Have you ever wondered how JavaScript executes code?
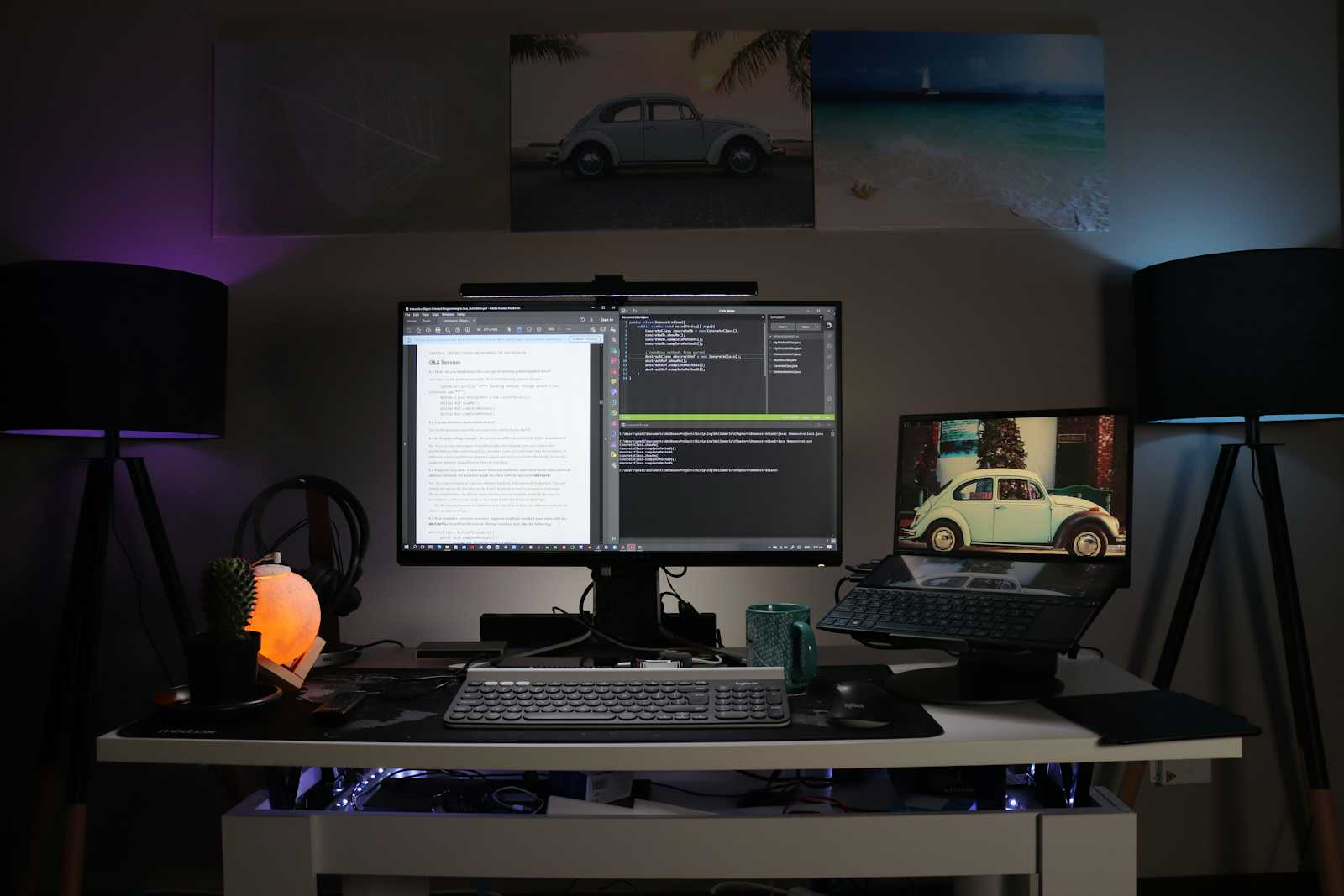
javascript executes code by creating and managing Execution contexts
There are two types of Execution Contexts
Global Execution contexts
Function Execution contexts
Global Execution Contexts
In JavaScript, a global execution context is created when the JS file is loaded. This context is set up for every execution context that is created. An execution context is a container that holds all the necessary information for the JavaScript code to be executed, such as variables, functions, and objects.
When the JS file is loaded, the code outside of any functions is executed in the global execution context. This includes any variable declarations, function declarations, and other statements that are not inside a function. This global execution context is available to all other execution contexts that are created, such as function contexts or block contexts.
It's important to note that the global execution context is only created once when the JS file is loaded. All subsequent execution contexts are created within this global context. This means that any variables or functions declared in the global context are available to all other contexts within the same file.
Function Execution Context
When a function is called, a new execution context is created. This execution context consists of the function's arguments, local variables, and references to any variables in the parent scope chain. The execution context is used to keep track of the function's state while it is executing, including the current value of the function's variables and the position in the code where the function is currently executing. Once the function completes its execution and returns a value, the execution context is destroyed, and any memory allocated to it is freed.
Creation of Execution Context
The creation of execution context involves two phases
Creation phase / Memory phase
Execution Phase
Memory phase
The execution context is associated with an Execution context object (ECO), which includes the variable object, scope chain, and the value of the 'this' keyword. The variable object stores variables and function declarations, setting up the environment for code execution
JavaScript is a single-threaded language, meaning that it executes code line by line. This behavior ensures that each line of code is executed before moving on to the next, which helps avoid any conflicts or issues that might arise due to parallel execution.
Code
Let's understand using the code sample
let a=10
let b= 5
function sumnum(num1,num2){
let total = num1+num2
return total
}
let result1 = sumnum(a,b)
let result2 = sumnum(10,2)
During the memory phase, the values of all variables will be undefined.
Variables | property |
a | undefined |
b | undefined |
sumnum() | definition |
result1 | undefined |
result2 | undefined |
In execution phase,
Variables | property |
a | 10 |
b | 5 |
sumnum | new variable environment + execution thread |
result1 | undefined |
result2 | undefined |
Call Stack
JavaScript uses a Call Stack to manage execution contexts. When a script starts executing, the GEC is pushed onto the stack. Each time a function is invoked, an FEC is pushed on top of the stack. When a function completes its task, its context is popped off the stack, returning control to the previous context.
Thanks for reading this article! If you have any feedback or questions, drop them in the comments and I'll get back to you.
connect with me on Twitter, Linkedin, and Hashnode. Let's stay in touch :)!!
Subscribe to my newsletter
Read articles from misba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
misba
misba
As someone who is passionate about technology, I have set my sights on becoming a full-stack developer. In addition to this, I am also very interested in the field of AI/ML. I am eager to learn more about this field and see how it can be applied to create innovative solutions. I am enhancing my knowledge and sharing my thoughts through blog posts.