How React Works: A Comprehensive Guide to JSX, Virtual DOM, and React Fiber

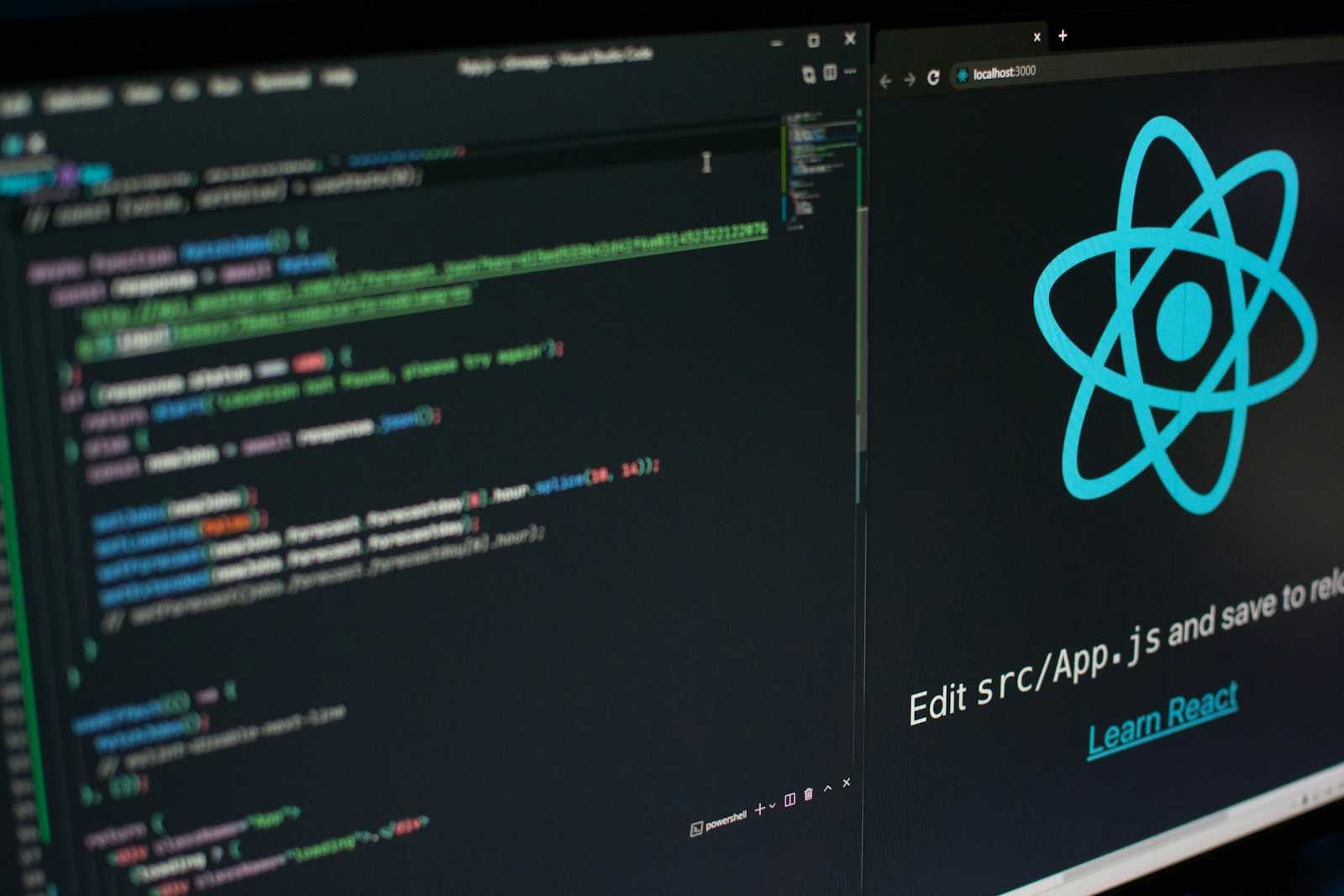
React has grown to be one of the most widely used libraries for developing user interfaces and for a reason. This is because it gives a way to construct fast, interactive apps, though all the magic actually happens behind the scenes with how it handles updates and rendering. In this post, we will explore how React does its rendering using concepts such as JSX, the Virtual DOM, and React Fiber to allow your app to run smoothly.
1. Setting Up the Root Container
In any React application, you have to create a root container first. This will be the base of your application, where all of your components will show up. You do this by using:
const root = ReactDOM.createRoot(document.getElementById('root'));
Here, root
becomes the entry point, at which React will render your components using the method root.render()
. For example:
root.render(<App />);
This would render the App
component into the root. You could say App
is the main "parent" container of all the other components. Now, this is where React Fiber steps in and makes things efficient by chunking these tasks into smaller bits so that your UI stays responsive.
