Android Learning Path with Jetpack Compose
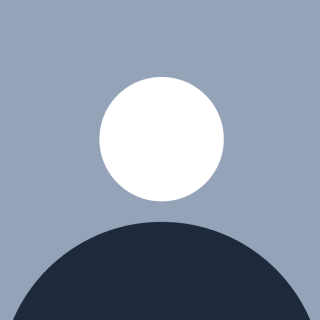
Table of contents
- Jetpack Compose Basics
- Layouts in Jetpack Compose
- Jetpack Compose Components
- Using State in Jetpack Compose
- Jetpack Compose Theming
- Jetpack Compose Animation
- Jetpack Compose Navigation
- Testing in Jetpack Compose
- Accessibility in Jetpack Compose
- Migrating to Jetpack Compose
- Advanced State and Side Effects in Jetpack Compose
- Android MultiThreading
- Services
- JobScheduler
- Broadcast Receivers
- Content Provider
- Data Persistence
- Android Networking
- Architecture Patterns
- Dependency Injection
- Design Patterns
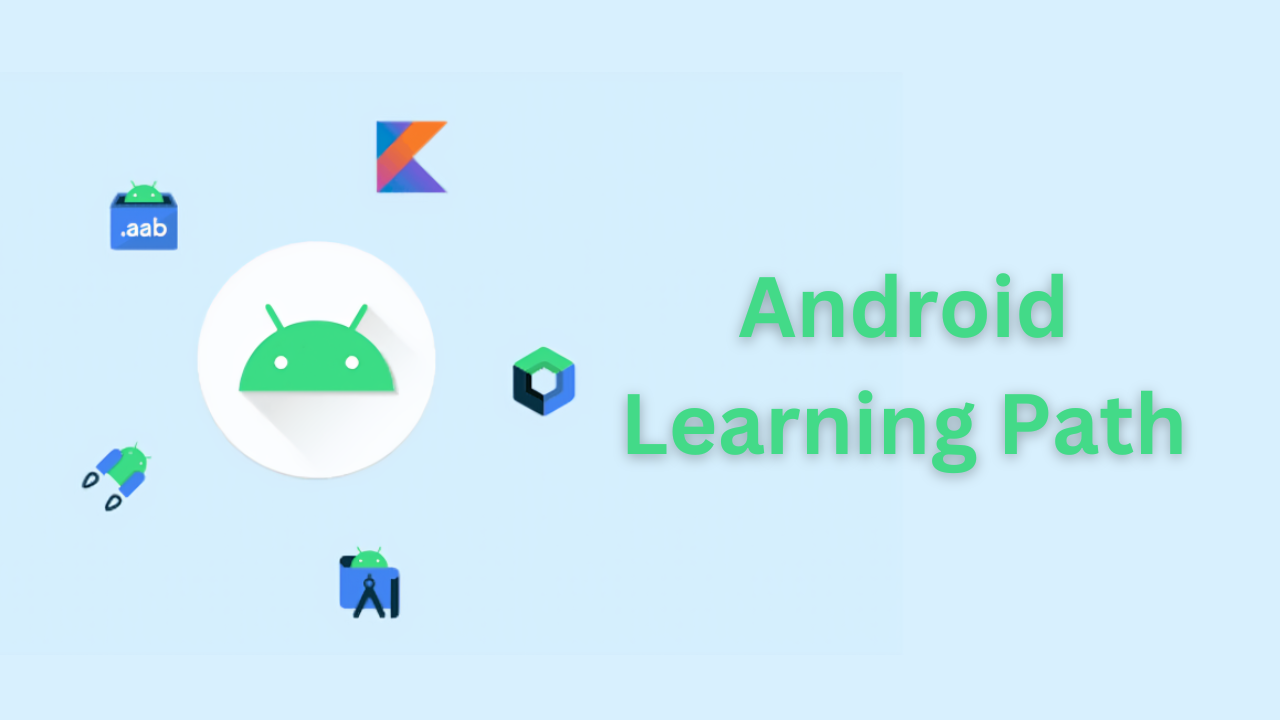
The article provides a comprehensive guide on learning Android development with Jetpack Compose. It covers the basics of Compose, building UIs, managing state, and data flow principles. It delves into layouts, components, theming, animation, navigation, testing, accessibility, and migration to Compose. Advanced topics include state management, multithreading, services, job scheduling, broadcast receivers, content providers, data persistence, networking, architecture patterns, dependency injection, and design patterns.
