Activity 30: HTTP Status Codes
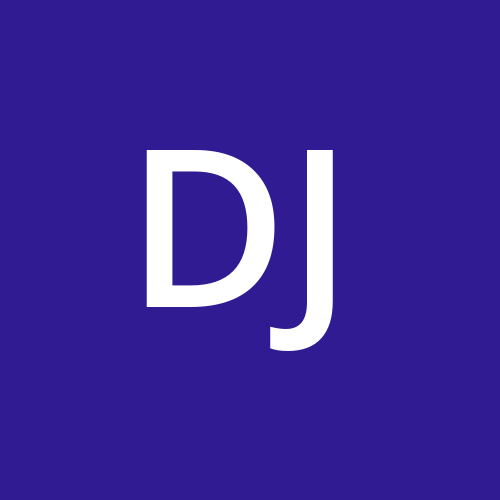
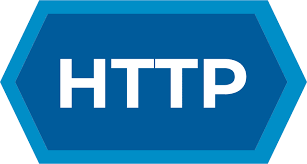
Understanding HTTP status codes is crucial for developing and interacting with RESTful APIs, as they communicate the outcome of an API request. Here's an overview of the various categories of HTTP status codes and their significance:
1xx (Informational)
These codes indicate that the server has received the request and is processing it, but there is no final response yet. They are mostly used in scenarios where the client needs to wait for further information.
- Example:
100 Continue
- This status indicates that the initial part of a request has been received, and the client can continue sending the request body.
HTTP/1.1 100 Continue
2xx (Success)
These codes confirm that the client's request was successfully received, understood, and accepted. They are critical for indicating that the requested action has been completed.
- 200 OK: This is the most common status code, indicating that the request was successful and the server has returned the requested data.
HTTP/1.1 200 OK
Content-Type: application/json
{
"message": "Request was successful",
"data": { "id": 1, "name": "John Doe" }
}
- 201 Created: This code indicates that a new resource was successfully created as a result of the request. It’s commonly used in POST requests.
HTTP/1.1 201 Created
Location: /users/1
Content-Type: application/json
{
"message": "User created successfully",
"data": { "id": 1, "name": "John Doe" }
}
- 204 No Content: This status indicates that the request was successful, but there is no content to return in the response. It is often used for DELETE requests.
HTTP/1.1 204 No Content
3xx (Redirection)
These codes indicate that the client must take additional actions to complete the request. This usually involves redirecting to a different URL.
- 301 Moved Permanently: This status code indicates that the resource has been permanently moved to a new URL. Clients should use the new URL in future requests.
HTTP/1.1 301 Moved Permanently
Location: https://www.new-url.com
- 302 Found: This status indicates that the resource is temporarily located at a different URL. The client should continue to use the original URL for future requests.
HTTP/1.1 302 Found
Location: https://www.temporary-url.com
4xx (Client Error)
These codes indicate that there was an error with the request from the client’s side. They provide feedback to the client about what went wrong.
- 400 Bad Request: This status indicates that the server cannot process the request due to invalid syntax. The client should modify the request and try again.
HTTP/1.1 400 Bad Request
Content-Type: application/json
{
"error": "Invalid request format"
}
- 401 Unauthorized: This status indicates that authentication is required and has failed or not been provided. The client must authenticate to access the requested resource.
HTTP/1.1 401 Unauthorized
Content-Type: application/json
{
"error": "Authentication required"
}
- 403 Forbidden: This status indicates that the server understood the request, but it refuses to authorize it. The client does not have permission to access the resource.
HTTP/1.1 403 Forbidden
Content-Type: application/json
{
"error": "You do not have permission to access this resource"
}
- 404 Not Found: This status indicates that the requested resource could not be found on the server. It’s a common response when an API endpoint does not exist.
HTTP/1.1 404 Not Found
Content-Type: application/json
{
"error": "Resource not found"
}
5xx (Server Error)
These codes indicate that the server failed to fulfill a valid request due to an error on the server side. They signal to the client that something went wrong beyond their control.
- 500 Internal Server Error: This status indicates that the server encountered an unexpected condition that prevented it from fulfilling the request. It’s a generic error message.
HTTP/1.1 500 Internal Server Error
Content-Type: application/json
{
"error": "An unexpected error occurred"
}
- 503 Service Unavailable: This status indicates that the server is temporarily unable to handle the request, often due to overload or maintenance. The client should retry later.
HTTP/1.1 503 Service Unavailable
Content-Type: application/json
{
"error": "Service temporarily unavailable. Please try again later."
}
Importance of HTTP Status Codes
Communication: Status codes serve as a language for the client and server to communicate effectively about the success or failure of a request.
Error Handling: They help in identifying issues, allowing clients to implement appropriate error handling and recovery mechanisms.
Client Experience: Properly handling status codes can lead to better user experiences, guiding users through successful and unsuccessful interactions with the API.
Summary
HTTP status codes are essential for understanding the outcomes of API requests in RESTful services. They provide a standardized way for servers to convey the result of a request, helping clients understand what happened and what actions, if any, they need to take next.
References:
HTTP response status codes
HTTP Status Codes
HTTP Status Messages
Subscribe to my newsletter
Read articles from Danilo Buenafe Jr directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
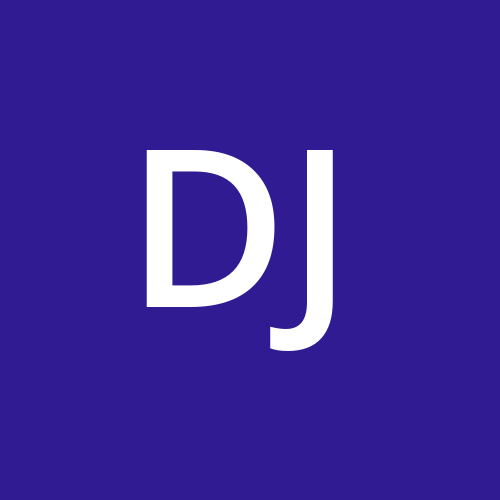