Building Agnishield: Developing a Chrome Extension Firewall with AI-Powered Domain Blocking
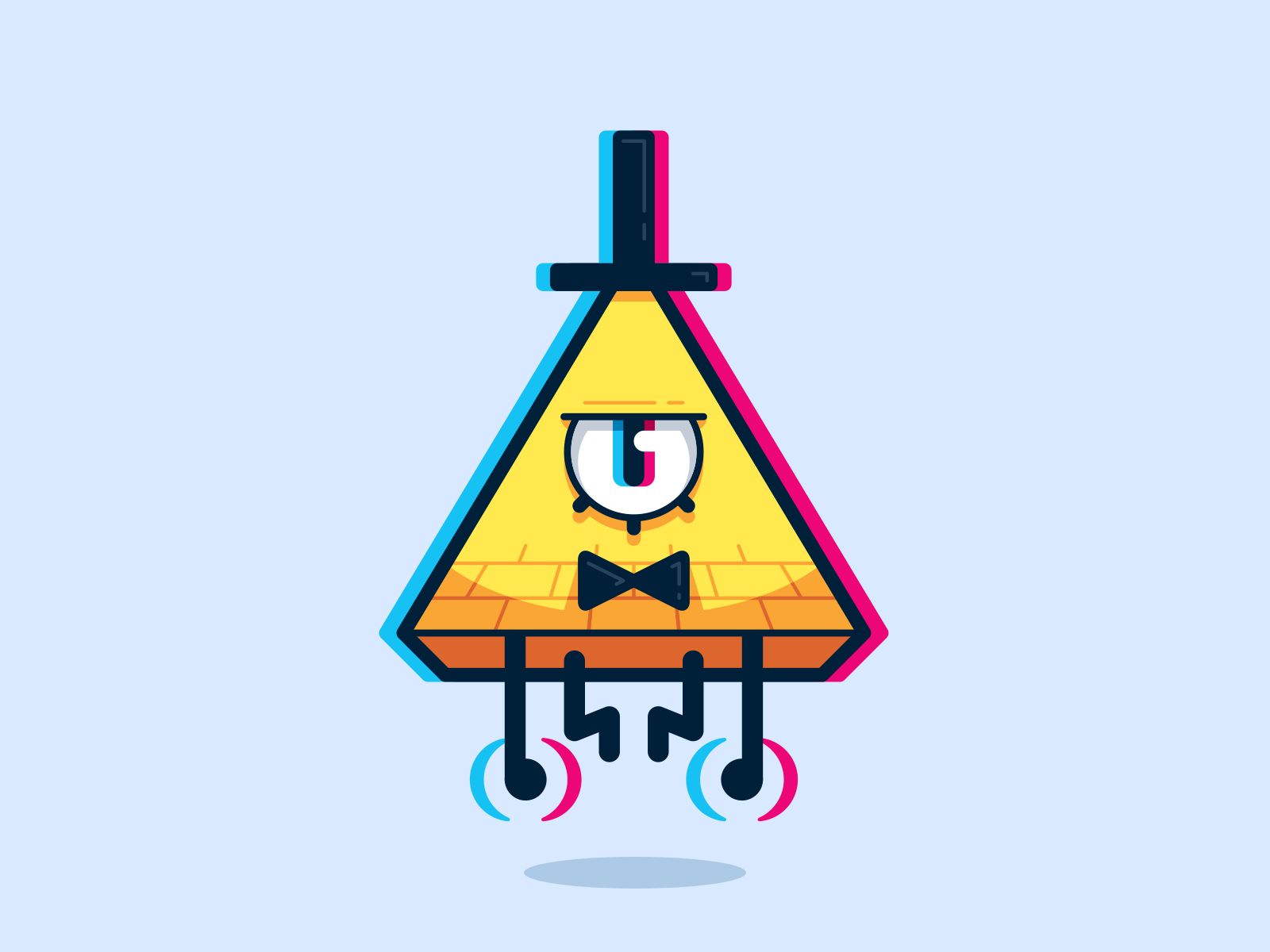
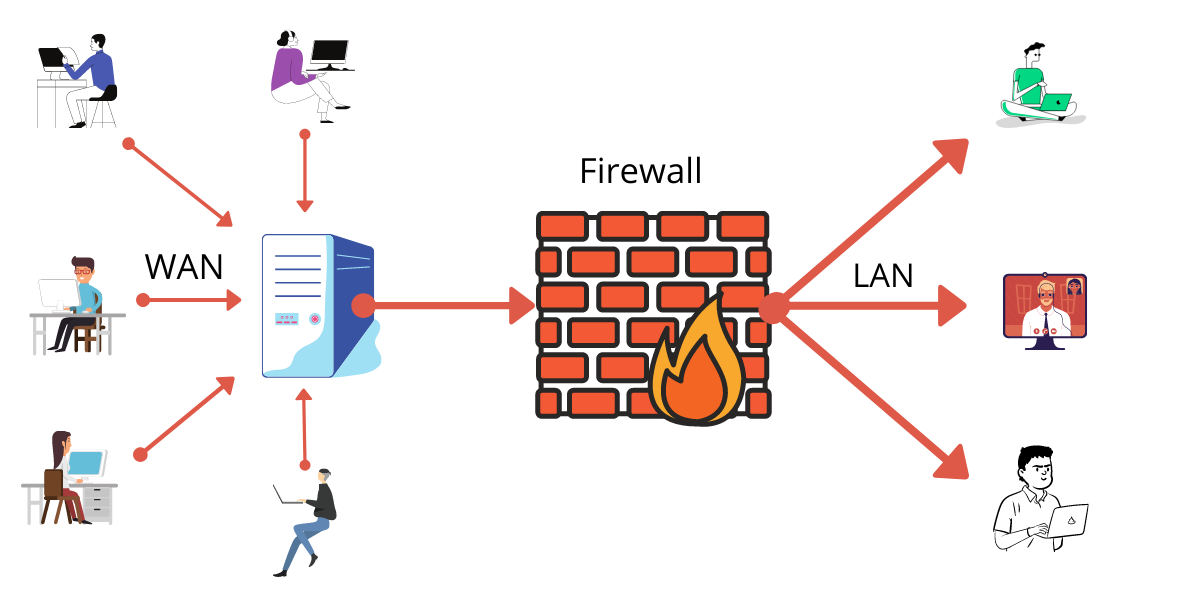
In an age where cyber threats are evolving at an unprecedented pace, safeguarding users against malicious websites is more critical than ever. Traditional security measures often lag behind sophisticated attacks, necessitating innovative solutions that can adapt in real-time. Enter Agnishield Web Firewall—a Chrome extension firewall enhanced with AI-powered domain blocking. This blog chronicles the technical journey of building Agnishield, detailing the challenges faced, solutions implemented, and the interconnected components that make it a robust security tool.
Introduction: The Genesis of Agnishield
The motivation behind Agnishield stemmed from a simple observation: while browsing the internet, users are constantly at risk of unknowingly accessing malicious domains that could compromise their security. Existing browser extensions and firewalls often rely on static blacklists, which are insufficient against new and emerging threats. Agnishield aims to bridge this gap by integrating artificial intelligence to predict and block malicious domains in real-time.
Objectives
Proactive Threat Detection: Utilize machine learning to identify and block malicious domains before they can harm the user.
Seamless Integration: Develop a Chrome extension that operates transparently, without disrupting the user's browsing experience.
Continuous Improvement: Implement a system that learns from new data, enhancing its detection capabilities over time.
Architectural Overview: Marrying AI with Browser Security
At the heart of Agnishield lies the synergy between a client-side Chrome extension and a backend server powered by AI models. The interconnected components work together to provide real-time protection:
Chrome Extension: Intercepts outgoing web requests and communicates with the backend to determine the legitimacy of domains.
Backend Server: Hosts machine learning models and APIs that analyze domain data and return classification results.
Database: Stores historical data on domains, user feedback, and supports the training of AI models.
AI Models: Utilize algorithms trained on vast datasets to predict the likelihood of a domain being malicious.
Figure 1: High-level architecture of Agnishield.
Developing the Chrome Extension Firewall
Intercepting Web Requests
To monitor and control web traffic, Agnishield uses the chrome.webRequest
API:
javascriptCopy codechrome.webRequest.onBeforeRequest.addListener(
handleRequest,
{ urls: ["<all_urls>"] },
["blocking"]
);
function handleRequest(details) {
const url = new URL(details.url);
const domain = url.hostname;
// Proceed to check the domain...
}
This listener intercepts all outgoing web requests, allowing the extension to analyze each domain before the browser proceeds.
