Introduction to Binary Search Trees (BST) [2024] Guide | Day #13
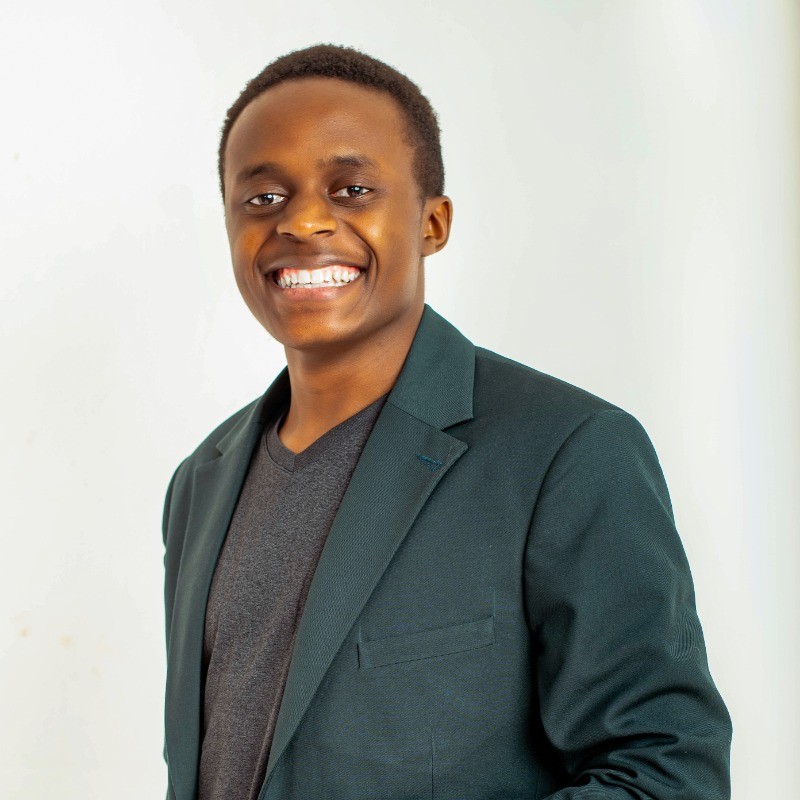
Table of contents
- What is a Binary Search Tree (BST)?
- Properties of Binary Search Trees
- Why are BSTs Essential?
- Basic Operations in BST with Code Examples
- Binary Tree Traversal Techniques
- Visualizing BST Operations and Traversal Paths
- Time and Space Complexity Analysis
- Comparison of BST with Other Data Structures
- Real-World Applications of Binary Search Trees
- Frequently Asked Questions (FAQ)
- Conclusion & Next Steps
- Related Resources, Advanced BST Topics & Extensions
- RB Tree
- AVL Tree
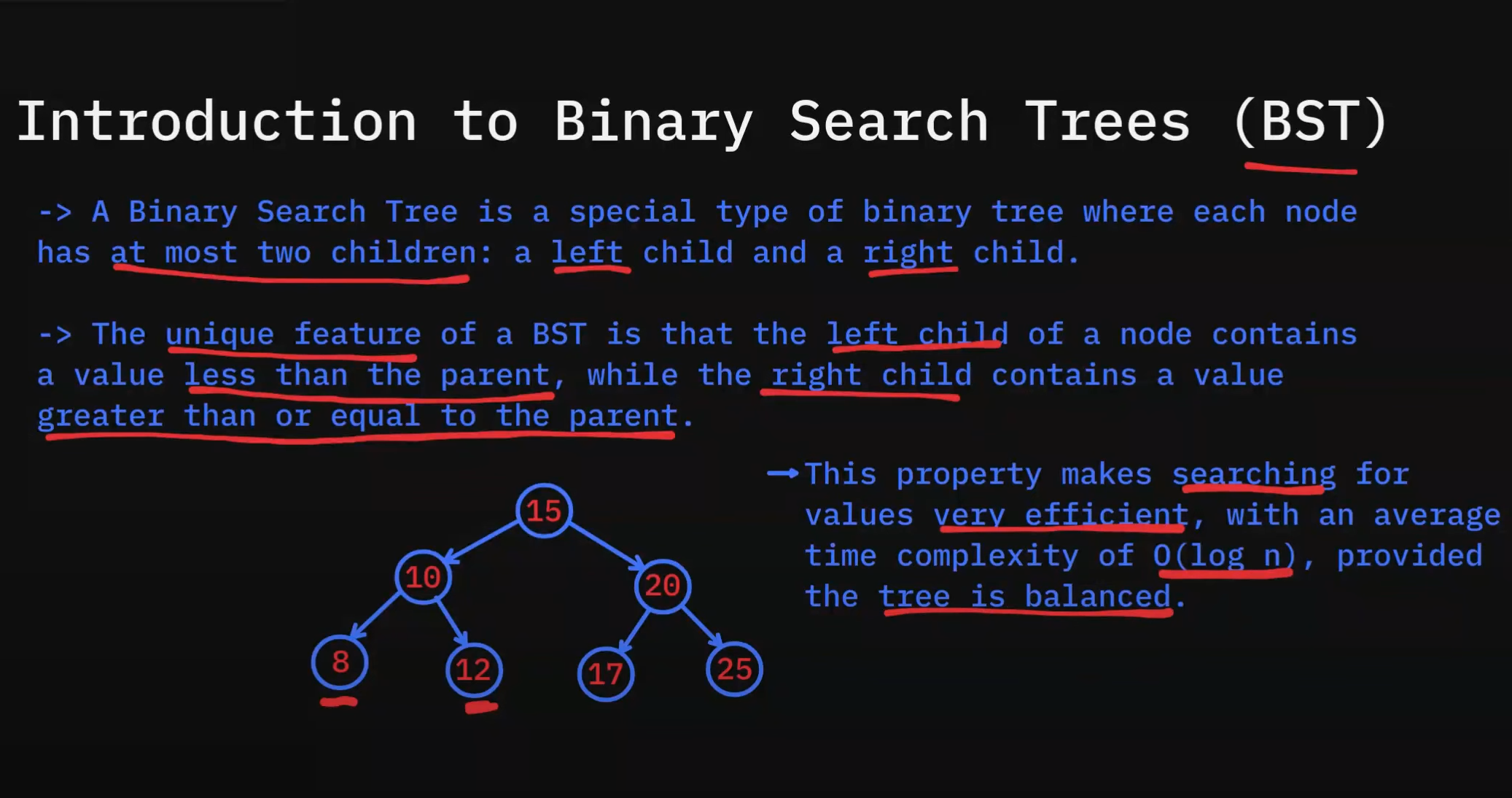
Binary Search Trees (BST) are one of the most fundamental data structures in computer science, widely used for organizing and retrieving data efficiently. In this guide, we will explore what a BST is, its properties, and how it supports operations like insertion, searching, and deletion. With Python and JavaScript code examples, visual diagrams, and a discussion of real-world applications, this article offers a practical introduction to BSTs.
What is a Binary Search Tree (BST)?
A Binary Search Tree (BST) is a type of binary tree where each node follows a specific ordering property:
The left child of a node contains a value less than the node’s value.
The right child of a node contains a value greater than or equal to the node’s value.
This ordering property ensures efficient searching, insertion, and deletion, making BSTs useful for tasks that require fast data lookups and modifications.
Properties of Binary Search Trees
Binary Structure: Each node has at most two children.
Ordering Property: Values in the left subtree are smaller, and values in the right subtree are larger or equal.
No Duplicate Nodes (in most implementations).
Height-Sensitive Performance: The efficiency of BST operations depends on the height of the tree.
Why are BSTs Essential?
Binary Search Trees offer an optimal way to organize and access hierarchical data efficiently, with average-case time complexities of O(log n) for insertion, search, and deletion operations.
