Activity 29: HTTP Methods
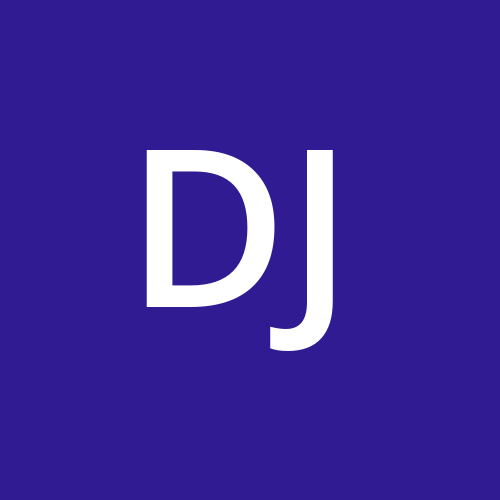
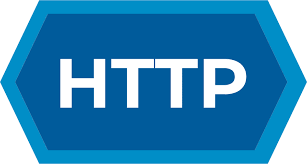
What is an HTTP Method?
HTTP methods are a set of request methods used in the Hypertext Transfer Protocol (HTTP) to indicate the desired action to be performed on a specific resource on a web server. Each method serves a different purpose and helps define the nature of the request.
Common Uses of HTTP Methods
HTTP methods are primarily used in web development and APIs to facilitate communication between clients (like web browsers or mobile apps) and servers. They allow clients to interact with resources (data, services) on the server effectively. Here's a breakdown of their uses:
GET: Retrieve data or resources from the server.
POST: Send data to the server to create new resources.
PUT: Update existing resources on the server with new data.
DELETE: Remove resources from the server.
PATCH: Apply partial updates to existing resources.
Importance of HTTP Methods
Standardization: HTTP methods provide a standardized way for clients and servers to communicate. This standardization allows for interoperability between different systems and platforms.
Clarity of Intent: Each method conveys a clear intent. For example, a GET request indicates that the client wants to retrieve data, while a POST request indicates that the client is submitting data. This clarity helps in building APIs that are easy to understand and use.
Separation of Concerns: By using different methods for different actions (e.g., reading, creating, updating, and deleting), developers can create cleaner and more maintainable code. This separation of concerns allows for better organization and structuring of applications.
RESTful Architecture: HTTP methods are fundamental to REST (Representational State Transfer) architecture. RESTful APIs leverage these methods to provide a stateless, scalable, and easy-to-use web service design.
Security: Different methods can have different security implications. For instance, GET requests can often be cached and are typically less secure for sensitive data, while POST requests can include sensitive information in the body and are not cached by default. Understanding this helps developers implement appropriate security measures.
Resource Management: By using the appropriate HTTP methods, developers can effectively manage resources on a server, ensuring that they can create, read, update, or delete resources as needed.
Sample Codes:
1. GET
Retrieve a list of users:
// src/index.ts
import express, { Request, Response } from 'express';
const app = express();
app.use(express.json());
interface User {
id: number;
name: string;
}
const users: User[] = [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Doe' },
];
// GET endpoint to retrieve all users
app.get('/users', (req: Request, res: Response) => {
res.json(users);
});
// GET endpoint to retrieve a user by ID
app.get('/users/:id', (req: Request, res: Response) => {
const userId = parseInt(req.params.id);
const user = users.find(u => u.id === userId);
if (user) {
res.json(user);
} else {
res.status(404).send('User not found');
}
});
2. POST
Create a new user:
// POST endpoint to create a new user
app.post('/users', (req: Request, res: Response) => {
const newUser: User = req.body;
users.push(newUser);
res.status(201).json(newUser);
});
3. PUT
Update an existing user:
// PUT endpoint to update an existing user
app.put('/users/:id', (req: Request, res: Response) => {
const userId = parseInt(req.params.id);
const userIndex = users.findIndex(u => u.id === userId);
if (userIndex !== -1) {
const updatedUser: User = { ...users[userIndex], ...req.body };
users[userIndex] = updatedUser;
res.json(updatedUser);
} else {
res.status(404).send('User not found');
}
});
4. DELETE
Remove a user:
// PATCH endpoint to partially update an existing user
app.patch('/users/:id', (req: Request, res: Response) => {
const userId = parseInt(req.params.id);
const userIndex = users.findIndex(u => u.id === userId);
if (userIndex !== -1) {
const updatedUser: User = { ...users[userIndex], ...req.body };
users[userIndex] = updatedUser;
res.json(updatedUser);
} else {
res.status(404).send('User not found');
}
});
5. PATCH
Partially update a user:
// PATCH endpoint to partially update an existing user
app.patch('/users/:id', (req: Request, res: Response) => {
const userId = parseInt(req.params.id);
const userIndex = users.findIndex(u => u.id === userId);
if (userIndex !== -1) {
const updatedUser: User = { ...users[userIndex], ...req.body };
users[userIndex] = updatedUser;
res.json(updatedUser);
} else {
res.status(404).send('User not found');
}
});
Type Safety: Using interfaces like
User
ensures that the data structure remains consistent, reducing runtime errors.Express Request and Response Types: TypeScript allows you to specify types for the request and response objects, which helps with IntelliSense and type checking.
JSON Middleware: The
express.json()
middleware is used to parse incoming JSON requests.
References:
HTTP request methods
What are HTTP methods?
How to exploit HTTP Methods
Subscribe to my newsletter
Read articles from Danilo Buenafe Jr directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
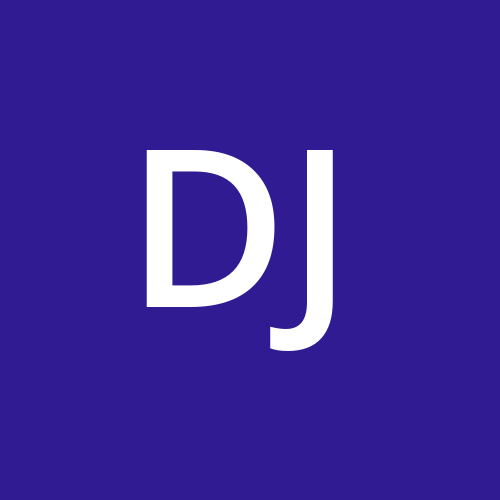