"Mastering RobotJS : A Beginner's Guide to Automating Desktop Interactions with JavaScript"

Table of contents
- Introduction to RobotJS
- What is RobotJS?
- Key Features of RobotJS
- Specifications of RobotJS
- Use Cases of RobotJS
- Pros of RobotJS
- Cons of RobotJS
- Alternatives to RobotJS
- 1. What You Need
- 2. Setting Up Electron
- 3. Installing RobotJS
- 4. Using RobotJS in Electron
- 5. More RobotJS Functions
- 6. Summary of Steps
- Conclusion
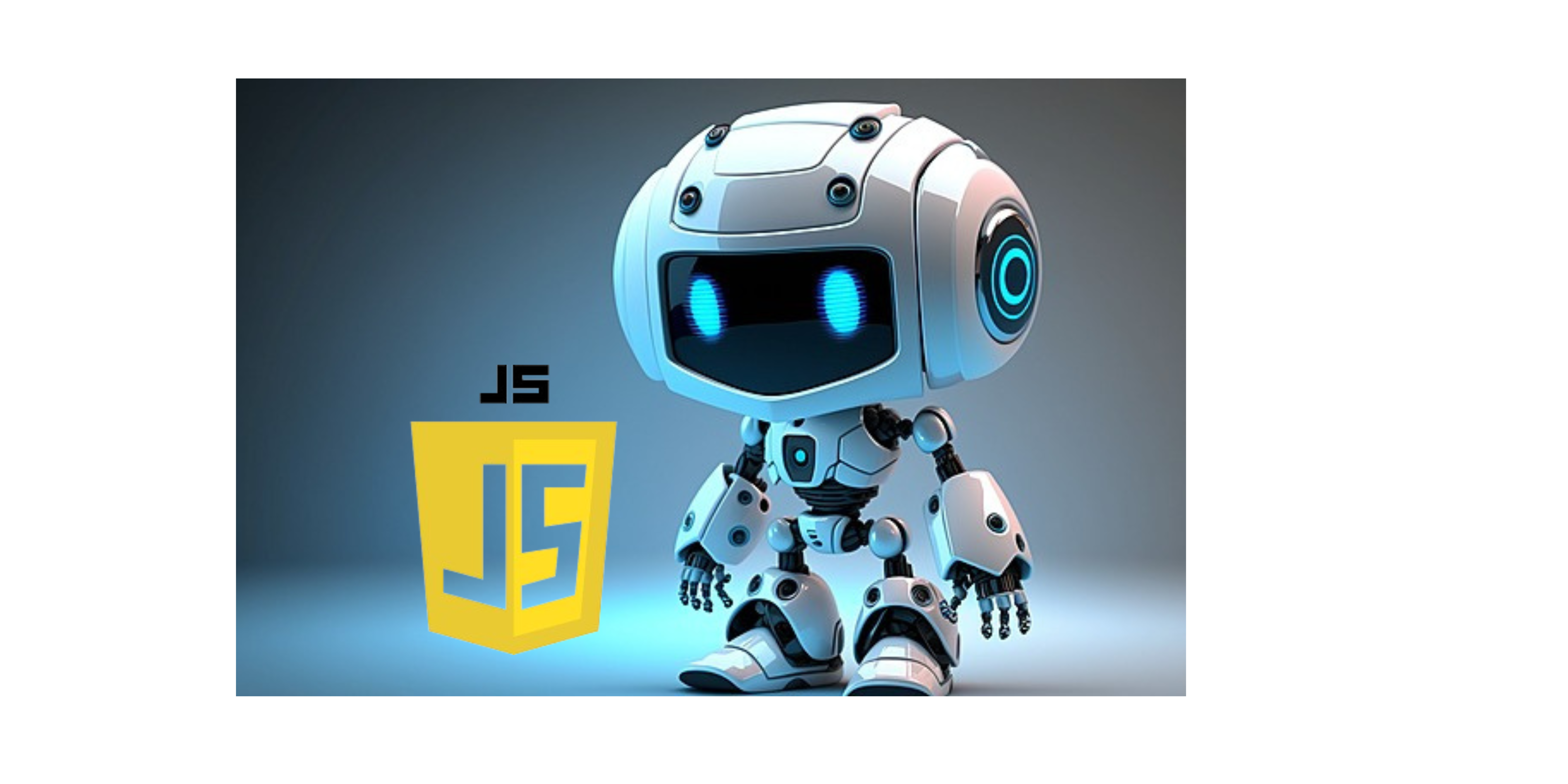
Introduction to RobotJS
RobotJS is an open-source Node.js library designed to automate desktop applications. It allows developers to simulate human interactions with a computer, such as keyboard inputs, mouse movements, and clicks. This makes it incredibly useful for automating repetitive tasks, testing desktop applications, or creating bots. Unlike other automation tools that require external dependencies or complex setups, RobotJS is lightweight, simple to use, and works cross-platform.
