Activity 27: CSS GRID
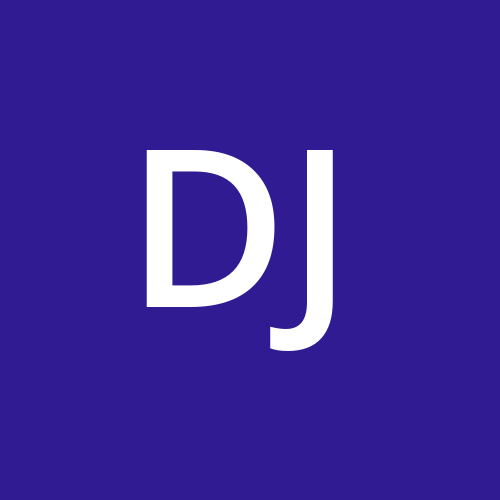
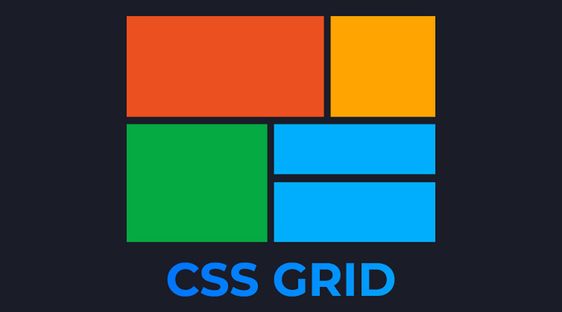
What is CSS Grid?
CSS Grid is a powerful layout system in CSS that allows developers to create complex web layouts in a more efficient and responsive manner. It provides a two-dimensional grid-based approach for arranging elements on a webpage, enabling both rows and columns to be defined.
Key Features of CSS Grid:
Two-Dimensional Layouts: Unlike Flexbox, which is primarily one-dimensional (either row or column), CSS Grid can manage both dimensions simultaneously.
Explicit Control: Developers can explicitly define the size and placement of grid items, providing precise control over the layout.
Responsive Design: CSS Grid enables responsive designs by easily adapting the layout for different screen sizes and orientations.
Importance of CSS Grid
Simplifies Complex Layouts: CSS Grid simplifies the creation of complex layouts that would be challenging to achieve with traditional methods (like floats or positioning).
Improves Maintainability: Code written with CSS Grid is often cleaner and easier to maintain, as it separates layout concerns from other styling.
Enhances Responsiveness: With features like
grid-template-areas
, developers can create responsive layouts that rearrange themselves based on screen size, providing a better user experience.Increased Flexibility: CSS Grid allows for greater flexibility in positioning items, making it easy to align elements in ways that enhance the overall design.
Using CSS Grid:
Example 1: List of Products
This example demonstrates how to create a grid layout for a list of products using CSS Grid.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>List of Products</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
}
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr); /* 3 columns */
gap: 20px; /* Space between items */
padding: 20px;
}
.grid-item {
background-color: #f8bbd0; /* Light pink */
padding: 15px;
border: 1px solid #e91e63; /* Darker pink */
}
</style>
</head>
<body>
<h1>List of Products</h1>
<div class="grid-container">
<div class="grid-item">Product 1</div>
<div class="grid-item">Product 2</div>
<div class="grid-item">Product 3</div>
<div class="grid-item">Product 4</div>
<div class="grid-item">Product 5</div>
<div class="grid-item">Product 6</div>
</div>
</body>
</html>
Grid Container: The
.grid-container
class is defined as a grid with three equal columns (repeat(3, 1fr)
).Grid Items: Each product is a grid item, styled with padding and a background color. The
gap
property creates space between the items.
