Developing Reactive Microservices with Spring Boot and Java
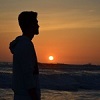
Understanding Reactive Programming
Before diving into microservices, let's grasp the concept of reactive programming. In contrast to traditional imperative programming, reactive programming focuses on non-blocking operations, allowing applications to handle a large number of concurrent requests efficiently. Think of it as a river flowing smoothly, compared to a dam holding back water
The Benefits of Reactive Microservices
Scalability: Reactive systems can handle a surge in traffic without compromising performance.
Resilience: They are more fault-tolerant, capable of recovering from failures gracefully.
Responsiveness: Users experience faster response times, even under heavy load.
Efficient Resource Utilisation: Reactive systems optimise resource usage, leading to cost savings.
Spring Boot: A Powerful Framework
Spring Boot simplifies the development of Spring-based applications, including reactive microservices. It provides a plethora of features and dependencies, reducing boilerplate code and speeding up development. Many Java training course in Delhi, Noida, Pune and other parts of India now incorporate Spring Boot as a key part of the curriculum, especially as reactive programming gains prominence.
Key Components for Reactive Microservices
WebFlux: This is the core reactive web framework in Spring Boot, offering non-blocking HTTP servers and reactive programming models.
Project Reactor: This provides the foundation for reactive programming in Spring Boot, offering reactive types and operators.
Spring Data Reactive: This extends Spring Data to support reactive data access, allowing you to work with databases and other data sources in a non-blocking manner.
Building a Reactive Microservice
Let's create a simple reactive microservice that fetches data from a REST API and returns it as a JSON response.
Create a Spring Boot Project: Use Spring Initializr to generate a new project with the WebFlux dependency.
Define a Reactive Repository: Create a repository interface using Spring Data Reactive to interact with your data source.
Implement a Reactive Service: Write a service class that uses the repository to fetch data and return it in a reactive format.
Create a Reactive Controller: Develop a controller class that handles HTTP requests and returns reactive responses using WebFlux.
Example Code:
Java
public class MyController {
private MyService myService;
("/data")
public Mono<String> getData() {
return myService.fetchData()
.map(data -> "Data: " + data);
}
}
Best Practices for Reactive Microservices
Avoid Blocking Operations: Ensure that your code is non-blocking to maintain responsiveness.
Leverage Backpressure: Use back pressure mechanisms to control the flow of data and prevent overloading downstream components.
Use Reactive Data Access: Employ reactive data access patterns to avoid blocking I/O operations.
Monitor and Test: Regularly monitor your reactive microservices to identify performance bottlenecks and ensure they are functioning as expected.
Conclusion
Reactive microservices offer a powerful approach to building scalable, resilient, and responsive applications. By leveraging Spring Boot and its reactive components, you can create microservices that effectively handle high concurrency and provide a great user experience.
Subscribe to my newsletter
Read articles from Sanjeet Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
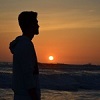
Sanjeet Singh
Sanjeet Singh
I am Sanjeet Singh, an IT professional with experience in the IT sector. I have a broad understanding of Data Analytics and proficiency across multiple layers of software development and testing, from the front end to the back end.