Communicating with the Backend
The extension needs to query the backend to determine if a domain is malicious:
javascriptCopy codeasync function isDomainMalicious(domain) {
try {
const response = await fetch(`https://api.agnishield.com/check?domain=${domain}`);
const result = await response.json();
return result.isMalicious;
} catch (error) {
console.error('Error fetching domain status:', error);
return false; // Default to allowing the domain if there's an error
}
}
Caching Mechanism for Efficiency
To minimize latency and reduce redundant network calls, the extension implements a caching system:
javascriptCopy codeconst domainCache = new Map();
function getCachedDomainStatus(domain) {
if (domainCache.has(domain)) {
const { status, timestamp } = domainCache.get(domain);
// Invalidate cache after a certain period
if (Date.now() - timestamp < CACHE_DURATION) {
return status;
}
}
return null;
}
function updateCache(domain, status) {
domainCache.set(domain, { status, timestamp: Date.now() });
}
Handling User Experience
When a domain is blocked, the extension provides feedback to the user:
Notification Bar: Displays a message indicating that the site has been blocked.
Override Option: Allows users to proceed to the site if they believe it's safe, adding it to a personal whitelist.
Reporting: Users can report false positives, which are sent back to the backend for model retraining.
Backend Infrastructure: Powering the AI Engine
API Development
The backend exposes RESTful APIs for the extension to interact with:
Domain Check Endpoint:
/check?domain=
example.com
- Returns a JSON response indicating whether the domain is malicious.
Feedback Endpoint:
/feedback
- Accepts user feedback for false positives or negatives.
Database and Data Storage
Agnishield uses a robust database system to store:
Domain Data: Features and classification results for each domain.
User Feedback: Logs of user reports to improve the AI models.
Model Data: Training data and model parameters.
AI Model Implementation
Data Collection and Preparation
Malicious Domains: Gathered from threat intelligence feeds and phishing databases.
Benign Domains: Collected from popular websites and user browsing patterns.
Feature Extraction: Includes lexical features (domain length, character distribution), WHOIS data, and hosting information.
Model Training
Utilized machine learning algorithms such as Random Forests and Gradient Boosting:
pythonCopy codefrom sklearn.ensemble import RandomForestClassifier
# Assuming features and labels are prepared
model = RandomForestClassifier(n_estimators=100)
model.fit(X_train, y_train)
Deployment
API Integration: The trained model is loaded into the backend API server.
Real-time Prediction: Incoming domain checks are processed by the model to return immediate results.
Overcoming Challenges: Debugging and Lessons Learned
The Issue of Blocking All Sites
During testing, an update led to the extension blocking all web requests indiscriminately.
Root Cause Analysis
Logical Error: A conditional statement was incorrectly configured, causing the extension to default to blocking every domain.
API Failure Handling: The extension didn't account for scenarios where the backend API was unreachable, leading to overly aggressive blocking.
Solution Implementation
Conditional Fix: Corrected the logic to accurately interpret the AI model's output.
Fallback Mechanism: Implemented default behaviors when the API is unreachable, such as allowing the request or using cached data.
Robust Error Handling: Enhanced error-catching mechanisms to prevent similar issues.
Ensuring Performance and Security
Performance Optimization: Profiled the extension to identify bottlenecks, optimized code paths, and minimized the extension's impact on browsing speed.
Security Measures: Enforced strict content security policies and ensured all communications between the extension and backend are encrypted.
Personal Contributions: Crafting the AI Dataset and Codebase
Dataset Creation
Feature Engineering: Developed algorithms to extract meaningful features from domain names, such as entropy calculations and n-gram analysis.
Balanced Dataset: Ensured a balanced representation of malicious and benign domains to prevent model bias.
Data Augmentation: Employed techniques to simulate phishing domains and expand the dataset.
Developing the Codebase
Extension Development: Wrote the core JavaScript code for the Chrome extension, implementing the request interception and user interface components.
API Server: Built the backend server using Node.js and Express, integrating the AI model for real-time predictions.
Continuous Integration: Set up pipelines for automated testing and deployment, facilitating rapid iteration and updates.
Future Roadmap: Enhancements and Expansion
Advanced Threat Detection
Deep Learning Models: Explore neural networks for improved detection accuracy, especially in identifying sophisticated phishing domains.
Behavioral Analysis: Incorporate user behavior patterns to detect anomalies indicative of compromised systems.
Cross-Browser Compatibility
Firefox Extension: Adapt the extension to work with Mozilla Firefox, leveraging the WebExtensions API for compatibility.
Edge and Safari Support: Extend support to other popular browsers, ensuring a wider user base.
User Empowerment Features
Customizable Settings: Allow users to adjust sensitivity levels, manage whitelists/blacklists, and configure notifications.
Community Feedback Loop: Create a platform for users to share insights, report new threats, and contribute to the security database.
Conclusion: Reflecting on the Journey
Building Agnishield was a complex endeavor that required integrating diverse technologies and addressing multifaceted challenges. The interconnected components—from the Chrome extension to the AI-powered backend—work in harmony to provide users with a proactive defense mechanism against web-based threats.