Jetpack Compose Basics
In this section, you will learn:
What Compose is
How to build UIs with Compose
Jetpack Compose architectural layering
Data flow principles in Compose
Lifecycle of composables
Side-effects in Compose
Jetpack Compose phases
How to manage state in composable functions
Layouts in Jetpack Compose
In this section, you'll learn how to use Compose's highest level of UI abstraction, Material Design, as well as low-level composables like Layout that allow you to measure and place elements on the screen.
Scaffold
- Scaffold composable to provide structure for your screens.
App Bars
- Various composables let you create app bars along the top and bottom of your screens.
Layouts in Compose
Compose Layout Basics
Compose Modifiers
Constraints and Modifier Order
Create Custom Modifiers
List of Compose Modifiers
Pager in Compose
Flow Layouts in Compose
Custom Layouts
Adaptive Layouts
Alignment Lines
Intrinsic Measurements
ConstraintLayout
Jetpack Compose Components
In this section, you'll explore various components available in Jetpack Compose to build interactive and visually appealing UIs.
Text and Typography: Tools for displaying and styling text.
Images and Graphics: Components for loading and displaying images.
Loading Images: Methods to efficiently load images.
ImageBitmap vs. ImageVector: Different formats for handling images.
Material Icons: Predefined icons following Material Design guidelines.
Customize an Image: Techniques to modify image appearance.
Custom Painter: Create custom drawings and graphics.
Box: Stack children on top of each other for overlays.
Column: Arrange children vertically.
Row: Arrange children horizontally.
Surface: Provides a material surface with customizable properties.
Spacer: Adds space between components.
TextField: Input text with various styles.
RadioButton: Select one option from a set.
ToggleButton: Switch between two states.
TabRow: Create tabbed navigation.
DropdownMenu: Display a dropdown menu with items.
Buttons: Interactive elements for user actions.
Floating Action Buttons: Circular buttons for primary actions.
Cards: Contain content and actions about a single topic.
Chips: Compact elements representing an input, attribute, or action.
Dialogs: Modal windows for user interactions.
Progress Indicators: Show progress of a task.
Sliders: Select a value from a range.
Switches: Toggle between on/off states.
Checkboxes: Select multiple options from a set.
Badges: Display small status descriptors.
Bottom Sheets: Slide-up panels for additional content.
Dividers: Separate content visually.
Navigation Drawers: Side panels for navigation.
Time Pickers: Select a time.
Date Pickers: Select a date.
Snackbars: Brief messages at the bottom of the screen.
Lists and Grids: Organize content in scrollable lists or grids.
LazyColumn: Efficient vertical scrolling lists.
LazyRow: Efficient horizontal scrolling lists.
Resources Management: Manage app resources like strings, dimensions, and colors.
Strings: Handle text resources.
Dimensions: Consistent sizing resources.
Colors: Maintain a color scheme.
Vector Assets and Image Resources: Scalable graphics.
Animated Vector Drawables: Dynamic visual effects.
Icons: Enhance UI with icon resources.
Fonts: Custom typography management.
Using State in Jetpack Compose
In this section, you'll learn about the state and how it can be used and manipulated by Jetpack Compose.
Jetpack Compose Theming
In this section, you will learn how to use Jetpack Compose's theming APIs to style your application. You'll see how to customize colors, shapes, and typography so that they're used consistently throughout your application, supporting multiple themes such as light and dark themes.
Anatomy of a theme
Material Design 3
Material 2 vs Material 3
Custom Theming
Jetpack Compose Animation
In this section, you will learn how to use some Animation APIs in Jetpack Compose.