OUTPUT:
Example 2: List of Employees
This example showcases a grid layout for displaying employee names.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>List of Employees</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
}
.grid-container {
display: grid;
grid-template-columns: repeat(2, 1fr);
/* 2 columns */
gap: 15px;
padding: 20px;
}
.grid-item {
background-color: #bbdefb;
/* Light blue */
padding: 15px;
border: 1px solid #2196f3;
/* Darker blue */
}
</style>
</head>
<body>
<h1>List of Employees</h1>
<div class="grid-container">
<div class="grid-item">John Doe</div>
<div class="grid-item">Jane Smith</div>
<div class="grid-item">Chris Brown</div>
<div class="grid-item">Redford White</div>
</div>
</body>
</html>
Grid Layout: The
.grid-container
is set to display as a grid with two columns. Each employee's name is contained in a grid item.Styling: Similar to the products, each grid item has a specific background color and border for visual clarity.
Certainly! Here’s a detailed overview of CSS Grid, its importance, and explanations of how to use it with examples.
CSS Grid: Overview and Importance
What is CSS Grid?
CSS Grid is a powerful layout system in CSS that allows developers to create complex web layouts in a more efficient and responsive manner. It provides a two-dimensional grid-based approach for arranging elements on a webpage, enabling both rows and columns to be defined.
Key Features of CSS Grid:
Two-Dimensional Layouts: Unlike Flexbox, which is primarily one-dimensional (either row or column), CSS Grid can manage both dimensions simultaneously.
Explicit Control: Developers can explicitly define the size and placement of grid items, providing precise control over the layout.
Responsive Design: CSS Grid enables responsive designs by easily adapting the layout for different screen sizes and orientations.
Importance of CSS Grid
Simplifies Complex Layouts: CSS Grid simplifies the creation of complex layouts that would be challenging to achieve with traditional methods (like floats or positioning).
Improves Maintainability: Code written with CSS Grid is often cleaner and easier to maintain, as it separates layout concerns from other styling.
Enhances Responsiveness: With features like
grid-template-areas
, developers can create responsive layouts that rearrange themselves based on screen size, providing a better user experience.Increased Flexibility: CSS Grid allows for greater flexibility in positioning items, making it easy to align elements in ways that enhance the overall design.
Using CSS Grid: Step-by-Step Examples
Here are some practical examples of how to implement CSS Grid for different types of lists.
Example 1: List of Products
This example demonstrates how to create a grid layout for a list of products using CSS Grid.
HTML Code
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>List of Products</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
}
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr); /* 3 columns */
gap: 20px; /* Space between items */
padding: 20px;
}
.grid-item {
background-color: #f8bbd0; /* Light pink */
padding: 15px;
border: 1px solid #e91e63; /* Darker pink */
}
</style>
</head>
<body>
<h1>List of Products</h1>
<div class="grid-container">
<div class="grid-item">Product 1</div>
<div class="grid-item">Product 2</div>
<div class="grid-item">Product 3</div>
<div class="grid-item">Product 4</div>
<div class="grid-item">Product 5</div>
<div class="grid-item">Product 6</div>
</div>
</body>
</html>
Explanation
Grid Container: The
.grid-container
class is defined as a grid with three equal columns (repeat(3, 1fr)
).Grid Items: Each product is a grid item, styled with padding and a background color. The
gap
property creates space between the items.
Example 2: List of Employees
This example showcases a grid layout for displaying employee names.
HTML Code
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>List of Employees</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
}
.grid-container {
display: grid;
grid-template-columns: repeat(2, 1fr); /* 2 columns */
gap: 15px;
padding: 20px;
}
.grid-item {
background-color: #bbdefb; /* Light blue */
padding: 15px;
border: 1px solid #2196f3; /* Darker blue */
}
</style>
</head>
<body>
<h1>List of Employees</h1>
<div class="grid-container">
<div class="grid-item">Employee 1</div>
<div class="grid-item">Employee 2</div>
<div class="grid-item">Employee 3</div>
<div class="grid-item">Employee 4</div>
</div>
</body>
</html>
Explanation
Grid Layout: The
.grid-container
is set to display as a grid with two columns. Each employee's name is contained in a grid item.Styling: Similar to the products, each grid item has a specific background color and border for visual clarity.
