Lazy Loading: A Simple Way to Improve Website Speed and SEO

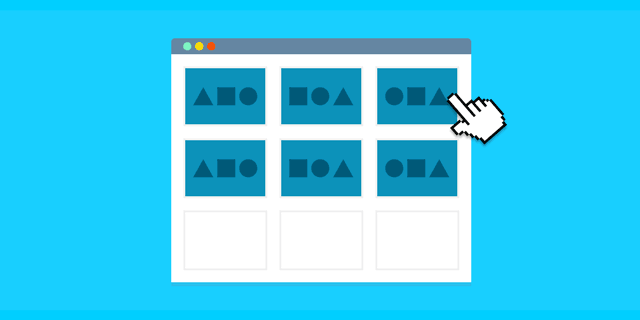
Imagine you're at a buffet, and instead of all the food arriving at once (overwhelming your plate), dishes come one at a time, just as you're ready for them. That’s exactly how lazy loading works for web content—serving resources only when needed.
In today's fast-paced digital world, website performance is paramount. Visitors expect websites to load instantly, and search engines prioritize fast-loading sites. This is where lazy loading comes in – a simple yet powerful technique to boost your site's speed and improve search engine optimization (SEO). But what exactly is lazy loading, and why should you implement it? Let’s explore.
How Lazy Loading Speeds Up Your Website and Improves SEO
Lazy loading defers the loading of non-critical resources, such as images or videos, until they're needed. Instead of loading everything at once when the page loads, resources are only fetched as the user scrolls to them. This technique significantly reduces initial load times and bandwidth consumption, leading to better user experiences and improved SEO rankings.
When your website loads faster, it not only enhances user experience but also aligns with Google’s Core Web Vitals, which measure load performance as a key factor for SEO. A faster site means lower bounce rates and higher engagement, both of which contribute to better search rankings.
Boost Website Speed with Lazy Loading for Better SEO
The impact of lazy loading is most noticeable on content-heavy websites – especially those with a large number of images, videos, or external resources. For instance, when images and media files are lazily loaded, the initial page load time decreases dramatically because the browser doesn’t need to load everything at once.
This has a direct effect on SEO. Google’s algorithms reward fast-loading pages with higher rankings. Moreover, faster websites provide a better experience, keeping users engaged longer, which reduces bounce rates – a key metric in SEO performance. Lazy loading can thus lead to improved visibility on search engine results pages (SERPs) and increased traffic over time.
Why Lazy Loading is Key to Faster Websites and Higher SEO Rankings
Lazy loading isn't just a fancy trick to improve loading times – it’s a necessity in modern web development. As websites grow in complexity, loading large media files all at once puts a heavy strain on your server and can overwhelm users on slower connections.
By implementing lazy loading, you ensure that resources like images, videos, and iframes are only loaded when they are needed, thereby lightening the load on the browser. This reduces the time to interactive (TTI), which is a crucial metric in determining how quickly a user can interact with your site. With better performance, your site is more likely to rank higher in search results, making lazy loading a key factor in boosting your SEO.
Here’s how you can easily implement lazy loading on your website:
- Native Lazy Loading: For images, the simplest way is using the
loading="lazy"
attribute directly in the HTML<img>
tag. This tells the browser to defer loading the image until it’s in the user’s viewport.
<img src="example.jpg" alt="example image" loading="lazy" />
JavaScript-based Lazy Loading: For more control or for elements like videos or background images, you can use JavaScript libraries such as
lazysizes
or implement your own Intersection Observer API. In this guide, we’ll be implementing our own intersection Observer API.Step-by-Step Guide to Implementing JavaScript-Based Lazy Loading
1. Defining Image Placeholders
We'll use two versions of the same image: a low-resolution image that is loaded by default and a high-resolution image that will load once the image comes into view on the screen. This approach enhances user experience by showing an image quickly, even if it's a low-quality one, while the higher-quality image loads in the background.
Here’s an example of how we structure the HTML:
<img
class="lazy"
src="low-resolution.png" <!-- default low-res image -->
data-src="high-resolution.png" <!-- high-res image to load when in view -->
alt="Descriptive Alt Text"
/>
class="lazy"
: This class will be used to apply a blur effect to the image initially, giving it a subtle appearance while the high-res image loads.src="low-resolution.png"
: This is the default low-resolution image that loads initially.data-src="high-resolution.png"
: This is where we store the URL of the high-resolution image that we want to load when the image is in view.
2. Applying a Blur Filter for Smooth Transitions
To make the transition between the low-res and high-res images less jarring, we apply a blur filter to the lazy
class. This filter will be removed once the high-resolution image is loaded.
.lazy {
filter: blur(20px); /* Blurs the image until the high-res version is loaded */
}
This line selects all img
elements in the document that have the data-src
attribute, which contains the URL for the high-resolution image. We store these selected images in the imgTarget
variable for further processing.
Step 2: Define the Intersection Observer Callback
The IntersectionObserver API calls a function whenever a target element (in this case, an image) enters or exits the viewport. This function is called for each observed target and receives two important parameters: entries
(which represents the elements being observed) and observer
(the instance of the IntersectionObserver).
