Directory Backup with Rotation

2 min read
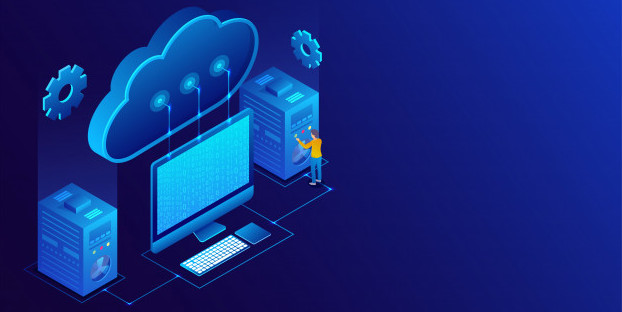
The script will create timestamped backup folders and copy all the files from the specified directory into the backup folder.
Additionally, the script will implement a rotation mechanism to keep only the last 3 backups.
#!/bin/bash
#This script will take backup with 3 days rotation
if [[ "$#" -eq 0 ]];
then
echo "Usage: $0 /path/to/dir"
exit 1
fi
source_dir=$1
# Check if the provided argument is a directory
if [[ ! -d "$source_dir" ]];
then
echo "Error: '$source_dir' is not a directory."
exit 1
fi
timestamp=$(date +%d-%m-%y_%H:%M:%S)
backup_dir="/home/backup/backup_$timestamp"
# Create the backup directory
sudo mkdir -p "$backup_dir"
echo "Directory Created: $backup_dir"
# Function to perform the backup
function backup {
sudo zip -r "$backup_dir/$timestamp.zip" "$source_dir" >/dev/null
}
# Perform the backup
backup
if [[ $? -eq 0 ]];
then
echo "Backup Created: $backup_dir"
else
echo "Backup Unsuccessful"
exit 1
fi
# Count the number of backups
backup_count=$(ls -d /home/backup/backup_* 2>/dev/null | wc -l)
echo "Number of backups: ${backup_count[@]}"
# Remove oldest backups, keeping the last 3
if [[ "$backup_count" -gt 3 ]];
then
ls -d /home/backup/backup_* | head -n -3 | sudo xargs rm -rf
echo "Old backups removed, only the last 3 are kept."
fi
exit 0
Cron Job:
If you'd like to automate the backup process using cron, add it to your crontab :
crontab -e
Example crontab entry to run the backup every 1 minute:
*/1 * * * * bash /home/backup/backup_with_rotation.sh /home/ubuntu/
This will automate the backup process while ensuring only the last 3 backups are retained.
See the process go into /home/backup
watch ls
#ctrt + c to exit
Happy Learning:)
0
Subscribe to my newsletter
Read articles from Imran Shaikh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