Example 3: List of Students
In this example, we will create a grid layout to display a list of student names.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>List of Students</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
}
.grid-container {
display: grid;
grid-template-columns: repeat(4, 1fr);
/* 4 columns */
gap: 15px;
padding: 20px;
}
.grid-item {
background-color: #c8e6c9;
/* Light green */
padding: 15px;
border: 1px solid #4caf50;
/* Darker green */
}
</style>
</head>
<body>
<h1>List of Students</h1>
<div class="grid-container">
<div class="grid-item">Alice Guo</div>
<div class="grid-item">Bob Smith</div>
<div class="grid-item">Charlie Put</div>
<div class="grid-item">David Cook</div>
<div class="grid-item">Eve Born</div>
<div class="grid-item">Frank Chavez</div>
<div class="grid-item">Grace Poe</div>
<div class="grid-item">Willie Ong</div>
</div>
</body>
</html>
Grid Layout: The
.grid-container
features four columns to accommodate more student names. The gap between items enhances visual spacing.Styling: Each student name is visually separated with a light green background and a solid green border.
OUTPUT:
Example 4: List of Books
This example demonstrates how to create a grid layout for displaying a list of books.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>List of Books</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
}
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr);
/* 3 columns */
gap: 20px;
/* Space between items */
padding: 20px;
}
.grid-item {
background-color: #ffe0b2;
/* Light orange */
padding: 15px;
border: 1px solid #ff9800;
/* Darker orange */
}
</style>
</head>
<body>
<h1>List of Books</h1>
<div class="grid-container">
<div class="grid-item">The Great Gatsby</div>
<div class="grid-item">1984</div>
<div class="grid-item">Moby Dick</div>
<div class="grid-item">To Kil a Mockingbird</div>
<div class="grid-item">War an Peace</div>
<div class="grid-item">Pride and Prejudice</div>
</div>
</body>
</html>
Grid Layout: The
.grid-container
is set to display as a grid with three columns, accommodating six book items.Styling: Each book item has a light orange background and a solid orange border, enhancing visibility and aesthetics.
OUPUT:
Example 5: List of Courses
This example demonstrates how to create a grid layout for a list of courses.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>List of Courses</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
}
.grid-container {
display: grid;
grid-template-columns: repeat(2, 1fr);
/* 2 columns */
gap: 15px;
/* Space between items */
padding: 20px;
}
.grid-item {
background-color: #d1c4e9;
/* Light purple */
padding: 15px;
border: 1px solid #673ab7;
/* Darker purple */
}
</style>
</head>
<body>
<h1>List of Courses</h1>
<div class="grid-container">
<div class="grid-item">Mathematics</div>
<div class="grid-item">Science</div>
<div class="grid-item">History</div>
<div class="grid-item">Literature</div>
<div class="grid-item">Computer Science</div>
<div class="grid-item">Art Hhistory</div>
</div>
</body>
</html>
Explanation
Grid Layout: The
.grid-container
is set to display as a grid with two columns, accommodating six course items.Styling: Each course item is styled with a light purple background and a solid darker purple border, creating a visually appealing layout.
OUTPUT:
Conclusion
These examples illustrate how to effectively use CSS Grid to create organized and responsive layouts for different types of lists. By employing grid properties such as grid-template-columns
and gap
, developers can create clean, structured, and visually appealing layouts that adapt to various screen sizes. CSS Grid enhances both the aesthetic and functional aspects of web design, making it an essential tool for modern web development.
GITHUB Repository Link: CSS_GRID
Subscribe to my newsletter
Read articles from Danilo Buenafe Jr directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
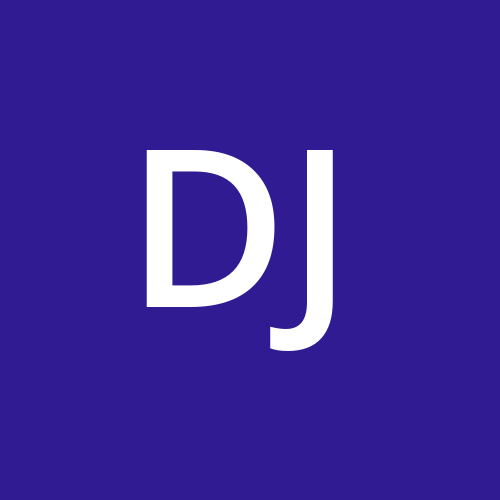