50-Day JavaScript Fun Roadmap ๐
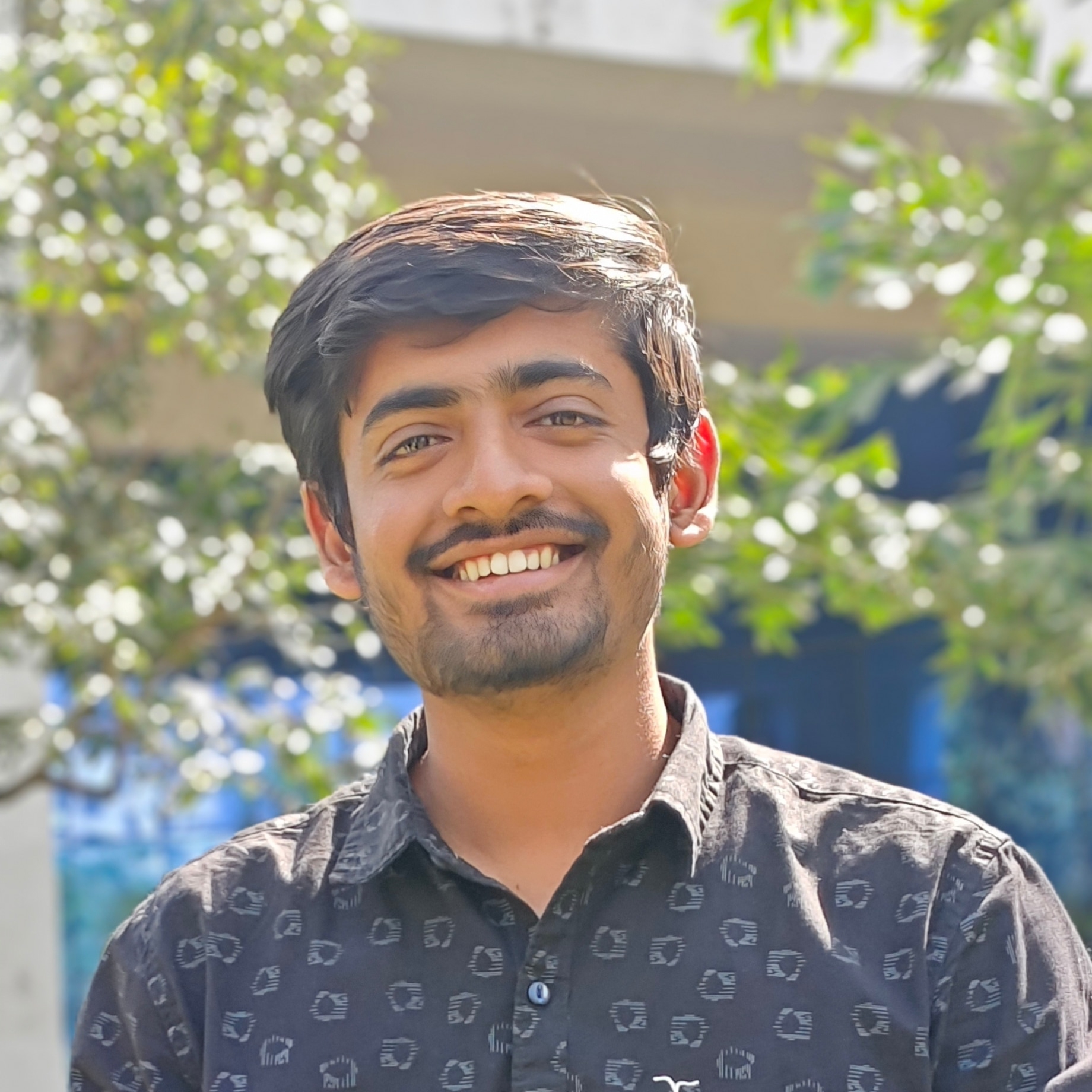
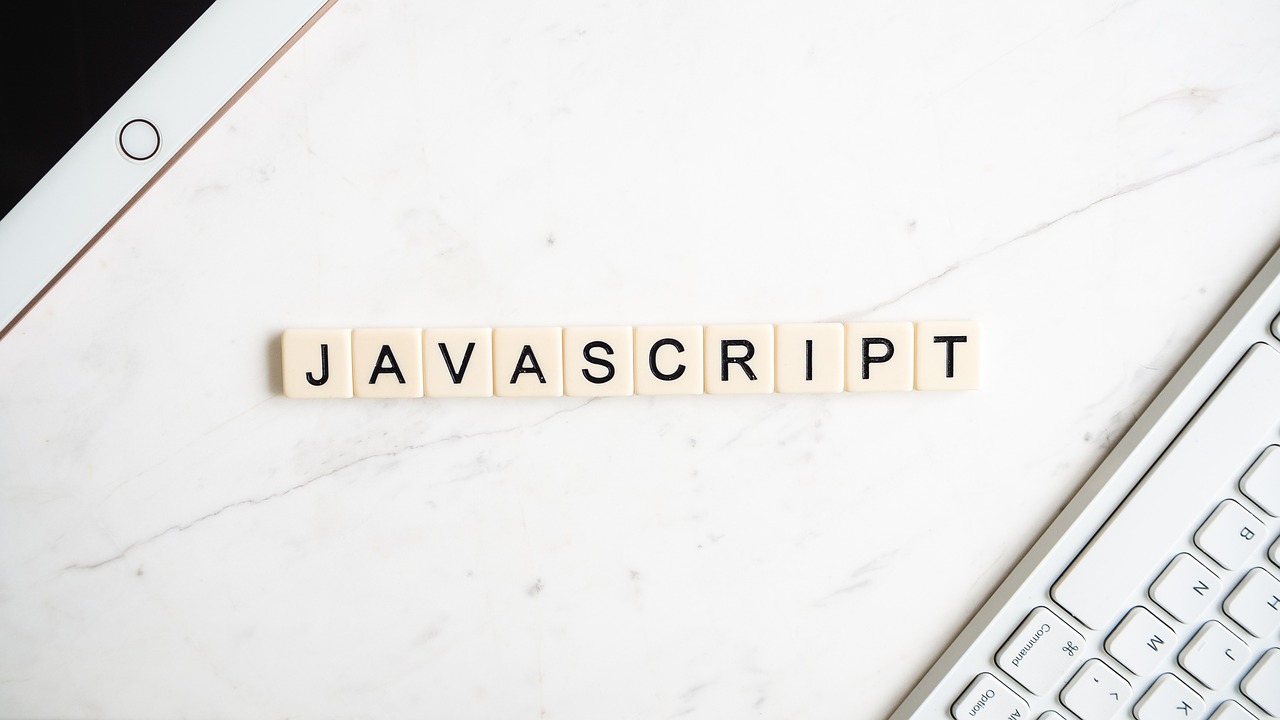
Phase 1: Foundations and Basics (Days 1-10)
Goal: Build a solid base with JavaScript essentials.
Day 1: Hello World! ๐
Intro to JS, setting up with browser console & CodePen.
Challenge: Make an alert pop up with your name!
Day 2: Variables: Pet Names Only ๐
Learn
var
,let
,const
.Task: Declare variables for three imaginary pets.
Day 3: Data Types Party ๐
Numbers, Strings, Booleans, Arrays, Objects.
Game: Spot the data type! (Provide examples and they guess the type).
Day 4: Operators Showdown ๐คบ
Arithmetic, comparison, and logical operators.
Quiz: "Will this expression return true or false?"
Day 5: Conditionals โ JS Drama ๐ฅท
if-else
,switch
.Fun task: Make a mini decision-maker: "Should I binge Netflix today?"
Day 6: Loops: Time Warp ๐
for
,while
,do-while
.Challenge: Loop through an array of favorite anime characters.
Day 7: Functions are Magic Spells โจ
Declaring and calling functions.
Task: Write a function that returns your favorite emoji.
Day 8: ES6: New Tricks ๐ ๏ธ
Arrow functions, template literals,
let
vsconst
.Meme day: Share your favorite ES6 meme!
Day 9: Arrays: Playlist Maker ๐ต
Array methods:
push
,pop
,slice
,map
,filter
.Fun Task: Create a "study playlist" using arrays.
Day 10: Objects: Create Your Avatar ๐ง
Key-value pairs, nested objects.
Task: Build a character profile using objects!
Phase 2: Intermediate JavaScript (Days 11-25)
Goal: Dive deeper into JavaScript with DOM manipulation and async concepts.
Day 11: DOM 101: Talking to the Web ๐
Select elements with
getElementById
,querySelector
.Task: Change the background color of a webpage.
Day 12: Events: Click and Play! ๐ฎ
Learn about click, keydown, and mouseover events.
Task: Add a button that alerts โHello World!โ
Day 13: Forms and Inputs: Build a Mini Survey ๐
Get values from forms using JS.
Fun Task: Create a feedback form for your students.
Day 14: CSS + JS: Styling Fun ๐๏ธ
Change styles with JavaScript.
Task: Add a button that toggles dark mode!
Day 15: Timers and Intervals: Tic-Tac-Tick ๐ฐ๏ธ
Learn
setTimeout
andsetInterval
.Task: Make a clock that updates every second.
Day 16: Error Handling: Bugs Everywhere ๐ชฒ
Try-catch blocks.
Fun twist: Share funny error messages youโve encountered.
Day 17: Arrays + DOM: Display Your Tasks ๐
- Create a to-do list using arrays and the DOM.
Day 18: LocalStorage: Save the Day! ๐พ
Learn how to store and retrieve data.
Task: Save your to-do list using localStorage.
Day 19: Fetch API: Call the Internet ๐ก
Introduction to
fetch()
and promises.Task: Fetch a random joke from an API and display it.
Day 20: Async-Await: JS on Chill Mode ๐
Rewrite promises with async-await.
Task: Create a weather app with OpenWeather API.
Day 21: Callbacks vs Promises: The Big Fight โ๏ธ
Understand the difference.
Meme day: Post a callback-hell meme.
Day 22: Destructuring: Unpack the Magic ๐
Array and object destructuring.
Task: Destructure your playlist array.
Day 23: Spread and Rest: Scatter and Gather ๐
Practice spreading and gathering elements.
Task: Use spread to combine two arrays.
Day 24: Modules: Break the Code ๐ฆ
Learn ES6 modules and imports.
Task: Split your to-do app into multiple files.
Day 25: Review Day: Build a Mini Project ๐๏ธ
- Build a random quote generator with everything learned.
Phase 3: Advanced Topics + Projects (Days 26-40)
Goal: Learn advanced concepts and build cool stuff.
Day 26: Classes: Create Your Own Pokรฉmon ๐
Learn ES6 classes.
Task: Create a Pokรฉmon class with methods.
Day 27: Prototypes: Under the Hood ๐ง
Learn about prototype inheritance.
Task: Implement simple prototype-based inheritance.
Day 28: Closures: Keep it Private ๐
Learn closures with examples.
Fun quiz: Guess whatโs logged to the console.
Day 29: Currying: JavaScript Chef ๐
Understand function currying.
Task: Curry a function to multiply numbers.
Day 30: Higher-Order Functions: Factory Time ๐ญ
Learn
map
,filter
,reduce
.Task: Create a factory function with
map
.
Day 31: Event Delegation: Party Manager ๐
- Learn event bubbling and delegation.
Day 32: Web Storage API: Note-Taker App ๐๏ธ
- Create a note-taking app using sessionStorage and localStorage.
Day 33: Debounce & Throttle: Stop the Spam ๐ฆ
- Implement debounce and throttle functions.
Day 34: Drag and Drop: Build a Puzzle ๐งฉ
- Learn drag-and-drop with events.
Day 35: Regex: Search the Matrix ๐ถ๏ธ
Learn regular expressions.
Task: Validate an email input.
Day 36-40: Build a Project: Weather or Task App ๐ฆ๏ธ
- Choose between weather or task manager projects using everything you learned.
Phase 4: Final Projects & Interview Prep (Days 41-50)
Goal: Solidify learning with projects and practice interview questions.
Day 41-43: Project: Build a Portfolio Website ๐
- Create a simple portfolio showcasing your work.
Day 44-46: Debugging and Code Optimization ๐
- Practice debugging techniques.
Day 47: Common Interview Questions ๐ง
- Review important JavaScript questions.
Day 48: Mock Interview: Practice Time! ๐ค
- Run a mock interview session.
Day 49: Code Review: Learn from Peers ๐ฅ
- Review code with a friend or mentor.
Day 50: Celebrate and Publish ๐
Celebrate your JavaScript journey!
Publish your final project and share on Hashnode.
This roadmap covers fun learning, interactive tasks, and hands-on projects. ๐ฏ Each day has something new, ensuring that learners stay engaged! You can gamify the experience further with badges or challenges for every milestone.
