Mastering GitHub Actions: A Comprehensive Guide to CI/CD
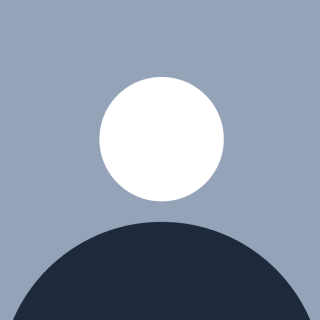
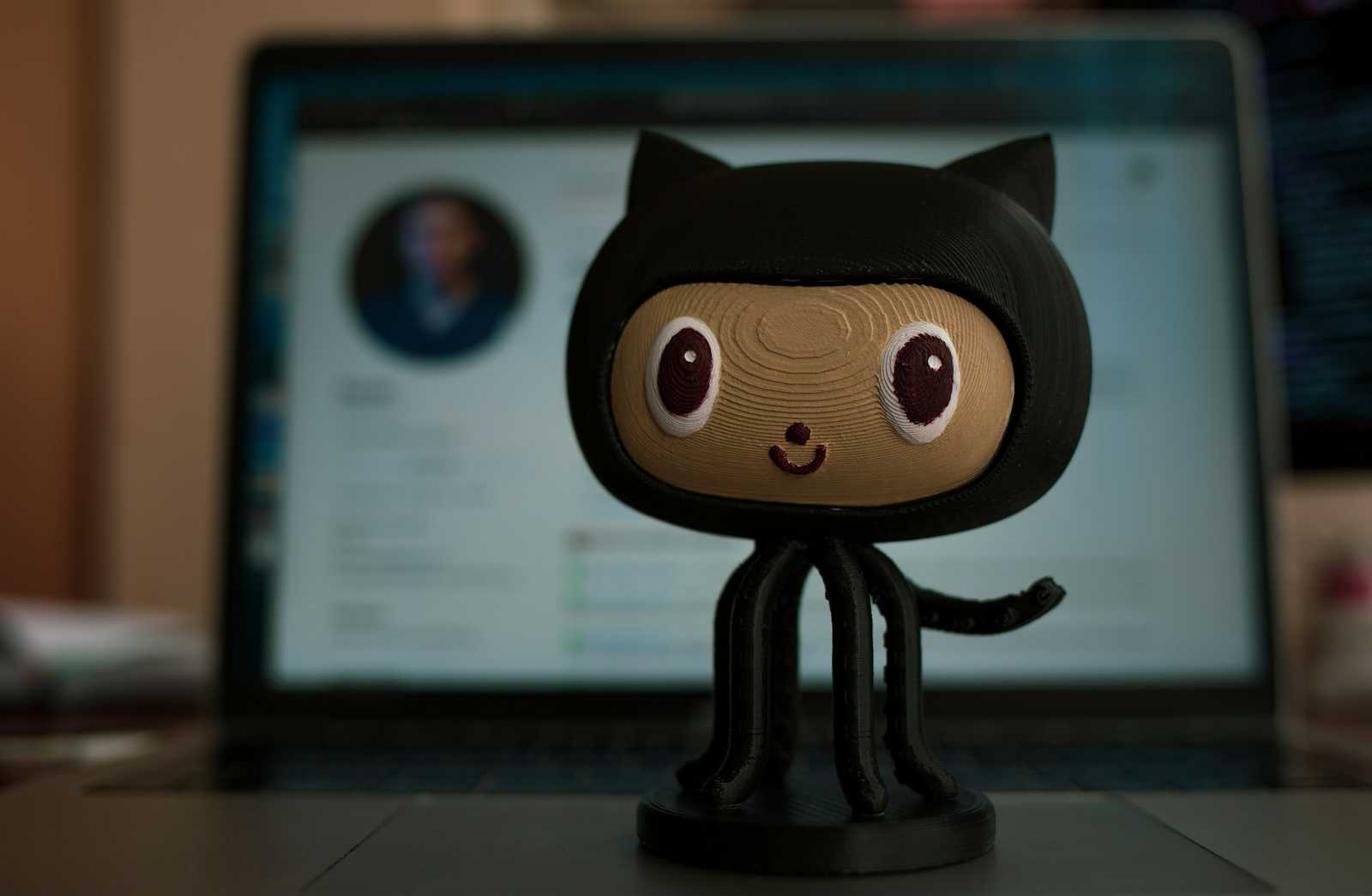
GitHub Actions has revolutionized the way developers approach continuous integration and continuous deployment (CI/CD). In this comprehensive guide, we'll explore the ins and outs of GitHub Actions, from basic concepts to advanced workflows.
