Step-by-Step Guide to System Monitoring with Flask and UI Display
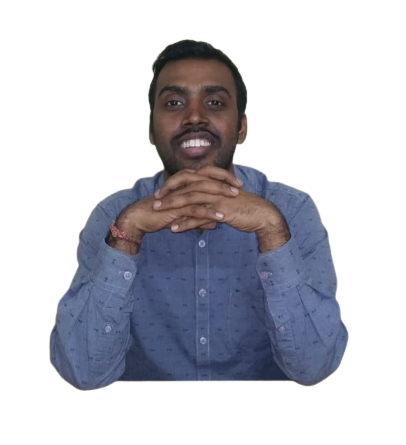
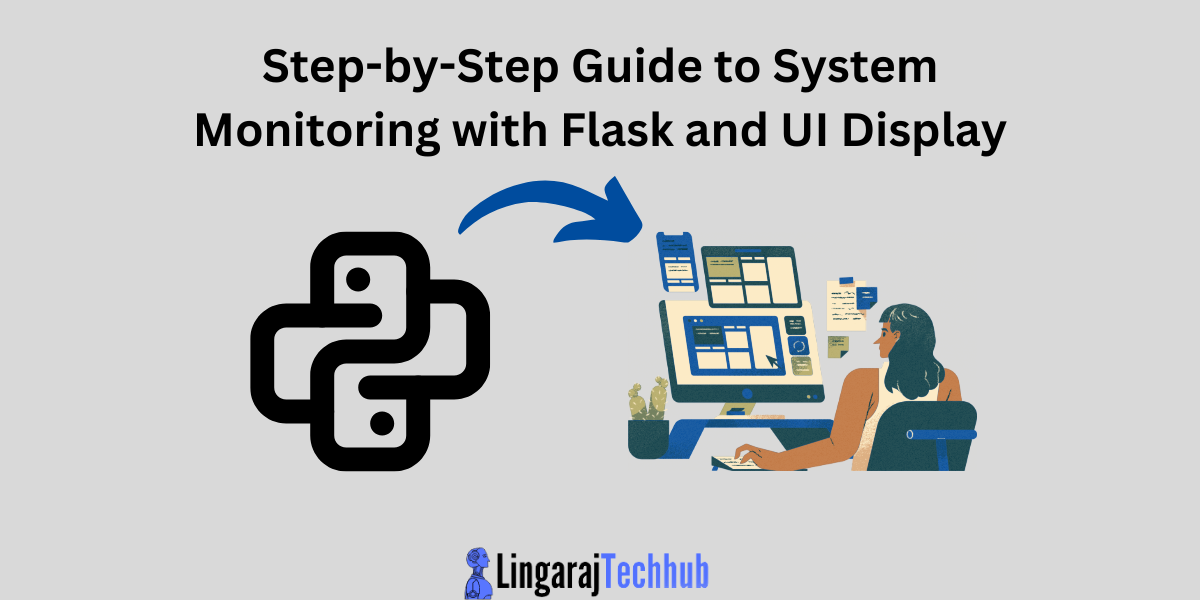
Here is an improved version of the Flask app that displays system monitoring data using the Plotly
JavaScript library for interactive charts. The app will have real-time updating graphs for CPU usage, memory usage, disk usage, and network activity.
