrome's V8 engine, allowing developers to execute JavaScript code server-side.
Node.js enables developers to use JavaScript for both client-side and server-side scripting, creating a seamless development experience.
Why are Node.js Packages Needed?
Node.js packages are essential because they allow developers to reuse code, simplify tasks, and break applications into modular components. This leads to faster development, easier maintenance, and access to a vast ecosystem of libraries for solving common problems, like handling requests, databases, and more.
10 Best Packages are :
1)Express
Express is the most popular web framework for Node.js, known for its minimalist design and flexibility.
It simplifies the process of building web applications and APIs by providing robust routing and middleware support.
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => res.send('Hello World!'));
app.listen(port, () => {
console.log(`Example app listening at http://localhost:${port}`);
});
2)Socket.io
- Socket.io enables real-time bidirectional communication between clients and servers.
It's essential for developing interactive applications like chat apps or live notifications, making it a go-to library for real-time features.
const http = require('http'); const socketIo = require('socket.io'); const server = http.createServer(); const io = socketIo(server); io.on('connection', (socket) => { console.log('A user connected'); socket.on('chat message', (msg) => { console.log('Message: ' + msg); io.emit('chat message', msg); }); }); server.listen(3000, () => { console.log('Server is running on port 3000'); });
3. Mongoose
- Mongoose is an Object Data Modeling (ODM) library for MongoDB and Node.js.
- It provides a schema-based solution to model application data, making it easier to work with MongoDB.
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/test', { useNewUrlParser: true, useUnifiedTopology: true });
const Schema = mongoose.Schema;
const UserSchema = new Schema({
name: String,
age: Number
});
const User = mongoose.model('User', UserSchema);
const user = new User({ name: 'Alice', age: 25 });
user.save().then(() => console.log('User saved!'));
4. Axios
Axios is a promise-based HTTP client that simplifies making requests to external APIs.
It works both in the browser and in Node.js, providing an easy way to handle asynchronous HTTP requests.
const axios = require('axios');
axios.get('https://jsonplaceholder.typicode.com/posts')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
5. PM2
PM2 is a process manager for Node.js applications that enables you to keep your apps alive forever.
It offers features like load balancing, monitoring, and zero-downtime reloads, making it essential for production environments.
# Install PM2 globally
npm install pm2 -g
# Start your application with PM2
pm2 start app.js
# Monitor your application
pm2 monit
6. Nodemon
Nodemon is a utility that automatically restarts your Node.js application when file changes are detected in the directory.
This tool significantly enhances the development workflow by eliminating manual restarts.
# Install Nodemon globally
npm install -g nodemon
# Start your application with Nodemon
nodemon app.js
7. Passport.js
- Passport is an authentication middleware for Node.js that supports various authentication strategies (e.g., local, OAuth).
- It's modular and flexible, making it easier to implement user authentication in web applications.
const express = require('express');
const passport = require('passport');
const app = express();
app.use(passport.initialize());
passport.serializeUser((user, done) => {
done(null, user.id);
});
passport.deserializeUser((id, done) => {
// Find user by ID and return user object
});
// Define authentication strategies here...
app.listen(3000, () => {
console.log('Server running on port 3000');
});
8. Jest
Jest is a delightful JavaScript testing framework maintained by Facebook.
- It provides a simple API for writing tests and includes features like snapshot testing, making it ideal for testing React applications as well as Node.js apps.
// simple.test.js
test('adds 1 + 2 to equal 3', () => {
expect(1 + 2).toBe(3);
});
// Run tests with the command:
// jest simple.test.js
9. Lodash
- Lodash is a utility library that provides helpful functions for common programming tasks such as manipulating arrays, objects, and strings.
- Its performance optimizations make it a favorite among developers.
const _ = require('lodash');
let array = [1, 2, 3];
let doubledArray = _.map(array, num => num * 2);
console.log(doubledArray); // [2, 4, 6]
10. Bluebird
Bluebird is a fully-featured promise library that enhances JavaScript's native promise capabilities with additional features like cancellation and error handling.
It's particularly useful in complex asynchronous workflows.
const Promise = require('bluebird');
Promise.resolve(5)
.then(value => value + 1)
.then(value => console.log(value)); // Outputs: 6
Conclusion
These packages significantly enhance the capabilities of Node.js applications, allowing developers to build robust and efficient applications quickly. By leveraging these tools, you can streamline your development process and focus on creating innovative solutions. Whether you're building a simple web server or a complex real-time application, these packages are essential in the Node.js ecosystem.
Subscribe to my newsletter
Read articles from Joshua Thomas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