Basic Operations in BST with Code Examples
1. Insertion Operation
The insertion process follows the BST property:
If the value is smaller, it goes to the left child.
If the value is larger or equal, it goes to the right child.
Python Code for Insertion:
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def insert(root, value):
if root is None:
return Node(value)
if value < root.value:
root.left = insert(root.left, value)
else:
root.right = insert(root.right, value)
return root
JavaScript Code for Insertion:
class Node {
constructor(value) {
this.value = value;
this.left = null;
this.right = null;
}
}
function insert(root, value) {
if (root === null) return new Node(value);
if (value < root.value) {
root.left = insert(root.left, value);
} else {
root.right = insert(root.right, value);
}
return root;
}
2. Search Operation
To search for a value in a BST, we recursively compare it with the node’s value and move left or right based on the comparison.
Python Code for Search:
def search(root, value):
if root is None or root.value == value:
return root
if value < root.value:
return search(root.left, value)
return search(root.right, value)
JavaScript Code for Search:
function search(root, value) {
if (root === null || root.value === value) return root;
if (value < root.value) return search(root.left, value);
return search(root.right, value);
}
3. Deletion Operation
Deleting a node requires handling three cases:
The node has no children (leaf node).
The node has one child.
The node has two children (replace the node with its in-order successor).
Python Code for Deletion:
def delete(root, value):
if root is None:
return root
if value < root.value:
root.left = delete(root.left, value)
elif value > root.value:
root.right = delete(root.right, value)
else:
if root.left is None:
return root.right
elif root.right is None:
return root.left
temp = find_min(root.right)
root.value = temp.value
root.right = delete(root.right, temp.value)
return root
def find_min(node):
while node.left:
node = node.left
return node
Binary Tree Traversal Techniques
Traversal techniques help us visit each node systematically.