2. JSX Transcompilation to JavaScript
React uses a syntax called JSX that looks like HTML. You are allowed to write components in a more natural way; however, browsers don't understand JSX. Babel—a JavaScript transpiler—takes it in and spits out regular old JavaScript that the browsers understand.
Example
If you write this in JSX:
<p id="greeting" className="intro">Hello, World!</p>
Then Babel transforms it into the following code:
React.createElement('p', { id: 'greeting', className: 'intro' }, 'Hello, World!');
Every JSX element will be converted to a React Element object; each object contains a type ('p'), properties ({ id: 'greeting', className: 'intro' }), and children ('Hello, World!'). That structure keeps React informed of what it is to render.
3. Virtual DOM: React's Memory Space
React creates a lightweight version of the actual DOM, known as the Virtual DOM. Think of it like a blueprint of your webpage, but it exists in memory. When the data of your app changes, React updates this Virtual DOM first and then uses it to efficiently compute the best way to update the actual webpage.
Example of Virtual DOM
Following is a simple component and what its Virtual DOM structure might look like:
{
type: 'div',
props: {
children: [
{
type: 'h1',
props: {
children: 'Hello, React!'
}
},
{
type: 'p',
props: {
children: 'Learning how React renders the DOM.'
}
}
]
}
}
This representation is used by React to know what has changed, without directly messing with the real DOM, which is slower to change.
4. React Fiber: Making Updates Efficient
Fiber is a mighty update manager. It breaks updates down into discrete, smaller pieces called fibers and decides which order to execute them—prioritizing execution, in other words. Before Fiber, if React had several successive updates to process, it would try to complete all of them at once. That might make your application sluggish, especially during periods where a lot changed in a brief span of time.
How Fiber Works
Fiber helps React pause, prioritize updates, and then resume those updates. For example, if a user clicks on a button that is of high priority while a huge data fetch is in progress—say, low priority—Fiber ensures the button click gets processed first by pausing the data fetch and resuming it when the high-priority task has been rendered. In this way, the app stays responsive and usable.
5. Reconciliation and Diffing
What is Reconciliation?
Once something has changed in a component—new data or a state update—React needs to re-render the UI so that it reflects those changes. This is called reconciliation. It is basically creating a new virtual DOM. React then compares this new version of the Virtual DOM with the previous version to see what is different.
The Diffing Algorithm
That comparison is done with the help of a diffing algorithm. It's like a game of spot-the-difference between what's changed in the new and old Virtual DOMs. React knows what has altered, so instead of re-rendering the entire page, it updates only what's changed in the real DOM.
How React Fiber Improves This
React Fiber is a kind of project manager in this regard. It breaks the work down into tiny units called "fibers" and figures out how to spread those changes over time; that is, it determines when to schedule tasks based on priority. This allows React to pause and resume work on tasks so the app remains responsive, even when dealing with complex updates. For example, if there are animations or user inputs, Fiber will make sure those are taken care of quickly before less critical updates.
6. Updating the Real DOM: Batching Changes
React doesn't update the actual DOM on every little change. What React does is batch updates: React groups several changes together and then applies all those changes at once. And that's exactly what makes updates faster—the fact that React interacts less with the DOM.
How Fiber Helps with Updates
React Fiber organizes the way these updates are being applied, ensuring that urgent changes—for example, animations or user clicks—get processed first, while less critical updates like background data take their turn. That's how your app feels smooth and fast.
After figuring out what needs to change, React uses the Virtual DOM differences to update the real DOM. It does this in three main ways:
- Updating existing elements: If the content of an element changes, React updates that part in the real DOM.
const h1Element = document.getElementById('some-id');
h1Element.textContent = 'Hello, World!';
Adding new elements: If you add a new element, React creates it using
document.createElement()
and adds it to the page.const newElement = document.createElement('p'); newElement.textContent = 'This is a new paragraph.'; parentElement.appendChild(newElement);
Removing elements: When an element is no longer needed, React finds it in the real DOM and removes it.
const elementToRemove = document.getElementById('some-id'); elementToRemove.parentNode.removeChild(elementToRemove);
7. Event Handling
React normalizes events so that they are cross-browser and synthetic. That means React puts these events at the root of the DOM, not on every element—this improves performance.
8. State and Props
If the state or props of a component change, then React updates that component and all of its children.
9. Unmounting and Cleanup
When something is no longer needed—say, when moving to another page—React unmounts it. This means taking the component from the DOM and cleaning up the remains that might have been left behind—including event listeners.
Conclusion
React's strength comes in how it handles the render cycle:
JSX makes it easier to write UI components.
The Virtual DOM helps React find the changes.
React Fiber updates declaratively, prioritizing high-priority changes and keeping your application interactive.
Subscribe to my newsletter
Read articles from Aayush Pant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Aayush Pant
Aayush Pant
Aspiring software developer with a passion for AI and web development.