Subscribe to my newsletter
Read articles from Risharth pardeshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
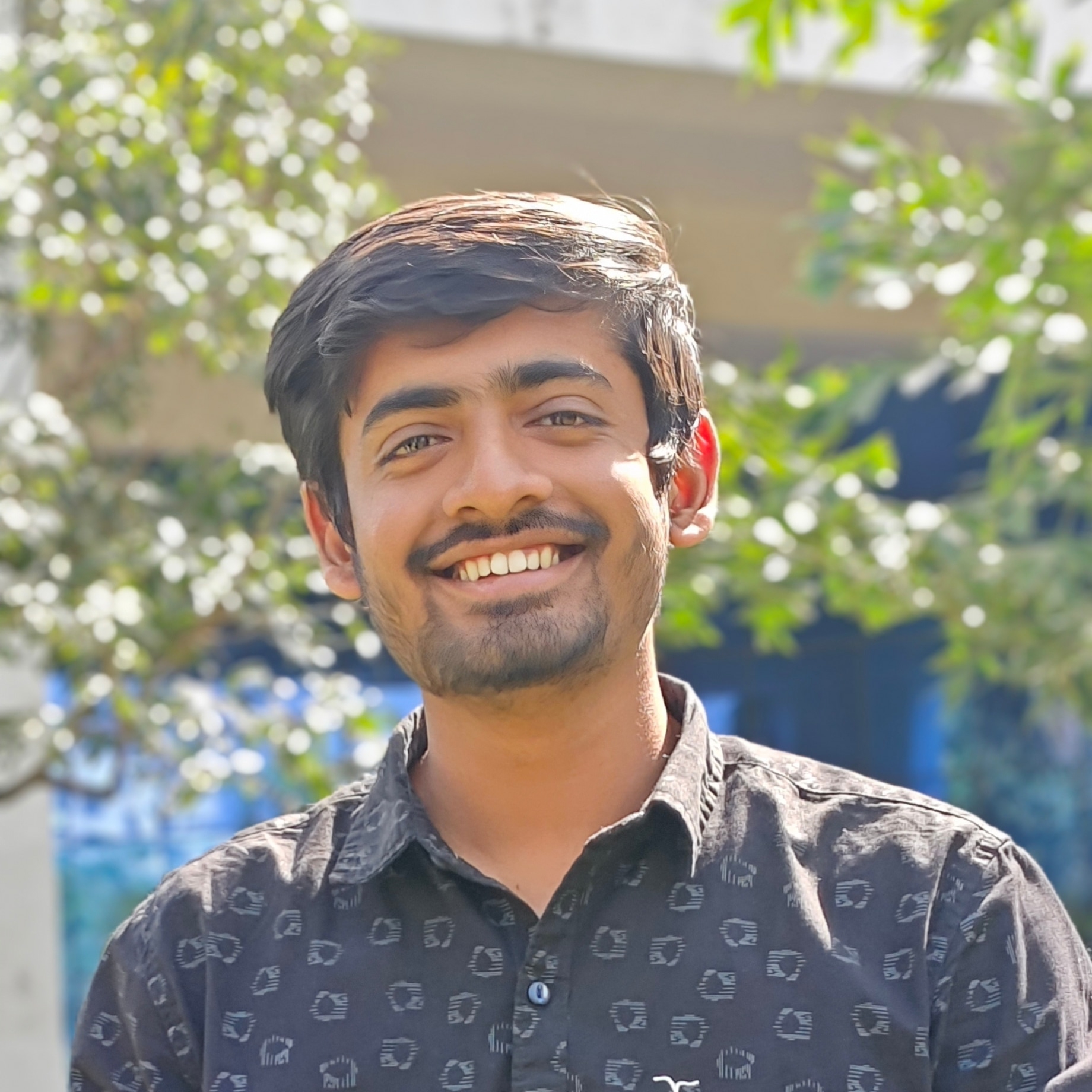
Risharth pardeshi
Risharth pardeshi
I hope this message finds you well. I am Risharth Pardeshi I am passionate about Computer Science and Engineering, and I have a strong foundation in this field. I graduated with a Bachelor of Engineering from the Laxmi Narayan College of Technology in Indore, India, in July 2024, with a CGPA of 7.98. Here is a brief overview of my technical strengths: Languages: C++, HTML, CSS, JavaScript, SQL Frameworks: React.js, Next.js, Tailwind CSS, Node.js Databases/OS: MySQL, MongoDB, Firebase, Linux Technical Skills: Data Structures and Algorithms, Git, DBMS, OS, Object-Oriented Programming, Computer Networking, Vercel, Postman