Here’s the callback function we use:
const loading = function (entries, observer) {
const [entry] = entries; // Destructuring the first entry (an image being observed)
if (!entry.isIntersecting) return; // If the image is not in the viewport, return early
// Replace the low-res image with the high-res image
entry.target.src = entry.target.dataset.src;
// Once the high-res image is fully loaded, remove the "lazy" class
entry.target.addEventListener("load", () => {
entry.target.classList.remove("lazy");
});
// Stop observing the image once it has been loaded
observer.unobserve(entry.target);
};
const [entry] = entries;
: Theentries
array contains all the observed images, but since we only care about the first image in view at any given time, we destructure the firstentry
for simplicity.if (!entry.isIntersecting) return;
: We only want to load the high-res image when it becomes visible in the viewport (isIntersecting === true
). If it's not visible, we do nothing.entry.target
.src =
entry.target
.dataset.src;
: Once the image is in view, we swap thesrc
(which initially contains the low-res image) with thedata-src
(the high-res image).entry.target
.addEventListener("load", ...)
: After replacing the imagesrc
, we listen for theload
event to ensure the high-res image has finished loading before removing thelazy
class, which removes the blur filter.observer.unobserve(
entry.target
);
: Once the image has been loaded, we stop observing it to prevent unnecessary callbacks.
Step 3: Initialize the IntersectionObserver
Now that the callback function loading
is defined, we can initialize the observer and set its options.
const imgObserver = new IntersectionObserver(loading, {
root: null, // Use the browser viewport as the root
threshold: 0.1, // Image needs to be 10% visible to trigger the callback
});
root: null
: This tells the observer to use the viewport (the visible part of the browser window) as the root.threshold: 0.1
: This means the observer will trigger when 10% of the image is visible in the viewport. Adjust this value depending on when you want the image to start loading.
Step 4: Observe Each Image
Finally, we instruct the observer to start observing each image from our imgTarget
collection.
imgTarget.forEach((img) => imgObserver.observe(img));
This loops over each image in the imgTarget
list and tells the observer to track it. When any of the images cross the visibility threshold, the loading
function will execute.
Putting It All Together
Here’s the full code for lazy loading images with the Intersection Observer API:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Lazy Loading Images</title>
<style>
.lazy {
filter: blur(20px); /* Blur effect for lazy-loaded images */
transition: filter 0.5s; /* Smooth transition when the blur is removed */
}
</style>
</head>
<body>
<img class="lazy" src="low-res1.jpg" data-src="high-res1.jpg" alt="Image 1" />
<img class="lazy" src="low-res2.jpg" data-src="high-res2.jpg" alt="Image 2" />
<img class="lazy" src="low-res3.jpg" data-src="high-res3.jpg" alt="Image 3" />
<script>
const imgTarget = document.querySelectorAll("img[data-src]");
const loading = function (entries, observer) {
const [entry] = entries;
if (!entry.isIntersecting) return;
entry.target.src = entry.target.dataset.src;
entry.target.addEventListener("load", () => {
entry.target.classList.remove("lazy");
});
observer.unobserve(entry.target);
};
const imgObserver = new IntersectionObserver(loading, {
root: null,
threshold: 0.1,
});
imgTarget.forEach((img) => imgObserver.observe(img));
</script>
</body>
</html>
- Framework-Specific Implementations: Popular web development frameworks like Reactjs, this framework also offer lazy loading solutions using React’s built-in
React.lazy()
function.
Lazy Loading in React with React.lazy()
In React, you can implement lazy loading of components using the built-in React.lazy()
function. This method is particularly useful when dealing with large components or libraries that are not needed during the initial render, deferring their loading until the user navigates to a part of the application where they are required.
How It Works:
Instead of importing all components upfront, you can dynamically load them when necessary. This can help reduce the initial bundle size and speed up the loading of your app.
Here’s a basic example of how to use React.lazy()
:
import React, { Suspense } from "react";
// Lazily load the component
const LazyComponent = React.lazy(() => import("./LazyComponent"));
function App() {
return (
<div className="App">
<h1>Main App Component</h1>
{/* Wrap the lazy-loaded component in Suspense and provide a fallback UI */}
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
export default App;
React.lazy(() => import('./LazyComponent'))
: This line tells React to loadLazyComponent
dynamically when it is needed. Theimport()
function is asynchronous, meaning it will fetch the component only when required.<Suspense fallback={<div>Loading...</div>}>
: Since the lazy component takes time to load, React needs a fallback UI (like a loading spinner or message). TheSuspense
component helps with this by displaying the fallback while the component is being loaded.Dynamic Importing: The
import('./LazyComponent')
function dynamically imports the module, allowing you to only fetch the code forLazyComponent
when the user navigates to it.
In an age where website speed is directly tied to SEO rankings and user satisfaction, lazy loading is an essential tool for developers and website owners. By delaying the loading of non-critical resources, you can drastically improve performance, lower bounce rates, and boost search rankings. Whether you’re working with a small blog or a large-scale e-commerce site, implementing lazy loading can make a significant difference in how users and search engines perceive your site.
So, if you haven't already implemented lazy loading, now is the time to start. By optimizing your site's performance, you’ll not only provide a better experience for your users but also stand a better chance of ranking higher in search engine results.
Subscribe to my newsletter
Read articles from ayoola lekan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ayoola lekan
ayoola lekan
Frontend Developer | Article Writer