What is RobotJS?
RobotJS is a desktop automation library that gives you programmatic control over your system's keyboard and mouse. It enables developers to simulate human-like interactions with the operating system through JavaScript. It works by accessing system-level APIs to control input devices and automate tasks that would otherwise require manual intervention.
Key Features of RobotJS
Keyboard Automation: You can simulate typing keys, pressing shortcuts, or sending special character inputs.
Mouse Automation: RobotJS allows precise control of the mouse, including movement, clicks, and scrolls.
Cross-Platform Compatibility: It works on all major operating systems—Windows, macOS, and Linux.
Simple API: RobotJS has an easy-to-understand API that allows developers to quickly write scripts for automation.
No Dependencies: Unlike many automation tools, RobotJS does not rely on complex dependencies or GUI automation frameworks. It’s lightweight and efficient.
Specifications of RobotJS
Platform Support: Windows, macOS, and Linux.
Language: JavaScript (Node.js).
Dependencies: None—RobotJS is a standalone library, making it efficient and easy to integrate.
Input Automation: Supports keyboard and mouse control.
Additional Features: Ability to take screenshots and detect pixel color values on the screen.
Use Cases of RobotJS
Automating Repetitive Tasks: If you need to perform the same set of actions repeatedly on your computer (e.g., form submissions, image processing), RobotJS can help automate these tasks.
Desktop Application Testing: RobotJS is useful for automated testing of desktop applications, simulating user input to test how the app responds.
Bot Creation: RobotJS can be used to create bots for specific tasks, like navigating through menus or playing simple games.
Custom Scripts for Personal Use: Many users employ RobotJS for personal automation, such as launching applications, managing windows, or even controlling hardware components.
Screenshots and Pixel Detection: RobotJS can also be used for image recognition tasks by taking screenshots and analyzing specific pixel values.
Pros of RobotJS
Lightweight and Fast: RobotJS is lightweight with no dependencies, making it quick and easy to integrate into projects.
Cross-Platform Support: It runs on all major operating systems, allowing you to write a script once and run it anywhere.
Simple API: The library's API is straightforward, which lowers the barrier to entry for developers.
Ideal for Small Automation Tasks: It’s perfect for simple automation tasks without the need for heavyweight frameworks or additional software.
Supports Modern JS Syntax: Being a Node.js library, RobotJS allows you to write scripts using modern JavaScript features.
Cons of RobotJS
Limited Capabilities: While RobotJS is great for basic automation, it lacks advanced features like window or element manipulation that other tools might offer.
No GUI Automation: It cannot interact with specific UI elements (e.g., buttons, text boxes) by identifying them, which limits its usability in more complex automation scenarios.
Performance Overhead: Since it is built on top of Node.js, some tasks may not be as fast as low-level automation tools or system-native APIs.
Development is Slow: The development of RobotJS has slowed down in recent years, so it may not have the latest features compared to other automation libraries.
Alternatives to RobotJS
While RobotJS is excellent for small-scale automation, there are several alternatives available, depending on your needs:
AutoHotKey (AHK): A powerful scripting language for Windows automation. Unlike RobotJS, AutoHotKey can interact with UI elements, perform window manipulation, and automate more complex workflows.
SikuliX: A visual automation tool that uses image recognition to automate tasks. It allows you to automate anything you see on your screen, but it’s heavier than RobotJS.
Puppeteer: Though Puppeteer focuses on browser automation, it can also be used for some desktop tasks, such as capturing screenshots and automating browsers.
PyAutoGUI: A Python library similar to RobotJS, PyAutoGUI automates the keyboard and mouse. It also supports screenshots and can identify UI elements through pixel matching.
Electron.js with Native APIs: For advanced desktop application automation, using Electron.js with native OS APIs (like Win32 on Windows) might be more powerful than RobotJS.
1. What You Need
Before you begin, make sure you have the following:
Node.js installed on your computer.
Electron installed and a basic Electron project set up.
RobotJS installed in your Electron project.
If you don’t have a basic Electron project yet, we’ll start by creating one from scratch.
