Reactjs Pure Components

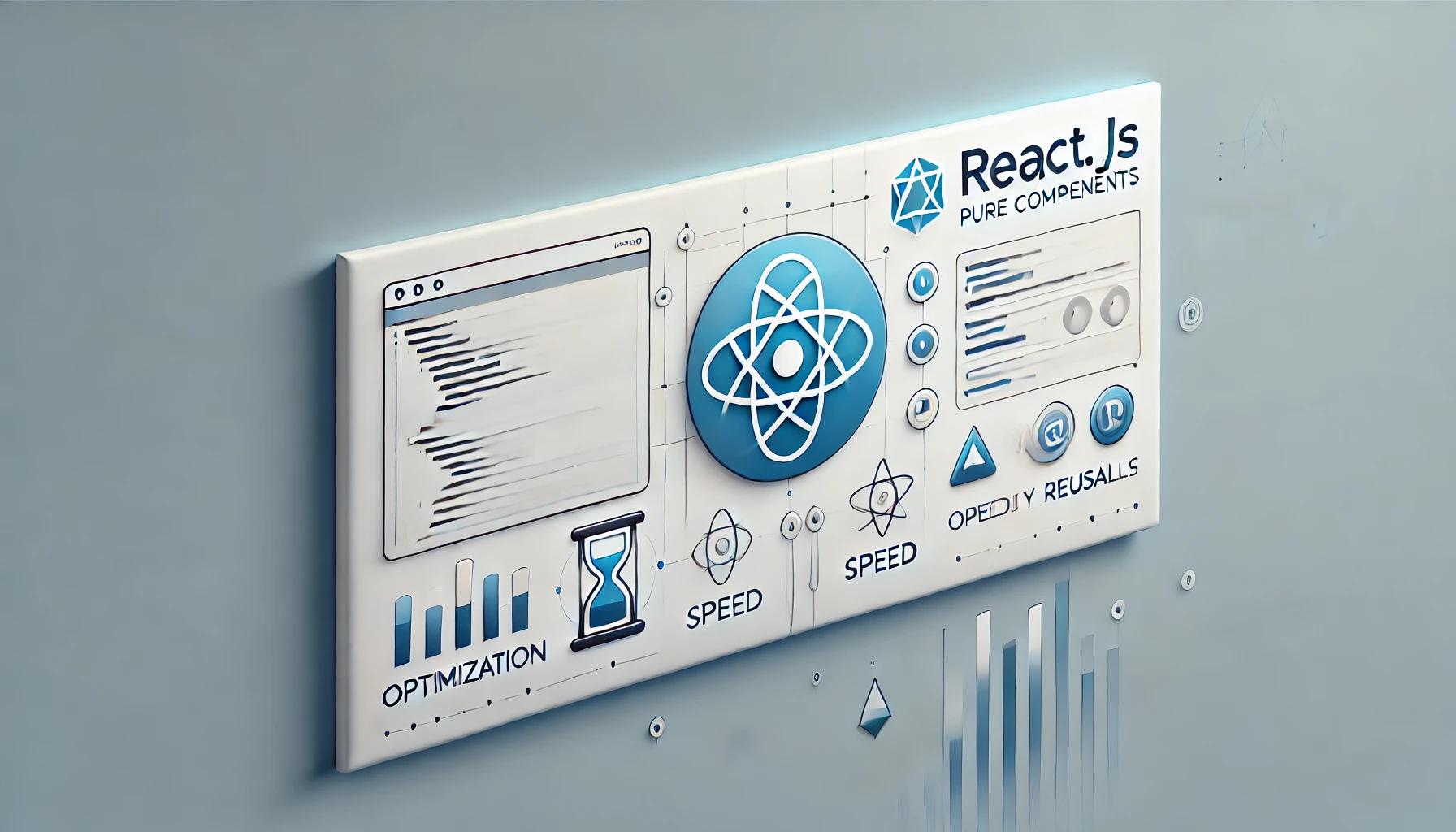
Keeping Component Pure
Some JavaScript functions are pure functions only do calculations and nothing else. By writing your components as pure functions, you can avoid many confusing bugs and unpredictable behavior as your codebase grows. To gain these benefits, you need to follow a few rules.
Purity: Components as formulas
In computer science (and especially the world of functional programming), a pure function is a function with the following characteristics:
It minds its own business. It does not change any objects or variables that existed before it was called.
Same inputs, same output. Given the same inputs, a pure function should always return the same result.
You might already be familiar with one example of pure functions: formulas in math.
Consider this math formula: y = 2x.
If x = 2 then y = 4. Always.
If x = 3 then y = 6. Always.
If x = 3
, y won’t sometimes be 9 or –1 or 2.5
depending on the time of day or the state of the stock market.
If y = 2x and x = 3, y will always be 6.
If we made this into a JavaScript function, it would look like this:
function double(number) {
return 2 * number;
}
In the above example, double
is a pure function. If you pass it 3
, it will return 6
. Always.
React is designed around this concept. React assumes that every component you write is a pure function. This means that React components you write must always return the same JSX given the same inputs:
function Recipe({ drinkers }) {
return (
<ol>
<li>Boil {drinkers} cups of water.</li>
<li>Add {drinkers} spoons of tea and {0.5 * drinkers} spoons of spice.</li>
<li>Add {0.5 * drinkers} cups of milk to boil and sugar to taste.</li>
</ol>
);
}
export default function App() {
return (
<section>
<h1>Spiced Chai Recipe</h1>
<h2>For two</h2>
<Recipe drinkers={2} />
<h2>For a gathering</h2>
<Recipe drinkers={4} />
</section>
);
}
Output :
When you pass drinkers={2}
to Recipe
, it will return JSX containing 2 cups of water
. Always.
If you pass drinkers={4}
, it will return JSX containing 4 cups of water
. Always.
Just like a math formula.
You could think of your components as recipes: if you follow them and don’t introduce new ingredients during the cooking process, you will get the same dish every time. That “dish” is the JSX that the component serves to React to render.
For more examples and detailed explanations, visit the official React.js website. There, you'll find in-depth documentation and best practices to help you master pure components and optimize your applications.
Stay up to date with the latest features and improvements by regularly checking the React community's resources!
Connect with Me on Social Media 📱
I love engaging with fellow developers and readers! If you have any questions, feedback, or just want to connect, feel free to reach out to me on social media. You can find me on Twitter and LinkedIn. I look forward to hearing from you and continuing the conversation! 🌐
Subscribe to my newsletter
Read articles from Harsh Goswami directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Harsh Goswami
Harsh Goswami
Harsh | Frontend Developer 👨💻 I’m a passionate frontend developer with 2 years of experience at Softcolon Pvt Ltd. I specialize in creating responsive, user-centric web applications using modern frameworks like React.js, Next.js, and Remix. Currently, I’m focused on enhancing my skills in web development and exploring new technologies. When I'm not coding, you’ll find me collaborating with developers worldwide, participating in hackathons, or working on my portfolio website. I’m also considering starting a blog to share insights and connect with the frontend community. Let’s build amazing things together!