Flask Application with Graphical Monitoring:
from flask import Flask, render_template, jsonify
import psutil
import time
app = Flask(__name__)
def get_system_stats():
stats = {
"cpu_usage": psutil.cpu_percent(interval=1),
"memory_usage": psutil.virtual_memory().percent,
"disk_usage": psutil.disk_usage('/').percent,
"bytes_sent": psutil.net_io_counters().bytes_sent / (1024 * 1024), # MB
"bytes_recv": psutil.net_io_counters().bytes_recv / (1024 * 1024) # MB
}
return stats
@app.route('/')
def index():
return render_template('index.html')
@app.route('/system_stats')
def system_stats():
stats = get_system_stats()
return jsonify(stats)
if __name__ == "__main__":
app.run(debug=True)
HTML Template with Plotly:
Save this file as index.html
in the templates
folder:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>System Monitoring Dashboard</title>
<script src="https://cdn.plot.ly/plotly-latest.min.js"></script>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
h1 {
color: #333;
}
.chart {
width: 100%;
height: 400px;
margin-bottom: 40px;
}
</style>
</head>
<body>
<h1>System Monitoring Dashboard</h1>
<div id="cpuChart" class="chart"></div>
<div id="memoryChart" class="chart"></div>
<div id="diskChart" class="chart"></div>
<div id="networkChart" class="chart"></div>
<script>
var cpuData = [{
y: [0],
type: 'line',
name: 'CPU Usage (%)'
}];
var memoryData = [{
y: [0],
type: 'line',
name: 'Memory Usage (%)'
}];
var diskData = [{
y: [0],
type: 'line',
name: 'Disk Usage (%)'
}];
var networkData = [{
y: [0],
type: 'line',
name: 'Network Sent (MB)'
}, {
y: [0],
type: 'line',
name: 'Network Received (MB)'
}];
Plotly.newPlot('cpuChart', cpuData, {title: 'CPU Usage'});
Plotly.newPlot('memoryChart', memoryData, {title: 'Memory Usage'});
Plotly.newPlot('diskChart', diskData, {title: 'Disk Usage'});
Plotly.newPlot('networkChart', networkData, {title: 'Network Activity'});
function updateCharts() {
fetch('/system_stats')
.then(response => response.json())
.then(data => {
var time = new Date().toLocaleTimeString();
Plotly.extendTraces('cpuChart', { y: [[data.cpu_usage]] }, [0]);
Plotly.extendTraces('memoryChart', { y: [[data.memory_usage]] }, [0]);
Plotly.extendTraces('diskChart', { y: [[data.disk_usage]] }, [0]);
Plotly.extendTraces('networkChart', { y: [[data.bytes_sent], [data.bytes_recv]] }, [0, 1]);
if(cpuData[0].y.length > 20) {
Plotly.relayout('cpuChart', {
xaxis: {
range: [cpuData[0].y.length - 20, cpuData[0].y.length]
}
});
Plotly.relayout('memoryChart', {
xaxis: {
range: [memoryData[0].y.length - 20, memoryData[0].y.length]
}
});
Plotly.relayout('diskChart', {
xaxis: {
range: [diskData[0].y.length - 20, diskData[0].y.length]
}
});
Plotly.relayout('networkChart', {
xaxis: {
range: [networkData[0].y.length - 20, networkData[0].y.length]
}
});
}
});
}
setInterval(updateCharts, 2000);
</script>
</body>
</html>
Explanation:
JavaScript Libraries:
Plotly: The script tag includes Plotly, a powerful library for making interactive plots.
Charts:
- Four charts are made to monitor CPU usage, memory usage, disk usage, and network activity. Each chart updates every 2 seconds with new data from the Flask endpoint.
Fetching System Stats:
The
/system_stats
Flask route returns system stats in JSON format.The JavaScript function
updateCharts()
gets these stats and updates the charts usingPlotly.extendTraces()
.
Real-Time Updates:
setInterval(updateCharts, 2000)
calls theupdateCharts
function every 2 seconds, keeping the charts showing real-time data.
Running the Application:
Save the Flask script (e.g.,
app.py
) and the HTML template in the appropriate directory structure.Run the Flask application from the command line:
python app.py
Open your web browser and navigate to
http://127.0.0.1:5000/
to view the graphical system monitoring dashboard.
Output:
When you visit the web page, you will see interactive line charts showing real-time CPU usage, memory usage, disk usage, and network activity. The charts update every 2 seconds, giving you a continuous view of the system's performance.
This visual interface makes it easier to monitor system performance and can be customized with more metrics or additional styling.
Subscribe to my newsletter
Read articles from LingarajTechhub All About Programming directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
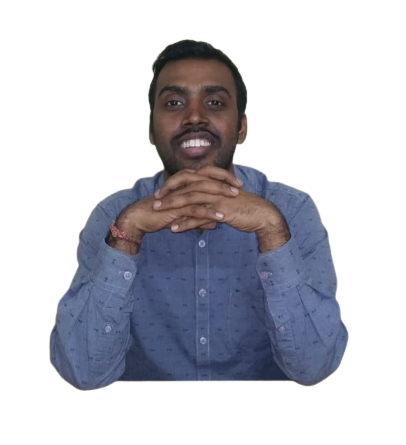
LingarajTechhub All About Programming
LingarajTechhub All About Programming
Corporate Software Development Trainer with a demonstrated track record of success in the IT and Ed-tech industries for product development. I started my career as a software developer and have since taught C, C++, Java, and Python to hundreds of IT enthusiasts, including corporate professionals, throughout the years. I have been developing software for over 12 years.