Understanding Exception Handling in Python

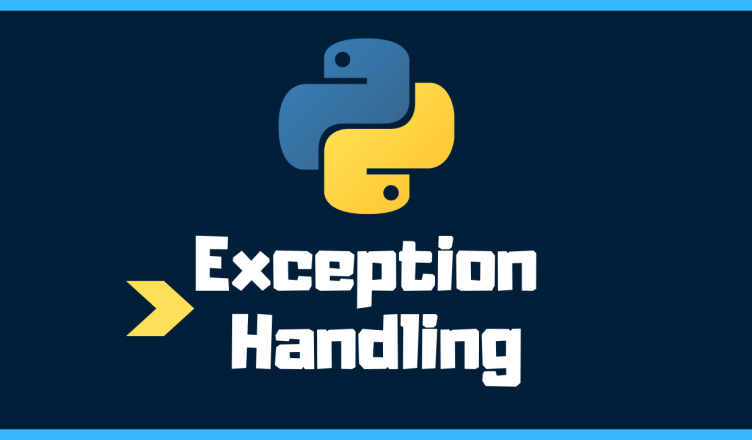
When writing code, errors and exceptions are inevitable. As developers, we strive to write robust applications that can gracefully handle these situations. In Python, exception handling is the mechanism that allows us to manage errors in a structured way, improving both the reliability and readability of our code.
In this blog, we will dive into Python's exception-handling system and learn how to manage errors effectively.
What are Exceptions?
Exceptions in Python are events that disrupt the normal flow of a program. They occur during the program's execution and can be triggered by various causes, such as invalid inputs, division by zero, or missing files.
For example:
pythonCopy code# A simple division by zero exception
x = 10 / 0
When the above code is executed, it raises a ZeroDivisionError
, which halts the program. Without handling it, the program will crash.
Basic Exception Handling with try-except
Python uses try-except
blocks to handle exceptions. The try
block contains the code that may raise an exception, and the except
block is executed when an exception occurs.
Here's a basic example:
pythonCopy codetry:
x = 10 / 0
except ZeroDivisionError:
print("You cannot divide by zero!")
In this case, the program will print the message "You cannot divide by zero!"
instead of crashing.
Multiple Exceptions Handling
You can handle multiple exceptions by adding multiple except
blocks:
pythonCopy codetry:
num = int(input("Enter a number: "))
result = 10 / num
except ZeroDivisionError:
print("You cannot divide by zero!")
except ValueError:
print("Please enter a valid number!")
This handles two types of errors: division by zero and invalid input.
The else and finally Clauses
In addition to try
and except
, Python also provides else
and finally
clauses for more control:
else
Clause: Theelse
block is executed if no exception occurs in thetry
block.Example:
pythonCopy codetry: result = 10 / 2 except ZeroDivisionError: print("Division by zero is not allowed.") else: print(f"Result is: {result}")
finally
Clause: Thefinally
block is always executed, whether an exception occurs or not. This is useful for cleaning up resources (e.g., closing files or database connections).Example:
pythonCopy codetry: file = open("example.txt", "r") # Perform file operations except FileNotFoundError: print("File not found!") finally: file.close() print("File closed.")
Why Use finally
?
You might wonder, "What if I simply add the cleanup code after the try-except
block without using finally
?"
For example:
pythonCopy codetry:
file = open("example.txt", "r")
except FileNotFoundError:
print("File not found!")
file.close() # Code placed outside the try-except
print("File closed.")
This will work perfectly fine if the exception occurs inside the same code block. However, if you are working inside a function, and an exception is raised, the program flow will exit the function, and the code after the try-except
block will not be executed.
Here's an example to illustrate this:
pythonCopy codedef open_file():
try:
file = open("example.txt", "r")
except FileNotFoundError:
print("File not found!")
print("File closed.") # This won't execute if exception occurs!
open_file()
Output:
arduinoCopy codeFile not found!
Notice that "File closed."
is not printed, since the exception caused an early exit from the function. To ensure that the cleanup always happens, you need the finally
block, as shown below:
pythonCopy codedef open_file():
try:
file = open("example.txt", "r")
except FileNotFoundError:
print("File not found!")
finally:
print("File closed.") # This will always be executed
open_file()
Output:
arduinoCopy codeFile not found!
File closed.
The finally
block guarantees that even if an exception occurs, the cleanup code will always run.
Raising Exceptions Manually
Sometimes, you may want to trigger an exception manually using the raise
statement. This is useful when certain conditions in your code need to be flagged as errors.
Example:
pythonCopy codedef check_positive_number(num):
if num < 0:
raise ValueError("Negative numbers are not allowed!")
return num
try:
print(check_positive_number(-10))
except ValueError as ve:
print(ve)
This will raise a ValueError
if a negative number is passed to the function.
Custom Exceptions
Python allows you to create custom exceptions by defining new classes that inherit from the base Exception
class. This can be helpful when you want to create more descriptive error types for specific cases in your code.
Example:
pythonCopy codeclass NegativeNumberError(Exception):
pass
def check_positive_number(num):
if num < 0:
raise NegativeNumberError("This is a negative number!")
return num
try:
print(check_positive_number(-5))
except NegativeNumberError as e:
print(e)
Best Practices for Exception Handling
Be Specific: Catch specific exceptions rather than using a general
except
block.pythonCopy codetry: # Code except (ZeroDivisionError, ValueError): # Handle specific exceptions
Avoid Catching All Exceptions: Using a broad
except
block likeexcept Exception:
can hide bugs and make debugging difficult.Use
finally
for Cleanup: Always usefinally
for resource cleanup (e.g., closing files or releasing database connections).Document Custom Exceptions: If you create custom exceptions, make sure to document their use so other developers can understand their purpose.
Conclusion
Exception handling is an essential part of writing resilient Python applications. By using try
, except
, else
, and finally
blocks, you can handle errors effectively and prevent your programs from crashing unexpectedly. Custom exceptions can further enhance error handling by providing meaningful feedback for specific cases.
While adding code outside of a try-except
block might seem like a quick solution, remember that if your error-handling is part of a function, you'll need to use the finally
clause to ensure critical cleanup steps always run.
Mastering exception handling will allow you to write cleaner, more robust Python code, ensuring a better experience for both developers and users.
Subscribe to my newsletter
Read articles from Aakanchha Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Aakanchha Sharma
Aakanchha Sharma
๐ Welcome to my Hashnode blog! I'm a tech enthusiast and cloud advocate with expertise in IT, backup solutions, and cloud technologies. Currently, Iโm diving deep into Python and exploring its applications in automation and data management. I share insights, tutorials, and tips on all things tech, aiming to help fellow developers and enthusiasts. Join me on this journey of learning and innovation!