2. Setting Up Electron
Step 1: Install Electron
If you haven’t installed Electron yet, follow these steps:
Create a new folder for your project. Open your terminal (Command Prompt, PowerShell, etc.) and type:
mkdir my-electron-app cd my-electron-app
Initialize a new Node.js project:
npm init -y
Install Electron:
npm install electron --save-dev
Step 2: Create Electron Files
Inside the
my-electron-app
folder, create two files:main.js (for Electron’s main process)
index.html (for the front-end user interface)
Open main.js and add this code:
const { app, BrowserWindow } = require('electron'); function createWindow() { const win = new BrowserWindow({ width: 800, height: 600, webPreferences: { nodeIntegration: true, contextIsolation: false, } }); win.loadFile('index.html'); } app.whenReady().then(createWindow);
Create a simple index.html file:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>My Electron App</title> </head> <body> <h1>Hello, Electron!</h1> <button id="clickMeBtn">Click Me</button> <script src="renderer.js"></script> </body> </html>
Create a renderer.js file:
- This is where we will later use RobotJS to control the mouse or keyboard from Electron.
3. Installing RobotJS
Now that we have an Electron app, let’s install RobotJS.
In your terminal, inside the project folder, run:
npm install robotjs
4. Using RobotJS in Electron
Let’s now integrate RobotJS into our Electron project to control the mouse or keyboard.
Step 1: Modify renderer.js to Use RobotJS
RobotJS can automate tasks like clicking a button or moving the mouse. We will add this functionality to our renderer.js file.
Open renderer.js and add this code:
const robot = require('robotjs'); // Example: Move the mouse to a specific location when a button is clicked document.getElementById('clickMeBtn').addEventListener('click', () => { // Get the screen size to avoid clicking outside the window const screenSize = robot.getScreenSize(); const x = screenSize.width / 2; // Move to the middle of the screen (x-axis) const y = screenSize.height / 2; // Move to the middle of the screen (y-axis) robot.moveMouse(x, y); // Move the mouse to the center of the screen robot.mouseClick(); // Perform a left-click alert('Mouse moved and clicked at the center of the screen!'); });
Here’s what happens:
- When you click the button on your Electron app, RobotJS moves the mouse to the center of the screen and performs a left-click.
Step 2: Run Your Electron App
Modify your
package.json
file to add a start script:"scripts": { "start": "electron ." }
Now, to run your app, type:
npm start
Your Electron app should open. When you click the "Click Me" button, the mouse will move to the center of the screen, and a click will happen automatically.
5. More RobotJS Functions
RobotJS can do much more than just move the mouse. Here are some additional examples you can try in your renderer.js file:
Example 1: Type Text Automatically
document.getElementById('clickMeBtn').addEventListener('click', () => {
robot.typeString('Hello from RobotJS!');
alert('Typed "Hello from RobotJS!"');
});
Example 2: Detect Pixel Color
You can detect the color of a pixel at a specific location.
document.getElementById('clickMeBtn').addEventListener('click', () => {
const mouse = robot.getMousePos();
const color = robot.getPixelColor(mouse.x, mouse.y);
alert(`Mouse is at (${mouse.x}, ${mouse.y}) and the color is #${color}`);
});
6. Summary of Steps
Step 1: Set up a basic Electron project.
Step 2: Install RobotJS via
npm install robotjs
.Step 3: Use RobotJS in the renderer process to simulate mouse movements, clicks, or keyboard inputs.
Step 4: Run your app using
npm start
and see RobotJS in action.
Conclusion
Using RobotJS with Electron allows you to build powerful desktop automation apps in JavaScript. You can automate tasks, control the keyboard and mouse, and even use advanced features like pixel color detection. With its simple API and cross-platform support, RobotJS makes it easy to create engaging and efficient desktop automation tools.
This step-by-step guide should help you get started with using RobotJS in an Electron environment. Feel free to explore more RobotJS features and enhance your Electron app!
Subscribe to my newsletter
Read articles from Payal Porwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Payal Porwal
Payal Porwal
Hi there, tech enthusiasts! I'm a passionate Software Developer driven by a love for continuous learning and innovation. I thrive on exploring new tools and technologies, pushing boundaries, and finding creative solutions to complex problems. What You'll Find Here On my Hashnode blog, I share: 🚀 In-depth explorations of emerging technologies 💡 Practical tutorials and how-to guides 🔧Insights on software development best practices 🚀Reviews of the latest tools and frameworks 💡 Personal experiences from real-world projects. Join me as we bridge imagination and implementation in the tech world. Whether you're a seasoned pro or just starting out, there's always something new to discover! Let’s connect and grow together! 🌟