Jetpack Compose Navigation
In this section, you'll learn how to use the Jetpack Navigation component to navigate between screens in Jetpack Compose.
Testing in Jetpack Compose
In this section, you'll learn about testing UIs created with Jetpack Compose. You will write your first tests while learning about testing in isolation, debugging tests, semantics trees, and synchronization.
Accessibility in Jetpack Compose
In this section, you will learn how to use Jetpack Compose to improve your app's accessibility. We will walk through several common use cases and improve a sample app step by step. We will cover touch target sizes, content descriptions, click labels, and more.
Migrating to Jetpack Compose
In this section, you'll be migrating parts of the XML screen to Compose.
Advanced State and Side Effects in Jetpack Compose
In this section, you will learn advanced concepts related to State and Side Effects APIs in Jetpack Compose. You'll see how to create a state holder for stateful composables whose logic isn't trivial, how to create coroutines and call suspend functions from Compose code, and how to trigger side effects to accomplish different use cases.
Android MultiThreading
Thread, Runnables, and Callables
- Basics of threading and how to use Runnables and Callables
Handlers and Loopers
- Use Handlers and Loopers for message handling
Executors and ThreadPool
- Manage threads using Executors and ThreadPools
AsyncTask
- Use AsyncTask for background operations (Note: Deprecated in API level 30)
RxJava
- Use RxJava for reactive programming
WorkManager
- Use WorkManager for background tasks
AlarmManager
- Schedule tasks using AlarmManager
Coroutines
- Use Kotlin Coroutines for asynchronous programming
Services
Background Service / Started Service
- Create and manage background services
Foreground Service
- Create and manage foreground services
Bound Service
- Create and manage bound services
Android Interface Definition Language (AIDL)
- Use AIDL for inter-process communication
IntentService / JobIntentService
- Use IntentService and JobIntentService for background tasks
JobScheduler
- Use JobScheduler for scheduling background tasks
Broadcast Receivers
Normal Broadcast Receiver
Use sendBroadcast()
Implicit and explicit broadcasts
Dynamic and static registration
Ordered Broadcast Receiver
Use sendOrderedBroadcast()
Implicit and explicit broadcasts
Dynamic and static registration
Sticky Broadcast
- Note: Deprecated in API 21
Local Broadcast
Use LocalBroadcastManager
Implicit and explicit broadcasts
Dynamic registration
Content Provider
- Use Content Providers for data sharing between applications
Data Persistence
SharedPreferences
- Store and retrieve simple data using SharedPreferences
Room Database
Use Room for database management
Entities, DAOs, and database migrations
SQLite
- Basics of SQLite database management
DataStore
- Use DataStore for data persistence
Android Networking
Volley
- Use Volley for network operations
OkHttp
- Use OkHttp for HTTP requests
Retrofit
- Use Retrofit for network operations and API calls
Ktor
- Use Ktor for asynchronous network operations
Architecture Patterns
MVC (Model — View — Controller)
- Basics of the MVC architecture pattern
MVP (Model — View — Presenter)
- MVP architecture pattern
MVVM (Model — View — ViewModel)
- Implement the MVVM architecture pattern using ViewModel and LiveData
Seign Pattern
- Seign pattern and its use cases in software architecture
Dependency Injection
Basics of Dependency Injection
- Principles of Dependency Injection (DI) and its benefits
Dagger
- Use Dagger for dependency injection in Android
Hilt
- Use Hilt, a dependency injection library built on top of Dagger, for simpler DI in Android applications
Koin
- Use Koin, a lightweight dependency injection framework for Kotlin
Design Patterns
Singleton Pattern
- Implement the Singleton pattern to ensure a class has only one instance
Factory Pattern
- Factory pattern for creating objects without specifying the exact class
Observer Pattern
- Use the Observer pattern to allow objects to be notified of changes in other objects
Builder Pattern
- Builder pattern for constructing complex objects step by step
Adapter Pattern
- Use the Adapter pattern to allow incompatible interfaces to work together
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer