JavaScript Hoisting
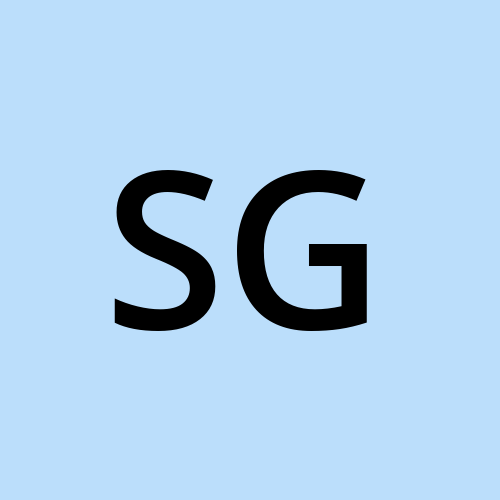
Table of contents
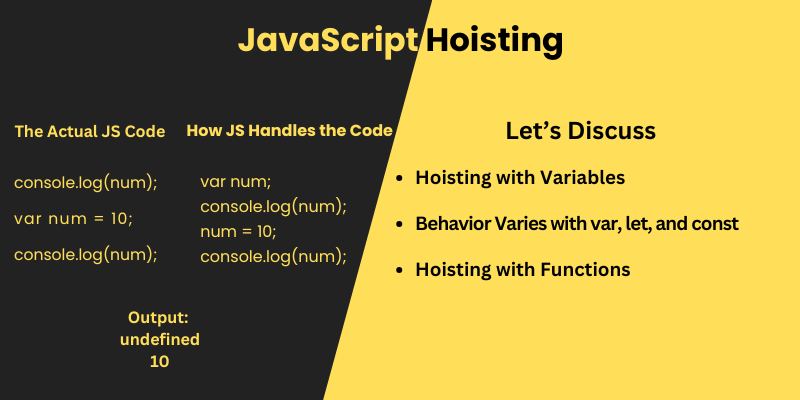
In JavaScript, the word "hoisting" refers to the behavior where variable and function declarations are moved to the top of their containing scope during the compilation phase, before the code is executed. This means that you can use variables and functions before they are actually declared in the code.
Example: In the below example, JavaScript treats the code as if the var num;
declaration was moved to the top, but the initialization (num = 10;
) stays in its original place.
var num; // Declaration hoisted
console.log(num);
// Output: undefined (since initialization hasn't occurred yet)
num = 10; // Initialization
console.log(num); // Output: 10
JavaScript Hoisting with Variables
Variables declared with var
are hoisted and initialized with undefined
, while let
and const
are also hoisted but remain uninitialized, leading to a ReferenceError
if accessed before their declaration.
JavaScript Hoisting with var
The declaration var a;
is hoisted to the top, but its assignment a = 10;
remains in place. Before the assignment, a
is undefined
.
console.log(a);
// Output: undefined
var a = 3;
console.log(a);
// Output: 3
JavaScript Hoisting with let
The variable b
is hoisted but remains uninitialized until the assignment line is reached. Accessing b
before initialization causes a ReferenceError
.
console.log(b);
// Output: ReferenceError: Cannot access 'b' before initialization
let b = 21;
console.log(b);
// Output: 21
JavaScript Hoisting with const
Similar to let
, the variable c
is hoisted but uninitialized. It also results in a ReferenceError
if accessed before its declaration and must be initialized at the time of declaration.
console.log(c);
// Output: ReferenceError: Cannot access 'c' before initialization
const c = 30;
console.log(c);
// Output: 30
Hoisting behavior Varies with var
, let
, and const
var
: Declarations are hoisted, and variables are initialized withundefined
. Accessing them before initialization returnsundefined
.let
andconst
: Declarations are hoisted, but the variables remain in a "temporal dead zone" until initialization. Accessing them before initialization results in aReferenceError
.
JavaScript Hoisting with Functions
Function Declaration
A function declaration is fully hoisted. This means that you can call the function before the actual declaration in the code.
Example: The entire function is hoisted, so the JavaScript engine knows about the function before the code is executed. You can invoke the function anywhere in the scope, even before the function is written.
demo(); // Output: "Hello!"
function demo() {
console.log("Hello!");
}
Function Expressions
A function expression assigns a function to a variable. Function expressions are not hoisted the same way as function declarations. The variable is hoisted, but its assignment (the function definition) is not. Trying to call a function expression before it is defined results in an error.
Example: Only the declaration of the greet
variable is hoisted (as undefined
), but not the function itself. This is why you get a TypeError
when you try to invoke it before the assignment.
greet(); // TypeError: greet is not a function
var greet = function(){
console.log("Hello!");
};
Arrow Functions
Arrow functions are a special type of function expression. Like other function expressions, arrow functions are not fully hoisted. This means the variable is hoisted, but the function definition is not.
Example: The variable greet
is hoisted, but the function definition is not. So, you cannot call it before the assignment.
greet(); // TypeError: greet is not a function
var greet = () => {
console.log("Hello!");
};
Anonymous Function Expressions
Anonymous functions are function expressions where the function has no name, assigned to a variable.
Example: Only the variable sayHello
is hoisted (as undefined
), but the function definition is not.
sayHello(); // TypeError: sayHello is not a function
var sayHello = function() {
console.log("Hi!");
};
Conclusion
In JavaScript, hoisting moves variable and function declarations to the top of their scope during compilation. Variables declared with var
are hoisted and initialized as undefined
, while let
and const
remain uninitialized, leading to ReferenceError
if accessed before initialization. Function declarations are fully hoisted, but function expressions and arrow functions are not.
Subscribe to my newsletter
Read articles from Shivani Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
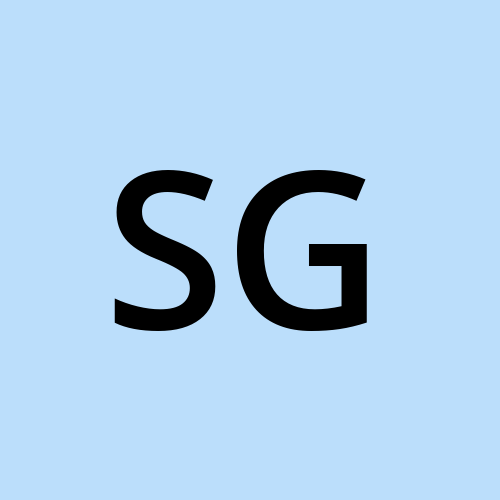