Day 11 - Working with CSV Files Using Pandas

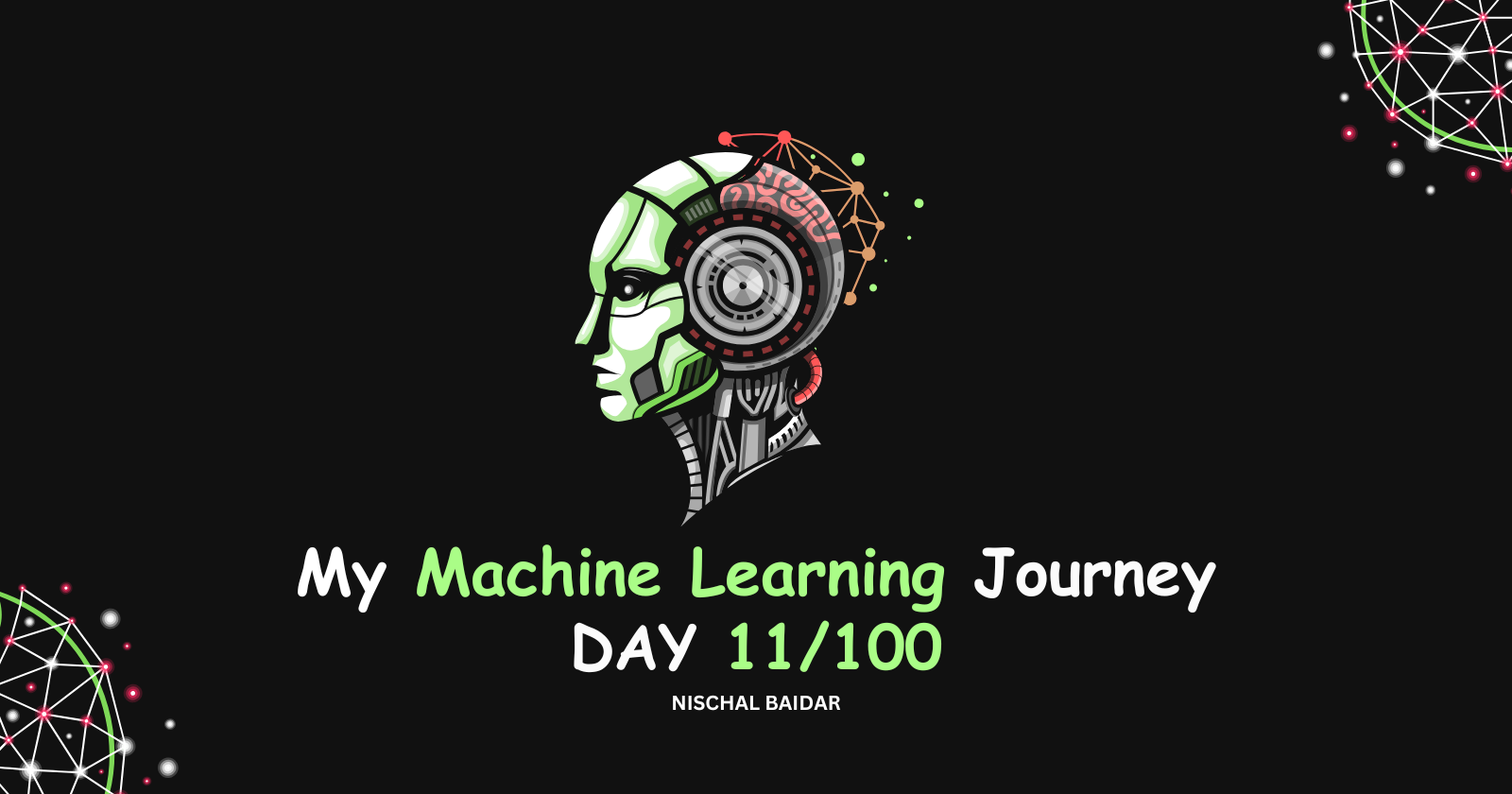
When working with data in Python, CSV (Comma-Separated Values) files are one of the most commonly used formats for data storage and exchange. Pandas, a powerful data manipulation library, makes it extremely easy to load, manipulate, and analyze CSV files.
What is a CSV File?
A CSV file stores tabular data (such as a spreadsheet or database) in plain text. Each line of the file corresponds to a row in the table, and the values within each line are separated by commas.
Setting Up Pandas in Jupyter Notebook
Before working with CSV files, you’ll need to have Pandas installed in your environment. If you’re working in a Jupyter Notebook, you can install Pandas by running:
!pip install pandas
Once Pandas is installed, import it into your notebook:
import pandas as pd
Pandas attributes and functions for working with CSV files:
pd.read_csv()
: Loads a CSV file into a Pandas DataFrame.
Example: df =
pd.read_csv('file.csv')
df.head()
: Displays the first 5 rows of the DataFrame.
Example: df.head()
df.tail()
: Displays the last 5 rows of the DataFrame.
Example: df.tail()
df.info()
: Provides a summary of the DataFrame (data types, non-null counts).
Example: df.info
()
df.describe()
: Returns a statistical summary of numeric columns.
Example: df.describe()
df.columns
: Lists all the column names in the DataFrame.
Example: df.columns
df.shape
: Returns the number of rows and columns as a tuple.
Example: df.shape
df.dtypes
: Shows the data types of each column.
Example: df.dtypes
df.isnull().sum()
: Counts the number of missing values in each column.
Example: df.isnull().sum()
df.to_csv()
: Exports the DataFrame to a CSV file.
Example: df.to
_csv('output.csv')
df.drop()
: Removes specified rows or columns from the DataFrame.
Example: df.drop(columns=['column_name'])
df.fillna()
: Fills missing values with a specified value.
Example: df.fillna(0)
df.sort_values()
: Sorts the DataFrame based on a specified column.
Example: df.sort_values('column_name')
Subscribe to my newsletter
Read articles from Nischal Baidar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