Key Takeaways
Interdisciplinary Collaboration: Success hinged on combining expertise in web development, cybersecurity, and machine learning.
User-Centric Design: Prioritizing the user experience ensured that the security measures did not become intrusive or hamper usability.
Adaptability: The constantly evolving threat landscape necessitates a solution that can learn and adapt over time.
Moving Forward
The battle against cyber threats is ongoing. With Agnishield, we take a step towards empowering users with tools that not only protect but also evolve. Collaboration and community involvement will be crucial as we refine the system, incorporate user feedback, and stay ahead of emerging threats.
Subscribe to my newsletter
Read articles from Utkarsh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
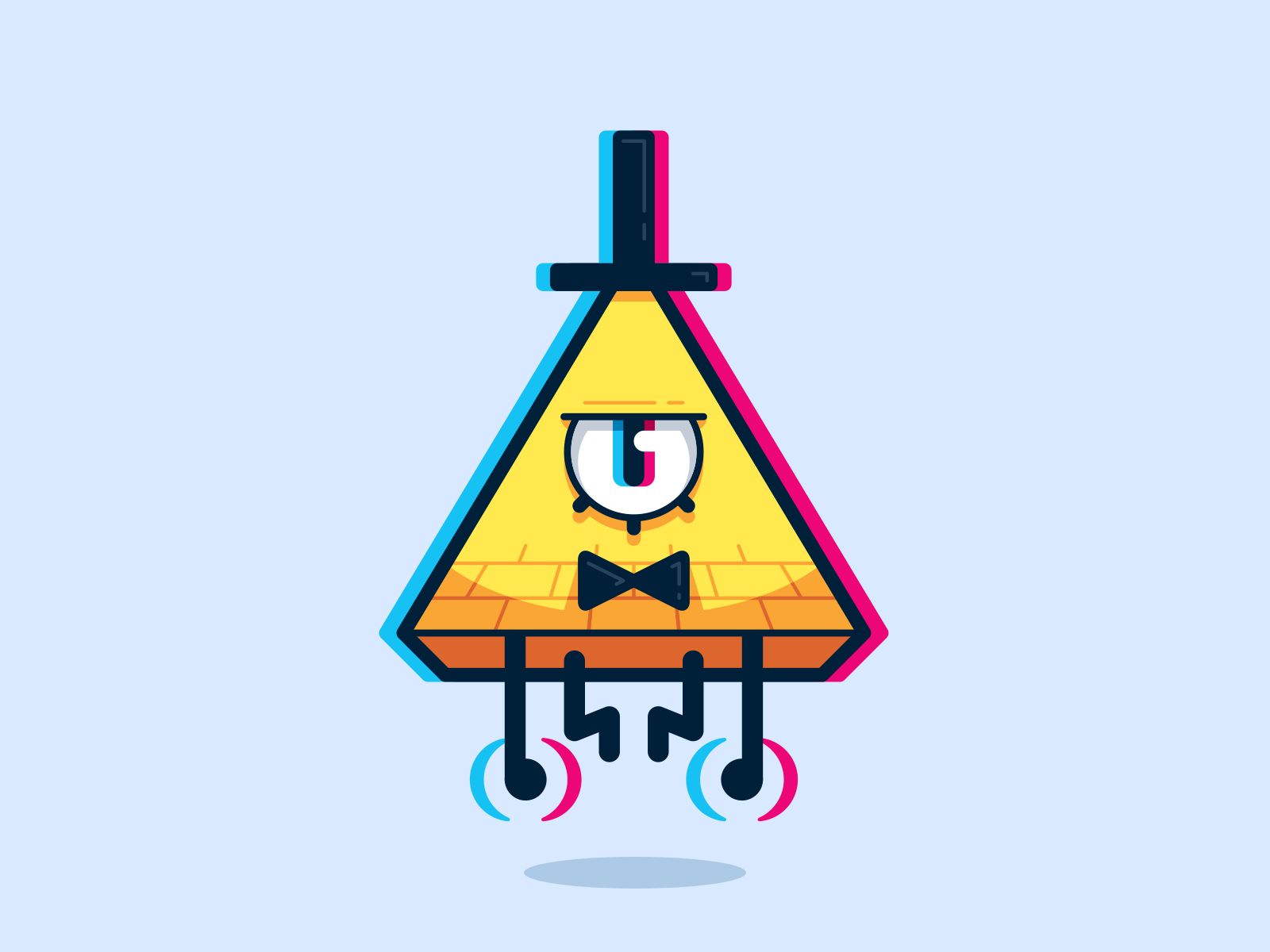