Inorder Traversal (Left → Root → Right)
- Visits nodes in ascending order for a BST.
Preorder Traversal (Root → Left → Right)
- Used for creating a copy of the tree.
Postorder Traversal (Left → Right → Root)
- Useful for deleting a tree from leaf nodes to root.
Visualizing BST Operations and Traversal Paths
Time and Space Complexity Analysis
Insertion: Average case: O(log n), Worst case: O(n)
Search: Average case: O(log n), Worst case: O(n)
Deletion: Average case: O(log n), Worst case: O(n)
Space Complexity: O(n)
Comparison of BST with Other Data Structures
Feature | BST | Array | Linked List |
Search Time | O(log n) | O(n) | O(n) |
Insertion Time | O(log n) | O(n) | O(1) |
Deletion Time | O(log n) | O(n) | O(1) |
Real-World Applications of Binary Search Trees
Database Indexing: BSTs help maintain indexes for fast lookups.
Auto-Complete Systems: Used to store and search dictionary words efficiently.
File Searching Algorithms: BSTs optimize hierarchical data searching.
Frequently Asked Questions (FAQ)
What makes a tree a Binary Search Tree?
A BST has an ordering property where left child nodes are smaller, and right child nodes are greater or equal to their parent nodes.
How does a BST ensure efficient searching?
By narrowing down the search space with each comparison, the time complexity is reduced to O(log n) on average.
What's the difference between a binary tree and a binary search tree?
A binary search tree follows a specific ordering property where left children are smaller and right children are larger than their parent, while a binary tree has no such ordering requirement.
When should I use a BST instead of an array?
Use a BST when you need efficient searching, insertion, and deletion operations with O(log n) average time complexity, especially for large datasets with frequent modifications.
How can I optimize BST performance?
Keep the tree balanced
Use appropriate self-balancing variants
Implement efficient traversal methods
Consider caching frequently accessed nodes
Conclusion & Next Steps
Binary Search Trees (BSTs) are a vital data structure for fast data lookups and modifications. With their hierarchical structure and ordering properties, BSTs find extensive use in databases, search engines, and routing algorithms. Practice the examples in this guide to deepen your understanding of BST operations.
To master BSTs:
Practice implementing basic operations
Study self-balancing variants
Solve related coding problems
Explore real-world applications
Stay updated with our latest data structure tutorials and coding guides by following our blog.
Related Resources, Advanced BST Topics & Extensions
Binary Tree Traversal Techniques
RB Tree
AVL Tree
Subscribe to my newsletter
Read articles from Bonaventure Ogeto directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
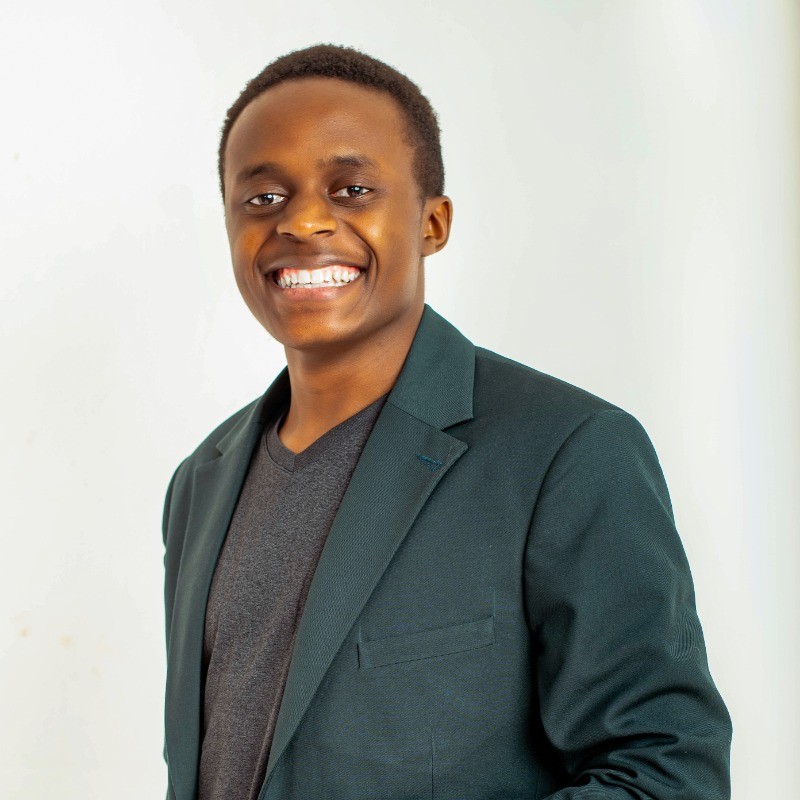
Bonaventure Ogeto
Bonaventure Ogeto
Software Engineer & Technical Writer