What is GitHub Actions?
GitHub Actions is a powerful automation tool that allows you to define custom workflows for your software development lifecycle. These workflows can handle everything from code testing to deployment, all within your GitHub repository.
Key Concepts in GitHub Actions
Workflows
Workflows are at the heart of GitHub Actions. They are automated processes defined in YAML files and stored in the .github/workflows/
directory of your repository.
Here's a simple example of a workflow:
name: CI Workflow
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Run Tests
run: npm test
Events
Events are the triggers that start a workflow. Common events include:
push
: Triggered when code is pushed to the repositorypull_request
: Triggered when a pull request is opened, updated, or mergedschedule
: Runs on a regular schedule using cron syntax
Jobs and Steps
A job is a set of steps that execute on the same runner. Steps are individual tasks within a job that can run commands or use actions.
Actions
Actions are reusable units of code that can perform complex but frequently repeated tasks. GitHub provides a marketplace full of pre-built actions, or you can create your own.
Creating a Workflow
Let's look at a more detailed example of a CI workflow:
name: CI Pipeline
on:
push:
branches:
- "**"
pull_request:
branches:
- main
jobs:
test-and-build:
runs-on: ubuntu-latest
strategy:
matrix:
node-version: [12, 14, 16]
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Set up Node.js
uses: actions/setup-node@v3
with:
node-version: \${{ matrix.node-version }}
- name: Install dependencies
run: npm ci
- name: Run Tests
run: npm test
- name: Lint Code
run: npm run lint
- name: Build Application
run: npm run build
- name: Upload Build Artifacts
uses: actions/upload-artifact@v3
with:
name: build-files
path: build/
This workflow does the following:
Triggers on pushes to any branch and pull requests to the main branch
Sets up a matrix to test across multiple Node.js versions
Checks out the code, installs dependencies, runs tests, lints the code, and builds the application
Uploads the build artifacts for further use
Advanced Features
Environment Variables and Secrets
GitHub Actions allows you to use environment variables and secrets to handle sensitive data securely:
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- name: Deploy to production
run: ./deploy.sh
env:
API_KEY: \${{ secrets.API_KEY }}
Matrix Builds
Matrix builds allow you to test across multiple configurations:
strategy:
matrix:
node-version: [12, 14, 16]
Artifacts and Caching
Artifacts allow you to persist data after a job has completed, while caching can help speed up workflows by reusing expensive fetch operations:
- name: Cache node modules
uses: actions/cache@v2
with:
path: node_modules
key: \${{ runner.os }}-node-\${{ hashFiles('package-lock.json') }}
Best Practices
Keep workflows small and focused
Use secrets for sensitive data
Leverage caching to speed up workflows
Test your scripts locally before adding them to workflows
Use the
actions/checkout@v2
step to ensure you're working with the latest code
Conclusion
GitHub Actions provides a powerful, flexible system for automating your development workflows. By mastering its concepts and features, you can significantly improve your team's productivity and code quality. Start small, experiment, and gradually build more complex workflows as you become more comfortable with the system.
Remember, the key to successful CI/CD is consistency and reliability. With GitHub Actions, you have the tools to achieve both, right within your GitHub repository.
Subscribe to my newsletter
Read articles from Kashinath Meshram directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Kashinath Meshram
Kashinath Meshram
DevOps Engineer with over 1 year of hands-on experience in AWS, Terraform, Docker, and CI/CD automation. Focused on optimizing cloud infrastructure and enhancing system scalability. Based in Nagpur, Maharashtra.