Use Cases of Python Dictionaries Data Structures

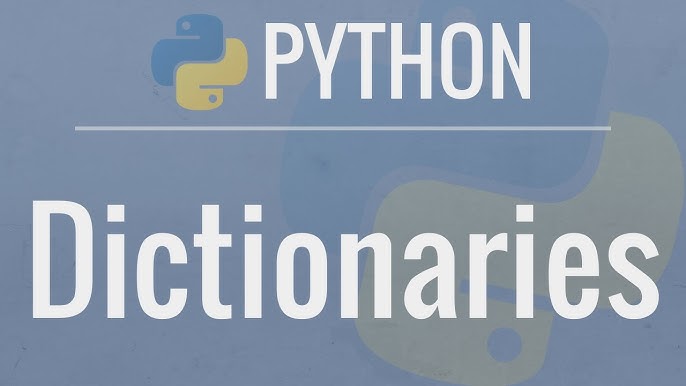
What is Python Dictionaries and how it works?
A Python dictionary is a data structure that allows us to easily write very efficient code. In many other languages, this data structure is called a hash table because its keys are hashable. We'll understand in a bit what this means.
A Python dictionary is a collection of key:value pairs. You can think about them as words and their meaning in an ordinary dictionary. Values are said to be mapped to keys. For example, in a physical dictionary, the definition science that searches for patterns in complex data using computer methods is mapped to the key Data Science.
Simple examples of how dictionaries can be used
Example of Student Enrollment
# Example of Student Enrollment data dictionaries
student_enrollment = {
"name" : "Guko",
"year_level" : "Grade 11 Senior High School",
"number" : "639912131321",
"lrn" : "223501140045",
"age" : 17,
"sex" : "Male",
"address" : "Caloocan City",
"is_fully_paid" : True
}
# Print the name Student Enrollment
print(student_enrollment["name"])
Tips on how to use dictionaries effectively
- When getting the value pairs inside the python dictionary use the
get()
method to avoid error when key is not available
car = {
"brand": "Lamborghini",
"model": "AVENTADOR LP 780-4 ULTIMAE",
"year": "2021–2022"
}
x = car.get("model")
print(x)
- When getting key : value pairs and using for loop use the
items()
method to easily get it
car = {
"brand": "Lamborghini",
"model": "AVENTADOR LP 780-4 ULTIMAE",
"year": "2021–2022"
}
for key, value in car.items():
print(key, value)
- Also use unique key to make the dictionary be easily distinguish and will not cause redundancy of key name in dictionary
References
https://glinteco.com/en/post/python-dictionary-tips-tricks-and-best-practices/
Subscribe to my newsletter
Read articles from Cañete,Brandon L. directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
